
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
ArduCAM.h
00001 /* 00002 ArduCAM.h - Arduino library support for CMOS Image Sensor 00003 Copyright (C)2011-2015 ArduCAM.com. All right reserved 00004 00005 Basic functionality of this library are based on the demo-code provided by 00006 ArduCAM.com. You can find the latest version of the library at 00007 http://www.ArduCAM.com 00008 00009 Now supported controllers: 00010 - OV7670 00011 - MT9D111 00012 - OV7675 00013 - OV2640 00014 - OV3640 00015 - OV5642 00016 - OV7660 00017 - OV7725 00018 - MT9M112 00019 - MT9V111 00020 - OV5640 00021 - MT9M001 00022 00023 We will add support for many other sensors in next release. 00024 00025 Supported MCU platform 00026 - Theoretically support all Arduino families 00027 - Arduino UNO R3 (Tested) 00028 - Arduino MEGA2560 R3 (Tested) 00029 - Arduino Leonardo R3 (Tested) 00030 - Arduino Nano (Tested) 00031 - Arduino DUE (Tested) 00032 - Arduino Yun (Tested) 00033 - Raspberry Pi (Tested) 00034 00035 00036 If you make any modifications or improvements to the code, I would appreciate 00037 that you share the code with me so that I might include it in the next release. 00038 I can be contacted through http://www.ArduCAM.com 00039 00040 This library is free software; you can redistribute it and/or 00041 modify it under the terms of the GNU Lesser General Public 00042 License as published by the Free Software Foundation; either 00043 version 2.1 of the License, or (at your option) any later version. 00044 00045 This library is distributed in the hope that it will be useful, 00046 but WITHOUT ANY WARRANTY; without even the implied warranty of 00047 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00048 Lesser General Public License for more details. 00049 00050 You should have received a copy of the GNU Lesser General Public 00051 License along with this library; if not, write to the Free Software 00052 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00053 */ 00054 00055 /*------------------------------------ 00056 Revision History: 00057 2012/09/20 V1.0.0 by Lee first release 00058 2012/10/23 V1.0.1 by Lee Resolved some timing issue for the Read/Write Register 00059 2012/11/29 V1.1.0 by Lee Add support for MT9D111 sensor 00060 2012/12/13 V1.2.0 by Lee Add support for OV7675 sensor 00061 2012/12/28 V1.3.0 by Lee Add support for OV2640,OV3640,OV5642 sensors 00062 2013/02/26 V2.0.0 by Lee New Rev.B shield hardware, add support for FIFO control 00063 and support Mega1280/2560 boards 00064 2013/05/28 V2.1.0 by Lee Add support all drawing functions derived from UTFT library 00065 2013/08/24 V3.0.0 by Lee Support ArudCAM shield Rev.C hardware, features SPI interface and low power mode. 00066 Support almost all series of Arduino boards including DUE. 00067 2014/03/09 V3.1.0 by Lee Add the more impressive example sketches. 00068 Optimise the OV5642 settings, improve image quality. 00069 Add live preview before JPEG capture. 00070 Add play back photos one by one after BMP capture. 00071 2014/05/01 V3.1.1 by Lee Minor changes to add support Arduino IDE for linux distributions. 00072 2014/09/30 V3.2.0 by Lee Improvement on OV5642 camera dirver. 00073 2014/10/06 V3.3.0 by Lee Add OV7660,OV7725 camera support. 00074 2015/02/27 V3.4.0 by Lee Add the support for Arduino Yun board, update the latest UTFT library for ArduCAM. 00075 2015/06/09 V3.4.1 by Lee Minor changes and add some comments 00076 2015/06/19 V3.4.2 by Lee Add support for MT9M112 camera. 00077 2015/06/20 V3.4.3 by Lee Add support for MT9V111 camera. 00078 2015/06/22 V3.4.4 by Lee Add support for OV5640 camera. 00079 2015/06/22 V3.4.5 by Lee Add support for MT9M001 camera. 00080 --------------------------------------*/ 00081 00082 00083 #ifndef ArduCAM_H 00084 #define ArduCAM_H 00085 00086 #include "mbed.h" 00087 00088 /****************************************************/ 00089 /* Sensor related definition */ 00090 /****************************************************/ 00091 #define BMP 0 00092 #define JPEG 1 00093 00094 #define OV7670 0 00095 #define MT9D111_A 1 00096 #define OV7675 2 00097 #define OV5642 3 00098 #define OV3640 4 00099 #define OV2640 5 00100 #define OV9655 6 00101 #define MT9M112 7 00102 #define OV7725 8 00103 #define OV7660 9 00104 #define MT9M001 10 00105 #define OV5640 11 00106 #define MT9D111_B 12 00107 #define OV9650 13 00108 #define MT9V111 14 00109 00110 #define OV2640_160x120 0 //160x120 00111 #define OV2640_176x144 1 //176x144 00112 #define OV2640_320x240 2 //320x240 00113 #define OV2640_352x288 3 //352x288 00114 #define OV2640_640x480 4 //640x480 00115 #define OV2640_800x600 5 //800x600 00116 #define OV2640_1024x768 6 //1024x768 00117 #define OV2640_1280x1024 7 //1280x1024 00118 #define OV2640_1600x1200 8 //1600x1200 00119 00120 #define OV5642_320x240 1 //320x240 00121 #define OV5642_640x480 2 //640x480 00122 #define OV5642_1280x720 3 //1280x720 00123 #define OV5642_1920x1080 4 //1920x1080 00124 #define OV5642_2048x1563 5 //2048x1563 00125 #define OV5642_2592x1944 6 //2592x1944 00126 00127 /****************************************************/ 00128 /* ArduChip related definition */ 00129 /****************************************************/ 00130 #define RWBIT 0x80 //READ AND WRITE BIT IS BIT[7] 00131 00132 #define ARDUCHIP_TEST1 0x00 //TEST register 00133 #define ARDUCHIP_TEST2 0x01 //TEST register 00134 00135 #define ARDUCHIP_FRAMES 0x01 //Bit[2:0]Number of frames to be captured 00136 00137 #define ARDUCHIP_MODE 0x02 //Mode register 00138 #define MCU2LCD_MODE 0x00 00139 #define CAM2LCD_MODE 0x01 00140 #define LCD2MCU_MODE 0x02 00141 00142 #define ARDUCHIP_TIM 0x03 //Timming control 00143 #define HREF_LEVEL_MASK 0x01 //0 = High active , 1 = Low active 00144 #define VSYNC_LEVEL_MASK 0x02 //0 = High active , 1 = Low active 00145 #define LCD_BKEN_MASK 0x04 //0 = Enable, 1 = Disable 00146 #define DELAY_MASK 0x08 //0 = no delay, 1 = delay one clock 00147 #define MODE_MASK 0x10 //0 = LCD mode, 1 = FIFO mode 00148 #define FIFO_PWRDN_MASK 0x20 //0 = Normal operation, 1 = FIFO power down 00149 #define LOW_POWER_MODE 0x40 //0 = Normal mode, 1 = Low power mode 00150 00151 #define ARDUCHIP_FIFO 0x04 //FIFO and I2C control 00152 #define FIFO_CLEAR_MASK 0x01 00153 #define FIFO_START_MASK 0x02 00154 #define FIFO_RDPTR_RST_MASK 0x10 00155 #define FIFO_WRPTR_RST_MASK 0x20 00156 00157 #define ARDUCHIP_GPIO 0x06 //GPIO Write Register 00158 #define GPIO_RESET_MASK 0x01 //0 = default state, 1 = Sensor reset IO value 00159 #define GPIO_PWDN_MASK 0x02 //0 = Sensor power down IO value, 1 = Sensor power enable IO value 00160 00161 #define BURST_FIFO_READ 0x3C //Burst FIFO read operation 00162 #define SINGLE_FIFO_READ 0x3D //Single FIFO read operation 00163 00164 #define ARDUCHIP_REV 0x40 //ArduCHIP revision 00165 #define VER_LOW_MASK 0x3F 00166 #define VER_HIGH_MASK 0xC0 00167 00168 #define ARDUCHIP_TRIG 0x41 //Trigger source 00169 #define VSYNC_MASK 0x01 00170 #define SHUTTER_MASK 0x02 00171 #define CAP_DONE_MASK 0x08 00172 00173 #define FIFO_SIZE1 0x42 //Camera write FIFO size[7:0] for burst to read 00174 #define FIFO_SIZE2 0x43 //Camera write FIFO size[15:8] 00175 #define FIFO_SIZE3 0x44 //Camera write FIFO size[18:16] 00176 00177 00178 /****************************************************/ 00179 00180 00181 /****************************************************************/ 00182 /* define a structure for sensor register initialization values */ 00183 /****************************************************************/ 00184 struct sensor_reg { 00185 uint16_t reg; 00186 uint16_t val; 00187 }; 00188 00189 class ArduCAM 00190 { 00191 public: 00192 00193 ArduCAM(uint8_t model,PinName cam_cs, SPI cam_spi, I2C cam_i2c); 00194 00195 ~ArduCAM(); 00196 00197 void InitCAM(); 00198 00199 void cs_high(void); 00200 void cs_low(void); 00201 00202 void flush_fifo(void); 00203 void start_capture(void); 00204 void clear_fifo_flag(void); 00205 uint8_t read_fifo(void); 00206 00207 uint8_t read_reg(uint8_t addr); 00208 void write_reg(uint8_t addr, uint8_t data); 00209 00210 uint32_t read_fifo_length(void); 00211 void set_fifo_burst(void); 00212 void set_bit(uint8_t addr, uint8_t bit); 00213 void clear_bit(uint8_t addr, uint8_t bit); 00214 uint8_t get_bit(uint8_t addr, uint8_t bit); 00215 void set_mode(uint8_t mode); 00216 00217 int wrSensorRegs(const struct sensor_reg*); 00218 int wrSensorRegs8_8(const struct sensor_reg*); 00219 int wrSensorRegs8_16(const struct sensor_reg*); 00220 int wrSensorRegs16_8(const struct sensor_reg*); 00221 int wrSensorRegs16_16(const struct sensor_reg*); 00222 00223 uint8_t wrSensorReg(int regID, int regDat); 00224 uint8_t wrSensorReg8_8(int regID, int regDat); 00225 uint8_t wrSensorReg8_16(int regID, int regDat); 00226 uint8_t wrSensorReg16_8(int regID, int regDat); 00227 uint8_t wrSensorReg16_16(int regID, int regDat); 00228 00229 uint8_t rdSensorReg8_8(uint8_t regID, uint8_t* regDat); 00230 uint8_t rdSensorReg16_8(uint16_t regID, uint8_t* regDat); 00231 uint8_t rdSensorReg8_16(uint8_t regID, uint16_t* regDat); 00232 uint8_t rdSensorReg16_16(uint16_t regID, uint16_t* regDat); 00233 00234 void OV5642_set_JPEG_size(uint8_t size); 00235 void set_format(uint8_t fmt); 00236 00237 int bus_write(int address, int value); 00238 uint8_t bus_read(int address); 00239 00240 protected: 00241 uint8_t m_fmt; 00242 uint8_t sensor_model; 00243 uint8_t sensor_addr; 00244 00245 public: 00246 DigitalOut _cam_cs; 00247 SPI _cam_spi; 00248 I2C _cam_i2c; 00249 }; 00250 00251 #endif 00252
Generated on Wed Jul 13 2022 15:58:45 by
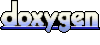