
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
HttpString.h
00001 //***************************************************************************** 00002 // Copyright (C) 2014 Texas Instruments Incorporated 00003 // 00004 // All rights reserved. Property of Texas Instruments Incorporated. 00005 // Restricted rights to use, duplicate or disclose this code are 00006 // granted through contract. 00007 // The program may not be used without the written permission of 00008 // Texas Instruments Incorporated or against the terms and conditions 00009 // stipulated in the agreement under which this program has been supplied, 00010 // and under no circumstances can it be used with non-TI connectivity device. 00011 // 00012 //***************************************************************************** 00013 00014 #ifndef _HTTP_STRING_H_ 00015 #define _HTTP_STRING_H_ 00016 00017 /** 00018 * @defgroup HttpString String routines helper module 00019 * This module implements some string and buffer-related helper routines 00020 * 00021 * @{ 00022 */ 00023 00024 #include "datatypes.h" 00025 00026 #ifdef __cplusplus 00027 extern "C" { 00028 #endif 00029 00030 struct HttpBlob 00031 { 00032 UINT16 uLength; 00033 UINT8* pData; 00034 }; 00035 00036 /** 00037 * Utility macro which sets an existing blob 00038 */ 00039 #define HttpBlobSetConst(blob, constString) \ 00040 { \ 00041 (blob).pData = (UINT8*)constString; \ 00042 (blob).uLength = sizeof(constString) - 1; \ 00043 } 00044 00045 static void ToLower(char * str, UINT16 len); 00046 00047 /** 00048 * Perform string comparison between two strings. 00049 * @param first Pointer to data about the first blob or string 00050 * @param second Pointer to data about the second blob or string 00051 * @return zero if equal, positive if first is later in order, negative if second is later in order 00052 */ 00053 int HttpString_strcmp(struct HttpBlob first, struct HttpBlob second); 00054 00055 /** 00056 * return pointer to the next token 00057 * @param token Pointer to data of the first token 00058 * @param blob Pointer to data of the search string/blob 00059 * @return pointer if found, otherwize NULL 00060 */ 00061 UINT8* HttpString_nextToken(char* pToken, UINT16 uTokenLength, struct HttpBlob blob); 00062 00063 UINT8* HttpString_nextDelimiter(char* pToken, UINT16 uTokenLength, struct HttpBlob blob); 00064 00065 /** 00066 * Parse a string representation of an unsigned decimal number 00067 * Stops at any non-digit character. 00068 * @param string The string to parse 00069 * @return The parsed value 00070 */ 00071 UINT32 HttpString_atou(struct HttpBlob string); 00072 00073 /** 00074 * Format an unsigned decimal number as a string 00075 * @param uNum The number to format 00076 * @param[in,out] pString A string buffer which returns the formatted string. On entry, uLength is the maximum length of the buffer, upon return uLength is the actual length of the formatted string 00077 */ 00078 void HttpString_utoa(UINT32 uNum, struct HttpBlob* pString); 00079 00080 /** 00081 * Format an unsigned decimal number as an hexdecimal string 00082 * @param uNum The number to format 00083 * @param bPadZero nonzero to pad with zeros up to the string length, or zero to use minimal length required to represent the number 00084 * @param[in,out] pString A string buffer which returns the formatted string. On entry, uLength is the maximum length of the buffer, upon return uLength is the actual length of the formatted string 00085 */ 00086 void HttpString_htoa(UINT32 uNum, struct HttpBlob* pString, UINT8 bPadZero); 00087 00088 /// @} 00089 #ifdef __cplusplus 00090 } 00091 #endif /* __cplusplus */ 00092 #endif // _HTTP_STRING_H_ 00093
Generated on Wed Jul 13 2022 15:58:45 by
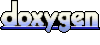