
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
HttpDynamic.cpp
00001 //***************************************************************************** 00002 // Copyright (C) 2014 Texas Instruments Incorporated 00003 // 00004 // All rights reserved. Property of Texas Instruments Incorporated. 00005 // Restricted rights to use, duplicate or disclose this code are 00006 // granted through contract. 00007 // The program may not be used without the written permission of 00008 // Texas Instruments Incorporated or against the terms and conditions 00009 // stipulated in the agreement under which this program has been supplied, 00010 // and under no circumstances can it be used with non-TI connectivity device. 00011 // 00012 //***************************************************************************** 00013 00014 /** 00015 * @addtogroup HttpDynamic 00016 * @{ 00017 */ 00018 00019 #include "HttpDynamic.h" 00020 #include "HttpRequest.h" 00021 #include "HttpResponse.h" 00022 #include "HttpCore.h" 00023 #include "string.h" 00024 00025 #ifdef HTTP_CORE_ENABLE_DYNAMIC 00026 00027 /** 00028 * @defgroup HttpDynamic Dynamic request handler module 00029 * This module implements dynamic content processing for HTTP requests. 00030 * All requests are handled by C code functions, and the response contents is returned via HttpResopnse routines 00031 * Note this module is only compiled if HTTP_CORE_ENABLE_DYNAMIC is defined in HttpConfig.h 00032 * 00033 * @{ 00034 */ 00035 00036 Resource g_RestContent[MAX_RESOURCE]; 00037 00038 int g_NumResource = 0; 00039 00040 int SetResources(unsigned char method, char* pBuf, unsigned char* (*pCbRestFunc)(void *pArgs) ) 00041 { 00042 00043 // POST is 0 and GET is 1 00044 00045 if(g_NumResource < MAX_RESOURCE) 00046 { 00047 g_RestContent[g_NumResource].rest_method = method; 00048 g_RestContent[g_NumResource].ResourceString = (unsigned char*)pBuf; 00049 g_RestContent[g_NumResource].pCbfunc = pCbRestFunc; 00050 g_NumResource++; 00051 } 00052 else 00053 return 0; 00054 00055 return 1; 00056 00057 } 00058 00059 /** 00060 * Initialize HttpDynamic module state for a new request, and identify the request 00061 * This function must examine the specified resource string and determine whether it can commit to process this request 00062 * If this function returns nonzero, then the core will call HttpDynamic_ProcessRequest() with the rest of the request details. 00063 * @param uConnection The number of the connection. This value is guaranteed to satisfy: 0 <= uConnection < HTTP_CORE_MAX_CONNECTIONS 00064 * @param resource The resource part of the URL, as specified by the browser in the request, including any query string (and hash) 00065 * Note: The resource string exists ONLY during the call to this function. The string pointer should not be copied by this function. 00066 * @param method The HTTP method sent from the client for the resource 00067 * @return nonzero if request is to be handled by this module. zero if not. 00068 */ 00069 int HttpDynamic_InitRequest(UINT16 uConnection, struct HttpBlob resource, UINT8 method) 00070 { 00071 00072 /* look for known resource names according to g_RestContent*/ 00073 for (g_NumResource = 0; g_NumResource < MAX_RESOURCE; g_NumResource++) 00074 { 00075 if (HttpString_nextToken((char*)g_RestContent[g_NumResource].ResourceString, strlen((const char*)g_RestContent[g_NumResource].ResourceString), resource) != NULL) 00076 break; 00077 } 00078 00079 /* Rest resource not found */ 00080 if (g_NumResource == MAX_RESOURCE) 00081 return 0; 00082 00083 /* Method doesn't match */ 00084 if(g_RestContent[g_NumResource].rest_method != method) 00085 return 0; 00086 00087 00088 return 1; 00089 } 00090 00091 /** 00092 * Process a dynamic-content HTTP request 00093 * This function is only be called by the core, if HttpDynamic_InitRequest() returns nonzero. 00094 * This function processes the specified HTTP request, and send the response on the connection specified by request->uConnection. 00095 * This function must call the HttpResponse_*() functions in order to send data back to the browser. 00096 * Please refer to HttpResponse.h for more information. 00097 * @param request Pointer to all data available about the request 00098 */ 00099 int HttpDynamic_ProcessRequest(struct HttpRequest* request) 00100 { 00101 struct HttpBlob contentType,nullBlob; 00102 struct HttpBlob contentBody; 00103 void *pArgs = NULL; 00104 00105 contentType.pData = NULL; 00106 contentType.uLength = 0; 00107 nullBlob = contentType; 00108 00109 /* 1. Call user defined API */ 00110 contentBody.pData = g_RestContent[g_NumResource].pCbfunc(pArgs); 00111 contentBody.uLength = strlen((const char*)contentBody.pData); 00112 00113 /* 2. Set header for HTTP Response */ 00114 if(!HttpResponse_Headers(request->uConnection, (UINT16)HTTP_STATUS_OK, 0, contentBody.uLength, contentType, nullBlob)) 00115 return 0; 00116 00117 /* 3. fill the content response (if exists) */ 00118 if (contentBody.uLength != 0) 00119 { 00120 if(!HttpResponse_Content(request->uConnection, contentBody)) 00121 return 0; 00122 } 00123 00124 return 1; 00125 } 00126 00127 00128 /// @} 00129 00130 #endif // HTTP_CORE_ENABLE_DYNAMIC 00131
Generated on Tue Jul 12 2022 22:22:38 by
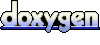