
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
md.c
00001 /** 00002 * \file md.c 00003 * 00004 * \brief Generic message digest wrapper for mbed TLS 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00009 * 00010 * This file is part of mbed TLS (https://tls.mbed.org) 00011 * 00012 * This program is free software; you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation; either version 2 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License along 00023 * with this program; if not, write to the Free Software Foundation, Inc., 00024 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00025 */ 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "polarssl/config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #if defined(POLARSSL_MD_C) 00034 00035 #include "polarssl/md.h" 00036 #include "polarssl/md_wrap.h" 00037 00038 #include <stdlib.h> 00039 #include <string.h> 00040 00041 #if defined(_MSC_VER) && !defined strcasecmp && !defined(EFIX64) && \ 00042 !defined(EFI32) 00043 #define strcasecmp _stricmp 00044 #endif 00045 00046 /* Implementation that should never be optimized out by the compiler */ 00047 static void polarssl_zeroize( void *v, size_t n ) { 00048 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00049 } 00050 00051 static const int supported_digests[] = { 00052 00053 #if defined(POLARSSL_SHA512_C) 00054 POLARSSL_MD_SHA512, 00055 POLARSSL_MD_SHA384, 00056 #endif 00057 00058 #if defined(POLARSSL_SHA256_C) 00059 POLARSSL_MD_SHA256, 00060 POLARSSL_MD_SHA224, 00061 #endif 00062 00063 #if defined(POLARSSL_SHA1_C) 00064 POLARSSL_MD_SHA1, 00065 #endif 00066 00067 #if defined(POLARSSL_RIPEMD160_C) 00068 POLARSSL_MD_RIPEMD160, 00069 #endif 00070 00071 #if defined(POLARSSL_MD5_C) 00072 POLARSSL_MD_MD5, 00073 #endif 00074 00075 #if defined(POLARSSL_MD4_C) 00076 POLARSSL_MD_MD4, 00077 #endif 00078 00079 #if defined(POLARSSL_MD2_C) 00080 POLARSSL_MD_MD2, 00081 #endif 00082 00083 POLARSSL_MD_NONE 00084 }; 00085 00086 const int *md_list( void ) 00087 { 00088 return( supported_digests ); 00089 } 00090 00091 const md_info_t *md_info_from_string( const char *md_name ) 00092 { 00093 if( NULL == md_name ) 00094 return( NULL ); 00095 00096 /* Get the appropriate digest information */ 00097 #if defined(POLARSSL_MD2_C) 00098 if( !strcasecmp( "MD2", md_name ) ) 00099 return md_info_from_type( POLARSSL_MD_MD2 ); 00100 #endif 00101 #if defined(POLARSSL_MD4_C) 00102 if( !strcasecmp( "MD4", md_name ) ) 00103 return md_info_from_type( POLARSSL_MD_MD4 ); 00104 #endif 00105 #if defined(POLARSSL_MD5_C) 00106 if( !strcasecmp( "MD5", md_name ) ) 00107 return md_info_from_type( POLARSSL_MD_MD5 ); 00108 #endif 00109 #if defined(POLARSSL_RIPEMD160_C) 00110 if( !strcasecmp( "RIPEMD160", md_name ) ) 00111 return md_info_from_type( POLARSSL_MD_RIPEMD160 ); 00112 #endif 00113 #if defined(POLARSSL_SHA1_C) 00114 if( !strcasecmp( "SHA1", md_name ) || !strcasecmp( "SHA", md_name ) ) 00115 return md_info_from_type( POLARSSL_MD_SHA1 ); 00116 #endif 00117 #if defined(POLARSSL_SHA256_C) 00118 if( !strcasecmp( "SHA224", md_name ) ) 00119 return md_info_from_type( POLARSSL_MD_SHA224 ); 00120 if( !strcasecmp( "SHA256", md_name ) ) 00121 return md_info_from_type( POLARSSL_MD_SHA256 ); 00122 #endif 00123 #if defined(POLARSSL_SHA512_C) 00124 if( !strcasecmp( "SHA384", md_name ) ) 00125 return md_info_from_type( POLARSSL_MD_SHA384 ); 00126 if( !strcasecmp( "SHA512", md_name ) ) 00127 return md_info_from_type( POLARSSL_MD_SHA512 ); 00128 #endif 00129 return( NULL ); 00130 } 00131 00132 const md_info_t *md_info_from_type( md_type_t md_type ) 00133 { 00134 switch( md_type ) 00135 { 00136 #if defined(POLARSSL_MD2_C) 00137 case POLARSSL_MD_MD2: 00138 return( &md2_info ); 00139 #endif 00140 #if defined(POLARSSL_MD4_C) 00141 case POLARSSL_MD_MD4: 00142 return( &md4_info ); 00143 #endif 00144 #if defined(POLARSSL_MD5_C) 00145 case POLARSSL_MD_MD5: 00146 return( &md5_info ); 00147 #endif 00148 #if defined(POLARSSL_RIPEMD160_C) 00149 case POLARSSL_MD_RIPEMD160: 00150 return( &ripemd160_info ); 00151 #endif 00152 #if defined(POLARSSL_SHA1_C) 00153 case POLARSSL_MD_SHA1: 00154 return( &sha1_info ); 00155 #endif 00156 #if defined(POLARSSL_SHA256_C) 00157 case POLARSSL_MD_SHA224: 00158 return( &sha224_info ); 00159 case POLARSSL_MD_SHA256: 00160 return( &sha256_info ); 00161 #endif 00162 #if defined(POLARSSL_SHA512_C) 00163 case POLARSSL_MD_SHA384: 00164 return( &sha384_info ); 00165 case POLARSSL_MD_SHA512: 00166 return( &sha512_info ); 00167 #endif 00168 default: 00169 return( NULL ); 00170 } 00171 } 00172 00173 void md_init( md_context_t *ctx ) 00174 { 00175 memset( ctx, 0, sizeof( md_context_t ) ); 00176 } 00177 00178 void md_free( md_context_t *ctx ) 00179 { 00180 if( ctx == NULL ) 00181 return; 00182 00183 if( ctx->md_ctx ) 00184 ctx->md_info->ctx_free_func( ctx->md_ctx ); 00185 00186 polarssl_zeroize( ctx, sizeof( md_context_t ) ); 00187 } 00188 00189 int md_init_ctx( md_context_t *ctx, const md_info_t *md_info ) 00190 { 00191 if( md_info == NULL || ctx == NULL ) 00192 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00193 00194 memset( ctx, 0, sizeof( md_context_t ) ); 00195 00196 if( ( ctx->md_ctx = md_info->ctx_alloc_func() ) == NULL ) 00197 return( POLARSSL_ERR_MD_ALLOC_FAILED ); 00198 00199 ctx->md_info = md_info; 00200 00201 md_info->starts_func( ctx->md_ctx ); 00202 00203 return( 0 ); 00204 } 00205 00206 #if ! defined(POLARSSL_DEPRECATED_REMOVED) 00207 int md_free_ctx( md_context_t *ctx ) 00208 { 00209 md_free( ctx ); 00210 00211 return( 0 ); 00212 } 00213 #endif 00214 00215 int md_starts( md_context_t *ctx ) 00216 { 00217 if( ctx == NULL || ctx->md_info == NULL ) 00218 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00219 00220 ctx->md_info->starts_func( ctx->md_ctx ); 00221 00222 return( 0 ); 00223 } 00224 00225 int md_update( md_context_t *ctx, const unsigned char *input, size_t ilen ) 00226 { 00227 if( ctx == NULL || ctx->md_info == NULL ) 00228 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00229 00230 ctx->md_info->update_func( ctx->md_ctx, input, ilen ); 00231 00232 return( 0 ); 00233 } 00234 00235 int md_finish( md_context_t *ctx, unsigned char *output ) 00236 { 00237 if( ctx == NULL || ctx->md_info == NULL ) 00238 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00239 00240 ctx->md_info->finish_func( ctx->md_ctx, output ); 00241 00242 return( 0 ); 00243 } 00244 00245 int md( const md_info_t *md_info, const unsigned char *input, size_t ilen, 00246 unsigned char *output ) 00247 { 00248 if( md_info == NULL ) 00249 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00250 00251 md_info->digest_func( input, ilen, output ); 00252 00253 return( 0 ); 00254 } 00255 00256 int md_file( const md_info_t *md_info, const char *path, unsigned char *output ) 00257 { 00258 #if defined(POLARSSL_FS_IO) 00259 int ret; 00260 #endif 00261 00262 if( md_info == NULL ) 00263 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00264 00265 #if defined(POLARSSL_FS_IO) 00266 ret = md_info->file_func( path, output ); 00267 if( ret != 0 ) 00268 return( POLARSSL_ERR_MD_FILE_IO_ERROR + ret ); 00269 00270 return( ret ); 00271 #else 00272 ((void) path); 00273 ((void) output); 00274 00275 return( POLARSSL_ERR_MD_FEATURE_UNAVAILABLE ); 00276 #endif /* POLARSSL_FS_IO */ 00277 } 00278 00279 int md_hmac_starts( md_context_t *ctx, const unsigned char *key, size_t keylen ) 00280 { 00281 if( ctx == NULL || ctx->md_info == NULL ) 00282 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00283 00284 ctx->md_info->hmac_starts_func( ctx->md_ctx, key, keylen ); 00285 00286 return( 0 ); 00287 } 00288 00289 int md_hmac_update( md_context_t *ctx, const unsigned char *input, size_t ilen ) 00290 { 00291 if( ctx == NULL || ctx->md_info == NULL ) 00292 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00293 00294 ctx->md_info->hmac_update_func( ctx->md_ctx, input, ilen ); 00295 00296 return( 0 ); 00297 } 00298 00299 int md_hmac_finish( md_context_t *ctx, unsigned char *output ) 00300 { 00301 if( ctx == NULL || ctx->md_info == NULL ) 00302 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00303 00304 ctx->md_info->hmac_finish_func( ctx->md_ctx, output ); 00305 00306 return( 0 ); 00307 } 00308 00309 int md_hmac_reset( md_context_t *ctx ) 00310 { 00311 if( ctx == NULL || ctx->md_info == NULL ) 00312 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00313 00314 ctx->md_info->hmac_reset_func( ctx->md_ctx ); 00315 00316 return( 0 ); 00317 } 00318 00319 int md_hmac( const md_info_t *md_info, const unsigned char *key, size_t keylen, 00320 const unsigned char *input, size_t ilen, 00321 unsigned char *output ) 00322 { 00323 if( md_info == NULL ) 00324 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00325 00326 md_info->hmac_func( key, keylen, input, ilen, output ); 00327 00328 return( 0 ); 00329 } 00330 00331 int md_process( md_context_t *ctx, const unsigned char *data ) 00332 { 00333 if( ctx == NULL || ctx->md_info == NULL ) 00334 return( POLARSSL_ERR_MD_BAD_INPUT_DATA ); 00335 00336 ctx->md_info->process_func( ctx->md_ctx, data ); 00337 00338 return( 0 ); 00339 } 00340 00341 #endif /* POLARSSL_MD_C */ 00342
Generated on Tue Jul 12 2022 22:22:38 by
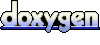