
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
cc3100_driver.h
00001 /* 00002 * driver.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 #ifndef DRIVER_INT_H_ 00038 #define DRIVER_INT_H_ 00039 00040 #include "mbed.h" 00041 #include "cc3100_simplelink.h" 00042 #include "cc3100_user.h" 00043 00044 #include "cc3100_protocol.h" 00045 #include "cc3100_spi.h" 00046 //#include "cc3100_nonos.h" 00047 #include "cc3100_netapp.h" 00048 //#include "cc3100.h" 00049 00050 //#ifdef SL_PLATFORM_MULTI_THREADED 00051 #if (defined (SL_PLATFORM_MULTI_THREADED)) || (defined (SL_PLATFORM_EXTERNAL_SPAWN)) 00052 #include "osi.h" 00053 #endif 00054 00055 /*****************************************************************************/ 00056 /* Macro declarations */ 00057 /*****************************************************************************/ 00058 00059 /* 2 LSB of the N2H_SYNC_PATTERN are for sequence number 00060 only in SPI interface 00061 support backward sync pattern */ 00062 #define N2H_SYNC_PATTERN_SEQ_NUM_BITS ((uint32_t)0x00000003) /* Bits 0..1 - use the 2 LBS for seq num */ 00063 #define N2H_SYNC_PATTERN_SEQ_NUM_EXISTS ((uint32_t)0x00000004) /* Bit 2 - sign that sequence number exists in the sync pattern */ 00064 #define N2H_SYNC_PATTERN_MASK ((uint32_t)0xFFFFFFF8) /* Bits 3..31 - constant SYNC PATTERN */ 00065 #define N2H_SYNC_SPI_BUGS_MASK ((uint32_t)0x7FFF7F7F) /* Bits 7,15,31 - ignore the SPI (8,16,32 bites bus) error bits */ 00066 #define BUF_SYNC_SPIM(pBuf) ((*(uint32_t *)(pBuf)) & N2H_SYNC_SPI_BUGS_MASK) 00067 #define N2H_SYNC_SPIM (N2H_SYNC_PATTERN & N2H_SYNC_SPI_BUGS_MASK) 00068 #define N2H_SYNC_SPIM_WITH_SEQ(TxSeqNum) ((N2H_SYNC_SPIM & N2H_SYNC_PATTERN_MASK) | N2H_SYNC_PATTERN_SEQ_NUM_EXISTS | ((TxSeqNum) & (N2H_SYNC_PATTERN_SEQ_NUM_BITS))) 00069 #define MATCH_WOUT_SEQ_NUM(pBuf) ( BUF_SYNC_SPIM(pBuf) == N2H_SYNC_SPIM ) 00070 #define MATCH_WITH_SEQ_NUM(pBuf, TxSeqNum) ( BUF_SYNC_SPIM(pBuf) == (N2H_SYNC_SPIM_WITH_SEQ(TxSeqNum)) ) 00071 #define N2H_SYNC_PATTERN_MATCH(pBuf, TxSeqNum) \ 00072 ( \ 00073 ( (*((uint32_t *)pBuf) & N2H_SYNC_PATTERN_SEQ_NUM_EXISTS) && ( MATCH_WITH_SEQ_NUM(pBuf, TxSeqNum) ) ) || \ 00074 ( !(*((uint32_t *)pBuf) & N2H_SYNC_PATTERN_SEQ_NUM_EXISTS) && ( MATCH_WOUT_SEQ_NUM(pBuf ) ) ) \ 00075 ) 00076 00077 #define OPCODE(_ptr) (((_SlResponseHeader_t *)(_ptr))->GenHeader.Opcode) 00078 #define RSP_PAYLOAD_LEN(_ptr) (((_SlResponseHeader_t *)(_ptr))->GenHeader.Len - _SL_RESP_SPEC_HDR_SIZE) 00079 #define SD(_ptr) (((_SocketAddrResponse_u *)(_ptr))->IpV4.sd) 00080 /* Actual size of Recv/Recvfrom response data */ 00081 #define ACT_DATA_SIZE(_ptr) (((_SocketAddrResponse_u *)(_ptr))->IpV4.statusOrLen) 00082 00083 #define _SL_PROTOCOL_ALIGN_SIZE(msgLen) (((msgLen)+3) & (~3)) 00084 #define _SL_IS_PROTOCOL_ALIGNED_SIZE(msgLen) (!((msgLen) & 3)) 00085 #define _SL_PROTOCOL_CALC_LEN(pCmdCtrl,pCmdExt) ((pCmdExt) ? \ 00086 (_SL_PROTOCOL_ALIGN_SIZE(pCmdCtrl->TxDescLen) + _SL_PROTOCOL_ALIGN_SIZE(pCmdExt->TxPayloadLen)) : \ 00087 (_SL_PROTOCOL_ALIGN_SIZE(pCmdCtrl->TxDescLen))) 00088 00089 00090 namespace mbed_cc3100 { 00091 00092 //class cc3100; 00093 00094 /*****************************************************************************/ 00095 /* Structure/Enum declarations */ 00096 /*****************************************************************************/ 00097 //typedef void(*_SlSpawnEntryFunc_t)(void* pValue); 00098 00099 typedef struct { 00100 _SlOpcode_t Opcode; 00101 _SlArgSize_t TxDescLen; 00102 _SlArgSize_t RxDescLen; 00103 } _SlCmdCtrl_t; 00104 00105 typedef struct { 00106 uint16_t TxPayloadLen; 00107 int16_t RxPayloadLen; 00108 int16_t ActualRxPayloadLen; 00109 uint8_t *pTxPayload; 00110 uint8_t *pRxPayload; 00111 } _SlCmdExt_t; 00112 00113 00114 typedef struct _SlArgsData_t { 00115 uint8_t *pArgs; 00116 uint8_t *pData; 00117 } _SlArgsData_t; 00118 00119 typedef struct { 00120 _SlSyncObj_t SyncObj; 00121 uint8_t *pRespArgs; 00122 uint8_t ActionID; 00123 uint8_t AdditionalData; /* use for socketID and one bit which indicate supprt IPV6 or not (1=support, 0 otherwise) */ 00124 uint8_t NextIndex; 00125 00126 } _SlPoolObj_t; 00127 00128 00129 typedef enum { 00130 SOCKET_0, 00131 SOCKET_1, 00132 SOCKET_2, 00133 SOCKET_3, 00134 SOCKET_4, 00135 SOCKET_5, 00136 SOCKET_6, 00137 SOCKET_7, 00138 MAX_SOCKET_ENUM_IDX, 00139 #ifndef SL_TINY_EXT 00140 ACCEPT_ID = MAX_SOCKET_ENUM_IDX, 00141 CONNECT_ID, 00142 #else 00143 CONNECT_ID = MAX_SOCKET_ENUM_IDX, 00144 #endif 00145 #ifndef SL_TINY_EXT 00146 SELECT_ID, 00147 #endif 00148 GETHOSYBYNAME_ID, 00149 #ifndef SL_TINY_EXT 00150 GETHOSYBYSERVICE_ID, 00151 PING_ID, 00152 #endif 00153 START_STOP_ID, 00154 RECV_ID 00155 } _SlActionID_e; 00156 00157 typedef struct _SlActionLookup_t { 00158 uint8_t ActionID; 00159 uint16_t ActionAsyncOpcode; 00160 _SlSpawnEntryFunc_t AsyncEventHandler; 00161 00162 } _SlActionLookup_t; 00163 00164 00165 typedef struct { 00166 uint8_t TxPoolCnt; 00167 _SlLockObj_t TxLockObj; 00168 _SlSyncObj_t TxSyncObj; 00169 } _SlFlowContCB_t; 00170 00171 typedef enum { 00172 RECV_RESP_CLASS, 00173 CMD_RESP_CLASS, 00174 ASYNC_EVT_CLASS, 00175 DUMMY_MSG_CLASS 00176 } _SlRxMsgClass_e; 00177 00178 typedef struct { 00179 uint8_t *pAsyncBuf; /* place to write pointer to buffer with CmdResp's Header + Arguments */ 00180 uint8_t ActionIndex; 00181 _SlSpawnEntryFunc_t AsyncEvtHandler; /* place to write pointer to AsyncEvent handler (calc-ed by Opcode) */ 00182 _SlRxMsgClass_e RxMsgClass; /* type of Rx message */ 00183 } AsyncExt_t; 00184 00185 typedef struct { 00186 _SlCmdCtrl_t *pCmdCtrl; 00187 uint8_t *pTxRxDescBuff; 00188 _SlCmdExt_t *pCmdExt; 00189 AsyncExt_t AsyncExt; 00190 } _SlFunctionParams_t; 00191 00192 typedef void (*P_INIT_CALLBACK)(uint32_t Status); 00193 00194 typedef struct { 00195 _SlFd_t FD; 00196 _SlLockObj_t GlobalLockObj; 00197 _SlCommandHeader_t TempProtocolHeader; 00198 P_INIT_CALLBACK pInitCallback; 00199 00200 _SlPoolObj_t ObjPool[MAX_CONCURRENT_ACTIONS]; 00201 uint8_t FreePoolIdx; 00202 uint8_t PendingPoolIdx; 00203 uint8_t ActivePoolIdx; 00204 uint32_t ActiveActionsBitmap; 00205 _SlLockObj_t ProtectionLockObj; 00206 00207 _SlSyncObj_t CmdSyncObj; 00208 uint8_t IsCmdRespWaited; 00209 _SlFlowContCB_t FlowContCB; 00210 uint8_t TxSeqNum; 00211 uint8_t RxDoneCnt; 00212 uint8_t SocketNonBlocking; 00213 uint8_t SocketTXFailure; 00214 /* for stack reduction the parameters are globals */ 00215 _SlFunctionParams_t FunctionParams; 00216 00217 uint8_t ActionIndex; 00218 }_SlDriverCb_t; 00219 00220 extern volatile uint8_t RxIrqCnt; 00221 00222 extern _SlDriverCb_t* g_pCB; 00223 typedef uint8_t _SlSd_t; 00224 00225 class cc3100_driver 00226 { 00227 00228 public: 00229 00230 //#ifndef SL_PLATFORM_MULTI_THREADED 00231 #if (!defined (SL_PLATFORM_MULTI_THREADED)) && (!defined (SL_PLATFORM_EXTERNAL_SPAWN)) 00232 cc3100_driver(cc3100_spi &spi, cc3100_nonos &nonos, cc3100_netapp &netapp, cc3100_flowcont &flowcont); 00233 #else 00234 cc3100_driver(cc3100_spi &spi, cc3100_netapp &netapp, cc3100_flowcont &flowcont); 00235 #endif 00236 ~cc3100_driver(); 00237 00238 00239 /*****************************************************************************/ 00240 /* Function prototypes */ 00241 /*****************************************************************************/ 00242 typedef _SlDriverCb_t pDriver; 00243 00244 uint8_t _SlDrvProtectAsyncRespSetting(uint8_t *pAsyncRsp, uint8_t ActionID, uint8_t SocketID); 00245 00246 bool _SL_PENDING_RX_MSG(pDriver* pDriverCB); 00247 00248 void _SlDrvDriverCBInit(void); 00249 00250 void _SlDrvDriverCBDeinit(void); 00251 00252 void _SlDrvRxIrqHandler(void *pValue); 00253 00254 _SlReturnVal_t _SlDrvCmdOp(_SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00255 00256 _SlReturnVal_t _SlDrvCmdSend(_SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00257 00258 _SlReturnVal_t _SlDrvDataReadOp(_SlSd_t Sd, _SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00259 00260 _SlReturnVal_t _SlDrvDataWriteOp(_SlSd_t Sd, _SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00261 #ifndef SL_TINY_EXT 00262 int16_t _SlDrvBasicCmd(_SlOpcode_t Opcode); 00263 #endif 00264 uint8_t _SlDrvWaitForPoolObj(uint8_t ActionID, uint8_t SocketID); 00265 00266 void _SlDrvReleasePoolObj(uint8_t pObj); 00267 00268 // void _SlDrvObjInit(void); 00269 00270 _SlReturnVal_t _SlDrvMsgRead(void); 00271 00272 _SlReturnVal_t _SlDrvMsgWrite(_SlCmdCtrl_t *pCmdCtrl,_SlCmdExt_t *pCmdExt, uint8_t *pTxRxDescBuff); 00273 00274 // _SlReturnVal_t _SlDrvMsgWrite(void); 00275 00276 _SlReturnVal_t _SlDrvMsgReadCmdCtx(void); 00277 00278 _SlReturnVal_t _SlDrvMsgReadSpawnCtx_(void *pValue); 00279 00280 void _SlDrvClassifyRxMsg(_SlOpcode_t Opcode ); 00281 00282 _SlReturnVal_t _SlDrvRxHdrRead(uint8_t *pBuf, uint8_t *pAlignSize); 00283 00284 void _SlDrvShiftDWord(uint8_t *pBuf); 00285 00286 void _SlAsyncEventGenericHandler(void); 00287 00288 void _SlDrvObjDeInit(void); 00289 00290 void _SlRemoveFromList(uint8_t* ListIndex, uint8_t ItemIndex); 00291 00292 _SlReturnVal_t _SlFindAndSetActiveObj(_SlOpcode_t Opcode, uint8_t Sd); 00293 00294 uint16_t _SlDrvAlignSize(uint16_t msgLen); 00295 void _SlDrvSyncObjWaitForever(_SlSyncObj_t *pSyncObj); 00296 void _SlDrvSyncObjSignal(_SlSyncObj_t *pSyncObj); 00297 void _SlDrvObjLock(_SlLockObj_t *pLockObj, _SlTime_t Timeout); 00298 void _SlDrvObjLockWaitForever(_SlLockObj_t *pLockObj); 00299 void _SlDrvObjUnLock(_SlLockObj_t *pLockObj); 00300 void _SlDrvProtectionObjLockWaitForever(); 00301 void _SlDrvProtectionObjUnLock(); 00302 void _SlDrvMemZero(void* Addr, uint16_t size); 00303 void _SlDrvResetCmdExt(_SlCmdExt_t* pCmdExt); 00304 00305 00306 private: 00307 00308 cc3100_spi &_spi; 00309 //#ifndef SL_PLATFORM_MULTI_THREADED 00310 #if (!defined (SL_PLATFORM_MULTI_THREADED)) && (!defined (SL_PLATFORM_EXTERNAL_SPAWN)) 00311 cc3100_nonos &_nonos; 00312 #endif 00313 cc3100_netapp &_netapp; 00314 cc3100_flowcont &_flowcont; 00315 00316 };//class 00317 00318 00319 00320 }//namespace mbed_cc3100 00321 00322 #endif /* __DRIVER_INT_H__ */ 00323
Generated on Tue Jul 12 2022 22:22:38 by
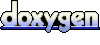