
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
cc3100_netapp.cpp
00001 /* 00002 * netapp.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "cc3100_simplelink.h" 00043 #include "cc3100_protocol.h" 00044 #include "cc3100_driver.h" 00045 00046 #include "cc3100_netapp.h" 00047 #include "fPtr_func.h" 00048 #include "cli_uart.h" 00049 00050 namespace mbed_cc3100 { 00051 00052 /*****************************************************************************/ 00053 /* Macro declarations */ 00054 /*****************************************************************************/ 00055 const uint32_t NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT = ((uint32_t)0x1 << 31); 00056 00057 #ifdef SL_TINY 00058 const uint8_t NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH = 63; 00059 #else 00060 const uint8_t NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH = 255; 00061 #endif 00062 00063 #ifndef SL_PLATFORM_MULTI_THREADED 00064 cc3100_netapp::cc3100_netapp(cc3100_driver &driver, cc3100_nonos &nonos) 00065 : _driver(driver), _nonos(nonos) 00066 { 00067 } 00068 #else 00069 cc3100_netapp::cc3100_netapp(cc3100_driver &driver) 00070 : _driver(driver) 00071 { 00072 } 00073 #endif 00074 cc3100_netapp::~cc3100_netapp() 00075 { 00076 00077 } 00078 00079 00080 /*****************************************************************************/ 00081 /* API functions */ 00082 /*****************************************************************************/ 00083 00084 /***************************************************************************** 00085 sl_NetAppStart 00086 *****************************************************************************/ 00087 typedef union { 00088 _NetAppStartStopCommand_t Cmd; 00089 _NetAppStartStopResponse_t Rsp; 00090 } _SlNetAppStartStopMsg_u; 00091 00092 #if _SL_INCLUDE_FUNC(sl_NetAppStart) 00093 const _SlCmdCtrl_t _SlNetAppStartCtrl = { 00094 SL_OPCODE_NETAPP_START_COMMAND, 00095 sizeof(_NetAppStartStopCommand_t), 00096 sizeof(_NetAppStartStopResponse_t) 00097 }; 00098 00099 int16_t cc3100_netapp::sl_NetAppStart(const uint32_t AppBitMap) 00100 { 00101 _SlNetAppStartStopMsg_u Msg; 00102 Msg.Cmd.appId = AppBitMap; 00103 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppStartCtrl, &Msg, NULL)); 00104 00105 return Msg.Rsp.status; 00106 } 00107 #endif 00108 00109 /***************************************************************************** 00110 sl_NetAppStop 00111 *****************************************************************************/ 00112 #if _SL_INCLUDE_FUNC(sl_NetAppStop) 00113 const _SlCmdCtrl_t _SlNetAppStopCtrl = 00114 { 00115 SL_OPCODE_NETAPP_STOP_COMMAND, 00116 sizeof(_NetAppStartStopCommand_t), 00117 sizeof(_NetAppStartStopResponse_t) 00118 }; 00119 int16_t cc3100_netapp::sl_NetAppStop(const uint32_t AppBitMap) 00120 { 00121 00122 _SlNetAppStartStopMsg_u Msg; 00123 Msg.Cmd.appId = AppBitMap; 00124 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppStopCtrl, &Msg, NULL)); 00125 00126 return Msg.Rsp.status; 00127 } 00128 #endif 00129 00130 00131 /******************************************************************************/ 00132 /* sl_NetAppGetServiceList */ 00133 /******************************************************************************/ 00134 typedef struct { 00135 uint8_t IndexOffest; 00136 uint8_t MaxServiceCount; 00137 uint8_t Flags; 00138 int8_t Padding; 00139 } NetappGetServiceListCMD_t; 00140 00141 typedef union { 00142 NetappGetServiceListCMD_t Cmd; 00143 _BasicResponse_t Rsp; 00144 } _SlNetappGetServiceListMsg_u; 00145 00146 #if _SL_INCLUDE_FUNC(sl_NetAppGetServiceList) 00147 const _SlCmdCtrl_t _SlGetServiceListeCtrl = { 00148 SL_OPCODE_NETAPP_NETAPP_MDNS_LOOKUP_SERVICE, 00149 sizeof(NetappGetServiceListCMD_t), 00150 sizeof(_BasicResponse_t) 00151 }; 00152 00153 int16_t cc3100_netapp::sl_NetAppGetServiceList(const uint8_t IndexOffest, 00154 const uint8_t MaxServiceCount, 00155 const uint8_t Flags, 00156 int8_t *pBuffer, 00157 const uint32_t RxBufferLength 00158 ) 00159 { 00160 int32_t retVal= 0; 00161 _SlNetappGetServiceListMsg_u Msg; 00162 _SlCmdExt_t CmdExt; 00163 uint16_t ServiceSize = 0; 00164 uint16_t BufferSize = 0; 00165 00166 /* 00167 Calculate RX pBuffer size 00168 WARNING: 00169 if this size is BufferSize than 1480 error should be returned because there 00170 is no place in the RX packet. 00171 */ 00172 switch(Flags) { 00173 case SL_NET_APP_FULL_SERVICE_WITH_TEXT_IPV4_TYPE: 00174 ServiceSize = sizeof(SlNetAppGetFullServiceWithTextIpv4List_t); 00175 break; 00176 00177 case SL_NET_APP_FULL_SERVICE_IPV4_TYPE: 00178 ServiceSize = sizeof(SlNetAppGetFullServiceIpv4List_t); 00179 break; 00180 00181 case SL_NET_APP_SHORT_SERVICE_IPV4_TYPE: 00182 ServiceSize = sizeof(SlNetAppGetShortServiceIpv4List_t); 00183 break; 00184 00185 default: 00186 ServiceSize = sizeof(_BasicResponse_t); 00187 break; 00188 } 00189 00190 00191 00192 BufferSize = MaxServiceCount * ServiceSize; 00193 00194 /*Check the size of the requested services is smaller than size of the user buffer. 00195 If not an error is returned in order to avoid overwriting memory. */ 00196 if(RxBufferLength <= BufferSize) { 00197 return SL_ERROR_NETAPP_RX_BUFFER_LENGTH_ERROR; 00198 } 00199 00200 _driver._SlDrvResetCmdExt(&CmdExt); 00201 CmdExt.RxPayloadLen = BufferSize; 00202 00203 CmdExt.pRxPayload = (uint8_t *)pBuffer; 00204 00205 Msg.Cmd.IndexOffest = IndexOffest; 00206 Msg.Cmd.MaxServiceCount = MaxServiceCount; 00207 Msg.Cmd.Flags = Flags; 00208 Msg.Cmd.Padding = 0; 00209 00210 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetServiceListeCtrl, &Msg, &CmdExt)); 00211 retVal = Msg.Rsp.status; 00212 00213 return (int16_t)retVal; 00214 } 00215 00216 #endif 00217 00218 /*****************************************************************************/ 00219 /* sl_mDNSRegisterService */ 00220 /*****************************************************************************/ 00221 /* 00222 * The below struct depicts the constant parameters of the command/API RegisterService. 00223 * 00224 1. ServiceLen - The length of the service should be smaller than NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00225 2. TextLen - The length of the text should be smaller than NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00226 3. port - The port on this target host. 00227 4. TTL - The TTL of the service 00228 5. Options - bitwise parameters: 00229 bit 0 - is unique (means if the service needs to be unique) 00230 bit 31 - for internal use if the service should be added or deleted (set means ADD). 00231 bit 1-30 for future. 00232 00233 NOTE: 00234 00235 1. There are another variable parameter is this API which is the service name and the text. 00236 2. According to now there is no warning and Async event to user on if the service is a unique. 00237 * 00238 */ 00239 00240 typedef struct { 00241 uint8_t ServiceNameLen; 00242 uint8_t TextLen; 00243 uint16_t Port; 00244 uint32_t TTL; 00245 uint32_t Options; 00246 } NetappMdnsSetService_t; 00247 00248 typedef union { 00249 NetappMdnsSetService_t Cmd; 00250 _BasicResponse_t Rsp; 00251 } _SlNetappMdnsRegisterServiceMsg_u; 00252 00253 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSRegisterUnregisterService) 00254 const _SlCmdCtrl_t _SlRegisterServiceCtrl = { 00255 SL_OPCODE_NETAPP_MDNSREGISTERSERVICE, 00256 sizeof(NetappMdnsSetService_t), 00257 sizeof(_BasicResponse_t) 00258 }; 00259 00260 /****************************************************************************** 00261 00262 sl_NetAppMDNSRegisterService 00263 00264 CALLER user from its host 00265 00266 00267 DESCRIPTION: 00268 Add/delete service 00269 The function manipulates the command that register the service and call 00270 to the NWP in order to add/delete the service to/from the mDNS package and to/from the DB. 00271 00272 This register service is a service offered by the application. 00273 This unregister service is a service offered by the application before. 00274 00275 The service name should be full service name according to RFC 00276 of the DNS-SD - means the value in name field in SRV answer. 00277 00278 Example for service name: 00279 1. PC1._ipp._tcp.local 00280 2. PC2_server._ftp._tcp.local 00281 00282 If the option is_unique is set, mDNS probes the service name to make sure 00283 it is unique before starting to announce the service on the network. 00284 Instance is the instance portion of the service name. 00285 00286 00287 00288 00289 PARAMETERS: 00290 00291 The command is from constant parameters and variables parameters. 00292 00293 Constant parameters are: 00294 00295 ServiceLen - The length of the service. 00296 TextLen - The length of the service should be smaller than 64. 00297 port - The port on this target host. 00298 TTL - The TTL of the service 00299 Options - bitwise parameters: 00300 bit 0 - is unique (means if the service needs to be unique) 00301 bit 31 - for internal use if the service should be added or deleted (set means ADD). 00302 bit 1-30 for future. 00303 00304 The variables parameters are: 00305 00306 Service name(full service name) - The service name. 00307 Example for service name: 00308 1. PC1._ipp._tcp.local 00309 2. PC2_server._ftp._tcp.local 00310 00311 Text - The description of the service. 00312 should be as mentioned in the RFC 00313 (according to type of the service IPP,FTP...) 00314 00315 NOTE - pay attention 00316 00317 1. Temporary - there is an allocation on stack of internal buffer. 00318 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00319 It means that the sum of the text length and service name length cannot be bigger than 00320 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00321 If it is - An error is returned. 00322 00323 2. According to now from certain constraints the variables parameters are set in the 00324 attribute part (contain constant parameters) 00325 00326 00327 00328 RETURNS: Status - the immediate response of the command status. 00329 0 means success. 00330 00331 00332 00333 ******************************************************************************/ 00334 int16_t cc3100_netapp::sl_NetAppMDNSRegisterUnregisterService(const char* pServiceName, 00335 const uint8_t ServiceNameLen, 00336 const char* pText, 00337 const uint8_t TextLen, 00338 const uint16_t Port, 00339 const uint32_t TTL, 00340 const uint32_t Options) 00341 { 00342 00343 _SlNetappMdnsRegisterServiceMsg_u Msg; 00344 _SlCmdExt_t CmdExt ; 00345 unsigned char ServiceNameAndTextBuffer[NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH]; 00346 unsigned char *TextPtr; 00347 00348 /* 00349 00350 NOTE - pay attention 00351 00352 1. Temporary - there is an allocation on stack of internal buffer. 00353 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00354 It means that the sum of the text length and service name length cannot be bigger than 00355 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00356 If it is - An error is returned. 00357 00358 2. According to now from certain constraints the variables parameters are set in the 00359 attribute part (contain constant parameters) 00360 00361 00362 */ 00363 00364 /*build the attribute part of the command. 00365 It contains the constant parameters of the command*/ 00366 00367 Msg.Cmd.ServiceNameLen = ServiceNameLen; 00368 Msg.Cmd.Options = Options; 00369 Msg.Cmd.Port = Port; 00370 Msg.Cmd.TextLen = TextLen; 00371 Msg.Cmd.TTL = TTL; 00372 00373 /*Build the payload part of the command 00374 Copy the service name and text to one buffer. 00375 NOTE - pay attention 00376 The size of the service length + the text length should be smaller than 255, 00377 Until the simplelink drive supports to variable length through SPI command. */ 00378 if(TextLen + ServiceNameLen > (NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH - 1 )) { /*-1 is for giving a place to set null termination at the end of the text*/ 00379 return -1; 00380 } 00381 00382 _driver._SlDrvMemZero(ServiceNameAndTextBuffer, NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH); 00383 00384 00385 /*Copy the service name*/ 00386 memcpy(ServiceNameAndTextBuffer, 00387 pServiceName, 00388 ServiceNameLen); 00389 00390 if(TextLen > 0 ) { 00391 00392 TextPtr = &ServiceNameAndTextBuffer[ServiceNameLen]; 00393 /*Copy the text just after the service name*/ 00394 memcpy(TextPtr, pText, TextLen); 00395 } 00396 00397 _driver._SlDrvResetCmdExt(&CmdExt); 00398 CmdExt.TxPayloadLen = (TextLen + ServiceNameLen); 00399 CmdExt.pTxPayload = (uint8_t *)ServiceNameAndTextBuffer; 00400 00401 00402 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlRegisterServiceCtrl, &Msg, &CmdExt)); 00403 00404 return (int16_t)Msg.Rsp.status; 00405 00406 00407 } 00408 #endif 00409 00410 /**********************************************************************************************/ 00411 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSRegisterService) 00412 int16_t cc3100_netapp::sl_NetAppMDNSRegisterService(const char* pServiceName, 00413 const uint8_t ServiceNameLen, 00414 const char* pText, 00415 const uint8_t TextLen, 00416 const uint16_t Port, 00417 const uint32_t TTL, 00418 uint32_t Options) 00419 { 00420 00421 /* 00422 00423 NOTE - pay attention 00424 00425 1. Temporary - there is an allocation on stack of internal buffer. 00426 Its size is NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00427 It means that the sum of the text length and service name length cannot be bigger than 00428 NETAPP_MDNS_MAX_SERVICE_NAME_AND_TEXT_LENGTH. 00429 If it is - An error is returned. 00430 00431 2. According to now from certain constraints the variables parameters are set in the 00432 attribute part (contain constant parameters) 00433 00434 */ 00435 00436 /*Set the add service bit in the options parameter. 00437 In order not use different opcodes for the register service and unregister service 00438 bit 31 in option is taken for this purpose. if it is set it means in NWP that the service should be added 00439 if it is cleared it means that the service should be deleted and there is only meaning to pServiceName 00440 and ServiceNameLen values. */ 00441 Options |= NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT; 00442 00443 return (sl_NetAppMDNSRegisterUnregisterService( pServiceName, ServiceNameLen, pText, TextLen, Port, TTL, Options)); 00444 00445 00446 } 00447 #endif 00448 /**********************************************************************************************/ 00449 00450 00451 00452 /**********************************************************************************************/ 00453 #if _SL_INCLUDE_FUNC(sl_NetAppMDNSUnRegisterService) 00454 int16_t cc3100_netapp::sl_NetAppMDNSUnRegisterService(const char* pServiceName, const uint8_t ServiceNameLen) 00455 { 00456 uint32_t Options = 0; 00457 00458 /* 00459 00460 NOTE - pay attention 00461 00462 The size of the service length should be smaller than 255, 00463 Until the simplelink drive supports to variable length through SPI command. 00464 00465 00466 */ 00467 00468 /*Clear the add service bit in the options parameter. 00469 In order not use different opcodes for the register service and unregister service 00470 bit 31 in option is taken for this purpose. if it is set it means in NWP that the service should be added 00471 if it is cleared it means that the service should be deleted and there is only meaning to pServiceName 00472 and ServiceNameLen values.*/ 00473 00474 Options &= (~NETAPP_MDNS_OPTIONS_ADD_SERVICE_BIT); 00475 00476 return (sl_NetAppMDNSRegisterUnregisterService(pServiceName, ServiceNameLen, NULL, 0, 0, 0, Options)); 00477 00478 00479 } 00480 #endif 00481 /**********************************************************************************************/ 00482 00483 00484 00485 /*****************************************************************************/ 00486 /* sl_DnsGetHostByService */ 00487 /*****************************************************************************/ 00488 /* 00489 * The below struct depicts the constant parameters of the command/API sl_DnsGetHostByService. 00490 * 00491 1. ServiceLen - The length of the service should be smaller than 255. 00492 2. AddrLen - TIPv4 or IPv6 (SL_AF_INET , SL_AF_INET6). 00493 * 00494 */ 00495 00496 typedef struct { 00497 uint8_t ServiceLen; 00498 uint8_t AddrLen; 00499 uint16_t Padding; 00500 } _GetHostByServiceCommand_t; 00501 00502 00503 00504 /* 00505 * The below structure depict the constant parameters that are returned in the Async event answer 00506 * according to command/API sl_DnsGetHostByService for IPv4 and IPv6. 00507 * 00508 1Status - The status of the response. 00509 2.Address - Contains the IP address of the service. 00510 3.Port - Contains the port of the service. 00511 4.TextLen - Contains the max length of the text that the user wants to get. 00512 it means that if the test of service is bigger that its value than 00513 the text is cut to inout_TextLen value. 00514 Output: Contain the length of the text that is returned. Can be full text or part 00515 of the text (see above). 00516 00517 * 00518 00519 typedef struct { 00520 uint16_t Status; 00521 uint16_t TextLen; 00522 uint32_t Port; 00523 uint32_t Address; 00524 } _GetHostByServiceIPv4AsyncResponse_t; 00525 */ 00526 00527 typedef struct { 00528 uint16_t Status; 00529 uint16_t TextLen; 00530 uint32_t Port; 00531 uint32_t Address[4]; 00532 } _GetHostByServiceIPv6AsyncResponse_t; 00533 00534 00535 typedef union { 00536 _GetHostByServiceIPv4AsyncResponse_t IpV4; 00537 _GetHostByServiceIPv6AsyncResponse_t IpV6; 00538 } _GetHostByServiceAsyncResponseAttribute_u; 00539 00540 typedef union { 00541 _GetHostByServiceCommand_t Cmd; 00542 _BasicResponse_t Rsp; 00543 } _SlGetHostByServiceMsg_u; 00544 00545 #if _SL_INCLUDE_FUNC(sl_NetAppDnsGetHostByService) 00546 const _SlCmdCtrl_t _SlGetHostByServiceCtrl = { 00547 SL_OPCODE_NETAPP_MDNSGETHOSTBYSERVICE, 00548 sizeof(_GetHostByServiceCommand_t), 00549 sizeof(_BasicResponse_t) 00550 }; 00551 00552 int32_t cc3100_netapp::sl_NetAppDnsGetHostByService(unsigned char *pServiceName, /* string containing all (or only part): name + subtype + service */ 00553 const uint8_t ServiceLen, 00554 const uint8_t Family, /* 4-IPv4 , 16-IPv6 */ 00555 uint32_t pAddr[], 00556 uint32_t *pPort, 00557 uint16_t *pTextLen, /* in: max len , out: actual len */ 00558 unsigned char *pText) 00559 { 00560 00561 _SlGetHostByServiceMsg_u Msg; 00562 _SlCmdExt_t CmdExt ; 00563 _GetHostByServiceAsyncResponse_t AsyncRsp; 00564 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00565 00566 /* 00567 Note: 00568 1. The return's attributes are belonged to first service that is found. 00569 It can be other services with the same service name will response to 00570 the query. The results of these responses are saved in the peer cache of the NWP, and 00571 should be read by another API. 00572 00573 2. Text length can be 120 bytes only - not more 00574 It is because of constraints in the NWP on the buffer that is allocated for the Async event. 00575 00576 3.The API waits to Async event by blocking. It means that the API is finished only after an Async event 00577 is sent by the NWP. 00578 00579 4.No rolling option!!! - only PTR type is sent. 00580 00581 00582 */ 00583 /*build the attribute part of the command. 00584 It contains the constant parameters of the command */ 00585 00586 Msg.Cmd.ServiceLen = ServiceLen; 00587 Msg.Cmd.AddrLen = Family; 00588 00589 /*Build the payload part of the command 00590 Copy the service name and text to one buffer.*/ 00591 _driver._SlDrvResetCmdExt(&CmdExt); 00592 CmdExt.TxPayloadLen = ServiceLen; 00593 00594 CmdExt.pTxPayload = (uint8_t *)pServiceName; 00595 00596 00597 /*set pointers to the output parameters (the returned parameters). 00598 This pointers are belonged to local struct that is set to global Async response parameter. 00599 It is done in order not to run more than one sl_DnsGetHostByService at the same time. 00600 The API should be run only if global parameter is pointed to NULL. */ 00601 AsyncRsp.out_pText = pText; 00602 AsyncRsp.inout_TextLen = (uint16_t* )pTextLen; 00603 AsyncRsp.out_pPort = pPort; 00604 AsyncRsp.out_pAddr = (uint32_t *)pAddr; 00605 00606 00607 ObjIdx = _driver._SlDrvProtectAsyncRespSetting((uint8_t*)&AsyncRsp, GETHOSYBYSERVICE_ID, SL_MAX_SOCKETS); 00608 00609 if (MAX_CONCURRENT_ACTIONS == ObjIdx) 00610 { 00611 return SL_POOL_IS_EMPTY; 00612 } 00613 00614 if (SL_AF_INET6 == Family) { 00615 g_pCB->ObjPool[ObjIdx].AdditionalData |= SL_NETAPP_FAMILY_MASK; 00616 } 00617 /* Send the command */ 00618 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetHostByServiceCtrl, &Msg, &CmdExt)); 00619 00620 00621 00622 /* If the immediate reponse is O.K. than wait for aSYNC event response. */ 00623 if(SL_RET_CODE_OK == Msg.Rsp.status) { 00624 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00625 00626 /* If we are - it means that Async event was sent. 00627 The results are copied in the Async handle return functions */ 00628 00629 Msg.Rsp.status = AsyncRsp.Status; 00630 } 00631 00632 _driver._SlDrvReleasePoolObj(ObjIdx); 00633 return Msg.Rsp.status; 00634 } 00635 #endif 00636 00637 /*****************************************************************************/ 00638 /* _sl_HandleAsync_DnsGetHostByAddr */ 00639 /*****************************************************************************/ 00640 #ifndef SL_TINY_EXT 00641 void cc3100_netapp::_sl_HandleAsync_DnsGetHostByAddr(void *pVoidBuf) 00642 { 00643 SL_TRACE0(DBG_MSG, MSG_303, "STUB: _sl_HandleAsync_DnsGetHostByAddr not implemented yet!"); 00644 return; 00645 } 00646 #endif 00647 /*****************************************************************************/ 00648 /* sl_DnsGetHostByName */ 00649 /*****************************************************************************/ 00650 typedef union { 00651 _GetHostByNameIPv4AsyncResponse_t IpV4; 00652 _GetHostByNameIPv6AsyncResponse_t IpV6; 00653 } _GetHostByNameAsyncResponse_u; 00654 00655 typedef union { 00656 _GetHostByNameCommand_t Cmd; 00657 _BasicResponse_t Rsp; 00658 } _SlGetHostByNameMsg_u; 00659 00660 #if _SL_INCLUDE_FUNC(sl_NetAppDnsGetHostByName) 00661 const _SlCmdCtrl_t _SlGetHostByNameCtrl = { 00662 SL_OPCODE_NETAPP_DNSGETHOSTBYNAME, 00663 sizeof(_GetHostByNameCommand_t), 00664 sizeof(_BasicResponse_t) 00665 }; 00666 00667 int16_t cc3100_netapp::sl_NetAppDnsGetHostByName(unsigned char * hostname, const uint16_t usNameLen, uint32_t* out_ip_addr, const uint8_t family) 00668 { 00669 _SlGetHostByNameMsg_u Msg; 00670 _SlCmdExt_t ExtCtrl; 00671 _GetHostByNameAsyncResponse_u AsyncRsp; 00672 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00673 00674 _driver._SlDrvResetCmdExt(&ExtCtrl); 00675 ExtCtrl.TxPayloadLen = usNameLen; 00676 00677 ExtCtrl.pTxPayload = (unsigned char *)hostname; 00678 00679 00680 Msg.Cmd.Len = usNameLen; 00681 Msg.Cmd.family = family; 00682 00683 /*Use Obj to issue the command, if not available try later */ 00684 ObjIdx = (uint8_t)_driver._SlDrvWaitForPoolObj(GETHOSYBYNAME_ID,SL_MAX_SOCKETS); 00685 if (MAX_CONCURRENT_ACTIONS == ObjIdx) { 00686 printf("SL_POOL_IS_EMPTY \r\n"); 00687 return SL_POOL_IS_EMPTY; 00688 } 00689 00690 _driver._SlDrvProtectionObjLockWaitForever(); 00691 00692 g_pCB->ObjPool[ObjIdx].pRespArgs = (uint8_t *)&AsyncRsp; 00693 /*set bit to indicate IPv6 address is expected */ 00694 if (SL_AF_INET6 == family) { 00695 g_pCB->ObjPool[ObjIdx].AdditionalData |= SL_NETAPP_FAMILY_MASK; 00696 } 00697 00698 _driver._SlDrvProtectionObjUnLock(); 00699 00700 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlGetHostByNameCtrl, &Msg, &ExtCtrl)); 00701 00702 if(SL_RET_CODE_OK == Msg.Rsp.status) { 00703 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00704 Msg.Rsp.status = AsyncRsp.IpV4.status; 00705 00706 if(SL_OS_RET_CODE_OK == (int16_t)Msg.Rsp.status) { 00707 memcpy((int8_t *)out_ip_addr, (signed char *)&AsyncRsp.IpV4.ip0, (SL_AF_INET == family) ? SL_IPV4_ADDRESS_SIZE : SL_IPV6_ADDRESS_SIZE); 00708 } 00709 } 00710 _driver._SlDrvReleasePoolObj(ObjIdx); 00711 return Msg.Rsp.status; 00712 } 00713 #endif 00714 00715 #ifndef SL_TINY_EXT 00716 void cc3100_netapp::CopyPingResultsToReport(_PingReportResponse_t *pResults,SlPingReport_t *pReport) 00717 { 00718 pReport->PacketsSent = pResults->numSendsPings; 00719 pReport->PacketsReceived = pResults->numSuccsessPings; 00720 pReport->MinRoundTime = pResults->rttMin; 00721 pReport->MaxRoundTime = pResults->rttMax; 00722 pReport->AvgRoundTime = pResults->rttAvg; 00723 pReport->TestTime = pResults->testTime; 00724 } 00725 #endif 00726 /*****************************************************************************/ 00727 /* sl_PingStart */ 00728 /*****************************************************************************/ 00729 typedef union { 00730 _PingStartCommand_t Cmd; 00731 _PingReportResponse_t Rsp; 00732 } _SlPingStartMsg_u; 00733 00734 00735 typedef enum { 00736 CMD_PING_TEST_RUNNING = 0, 00737 CMD_PING_TEST_STOPPED 00738 } _SlPingStatus_e; 00739 00740 P_SL_DEV_PING_CALLBACK pPingCallBackFunc; 00741 00742 #if _SL_INCLUDE_FUNC(sl_NetAppPingStart) 00743 int16_t cc3100_netapp::sl_NetAppPingStart(const SlPingStartCommand_t* pPingParams, const uint8_t family, SlPingReport_t *pReport, const P_SL_DEV_PING_CALLBACK pPingCallback) 00744 { 00745 00746 _SlCmdCtrl_t CmdCtrl = {0, sizeof(_PingStartCommand_t), sizeof(_BasicResponse_t)}; 00747 _SlPingStartMsg_u Msg; 00748 _PingReportResponse_t PingRsp; 00749 uint8_t ObjIdx = MAX_CONCURRENT_ACTIONS; 00750 00751 if( 0 == pPingParams->Ip ) 00752 { /* stop any ongoing ping */ 00753 return _driver._SlDrvBasicCmd(SL_OPCODE_NETAPP_PINGSTOP); 00754 } 00755 00756 if(SL_AF_INET == family) { 00757 CmdCtrl.Opcode = SL_OPCODE_NETAPP_PINGSTART; 00758 memcpy(&Msg.Cmd.ip0, &pPingParams->Ip, SL_IPV4_ADDRESS_SIZE); 00759 } else { 00760 CmdCtrl.Opcode = SL_OPCODE_NETAPP_PINGSTART_V6; 00761 memcpy(&Msg.Cmd.ip0, &pPingParams->Ip, SL_IPV6_ADDRESS_SIZE); 00762 } 00763 00764 Msg.Cmd.pingIntervalTime = pPingParams->PingIntervalTime; 00765 Msg.Cmd.PingSize = pPingParams->PingSize; 00766 Msg.Cmd.pingRequestTimeout = pPingParams->PingRequestTimeout; 00767 Msg.Cmd.totalNumberOfAttempts = pPingParams->TotalNumberOfAttempts; 00768 Msg.Cmd.flags = pPingParams->Flags; 00769 00770 if( pPingCallback ) { 00771 pPingCallBackFunc = pPingCallback; 00772 } else { 00773 /*Use Obj to issue the command, if not available try later */ 00774 ObjIdx = (uint8_t)_driver._SlDrvWaitForPoolObj(PING_ID,SL_MAX_SOCKETS); 00775 if (MAX_CONCURRENT_ACTIONS == ObjIdx) { 00776 return SL_POOL_IS_EMPTY; 00777 } 00778 #ifndef SL_PLATFORM_MULTI_THREADED 00779 OSI_RET_OK_CHECK(_nonos.sl_LockObjLock(&g_pCB->ProtectionLockObj, SL_OS_WAIT_FOREVER)); 00780 #else 00781 OSI_RET_OK_CHECK(sl_LockObjLock(&g_pCB->ProtectionLockObj, SL_OS_WAIT_FOREVER)); 00782 #endif 00783 /* async response handler for non callback mode */ 00784 g_pCB->ObjPool[ObjIdx].pRespArgs = (uint8_t *)&PingRsp; 00785 pPingCallBackFunc = NULL; 00786 #ifndef SL_PLATFORM_MULTI_THREADED 00787 OSI_RET_OK_CHECK(_nonos.sl_LockObjUnlock(&g_pCB->ProtectionLockObj)); 00788 #else 00789 OSI_RET_OK_CHECK(sl_LockObjUnlock(&g_pCB->ProtectionLockObj)); 00790 #endif 00791 } 00792 00793 00794 VERIFY_RET_OK(_driver._SlDrvCmdOp(&CmdCtrl, &Msg, NULL)); 00795 /*send the command*/ 00796 if(CMD_PING_TEST_RUNNING == (int16_t)Msg.Rsp.status || CMD_PING_TEST_STOPPED == (int16_t)Msg.Rsp.status ) { 00797 /* block waiting for results if no callback function is used */ 00798 if( NULL == pPingCallback ) { 00799 _driver._SlDrvSyncObjWaitForever(&g_pCB->ObjPool[ObjIdx].SyncObj); 00800 if( SL_OS_RET_CODE_OK == (int16_t)PingRsp.status ) { 00801 CopyPingResultsToReport(&PingRsp,pReport); 00802 } 00803 _driver._SlDrvReleasePoolObj(ObjIdx); 00804 } 00805 } else { 00806 /* ping failure, no async response */ 00807 if( NULL == pPingCallback ) { 00808 _driver._SlDrvReleasePoolObj(ObjIdx); 00809 } 00810 } 00811 00812 return Msg.Rsp.status; 00813 } 00814 #endif 00815 00816 /*****************************************************************************/ 00817 /* sl_NetAppSet */ 00818 /*****************************************************************************/ 00819 typedef union { 00820 _NetAppSetGet_t Cmd; 00821 _BasicResponse_t Rsp; 00822 } _SlNetAppMsgSet_u; 00823 00824 #if _SL_INCLUDE_FUNC(sl_NetAppSet) 00825 const _SlCmdCtrl_t _SlNetAppSetCmdCtrl = { 00826 SL_OPCODE_NETAPP_NETAPPSET, 00827 sizeof(_NetAppSetGet_t), 00828 sizeof(_BasicResponse_t) 00829 }; 00830 00831 int32_t cc3100_netapp::sl_NetAppSet(const uint8_t AppId ,const uint8_t Option, const uint8_t OptionLen, const uint8_t *pOptionValue) 00832 { 00833 00834 _SlNetAppMsgSet_u Msg; 00835 _SlCmdExt_t CmdExt; 00836 00837 _driver._SlDrvResetCmdExt(&CmdExt); 00838 CmdExt.TxPayloadLen = (OptionLen+3) & (~3); 00839 00840 CmdExt.pTxPayload = (uint8_t *)pOptionValue; 00841 00842 Msg.Cmd.AppId = AppId; 00843 Msg.Cmd.ConfigLen = OptionLen; 00844 Msg.Cmd.ConfigOpt = Option; 00845 00846 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppSetCmdCtrl, &Msg, &CmdExt)); 00847 00848 return (int16_t)Msg.Rsp.status; 00849 } 00850 #endif 00851 00852 /*****************************************************************************/ 00853 /* sl_NetAppSendTokenValue */ 00854 /*****************************************************************************/ 00855 typedef union { 00856 sl_NetAppHttpServerSendToken_t Cmd; 00857 _BasicResponse_t Rsp; 00858 } _SlNetAppMsgSendTokenValue_u; 00859 00860 #if defined(sl_HttpServerCallback) || defined(EXT_LIB_REGISTERED_HTTP_SERVER_EVENTS) 00861 const _SlCmdCtrl_t _SlNetAppSendTokenValueCmdCtrl = { 00862 SL_OPCODE_NETAPP_HTTPSENDTOKENVALUE, 00863 sizeof(sl_NetAppHttpServerSendToken_t), 00864 sizeof(_BasicResponse_t) 00865 }; 00866 00867 uint16_t cc3100_netapp::sl_NetAppSendTokenValue(slHttpServerData_t * Token_value) 00868 { 00869 00870 _SlNetAppMsgSendTokenValue_u Msg; 00871 _SlCmdExt_t CmdExt; 00872 00873 CmdExt.TxPayloadLen = (Token_value->value_len+3) & (~3); 00874 CmdExt.RxPayloadLen = 0; 00875 CmdExt.pTxPayload = (uint8_t *) Token_value->token_value; 00876 CmdExt.pRxPayload = NULL; 00877 00878 Msg.Cmd.token_value_len = Token_value->value_len; 00879 Msg.Cmd.token_name_len = Token_value->name_len; 00880 memcpy(&Msg.Cmd.token_name[0], Token_value->token_name, Token_value->name_len); 00881 00882 00883 VERIFY_RET_OK(_driver._SlDrvCmdSend((_SlCmdCtrl_t *)&_SlNetAppSendTokenValueCmdCtrl, &Msg, &CmdExt)); 00884 00885 return Msg.Rsp.status; 00886 } 00887 #endif 00888 00889 /*****************************************************************************/ 00890 /* sl_NetAppGet */ 00891 /*****************************************************************************/ 00892 typedef union { 00893 _NetAppSetGet_t Cmd; 00894 _NetAppSetGet_t Rsp; 00895 } _SlNetAppMsgGet_u; 00896 00897 #if _SL_INCLUDE_FUNC(sl_NetAppGet) 00898 const _SlCmdCtrl_t _SlNetAppGetCmdCtrl = { 00899 SL_OPCODE_NETAPP_NETAPPGET, 00900 sizeof(_NetAppSetGet_t), 00901 sizeof(_NetAppSetGet_t) 00902 }; 00903 00904 int32_t cc3100_netapp::sl_NetAppGet(const uint8_t AppId, const uint8_t Option, uint8_t *pOptionLen, uint8_t *pOptionValue) 00905 { 00906 _SlNetAppMsgGet_u Msg; 00907 _SlCmdExt_t CmdExt; 00908 00909 if (*pOptionLen == 0) { 00910 return SL_EZEROLEN; 00911 } 00912 00913 _driver._SlDrvResetCmdExt(&CmdExt); 00914 CmdExt.RxPayloadLen = *pOptionLen; 00915 00916 CmdExt.pRxPayload = (uint8_t *)pOptionValue; 00917 00918 Msg.Cmd.AppId = AppId; 00919 Msg.Cmd.ConfigOpt = Option; 00920 VERIFY_RET_OK(_driver._SlDrvCmdOp((_SlCmdCtrl_t *)&_SlNetAppGetCmdCtrl, &Msg, &CmdExt)); 00921 00922 00923 if (CmdExt.RxPayloadLen < CmdExt.ActualRxPayloadLen) { 00924 *pOptionLen = (uint8_t)CmdExt.RxPayloadLen; 00925 return SL_ESMALLBUF; 00926 } else { 00927 *pOptionLen = (uint8_t)CmdExt.ActualRxPayloadLen; 00928 } 00929 00930 return (int16_t)Msg.Rsp.Status; 00931 } 00932 #endif 00933 00934 #ifndef SL_PLATFORM_MULTI_THREADED 00935 cc3100_flowcont::cc3100_flowcont(cc3100_driver &driver, cc3100_nonos &nonos) 00936 : _driver(driver), _nonos(nonos) 00937 { 00938 } 00939 #else 00940 cc3100_flowcont::cc3100_flowcont(cc3100_driver &driver) 00941 : _driver(driver) 00942 { 00943 } 00944 #endif 00945 cc3100_flowcont::~cc3100_flowcont() 00946 { 00947 00948 } 00949 #if 0 00950 /*****************************************************************************/ 00951 /* _SlDrvFlowContInit */ 00952 /*****************************************************************************/ 00953 void cc3100_flowcont::_SlDrvFlowContInit(void) 00954 { 00955 g_pCB->FlowContCB.TxPoolCnt = FLOW_CONT_MIN; 00956 00957 OSI_RET_OK_CHECK(_nonos.sl_LockObjCreate(&g_pCB->FlowContCB.TxLockObj, "TxLockObj")); 00958 00959 OSI_RET_OK_CHECK(_nonos.sl_SyncObjCreate(&g_pCB->FlowContCB.TxSyncObj, "TxSyncObj")); 00960 } 00961 00962 /*****************************************************************************/ 00963 /* _SlDrvFlowContDeinit */ 00964 /*****************************************************************************/ 00965 void cc3100_flowcont::_SlDrvFlowContDeinit(void) 00966 { 00967 g_pCB->FlowContCB.TxPoolCnt = 0; 00968 00969 OSI_RET_OK_CHECK(_nonos.sl_LockObjDelete(&g_pCB->FlowContCB.TxLockObj, 0)); 00970 00971 OSI_RET_OK_CHECK(_nonos.sl_SyncObjDelete(&g_pCB->FlowContCB.TxSyncObj, 0)); 00972 } 00973 #endif 00974 }//namespace mbed_cc3100 00975 00976
Generated on Wed Jul 13 2022 15:58:45 by
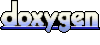