
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
fPtr_func.cpp
00001 /* 00002 * - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 #include "simplelink/cc3100_simplelink.h" 00038 00039 #include "simplelink/cc3100.h" 00040 #include "simplelink/cc3100_driver.h" 00041 #include "simplelink/G_functions/fPtr_func.h" 00042 #include "simplelink/cc3100_nonos.h" 00043 #include "cli_uart.h" 00044 #include "Led_config.h" 00045 00046 #include "cc3100_trace.h" 00047 #include "myBoardInit.h" 00048 00049 using namespace mbed_cc3100; 00050 00051 cc3100 _cc3100_(NC, NC, PD_12, PD_13, PD_11, SPI(PC_3, PC_2, PB_10));//Seeed_Arch_Max irq, nHib, cs, mosi, miso, sck 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 unsigned char POST_token[] = "__SL_P_ULD"; 00058 unsigned char GET_token[] = "__SL_G_ULD"; 00059 00060 00061 #define PRINT_BUF_LEN 128 00062 extern int8_t print_buf[PRINT_BUF_LEN]; 00063 00064 #define LED_STRING "LED" 00065 #define LED1_STRING "LED1_" 00066 #define LED2_STRING ",LED2_" 00067 #define LED_ON_STRING "ON" 00068 #define LED_OFF_STRING "OFF" 00069 00070 /* General Events handling*/ 00071 #if defined (EXT_LIB_REGISTERED_GENERAL_EVENTS) 00072 00073 typedef _SlEventPropogationStatus_e (*general_callback) (SlDeviceEvent_t *); 00074 00075 static const general_callback general_callbacks[] = 00076 { 00077 #ifdef SlExtLib1GeneralEventHandler 00078 SlExtLib1GeneralEventHandler, 00079 #endif 00080 00081 #ifdef SlExtLib2GeneralEventHandler 00082 SlExtLib2GeneralEventHandler, 00083 #endif 00084 00085 #ifdef SlExtLib3GeneralEventHandler 00086 SlExtLib3GeneralEventHandler, 00087 #endif 00088 00089 #ifdef SlExtLib4GeneralEventHandler 00090 SlExtLib4GeneralEventHandler, 00091 #endif 00092 00093 #ifdef SlExtLib5GeneralEventHandler 00094 SlExtLib5GeneralEventHandler, 00095 #endif 00096 }; 00097 00098 #undef _SlDrvHandleGeneralEvents 00099 00100 /******************************************************************** 00101 _SlDrvHandleGeneralEvents 00102 Iterates through all the general(device) event handlers which are 00103 registered by the external libs/user application. 00104 *********************************************************************/ 00105 void _SlDrvHandleGeneralEvents(SlDeviceEvent_t *slGeneralEvent) 00106 { 00107 uint8_t i; 00108 00109 /* Iterate over all the extenal libs handlers */ 00110 for ( i = 0 ; i < sizeof(general_callbacks)/sizeof(general_callbacks[0]) ; i++ ) 00111 { 00112 if (EVENT_PROPAGATION_BLOCK == general_callbacks[i](slGeneralEvent) ) 00113 { 00114 /* exit immediately and do not call the user specific handler as well */ 00115 return; 00116 } 00117 } 00118 00119 /* At last call the Application specific handler if registered */ 00120 #ifdef sl_GeneralEvtHdlr 00121 sl_GeneralEvtHdlr(slGeneralEvent); 00122 #endif 00123 00124 } 00125 #endif 00126 00127 00128 00129 /* WLAN Events handling*/ 00130 00131 #if defined (EXT_LIB_REGISTERED_WLAN_EVENTS) 00132 00133 typedef _SlEventPropogationStatus_e (*wlan_callback) (SlWlanEvent_t *); 00134 00135 static wlan_callback wlan_callbacks[] = 00136 { 00137 #ifdef SlExtLib1WlanEventHandler 00138 SlExtLib1WlanEventHandler, 00139 #endif 00140 00141 #ifdef SlExtLib2WlanEventHandler 00142 SlExtLib2WlanEventHandler, 00143 #endif 00144 00145 #ifdef SlExtLib3WlanEventHandler 00146 SlExtLib3WlanEventHandler, 00147 #endif 00148 00149 #ifdef SlExtLib4WlanEventHandler 00150 SlExtLib4WlanEventHandler, 00151 #endif 00152 00153 #ifdef SlExtLib5WlanEventHandler 00154 SlExtLib5WlanEventHandler, 00155 #endif 00156 }; 00157 00158 #undef _SlDrvHandleWlanEvents 00159 00160 /*********************************************************** 00161 _SlDrvHandleWlanEvents 00162 Iterates through all the wlan event handlers which are 00163 registered by the external libs/user application. 00164 ************************************************************/ 00165 void _SlDrvHandleWlanEvents(SlWlanEvent_t *slWlanEvent) 00166 { 00167 uint8_t i; 00168 00169 /* Iterate over all the extenal libs handlers */ 00170 for ( i = 0 ; i < sizeof(wlan_callbacks)/sizeof(wlan_callbacks[0]) ; i++ ) 00171 { 00172 if ( EVENT_PROPAGATION_BLOCK == wlan_callbacks[i](slWlanEvent) ) 00173 { 00174 /* exit immediately and do not call the user specific handler as well */ 00175 return; 00176 } 00177 } 00178 00179 /* At last call the Application specific handler if registered */ 00180 #ifdef sl_WlanEvtHdlr 00181 sl_WlanEvtHdlr(slWlanEvent); 00182 #endif 00183 00184 } 00185 #endif 00186 00187 00188 /* NetApp Events handling */ 00189 #if defined (EXT_LIB_REGISTERED_NETAPP_EVENTS) 00190 00191 typedef _SlEventPropogationStatus_e (*netApp_callback) (SlNetAppEvent_t *); 00192 00193 static const netApp_callback netApp_callbacks[] = 00194 { 00195 #ifdef SlExtLib1NetAppEventHandler 00196 SlExtLib1NetAppEventHandler, 00197 #endif 00198 00199 #ifdef SlExtLib2NetAppEventHandler 00200 SlExtLib2NetAppEventHandler, 00201 #endif 00202 00203 #ifdef SlExtLib3NetAppEventHandler 00204 SlExtLib3NetAppEventHandler, 00205 #endif 00206 00207 #ifdef SlExtLib4NetAppEventHandler 00208 SlExtLib4NetAppEventHandler, 00209 #endif 00210 00211 #ifdef SlExtLib5NetAppEventHandler 00212 SlExtLib5NetAppEventHandler, 00213 #endif 00214 }; 00215 00216 #undef _SlDrvHandleNetAppEvents 00217 00218 /************************************************************ 00219 _SlDrvHandleNetAppEvents 00220 Iterates through all the net app event handlers which are 00221 registered by the external libs/user application. 00222 ************************************************************/ 00223 void _SlDrvHandleNetAppEvents(SlNetAppEvent_t *slNetAppEvent) 00224 { 00225 uint8_t i; 00226 00227 /* Iterate over all the extenal libs handlers */ 00228 for ( i = 0 ; i < sizeof(netApp_callbacks)/sizeof(netApp_callbacks[0]) ; i++ ) 00229 { 00230 if (EVENT_PROPAGATION_BLOCK == netApp_callbacks[i](slNetAppEvent) ) 00231 { 00232 /* exit immediately and do not call the user specific handler as well */ 00233 return; 00234 } 00235 } 00236 00237 /* At last call the Application specific handler if registered */ 00238 #ifdef sl_NetAppEvtHdlr 00239 sl_NetAppEvtHdlr(slNetAppEvent); 00240 #endif 00241 00242 } 00243 #endif 00244 00245 00246 /* Http Server Events handling */ 00247 #if defined (EXT_LIB_REGISTERED_HTTP_SERVER_EVENTS) 00248 00249 typedef _SlEventPropogationStatus_e (*httpServer_callback) (SlHttpServerEvent_t*, SlHttpServerResponse_t*); 00250 00251 static const httpServer_callback httpServer_callbacks[] = 00252 { 00253 #ifdef SlExtLib1HttpServerEventHandler 00254 SlExtLib1HttpServerEventHandler, 00255 #endif 00256 00257 #ifdef SlExtLib2HttpServerEventHandler 00258 SlExtLib2HttpServerEventHandler, 00259 #endif 00260 00261 #ifdef SlExtLib3HttpServerEventHandler 00262 SlExtLib3HttpServerEventHandler, 00263 #endif 00264 00265 #ifdef SlExtLib4HttpServerEventHandler 00266 SlExtLib4HttpServerEventHandler, 00267 #endif 00268 00269 #ifdef SlExtLib5HttpServerEventHandler 00270 SlExtLib5HttpServerEventHandler, 00271 #endif 00272 }; 00273 00274 #undef _SlDrvHandleHttpServerEvents 00275 00276 /******************************************************************* 00277 _SlDrvHandleHttpServerEvents 00278 Iterates through all the http server event handlers which are 00279 registered by the external libs/user application. 00280 ********************************************************************/ 00281 void _SlDrvHandleHttpServerEvents(SlHttpServerEvent_t *slHttpServerEvent, SlHttpServerResponse_t *slHttpServerResponse) 00282 { 00283 _u8 i; 00284 00285 /* Iterate over all the external libs handlers */ 00286 for ( i = 0 ; i < sizeof(httpServer_callbacks)/sizeof(httpServer_callbacks[0]) ; i++ ) 00287 { 00288 if ( EVENT_PROPAGATION_BLOCK == httpServer_callbacks[i](slHttpServerEvent, slHttpServerResponse) ) 00289 { 00290 /* exit immediately and do not call the user specific handler as well */ 00291 return; 00292 } 00293 } 00294 00295 /* At last call the Application specific handler if registered */ 00296 #ifdef sl_HttpServerCallback 00297 sl_HttpServerCallback(slHttpServerEvent, slHttpServerResponse); 00298 #endif 00299 00300 } 00301 #endif 00302 00303 00304 /* Socket Events */ 00305 #if defined (EXT_LIB_REGISTERED_SOCK_EVENTS) 00306 00307 typedef _SlEventPropogationStatus_e (*sock_callback) (SlSockEvent_t *); 00308 00309 static const sock_callback sock_callbacks[] = 00310 { 00311 #ifdef SlExtLib1SockEventHandler 00312 SlExtLib1SockEventHandler, 00313 #endif 00314 00315 #ifdef SlExtLib2SockEventHandler 00316 SlExtLib2SockEventHandler, 00317 #endif 00318 00319 #ifdef SlExtLib3SockEventHandler 00320 SlExtLib3SockEventHandler, 00321 #endif 00322 00323 #ifdef SlExtLib4SockEventHandler 00324 SlExtLib4SockEventHandler, 00325 #endif 00326 00327 #ifdef SlExtLib5SockEventHandler 00328 SlExtLib5SockEventHandler, 00329 #endif 00330 }; 00331 00332 /************************************************************* 00333 _SlDrvHandleSockEvents 00334 Iterates through all the socket event handlers which are 00335 registered by the external libs/user application. 00336 **************************************************************/ 00337 void _SlDrvHandleSockEvents(SlSockEvent_t *slSockEvent) 00338 { 00339 uint8_t i; 00340 00341 /* Iterate over all the external libs handlers */ 00342 for ( i = 0 ; i < sizeof(sock_callbacks)/sizeof(sock_callbacks[0]) ; i++ ) 00343 { 00344 if ( EVENT_PROPAGATION_BLOCK == sock_callbacks[i](slSockEvent) ) 00345 { 00346 /* exit immediately and do not call the user specific handler as well */ 00347 return; 00348 } 00349 } 00350 00351 /* At last call the Application specific handler if registered */ 00352 #ifdef sl_SockEvtHdlr 00353 sl_SockEvtHdlr(slSockEvent); 00354 #endif 00355 00356 } 00357 00358 #endif 00359 00360 /*! 00361 \brief This function handles ping report events 00362 00363 \param[in] pPingReport holds the ping report statistics 00364 00365 \return None 00366 00367 \note 00368 00369 \warning 00370 */ 00371 void SimpleLinkPingReport(SlPingReport_t *pPingReport) 00372 { 00373 _cc3100_.SET_STATUS_BIT(g_Status, STATUS_BIT_PING_DONE); 00374 00375 if(pPingReport == NULL) 00376 Uart_Write((uint8_t *)" [PING REPORT] NULL Pointer Error\r\n"); 00377 00378 g_PingPacketsRecv = pPingReport->PacketsReceived; 00379 } 00380 00381 00382 /*******************************************************************************/ 00383 /* _sl_HandleAsync_Accept */ 00384 /*******************************************************************************/ 00385 #ifndef SL_TINY_EXT 00386 void _sl_HandleAsync_Accept(void *pVoidBuf) 00387 { 00388 _SocketAddrResponse_u *pMsgArgs = (_SocketAddrResponse_u *)_SL_RESP_ARGS_START(pVoidBuf); 00389 00390 _cc3100_._driver._SlDrvProtectionObjLockWaitForever(); 00391 00392 VERIFY_PROTOCOL(( pMsgArgs->IpV4.sd & BSD_SOCKET_ID_MASK) <= SL_MAX_SOCKETS); 00393 VERIFY_SOCKET_CB(NULL != g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs); 00394 00395 memcpy(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs, pMsgArgs,sizeof(_SocketAddrResponse_u)); 00396 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00397 00398 _cc3100_._driver._SlDrvProtectionObjUnLock(); 00399 return; 00400 } 00401 00402 /*******************************************************************************/ 00403 /* _sl_HandleAsync_Connect */ 00404 /*******************************************************************************/ 00405 void _sl_HandleAsync_Connect(void *pVoidBuf) 00406 { 00407 _SocketResponse_t *pMsgArgs = (_SocketResponse_t *)_SL_RESP_ARGS_START(pVoidBuf); 00408 00409 _cc3100_._driver._SlDrvProtectionObjLockWaitForever(); 00410 00411 VERIFY_PROTOCOL((pMsgArgs->sd & BSD_SOCKET_ID_MASK) <= SL_MAX_SOCKETS); 00412 VERIFY_SOCKET_CB(NULL != g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs); 00413 00414 ((_SocketResponse_t *)(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs))->sd = pMsgArgs->sd; 00415 ((_SocketResponse_t *)(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs))->statusOrLen = pMsgArgs->statusOrLen; 00416 00417 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00418 _cc3100_._driver._SlDrvProtectionObjUnLock(); 00419 return; 00420 } 00421 00422 /*******************************************************************************/ 00423 /* _sl_HandleAsync_Select */ 00424 /*******************************************************************************/ 00425 void _sl_HandleAsync_Select(void *pVoidBuf) 00426 { 00427 _SelectAsyncResponse_t *pMsgArgs = (_SelectAsyncResponse_t *)_SL_RESP_ARGS_START(pVoidBuf); 00428 00429 _cc3100_._driver._SlDrvProtectionObjLockWaitForever(); 00430 00431 VERIFY_SOCKET_CB(NULL != g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs); 00432 00433 memcpy(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs, pMsgArgs, sizeof(_SelectAsyncResponse_t)); 00434 00435 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00436 _cc3100_._driver._SlDrvProtectionObjUnLock(); 00437 00438 return; 00439 } 00440 00441 #endif 00442 00443 /******************************************************************************/ 00444 /* _sl_HandleAsync_DnsGetHostByName */ 00445 /******************************************************************************/ 00446 void _sl_HandleAsync_DnsGetHostByName(void *pVoidBuf) 00447 { 00448 _GetHostByNameIPv4AsyncResponse_t *pMsgArgs = (_GetHostByNameIPv4AsyncResponse_t *)_SL_RESP_ARGS_START(pVoidBuf); 00449 00450 _cc3100_._driver._SlDrvProtectionObjLockWaitForever(); 00451 00452 VERIFY_SOCKET_CB(NULL != g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs); 00453 00454 /*IPv6 */ 00455 if(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].AdditionalData & SL_NETAPP_FAMILY_MASK) { 00456 memcpy(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs, pMsgArgs, sizeof(_GetHostByNameIPv6AsyncResponse_t)); 00457 } 00458 /*IPv4 */ 00459 else 00460 { 00461 memcpy(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs, pMsgArgs, sizeof(_GetHostByNameIPv4AsyncResponse_t)); 00462 } 00463 00464 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00465 _cc3100_._driver._SlDrvProtectionObjUnLock(); 00466 00467 return; 00468 } 00469 00470 /******************************************************************************/ 00471 00472 /****************************************************************************** 00473 _sl_HandleAsync_DnsGetHostByService 00474 00475 CALLER NWP - Async event on sl_DnsGetHostByService with IPv4 Family 00476 00477 00478 DESCRIPTION: 00479 00480 Async event on sl_DnsGetHostByService command with IPv4 Family. 00481 Return service attributes like IP address, port and text according to service name. 00482 The user sets a service name Full/Part (see example below), and should get the: 00483 1. IP of the service 00484 2. The port of service. 00485 3. The text of service. 00486 00487 Hence it can make a connection to the specific service and use it. 00488 It is similar to get host by name method. 00489 00490 It is done by a single shot query with PTR type on the service name. 00491 00492 00493 00494 Note: 00495 1. The return's attributes are belonged to first service that is found. 00496 It can be other services with the same service name will response to 00497 the query. The results of these responses are saved in the peer cache of the NWP, and 00498 should be read by another API. 00499 00500 00501 PARAMETERS: 00502 00503 pVoidBuf - is point to opcode of the event. 00504 it contains the outputs that are given to the user 00505 00506 outputs description: 00507 00508 1.out_pAddr[] - output: Contain the IP address of the service. 00509 2.out_pPort - output: Contain the port of the service. 00510 3.inout_TextLen - Input: Contain the max length of the text that the user wants to get. 00511 it means that if the test of service is bigger that its value than 00512 the text is cut to inout_TextLen value. 00513 Output: Contain the length of the text that is returned. Can be full text or part 00514 of the text (see above). 00515 00516 4.out_pText - Contain the text of the service (full or part see above- inout_TextLen description). 00517 00518 * 00519 00520 00521 RETURNS: success or fail. 00522 00523 ******************************************************************************/ 00524 #ifndef SL_TINY_EXT 00525 void _sl_HandleAsync_DnsGetHostByService(void *pVoidBuf) 00526 { 00527 _GetHostByServiceAsyncResponse_t* Res; 00528 uint16_t TextLen; 00529 uint16_t UserTextLen; 00530 00531 /*pVoidBuf - is point to opcode of the event.*/ 00532 00533 /*set pMsgArgs to point to the attribute of the event.*/ 00534 _GetHostByServiceIPv4AsyncResponse_t *pMsgArgs = (_GetHostByServiceIPv4AsyncResponse_t *)_SL_RESP_ARGS_START(pVoidBuf); 00535 00536 VERIFY_SOCKET_CB(NULL != g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs); 00537 00538 /*IPv6*/ 00539 if(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].AdditionalData & SL_NETAPP_FAMILY_MASK) { 00540 return; 00541 } 00542 /*IPv4*/ 00543 else { 00544 /************************************************************************************************* 00545 00546 1. Copy the attribute part of the evnt to the attribute part of the response 00547 memcpy(g_pCB->GetHostByServiceCB.pAsyncRsp, pMsgArgs, sizeof(_GetHostByServiceIPv4AsyncResponse_t)); 00548 00549 set to TextLen the text length of the service.*/ 00550 TextLen = pMsgArgs->TextLen; 00551 00552 /*Res pointed to mDNS global object struct */ 00553 Res = (_GetHostByServiceAsyncResponse_t*)g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs; 00554 00555 00556 00557 /*It is 4 bytes so we avoid from memcpy*/ 00558 Res->out_pAddr[0] = pMsgArgs->Address; 00559 Res->out_pPort[0] = pMsgArgs->Port; 00560 Res->Status = pMsgArgs->Status; 00561 00562 /*set to TextLen the text length of the user (input fromthe user).*/ 00563 UserTextLen = Res->inout_TextLen[0]; 00564 00565 /*Cut the service text if the user requested for smaller text.*/ 00566 UserTextLen = (TextLen <= UserTextLen) ? TextLen : UserTextLen; 00567 Res->inout_TextLen[0] = UserTextLen ; 00568 00569 /************************************************************************************************** 00570 00571 2. Copy the payload part of the evnt (the text) to the payload part of the response 00572 the lenght of the copy is according to the text length in the attribute part. */ 00573 00574 00575 memcpy(Res->out_pText, 00576 (int8_t *)(& pMsgArgs[1]), /* & pMsgArgs[1] -> 1st byte after the fixed header = 1st byte of variable text.*/ 00577 UserTextLen); 00578 00579 00580 /**************************************************************************************************/ 00581 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00582 return; 00583 } 00584 } 00585 #endif 00586 00587 /*****************************************************************************/ 00588 /* _sl_HandleAsync_PingResponse */ 00589 /*****************************************************************************/ 00590 #ifndef SL_TINY_EXT 00591 void _sl_HandleAsync_PingResponse(void *pVoidBuf) 00592 { 00593 _PingReportResponse_t *pMsgArgs = (_PingReportResponse_t *)_SL_RESP_ARGS_START(pVoidBuf); 00594 SlPingReport_t pingReport; 00595 00596 if(pPingCallBackFunc) { 00597 _cc3100_._netapp.CopyPingResultsToReport(pMsgArgs,&pingReport); 00598 pPingCallBackFunc(&pingReport); 00599 } else { 00600 00601 _cc3100_._driver._SlDrvProtectionObjLockWaitForever(); 00602 VERIFY_SOCKET_CB(NULL != g_pCB->PingCB.PingAsync.pAsyncRsp); 00603 00604 if (NULL != g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs) { 00605 memcpy(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs, pMsgArgs, sizeof(_PingReportResponse_t)); 00606 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00607 } 00608 _cc3100_._driver._SlDrvProtectionObjUnLock(); 00609 } 00610 return; 00611 } 00612 #endif 00613 00614 /* ******************************************************************************/ 00615 /* _SlDrvMsgReadSpawnCtx */ 00616 /* ******************************************************************************/ 00617 _SlReturnVal_t _SlDrvMsgReadSpawnCtx(void *pValue) 00618 { 00619 00620 #ifdef SL_POLLING_MODE_USED 00621 int16_t retCode = OSI_OK; 00622 /* for polling based systems */ 00623 do { 00624 retCode = _cc3100_._nonos.sl_LockObjLock(&g_pCB->GlobalLockObj, 0); 00625 if ( OSI_OK != retCode ) { 00626 if (TRUE == g_pCB->IsCmdRespWaited) { 00627 OSI_RET_OK_CHECK( _cc3100_._nonos.sl_SyncObjSignal(&g_pCB->CmdSyncObj) ); 00628 return SL_RET_CODE_OK; 00629 } 00630 } 00631 00632 } while (OSI_OK != retCode); 00633 00634 #else 00635 //#ifndef SL_PLATFORM_MULTI_THREADED 00636 #if (!defined (SL_PLATFORM_MULTI_THREADED)) && (!defined (SL_PLATFORM_EXTERNAL_SPAWN)) 00637 _cc3100_._driver._SlDrvObjLockWaitForever(&g_pCB->GlobalLockObj); 00638 #else 00639 OSI_RET_OK_CHECK(sl_LockObjLock(&g_pCB->GlobalLockObj, SL_OS_WAIT_FOREVER) ); 00640 #endif 00641 #endif 00642 g_pCB->FunctionParams.AsyncExt.pAsyncBuf = NULL;/* buffer must be allocated by _SlDrvMsgRead */ 00643 g_pCB->FunctionParams.AsyncExt.AsyncEvtHandler= NULL; 00644 g_pCB->FunctionParams.AsyncExt.RxMsgClass = CMD_RESP_CLASS;/* init to illegal value and verify it's overwritten with the valid one */ 00645 00646 /* Messages might have been read by CmdResp context. Therefore after */ 00647 /* getting LockObj, check again where the Pending Rx Msg is still present. */ 00648 if(FALSE == (_cc3100_._driver._SL_PENDING_RX_MSG(g_pCB))) { 00649 //#ifndef SL_PLATFORM_MULTI_THREADED 00650 #if (!defined (SL_PLATFORM_MULTI_THREADED)) && (!defined (SL_PLATFORM_EXTERNAL_SPAWN)) 00651 _cc3100_._driver._SlDrvObjUnLock(&g_pCB->GlobalLockObj); 00652 #else 00653 OSI_RET_OK_CHECK(sl_LockObjUnlock(&g_pCB->GlobalLockObj)); 00654 #endif 00655 return SL_RET_CODE_OK; 00656 } 00657 00658 VERIFY_RET_OK(_cc3100_._driver._SlDrvMsgRead()); 00659 00660 g_pCB->RxDoneCnt++; 00661 00662 switch(g_pCB->FunctionParams.AsyncExt.RxMsgClass) { 00663 case ASYNC_EVT_CLASS: 00664 /* If got here and protected by LockObj a message is waiting */ 00665 /* to be read */ 00666 VERIFY_PROTOCOL(NULL != g_pCB->FunctionParams.AsyncExt.pAsyncBuf); 00667 00668 _cc3100_._driver._SlAsyncEventGenericHandler(); 00669 00670 #if (SL_MEMORY_MGMT == SL_MEMORY_MGMT_STATIC) 00671 g_pCB->FunctionParams.AsyncExt.pAsyncBuf = NULL; 00672 #else 00673 free(g_pCB->FunctionParams.AsyncExt.pAsyncBuf); 00674 #endif 00675 break; 00676 case DUMMY_MSG_CLASS: 00677 case RECV_RESP_CLASS: 00678 /* These types are legal in this context. Do nothing */ 00679 break; 00680 case CMD_RESP_CLASS: 00681 /* Command response is illegal in this context. */ 00682 /* No 'break' here: Assert! */ 00683 default: 00684 VERIFY_PROTOCOL(0); 00685 } 00686 //#ifndef SL_PLATFORM_MULTI_THREADED 00687 #if (!defined (SL_PLATFORM_MULTI_THREADED)) && (!defined (SL_PLATFORM_EXTERNAL_SPAWN)) 00688 _cc3100_._driver._SlDrvObjUnLock(&g_pCB->GlobalLockObj); 00689 // OSI_RET_OK_CHECK(_cc3100_._nonos.sl_LockObjUnlock(&g_pCB->GlobalLockObj)); 00690 #else 00691 OSI_RET_OK_CHECK(sl_LockObjUnlock(&g_pCB->GlobalLockObj)); 00692 #endif 00693 return(SL_RET_CODE_OK); 00694 00695 } 00696 00697 /*************************************************************************** 00698 _sl_HandleAsync_Stop - handles stop signalling to 00699 a waiting object 00700 ****************************************************************************/ 00701 void _sl_HandleAsync_Stop(void *pVoidBuf) 00702 { 00703 _BasicResponse_t *pMsgArgs = (_BasicResponse_t *)_SL_RESP_ARGS_START(pVoidBuf); 00704 00705 VERIFY_SOCKET_CB(NULL != g_pCB->StopCB.pAsyncRsp); 00706 00707 _cc3100_._driver._SlDrvProtectionObjLockWaitForever(); 00708 00709 memcpy(g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].pRespArgs, pMsgArgs, sizeof(_BasicResponse_t)); 00710 _cc3100_._driver._SlDrvSyncObjSignal(&g_pCB->ObjPool[g_pCB->FunctionParams.AsyncExt.ActionIndex].SyncObj); 00711 _cc3100_._driver._SlDrvProtectionObjUnLock(); 00712 return; 00713 } 00714 00715 /****************************************************************************** 00716 _SlDrvDeviceEventHandler - handles internally device async events 00717 ******************************************************************************/ 00718 void _SlDrvDeviceEventHandler(void *pArgs) 00719 { 00720 _SlResponseHeader_t *pHdr = (_SlResponseHeader_t *)pArgs; 00721 00722 switch(pHdr->GenHeader.Opcode) { 00723 case SL_OPCODE_DEVICE_INITCOMPLETE: 00724 _cc3100_._sl_HandleAsync_InitComplete(pHdr); 00725 00726 break; 00727 case SL_OPCODE_DEVICE_STOP_ASYNC_RESPONSE: 00728 _sl_HandleAsync_Stop(pHdr); 00729 00730 break; 00731 00732 00733 case SL_OPCODE_DEVICE_ABORT: 00734 { 00735 #if defined (sl_GeneralEvtHdlr) || defined(EXT_LIB_REGISTERED_GENERAL_EVENTS) 00736 SlDeviceEvent_t devHandler; 00737 devHandler.Event = SL_DEVICE_ABORT_ERROR_EVENT; 00738 devHandler.EventData.deviceReport.AbortType = *((uint32_t*)pArgs + 2); 00739 devHandler.EventData.deviceReport.AbortData = *((uint32_t*)pArgs + 3); 00740 _SlDrvHandleGeneralEvents(&devHandler); 00741 #endif 00742 } 00743 break; 00744 case SL_OPCODE_DEVICE_DEVICEASYNCFATALERROR: 00745 #if defined (sl_GeneralEvtHdlr) || defined(EXT_LIB_REGISTERED_GENERAL_EVENTS) 00746 { 00747 _BasicResponse_t *pMsgArgs = (_BasicResponse_t *)_SL_RESP_ARGS_START(pHdr); 00748 SlDeviceEvent_t devHandler; 00749 devHandler.Event = SL_DEVICE_FATAL_ERROR_EVENT; 00750 devHandler.EventData.deviceEvent.status = pMsgArgs->status & 0xFF; 00751 devHandler.EventData.deviceEvent.sender = (SlErrorSender_e)((pMsgArgs->status >> 8) & 0xFF); 00752 _SlDrvHandleGeneralEvents(&devHandler); 00753 } 00754 #endif 00755 break; 00756 default: 00757 SL_ERROR_TRACE2(MSG_306, "ASSERT: _SlDrvDeviceEventHandler : invalid opcode = 0x%x = %i", pHdr->GenHeader.Opcode, pHdr->GenHeader.Opcode); 00758 //printf("ASSERT: _SlDrvDeviceEventHandler : invalid opcode = 0x%x = %i", pHdr->GenHeader.Opcode, pHdr->GenHeader.Opcode); 00759 00760 } 00761 } 00762 00763 /*****************************************************************************/ 00764 /* _SlDrvNetAppEventHandler */ 00765 /*****************************************************************************/ 00766 void _SlDrvNetAppEventHandler(void *pArgs) 00767 { 00768 _SlResponseHeader_t *pHdr = (_SlResponseHeader_t *)pArgs; 00769 #if defined(sl_HttpServerCallback) || defined(EXT_LIB_REGISTERED_HTTP_SERVER_EVENTS) 00770 SlHttpServerEvent_t httpServerEvent; 00771 SlHttpServerResponse_t httpServerResponse; 00772 #endif 00773 switch(pHdr->GenHeader.Opcode) { 00774 case SL_OPCODE_NETAPP_DNSGETHOSTBYNAMEASYNCRESPONSE: 00775 case SL_OPCODE_NETAPP_DNSGETHOSTBYNAMEASYNCRESPONSE_V6: 00776 _sl_HandleAsync_DnsGetHostByName(pArgs); 00777 break; 00778 #ifndef SL_TINY_EXT 00779 case SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICEASYNCRESPONSE: 00780 case SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICEASYNCRESPONSE_V6: 00781 _sl_HandleAsync_DnsGetHostByService(pArgs); 00782 break; 00783 case SL_OPCODE_NETAPP_PINGREPORTREQUESTRESPONSE: 00784 _sl_HandleAsync_PingResponse(pArgs); 00785 break; 00786 #endif 00787 00788 #if defined(sl_HttpServerCallback) || defined(EXT_LIB_REGISTERED_HTTP_SERVER_EVENTS) 00789 case SL_OPCODE_NETAPP_HTTPGETTOKENVALUE: { 00790 00791 uint8_t *pTokenName; 00792 slHttpServerData_t Token_value; 00793 sl_NetAppHttpServerGetToken_t *httpGetToken = (sl_NetAppHttpServerGetToken_t *)_SL_RESP_ARGS_START(pHdr); 00794 pTokenName = (uint8_t *)((sl_NetAppHttpServerGetToken_t *)httpGetToken + 1); 00795 00796 httpServerResponse.Response = SL_NETAPP_HTTPSETTOKENVALUE; 00797 httpServerResponse.ResponseData.token_value.len = MAX_TOKEN_VALUE_LEN; 00798 00799 /* Reuse the async buffer for getting the token value response from the user */ 00800 httpServerResponse.ResponseData.token_value.data = (uint8_t *)_SL_RESP_ARGS_START(pHdr) + MAX_TOKEN_NAME_LEN; 00801 httpServerEvent.Event = SL_NETAPP_HTTPGETTOKENVALUE_EVENT; 00802 00803 httpServerEvent.EventData.httpTokenName.len = httpGetToken->token_name_len; 00804 httpServerEvent.EventData.httpTokenName.data = pTokenName; 00805 00806 Token_value.token_name = pTokenName; 00807 00808 _SlDrvHandleHttpServerEvents (&httpServerEvent, &httpServerResponse); 00809 00810 Token_value.value_len = httpServerResponse.ResponseData.token_value.len; 00811 Token_value.name_len = httpServerEvent.EventData.httpTokenName.len; 00812 00813 Token_value.token_value = httpServerResponse.ResponseData.token_value.data; 00814 00815 00816 _cc3100_._netapp.sl_NetAppSendTokenValue(&Token_value); 00817 #endif 00818 } 00819 break; 00820 00821 case SL_OPCODE_NETAPP_HTTPPOSTTOKENVALUE: { 00822 #ifdef sl_HttpServerCallback 00823 uint8_t *pPostParams; 00824 00825 sl_NetAppHttpServerPostToken_t *httpPostTokenArgs = (sl_NetAppHttpServerPostToken_t *)_SL_RESP_ARGS_START(pHdr); 00826 pPostParams = (uint8_t *)((sl_NetAppHttpServerPostToken_t *)httpPostTokenArgs + 1); 00827 00828 httpServerEvent.Event = SL_NETAPP_HTTPPOSTTOKENVALUE_EVENT; 00829 00830 httpServerEvent.EventData.httpPostData.action.len = httpPostTokenArgs->post_action_len; 00831 httpServerEvent.EventData.httpPostData.action.data = pPostParams; 00832 pPostParams+=httpPostTokenArgs->post_action_len; 00833 00834 httpServerEvent.EventData.httpPostData.token_name.len = httpPostTokenArgs->token_name_len; 00835 httpServerEvent.EventData.httpPostData.token_name.data = pPostParams; 00836 pPostParams+=httpPostTokenArgs->token_name_len; 00837 00838 httpServerEvent.EventData.httpPostData.token_value.len = httpPostTokenArgs->token_value_len; 00839 httpServerEvent.EventData.httpPostData.token_value.data = pPostParams; 00840 00841 httpServerResponse.Response = SL_NETAPP_RESPONSE_NONE; 00842 00843 00844 _SlDrvHandleHttpServerEvents (&httpServerEvent, &httpServerResponse); 00845 } 00846 break; 00847 #endif 00848 default: 00849 SL_ERROR_TRACE2(MSG_305, "ASSERT: _SlDrvNetAppEventHandler : invalid opcode = 0x%x = %i", pHdr->GenHeader.Opcode, pHdr->GenHeader.Opcode); 00850 VERIFY_PROTOCOL(0); 00851 } 00852 } 00853 00854 /* 00855 * ASYNCHRONOUS EVENT HANDLERS -- Start 00856 */ 00857 00858 /*! 00859 \brief This function handles WLAN events 00860 00861 \param[in] pWlanEvent is the event passed to the handler 00862 00863 \return None 00864 00865 \note 00866 00867 \warning 00868 */ 00869 #if (defined(sl_WlanEvtHdlr)) 00870 void SimpleLinkWlanEventHandler(SlWlanEvent_t *pWlanEvent) 00871 { 00872 unsigned char g_ucConnectionSSID[32+1]; //Connection SSID 00873 unsigned char g_ucConnectionBSSID[6]; //Connection BSSID 00874 00875 if(pWlanEvent == NULL) 00876 Uart_Write((uint8_t *)" [WLAN EVENT] NULL Pointer Error \n\r"); 00877 00878 switch(pWlanEvent->Event) { 00879 case SL_WLAN_CONNECT_EVENT: { 00880 _cc3100_.SET_STATUS_BIT(g_Status, STATUS_BIT_CONNECTION); 00881 00882 // Copy new connection SSID and BSSID to global parameters 00883 memcpy(g_ucConnectionSSID,pWlanEvent->EventData. 00884 STAandP2PModeWlanConnected.ssid_name, 00885 pWlanEvent->EventData.STAandP2PModeWlanConnected.ssid_len); 00886 memcpy(g_ucConnectionBSSID, 00887 pWlanEvent->EventData.STAandP2PModeWlanConnected.bssid, 00888 SL_BSSID_LENGTH); 00889 memset(print_buf, 0x00, PRINT_BUF_LEN); 00890 sprintf((char*) print_buf, "[WLAN EVENT] STA Connected to the AP: %s ,""BSSID: %x:%x:%x:%x:%x:%x\n\r", 00891 g_ucConnectionSSID,g_ucConnectionBSSID[0], 00892 g_ucConnectionBSSID[1],g_ucConnectionBSSID[2], 00893 g_ucConnectionBSSID[3],g_ucConnectionBSSID[4], 00894 g_ucConnectionBSSID[5]); 00895 Uart_Write((uint8_t *) print_buf); 00896 /* 00897 * Information about the connected AP (like name, MAC etc) will be 00898 * available in 'slWlanConnectAsyncResponse_t' - Applications 00899 * can use it if required 00900 * 00901 * slWlanConnectAsyncResponse_t *pEventData = NULL; 00902 * pEventData = &pWlanEvent->EventData.STAandP2PModeWlanConnected; 00903 * 00904 */ 00905 } 00906 break; 00907 00908 case SL_WLAN_DISCONNECT_EVENT: { 00909 slWlanConnectAsyncResponse_t* pEventData = NULL; 00910 00911 _cc3100_.CLR_STATUS_BIT(g_Status, STATUS_BIT_CONNECTION); 00912 _cc3100_.CLR_STATUS_BIT(g_Status, STATUS_BIT_IP_ACQUIRED); 00913 00914 pEventData = &pWlanEvent->EventData.STAandP2PModeDisconnected; 00915 00916 /* If the user has initiated 'Disconnect' request, 'reason_code' is SL_USER_INITIATED_DISCONNECTION */ 00917 if(SL_USER_INITIATED_DISCONNECTION == pEventData->reason_code) { 00918 memset(print_buf, 0x00, PRINT_BUF_LEN); 00919 sprintf((char*) print_buf, "[WLAN EVENT]Device disconnected from the AP: %s," 00920 "BSSID: %x:%x:%x:%x:%x:%x on application's request \n\r", 00921 g_ucConnectionSSID,g_ucConnectionBSSID[0], 00922 g_ucConnectionBSSID[1],g_ucConnectionBSSID[2], 00923 g_ucConnectionBSSID[3],g_ucConnectionBSSID[4], 00924 g_ucConnectionBSSID[5]); 00925 Uart_Write((uint8_t *) print_buf); 00926 } else { 00927 memset(print_buf, 0x00, PRINT_BUF_LEN); 00928 sprintf((char*) print_buf, "[WLAN ERROR]Device disconnected from the AP AP: %s," 00929 "BSSID: %x:%x:%x:%x:%x:%x on an ERROR..!! \n\r", 00930 g_ucConnectionSSID,g_ucConnectionBSSID[0], 00931 g_ucConnectionBSSID[1],g_ucConnectionBSSID[2], 00932 g_ucConnectionBSSID[3],g_ucConnectionBSSID[4], 00933 g_ucConnectionBSSID[5]); 00934 Uart_Write((uint8_t *) print_buf); 00935 } 00936 memset(g_ucConnectionSSID,0,sizeof(g_ucConnectionSSID)); 00937 memset(g_ucConnectionBSSID,0,sizeof(g_ucConnectionBSSID)); 00938 } 00939 break; 00940 00941 case SL_WLAN_STA_CONNECTED_EVENT: { 00942 _cc3100_.SET_STATUS_BIT(g_Status, STATUS_BIT_STA_CONNECTED); 00943 } 00944 break; 00945 00946 case SL_WLAN_STA_DISCONNECTED_EVENT: { 00947 _cc3100_.CLR_STATUS_BIT(g_Status, STATUS_BIT_STA_CONNECTED); 00948 _cc3100_.CLR_STATUS_BIT(g_Status, STATUS_BIT_IP_LEASED); 00949 } 00950 break; 00951 00952 default: { 00953 memset(print_buf, 0x00, PRINT_BUF_LEN); 00954 sprintf((char*) print_buf, "[WLAN EVENT] Unexpected event [0x%x]\n\r",pWlanEvent->Event); 00955 Uart_Write((uint8_t *) print_buf); 00956 } 00957 break; 00958 } 00959 } 00960 #endif 00961 00962 /*! 00963 \brief This function handles events for IP address acquisition via DHCP 00964 indication 00965 00966 \param[in] pNetAppEvent is the event passed to the handler 00967 00968 \return None 00969 00970 \note 00971 00972 \warning 00973 */ 00974 #if (defined(sl_NetAppEvtHdlr)) 00975 void SimpleLinkNetAppEventHandler(SlNetAppEvent_t *pNetAppEvent) 00976 { 00977 00978 if(pNetAppEvent == NULL){ 00979 Uart_Write((uint8_t *)" [NETAPP EVENT] NULL Pointer Error \n\r"); 00980 } 00981 00982 switch(pNetAppEvent->Event) { 00983 case SL_NETAPP_IPV4_IPACQUIRED_EVENT: { 00984 SlIpV4AcquiredAsync_t *pEventData = NULL; 00985 _cc3100_.SET_STATUS_BIT(g_Status, STATUS_BIT_IP_ACQUIRED); 00986 pEventData = &pNetAppEvent->EventData.ipAcquiredV4; 00987 g_GatewayIP = pEventData->gateway; 00988 00989 memset(print_buf, 0x00, PRINT_BUF_LEN); 00990 sprintf((char*) print_buf, "[NETAPP EVENT] IP Acquired: IP=%d.%d.%d.%d , ""Gateway=%d.%d.%d.%d\n\r", 00991 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.ip,3), 00992 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.ip,2), 00993 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.ip,1), 00994 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.ip,0), 00995 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.gateway,3), 00996 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.gateway,2), 00997 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.gateway,1), 00998 _cc3100_._netcfg.SL_IPV4_BYTE(pNetAppEvent->EventData.ipAcquiredV4.gateway,0)); 00999 Uart_Write((uint8_t *) print_buf); 01000 } 01001 break; 01002 01003 case SL_NETAPP_IP_LEASED_EVENT: { 01004 g_StationIP = pNetAppEvent->EventData.ipLeased.ip_address; 01005 _cc3100_.SET_STATUS_BIT(g_Status, STATUS_BIT_IP_LEASED); 01006 01007 } 01008 break; 01009 01010 default: { 01011 memset(print_buf, 0x00, PRINT_BUF_LEN); 01012 sprintf((char*) print_buf, "[NETAPP EVENT] Unexpected event [0x%x] \n\r",pNetAppEvent->Event); 01013 Uart_Write((uint8_t *) print_buf); 01014 } 01015 break; 01016 } 01017 } 01018 #endif 01019 01020 /*! 01021 \brief This function handles socket events indication 01022 01023 \param[in] pSock is the event passed to the handler 01024 01025 \return None 01026 */ 01027 #if (defined(sl_SockEvtHdlr)) 01028 void SimpleLinkSockEventHandler(SlSockEvent_t *pSock) 01029 { 01030 if(pSock == NULL) 01031 Uart_Write((uint8_t *)" [SOCK EVENT] NULL Pointer Error \n\r"); 01032 01033 switch( pSock->Event ) 01034 { 01035 case SL_SOCKET_TX_FAILED_EVENT: 01036 /* 01037 * TX Failed 01038 * 01039 * Information about the socket descriptor and status will be 01040 * available in 'SlSockEventData_t' - Applications can use it if 01041 * required 01042 * 01043 * SlSockEventData_t *pEventData = NULL; 01044 * pEventData = & pSock->EventData; 01045 */ 01046 01047 switch( pSock->socketAsyncEvent.SockTxFailData.status ) 01048 { 01049 case SL_ECLOSE: 01050 Uart_Write((uint8_t *)" [SOCK EVENT] Close socket operation, failed to transmit all queued packets\n\r"); 01051 break; 01052 default: 01053 memset(print_buf, 0x00, PRINT_BUF_LEN); 01054 sprintf((char*) print_buf, "[SOCK ERROR] - TX FAILED : socket %d , reason""(%d) \n\n", pSock->socketAsyncEvent.SockTxFailData.sd, pSock->socketAsyncEvent.SockTxFailData.status); 01055 Uart_Write((uint8_t *) print_buf); 01056 01057 break; 01058 } 01059 break; 01060 01061 default: 01062 memset(print_buf, 0x00, PRINT_BUF_LEN); 01063 sprintf((char*) print_buf, "[SOCK EVENT] - Unexpected Event [%x0x]\n\n",pSock->Event); 01064 Uart_Write((uint8_t *) print_buf); 01065 break; 01066 } 01067 } 01068 #endif 01069 01070 /*! 01071 \brief This function handles callback for the HTTP server events 01072 01073 \param[in] pHttpEvent - Contains the relevant event information 01074 \param[in] pHttpResponse - Should be filled by the user with the 01075 relevant response information 01076 01077 \return None 01078 01079 \note 01080 01081 \warning 01082 */ 01083 #if (defined(sl_HttpServerCallback)) 01084 void SimpleLinkHttpServerCallback(SlHttpServerEvent_t *pSlHttpServerEvent, SlHttpServerResponse_t *pSlHttpServerResponse) 01085 { 01086 unsigned char strLenVal = 0; 01087 01088 if(!pSlHttpServerEvent || !pSlHttpServerResponse) 01089 { 01090 return; 01091 } 01092 01093 switch (pSlHttpServerEvent->Event) 01094 { 01095 case SL_NETAPP_HTTPGETTOKENVALUE_EVENT: 01096 { 01097 unsigned char status, *ptr; 01098 01099 ptr = pSlHttpServerResponse->ResponseData.token_value.data; 01100 pSlHttpServerResponse->ResponseData.token_value.len = 0; 01101 if(memcmp(pSlHttpServerEvent->EventData.httpTokenName.data, GET_token, 01102 strlen((const char *)GET_token)) == 0) 01103 { 01104 status = GPIO_IF_LedStatus(MCU_RED_LED_GPIO); 01105 strLenVal = strlen(LED1_STRING); 01106 memcpy(ptr, LED1_STRING, strLenVal); 01107 ptr += strLenVal; 01108 pSlHttpServerResponse->ResponseData.token_value.len += strLenVal; 01109 if(status & 0x01) 01110 { 01111 strLenVal = strlen(LED_ON_STRING); 01112 memcpy(ptr, LED_ON_STRING, strLenVal); 01113 ptr += strLenVal; 01114 pSlHttpServerResponse->ResponseData.token_value.len += strLenVal; 01115 } 01116 else 01117 { 01118 strLenVal = strlen(LED_OFF_STRING); 01119 memcpy(ptr, LED_OFF_STRING, strLenVal); 01120 ptr += strLenVal; 01121 pSlHttpServerResponse->ResponseData.token_value.len += strLenVal; 01122 } 01123 status = GPIO_IF_LedStatus(MCU_GREEN_LED_GPIO); 01124 strLenVal = strlen(LED2_STRING); 01125 memcpy(ptr, LED2_STRING, strLenVal); 01126 ptr += strLenVal; 01127 pSlHttpServerResponse->ResponseData.token_value.len += strLenVal; 01128 if(status & 0x01) 01129 { 01130 strLenVal = strlen(LED_ON_STRING); 01131 memcpy(ptr, LED_ON_STRING, strLenVal); 01132 ptr += strLenVal; 01133 pSlHttpServerResponse->ResponseData.token_value.len += strLenVal; 01134 } 01135 else 01136 { 01137 strLenVal = strlen(LED_OFF_STRING); 01138 memcpy(ptr, LED_OFF_STRING, strLenVal); 01139 ptr += strLenVal; 01140 pSlHttpServerResponse->ResponseData.token_value.len += strLenVal; 01141 } 01142 *ptr = '\0'; 01143 } 01144 01145 } 01146 break; 01147 01148 case SL_NETAPP_HTTPPOSTTOKENVALUE_EVENT: 01149 { 01150 unsigned char led; 01151 unsigned char *ptr = pSlHttpServerEvent->EventData.httpPostData.token_name.data; 01152 01153 if(memcmp(ptr, POST_token, strlen((const char *)POST_token)) == 0) 01154 { 01155 ptr = pSlHttpServerEvent->EventData.httpPostData.token_value.data; 01156 strLenVal = strlen(LED_STRING); 01157 if(memcmp(ptr, LED_STRING, strLenVal) != 0) 01158 break; 01159 ptr += strLenVal; 01160 led = *ptr; 01161 strLenVal = strlen(LED_ON_STRING); 01162 ptr += strLenVal; 01163 if(led == '1') 01164 { 01165 if(memcmp(ptr, LED_ON_STRING, strLenVal) == 0) 01166 { 01167 GPIO_IF_LedOn(MCU_RED_LED_GPIO); 01168 } 01169 else 01170 { 01171 GPIO_IF_LedOff(MCU_RED_LED_GPIO); 01172 } 01173 } 01174 else if(led == '2') 01175 { 01176 if(memcmp(ptr, LED_ON_STRING, strLenVal) == 0) 01177 { 01178 GPIO_IF_LedOn(MCU_GREEN_LED_GPIO); 01179 } 01180 else 01181 { 01182 GPIO_IF_LedOff(MCU_GREEN_LED_GPIO); 01183 } 01184 } 01185 01186 } 01187 } 01188 break; 01189 default: 01190 break; 01191 } 01192 } 01193 #endif 01194 /*! 01195 \brief This function handles general error events indication 01196 01197 \param[in] pDevEvent is the event passed to the handler 01198 01199 \return None 01200 */ 01201 #if (defined(sl_GeneralEvtHdlr)) 01202 void SimpleLinkGeneralEventHandler(SlDeviceEvent_t *pDevEvent) 01203 { 01204 /* 01205 * Most of the general errors are not FATAL and are to be handled 01206 * appropriately by the application 01207 */ 01208 memset(print_buf, 0x00, PRINT_BUF_LEN); 01209 sprintf((char*) print_buf, "[GENERAL EVENT] - ID=[%d] Sender=[%d]\n\n", pDevEvent->EventData.deviceEvent.status, pDevEvent->EventData.deviceEvent.sender); 01210 Uart_Write((uint8_t *) print_buf); 01211 } 01212 #endif 01213 01214 #ifdef __cplusplus 01215 } 01216 #endif /* __cplusplus */ 01217 01218 //}//namespace
Generated on Tue Jul 12 2022 22:22:38 by
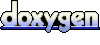