
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
HttpRequest.h
00001 //***************************************************************************** 00002 // Copyright (C) 2014 Texas Instruments Incorporated 00003 // 00004 // All rights reserved. Property of Texas Instruments Incorporated. 00005 // Restricted rights to use, duplicate or disclose this code are 00006 // granted through contract. 00007 // The program may not be used without the written permission of 00008 // Texas Instruments Incorporated or against the terms and conditions 00009 // stipulated in the agreement under which this program has been supplied, 00010 // and under no circumstances can it be used with non-TI connectivity device. 00011 // 00012 //***************************************************************************** 00013 00014 #ifndef _HTTP_REQUEST_H_ 00015 #define _HTTP_REQUEST_H_ 00016 00017 /** 00018 * @defgroup HttpRequest HTTP Request definitions 00019 * This header file defines the structure which holds information about an HTTP request. 00020 * Such a structure is generated by the core module and then passed to a content handler module for processing. 00021 * 00022 * @{ 00023 */ 00024 00025 #include "HttpString.h" 00026 00027 /// The client wishes to close the connection after this request 00028 #define HTTP_REQUEST_FLAG_CLOSE (1 << 0) 00029 /// The client accepts gzip-compressed content 00030 #define HTTP_REQUEST_FLAG_ACCEPT_GZIP (1 << 1) 00031 /// The request is POST. Otherwise it's GET. 00032 #define HTTP_REQUEST_FLAG_METHOD_POST (1 << 2) 00033 /// The request was authenticated correctly 00034 #define HTTP_REQUEST_FLAG_AUTHENTICATED (1 << 3) 00035 /// The request uses HTTP/1.1 otherwise HTTP/1.0 00036 #define HTTP_REQUEST_1_1 (1 << 4) 00037 /// The request containes content, longer than supported 00038 #define HTTP_REQUEST_CONTENT_IGNORED (1 << 5) 00039 /// The request for websocket upgrade is from a browser client 00040 #define WS_REQUEST_BROWSER (1 << 6) 00041 00042 00043 /* 00044 * defgroup for all WebSocket Opcodes 00045 */ 00046 00047 ///Continuation frame 00048 #define WS_CONTINUATION (0x00) 00049 ///Text frame in data packet 00050 #define WS_TEXT (0x01) 00051 ///Binary frame in data packet 00052 #define WS_BINARY (0x02) 00053 ///Close connection with client 00054 #define WS_CLOSE (0x08) 00055 ///Ping 00056 #define WS_PING (0x09) 00057 ///Pong 00058 #define WS_PONG (0x0A) 00059 00060 // End of opcode 00061 00062 /** 00063 * A structure to hold all data about an HTTP request 00064 * Note: The request's resource string is not passed as part of this structure, but rather directly to the Http*_InitRequest() function 00065 */ 00066 struct HttpRequest 00067 { 00068 /// Flags. See HTTP_REQUEST_FLAG_* 00069 UINT16 uFlags; 00070 /// Connection number in HttpCore. This value is guaranteed to satisfy: 0 <= uConnection < HTTP_CORE_MAX_CONNECTIONS 00071 UINT16 uConnection; 00072 /// Request content information (e.g. POST data). uLength value of 0 indicates no content was sent in the request. 00073 struct HttpBlob requestContent; 00074 }; 00075 00076 /// @} 00077 00078 #endif //_HTTP_REQUEST_H_ 00079
Generated on Tue Jul 12 2022 22:22:38 by
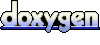