
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
cli_uart.cpp
00001 00002 #include "mbed.h" 00003 00004 // Standard includes 00005 #include <stdarg.h> 00006 00007 #include "myBoardInit.h" 00008 #include "cli_uart.h" 00009 #include "osi.h" 00010 00011 OsiLockObj_t g_printLock; 00012 00013 00014 Serial uart(USBTX, USBRX); 00015 00016 int Uart_Write(unsigned char *inBuff) 00017 { 00018 uint16_t ret, ecount, usLength = strlen((const char *)inBuff); 00019 ecount = 0; 00020 ret = 0; 00021 00022 while(!(uart.writeable())){ecount++;if(ecount>3000)break;}; 00023 00024 if(uart.writeable()) { 00025 00026 if(inBuff == NULL) { 00027 printf("Uart Write buffer empty\r\n"); 00028 return -1; 00029 } 00030 00031 RTOS_MUTEX_ACQUIRE(&g_printLock); 00032 ret = usLength; 00033 00034 while (usLength) { 00035 uart.putc(*inBuff); 00036 usLength--; 00037 inBuff++; 00038 } 00039 00040 RTOS_MUTEX_RELEASE(&g_printLock); 00041 } else { 00042 printf("Uart Write failed [uart not writeable] now trying printf\r\n"); 00043 while (usLength) { 00044 printf("%c",*inBuff); 00045 usLength--; 00046 inBuff++; 00047 } 00048 return -1; 00049 } 00050 00051 return (int)ret; 00052 00053 } 00054 00055 void CLI_Configure(void) 00056 { 00057 uart.baud(115200); 00058 00059 RTOS_MUTEX_CREATE(&g_printLock); 00060 00061 } 00062 00063 //***************************************************************************** 00064 // 00065 //! prints the formatted string on to the console 00066 //! 00067 //! \param format is a pointer to the character string specifying the format in 00068 //! the following arguments need to be interpreted. 00069 //! \param [variable number of] arguments according to the format in the first 00070 //! parameters 00071 //! This function 00072 //! 1. prints the formatted error statement. 00073 //! 00074 //! \return count of characters printed 00075 // 00076 //***************************************************************************** 00077 int Report(const char *pcFormat, ...) 00078 { 00079 int iRet = 0; 00080 //#ifndef NOTERM 00081 00082 char *pcBuff, *pcTemp; 00083 int iSize = 256; 00084 00085 va_list list; 00086 pcBuff = (char*)malloc(iSize); 00087 if(pcBuff == NULL) 00088 { 00089 return -1; 00090 } 00091 while(1) 00092 { 00093 va_start(list,pcFormat); 00094 iRet = vsnprintf((char*)pcBuff,iSize,pcFormat,list); 00095 va_end(list); 00096 if(iRet > -1 && iRet < iSize) 00097 { 00098 break; 00099 } 00100 else 00101 { 00102 iSize*=2; 00103 if((pcTemp=(char*)realloc(pcBuff,iSize))==NULL) 00104 { 00105 Message("Could not reallocate memory\n\r"); 00106 iRet = -1; 00107 break; 00108 } 00109 else 00110 { 00111 pcBuff=pcTemp; 00112 } 00113 00114 } 00115 } 00116 Message(pcBuff); 00117 free(pcBuff); 00118 00119 //#endif 00120 return iRet; 00121 } 00122 00123 //***************************************************************************** 00124 // 00125 //! Outputs a character string to the console 00126 //! 00127 //! \param str is the pointer to the string to be printed 00128 //! 00129 //! This function 00130 //! 1. prints the input string character by character on to the console. 00131 //! 00132 //! \return none 00133 // 00134 //***************************************************************************** 00135 void 00136 Message(const char *str) 00137 { 00138 00139 uint16_t ecount = 0; 00140 00141 //#ifndef NOTERM 00142 while(!(uart.writeable())){ecount++;if(ecount>3000)break;}; 00143 00144 if(uart.writeable()) { 00145 00146 if(str != NULL){ 00147 RTOS_MUTEX_ACQUIRE(&g_printLock); 00148 while(*str!='\0') 00149 { 00150 uart.putc(*str++); 00151 } 00152 RTOS_MUTEX_RELEASE(&g_printLock); 00153 } 00154 } 00155 //#endif 00156 } 00157 00158
Generated on Wed Jul 13 2022 15:58:45 by
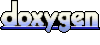