
TI's MQTT Demo with freertos CM4F
Embed:
(wiki syntax)
Show/hide line numbers
server_util.cpp
00001 /****************************************************************************** 00002 * 00003 * Copyright (C) 2014 Texas Instruments Incorporated 00004 * 00005 * All rights reserved. Property of Texas Instruments Incorporated. 00006 * Restricted rights to use, duplicate or disclose this code are 00007 * granted through contract. 00008 * 00009 * The program may not be used without the written permission of 00010 * Texas Instruments Incorporated or against the terms and conditions 00011 * stipulated in the agreement under which this program has been supplied, 00012 * and under no circumstances can it be used with non-TI connectivity device. 00013 * 00014 ******************************************************************************/ 00015 00016 #include "server_util.h" 00017 00018 namespace mbed_mqtt { 00019 00020 static uint16_t msg_id = 0xFFFF; 00021 static inline uint16_t assign_new_msg_id() 00022 { 00023 return msg_id += 2; 00024 } 00025 00026 uint16_t mqp_new_id_server(void) 00027 { 00028 return assign_new_msg_id(); 00029 } 00030 00031 static void my_pkt_free(struct mqtt_packet *mqp) 00032 { 00033 my_free((void*) mqp); 00034 } 00035 00036 /*---------------------------------------------------------------------------- 00037 * Try to prevent fragmentation by consistently allocating a fixed memory size 00038 *---------------------------------------------------------------------------- 00039 */ 00040 #ifndef CFG_SR_MAX_MQP_TX_LEN 00041 #define MQP_SERVER_TX_LEN 1024 00042 #else 00043 #define MQP_SERVER_TX_LEN CFG_SR_MAX_MQP_TX_LEN 00044 #endif 00045 00046 /*------------------ Fixed memory configuration ends -----------------------*/ 00047 00048 static struct mqtt_packet *server_mqp_alloc(uint8_t msg_type, uint32_t buf_sz, uint8_t offset) 00049 { 00050 uint32_t mqp_sz = sizeof(struct mqtt_packet); 00051 struct mqtt_packet *mqp = NULL; 00052 00053 buf_sz += offset; 00054 00055 if((mqp_sz + buf_sz) > MQP_SERVER_TX_LEN) { 00056 USR_INFO("S: fatal, buf alloc len > MQP_SERVER_TX_LEN\n\r"); 00057 return NULL; 00058 } 00059 00060 mqp = (mqtt_packet*)my_malloc(MQP_SERVER_TX_LEN); 00061 if(NULL != mqp) { 00062 mqp_init(mqp, offset); 00063 00064 mqp->msg_type = msg_type; 00065 mqp->maxlen = buf_sz; 00066 mqp->buffer = (uint8_t*)mqp + mqp_sz; 00067 00068 mqp->free = my_pkt_free; 00069 00070 } else { 00071 USR_INFO("S: fatal, failed to alloc Server MQP\n\r"); 00072 } 00073 00074 return mqp; 00075 } 00076 00077 struct mqtt_packet *mqp_server_alloc(uint8_t msg_type, uint32_t buf_sz) 00078 { 00079 return server_mqp_alloc(msg_type, buf_sz, MAX_FH_LEN); 00080 } 00081 00082 struct mqtt_packet *mqp_server_copy(const struct mqtt_packet *mqp) 00083 { 00084 struct mqtt_packet *cpy = server_mqp_alloc(mqp->msg_type, 00085 mqp->maxlen, 0); 00086 if(NULL != cpy) { 00087 uint8_t *buffer = cpy->buffer; 00088 00089 /* Copy to overwrite everything in 'cpy' from source 'mqp' */ 00090 buf_wr_nbytes((uint8_t*)cpy, (uint8_t*)mqp, sizeof(struct mqtt_packet)); 00091 00092 cpy->buffer = buffer; /* Restore buffer and copy */ 00093 buf_wr_nbytes(cpy->buffer, mqp->buffer, mqp->maxlen); 00094 } 00095 00096 return cpy; 00097 } 00098 00099 int32_t (*util_dbg_prn)(const char *fmt, ...) = NULL; 00100 bool util_prn_aux = false; 00101 00102 static void (*lockin_mutex)(void*) = NULL; 00103 static void (*unlock_mutex)(void*) = NULL; 00104 static void *mutex_hnd = NULL; 00105 00106 void util_mutex_lockin(void) 00107 { 00108 if(lockin_mutex) 00109 lockin_mutex(mutex_hnd); 00110 00111 return; 00112 } 00113 00114 void util_mutex_unlock(void) 00115 { 00116 if(unlock_mutex) 00117 unlock_mutex(mutex_hnd); 00118 00119 return; 00120 } 00121 00122 void util_params_set(int32_t (*dbg_prn)(const char *fmt, ...), 00123 void *mutex, 00124 void (*mutex_lockin)(void*), 00125 void (*mutex_unlock)(void*)) 00126 { 00127 util_dbg_prn = dbg_prn; 00128 mutex_hnd = mutex; 00129 lockin_mutex = mutex_lockin; 00130 unlock_mutex = mutex_unlock; 00131 00132 return; 00133 } 00134 00135 }//namespace mbed_mqtt {
Generated on Wed Jul 13 2022 09:55:39 by
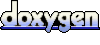