
TI's MQTT Demo with freertos CM4F
Embed:
(wiki syntax)
Show/hide line numbers
cc3100_driver.h
00001 /* 00002 * driver.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 #ifndef DRIVER_INT_H_ 00038 #define DRIVER_INT_H_ 00039 00040 #include "mbed.h" 00041 #include "cc3100_simplelink.h" 00042 00043 #include "cc3100_protocol.h" 00044 #include "cc3100_spi.h" 00045 //#include "cc3100_nonos.h" 00046 #include "cc3100_netapp.h" 00047 //#include "cc3100.h" 00048 00049 #ifdef SL_PLATFORM_MULTI_THREADED 00050 #include "osi.h" 00051 #endif 00052 00053 /*****************************************************************************/ 00054 /* Macro declarations */ 00055 /*****************************************************************************/ 00056 00057 /* 2 LSB of the N2H_SYNC_PATTERN are for sequence number 00058 only in SPI interface 00059 support backward sync pattern */ 00060 #define N2H_SYNC_PATTERN_SEQ_NUM_BITS ((uint32_t)0x00000003) /* Bits 0..1 - use the 2 LBS for seq num */ 00061 #define N2H_SYNC_PATTERN_SEQ_NUM_EXISTS ((uint32_t)0x00000004) /* Bit 2 - sign that sequence number exists in the sync pattern */ 00062 #define N2H_SYNC_PATTERN_MASK ((uint32_t)0xFFFFFFF8) /* Bits 3..31 - constant SYNC PATTERN */ 00063 #define N2H_SYNC_SPI_BUGS_MASK ((uint32_t)0x7FFF7F7F) /* Bits 7,15,31 - ignore the SPI (8,16,32 bites bus) error bits */ 00064 #define BUF_SYNC_SPIM(pBuf) ((*(uint32_t *)(pBuf)) & N2H_SYNC_SPI_BUGS_MASK) 00065 #define N2H_SYNC_SPIM (N2H_SYNC_PATTERN & N2H_SYNC_SPI_BUGS_MASK) 00066 #define N2H_SYNC_SPIM_WITH_SEQ(TxSeqNum) ((N2H_SYNC_SPIM & N2H_SYNC_PATTERN_MASK) | N2H_SYNC_PATTERN_SEQ_NUM_EXISTS | ((TxSeqNum) & (N2H_SYNC_PATTERN_SEQ_NUM_BITS))) 00067 #define MATCH_WOUT_SEQ_NUM(pBuf) ( BUF_SYNC_SPIM(pBuf) == N2H_SYNC_SPIM ) 00068 #define MATCH_WITH_SEQ_NUM(pBuf, TxSeqNum) ( BUF_SYNC_SPIM(pBuf) == (N2H_SYNC_SPIM_WITH_SEQ(TxSeqNum)) ) 00069 #define N2H_SYNC_PATTERN_MATCH(pBuf, TxSeqNum) \ 00070 ( \ 00071 ( (*((uint32_t *)pBuf) & N2H_SYNC_PATTERN_SEQ_NUM_EXISTS) && ( MATCH_WITH_SEQ_NUM(pBuf, TxSeqNum) ) ) || \ 00072 ( !(*((uint32_t *)pBuf) & N2H_SYNC_PATTERN_SEQ_NUM_EXISTS) && ( MATCH_WOUT_SEQ_NUM(pBuf ) ) ) \ 00073 ) 00074 00075 #define OPCODE(_ptr) (((_SlResponseHeader_t *)(_ptr))->GenHeader.Opcode) 00076 #define RSP_PAYLOAD_LEN(_ptr) (((_SlResponseHeader_t *)(_ptr))->GenHeader.Len - _SL_RESP_SPEC_HDR_SIZE) 00077 #define SD(_ptr) (((_SocketAddrResponse_u *)(_ptr))->IpV4.sd) 00078 /* Actual size of Recv/Recvfrom response data */ 00079 #define ACT_DATA_SIZE(_ptr) (((_SocketAddrResponse_u *)(_ptr))->IpV4.statusOrLen) 00080 00081 #define _SL_PROTOCOL_ALIGN_SIZE(msgLen) (((msgLen)+3) & (~3)) 00082 #define _SL_IS_PROTOCOL_ALIGNED_SIZE(msgLen) (!((msgLen) & 3)) 00083 #define _SL_PROTOCOL_CALC_LEN(pCmdCtrl,pCmdExt) ((pCmdExt) ? \ 00084 (_SL_PROTOCOL_ALIGN_SIZE(pCmdCtrl->TxDescLen) + _SL_PROTOCOL_ALIGN_SIZE(pCmdExt->TxPayloadLen)) : \ 00085 (_SL_PROTOCOL_ALIGN_SIZE(pCmdCtrl->TxDescLen))) 00086 00087 00088 namespace mbed_cc3100 { 00089 00090 //class cc3100; 00091 00092 /*****************************************************************************/ 00093 /* Structure/Enum declarations */ 00094 /*****************************************************************************/ 00095 //typedef void(*_SlSpawnEntryFunc_t)(void* pValue); 00096 00097 typedef struct { 00098 _SlOpcode_t Opcode; 00099 _SlArgSize_t TxDescLen; 00100 _SlArgSize_t RxDescLen; 00101 } _SlCmdCtrl_t; 00102 00103 typedef struct { 00104 uint16_t TxPayloadLen; 00105 int16_t RxPayloadLen; 00106 int16_t ActualRxPayloadLen; 00107 uint8_t *pTxPayload; 00108 uint8_t *pRxPayload; 00109 } _SlCmdExt_t; 00110 00111 00112 typedef struct _SlArgsData_t { 00113 uint8_t *pArgs; 00114 uint8_t *pData; 00115 } _SlArgsData_t; 00116 00117 typedef struct { 00118 _SlSyncObj_t SyncObj; 00119 uint8_t *pRespArgs; 00120 uint8_t ActionID; 00121 uint8_t AdditionalData; /* use for socketID and one bit which indicate supprt IPV6 or not (1=support, 0 otherwise) */ 00122 uint8_t NextIndex; 00123 00124 } _SlPoolObj_t; 00125 00126 00127 typedef enum { 00128 SOCKET_0, 00129 SOCKET_1, 00130 SOCKET_2, 00131 SOCKET_3, 00132 SOCKET_4, 00133 SOCKET_5, 00134 SOCKET_6, 00135 SOCKET_7, 00136 MAX_SOCKET_ENUM_IDX, 00137 #ifndef SL_TINY_EXT 00138 ACCEPT_ID = MAX_SOCKET_ENUM_IDX, 00139 CONNECT_ID, 00140 #else 00141 CONNECT_ID = MAX_SOCKET_ENUM_IDX, 00142 #endif 00143 #ifndef SL_TINY_EXT 00144 SELECT_ID, 00145 #endif 00146 GETHOSYBYNAME_ID, 00147 #ifndef SL_TINY_EXT 00148 GETHOSYBYSERVICE_ID, 00149 PING_ID, 00150 #endif 00151 START_STOP_ID, 00152 RECV_ID 00153 } _SlActionID_e; 00154 00155 typedef struct _SlActionLookup_t { 00156 uint8_t ActionID; 00157 uint16_t ActionAsyncOpcode; 00158 _SlSpawnEntryFunc_t AsyncEventHandler; 00159 00160 } _SlActionLookup_t; 00161 00162 00163 typedef struct { 00164 uint8_t TxPoolCnt; 00165 _SlLockObj_t TxLockObj; 00166 _SlSyncObj_t TxSyncObj; 00167 } _SlFlowContCB_t; 00168 00169 typedef enum { 00170 RECV_RESP_CLASS, 00171 CMD_RESP_CLASS, 00172 ASYNC_EVT_CLASS, 00173 DUMMY_MSG_CLASS 00174 } _SlRxMsgClass_e; 00175 00176 typedef struct { 00177 uint8_t *pAsyncBuf; /* place to write pointer to buffer with CmdResp's Header + Arguments */ 00178 uint8_t ActionIndex; 00179 _SlSpawnEntryFunc_t AsyncEvtHandler; /* place to write pointer to AsyncEvent handler (calc-ed by Opcode) */ 00180 _SlRxMsgClass_e RxMsgClass; /* type of Rx message */ 00181 } AsyncExt_t; 00182 00183 typedef struct { 00184 _SlCmdCtrl_t *pCmdCtrl; 00185 uint8_t *pTxRxDescBuff; 00186 _SlCmdExt_t *pCmdExt; 00187 AsyncExt_t AsyncExt; 00188 } _SlFunctionParams_t; 00189 00190 typedef void (*P_INIT_CALLBACK)(uint32_t Status); 00191 00192 typedef struct { 00193 _SlFd_t FD; 00194 _SlLockObj_t GlobalLockObj; 00195 _SlCommandHeader_t TempProtocolHeader; 00196 P_INIT_CALLBACK pInitCallback; 00197 00198 _SlPoolObj_t ObjPool[MAX_CONCURRENT_ACTIONS]; 00199 uint8_t FreePoolIdx; 00200 uint8_t PendingPoolIdx; 00201 uint8_t ActivePoolIdx; 00202 uint32_t ActiveActionsBitmap; 00203 _SlLockObj_t ProtectionLockObj; 00204 00205 _SlSyncObj_t CmdSyncObj; 00206 uint8_t IsCmdRespWaited; 00207 _SlFlowContCB_t FlowContCB; 00208 uint8_t TxSeqNum; 00209 uint8_t RxDoneCnt; 00210 uint8_t SocketNonBlocking; 00211 uint8_t SocketTXFailure; 00212 /* for stack reduction the parameters are globals */ 00213 _SlFunctionParams_t FunctionParams; 00214 00215 uint8_t ActionIndex; 00216 }_SlDriverCb_t; 00217 00218 extern volatile uint8_t RxIrqCnt; 00219 00220 extern _SlDriverCb_t* g_pCB; 00221 typedef uint8_t _SlSd_t; 00222 00223 class cc3100_driver 00224 { 00225 00226 public: 00227 00228 #ifndef SL_PLATFORM_MULTI_THREADED 00229 cc3100_driver(cc3100_spi &spi, cc3100_nonos &nonos, cc3100_netapp &netapp, cc3100_flowcont &flowcont); 00230 #else 00231 cc3100_driver(cc3100_spi &spi, cc3100_netapp &netapp, cc3100_flowcont &flowcont); 00232 #endif 00233 ~cc3100_driver(); 00234 00235 00236 /*****************************************************************************/ 00237 /* Function prototypes */ 00238 /*****************************************************************************/ 00239 typedef _SlDriverCb_t pDriver; 00240 00241 uint8_t _SlDrvProtectAsyncRespSetting(uint8_t *pAsyncRsp, uint8_t ActionID, uint8_t SocketID); 00242 00243 bool _SL_PENDING_RX_MSG(pDriver* pDriverCB); 00244 00245 void _SlDrvDriverCBInit(void); 00246 00247 void _SlDrvDriverCBDeinit(void); 00248 00249 void _SlDrvRxIrqHandler(void *pValue); 00250 00251 _SlReturnVal_t _SlDrvCmdOp(_SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00252 00253 _SlReturnVal_t _SlDrvCmdSend(_SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00254 00255 _SlReturnVal_t _SlDrvDataReadOp(_SlSd_t Sd, _SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00256 00257 _SlReturnVal_t _SlDrvDataWriteOp(_SlSd_t Sd, _SlCmdCtrl_t *pCmdCtrl , void* pTxRxDescBuff , _SlCmdExt_t* pCmdExt); 00258 #ifndef SL_TINY_EXT 00259 int16_t _SlDrvBasicCmd(_SlOpcode_t Opcode); 00260 #endif 00261 uint8_t _SlDrvWaitForPoolObj(uint8_t ActionID, uint8_t SocketID); 00262 00263 void _SlDrvReleasePoolObj(uint8_t pObj); 00264 00265 // void _SlDrvObjInit(void); 00266 00267 _SlReturnVal_t _SlDrvMsgRead(void); 00268 00269 _SlReturnVal_t _SlDrvMsgWrite(_SlCmdCtrl_t *pCmdCtrl,_SlCmdExt_t *pCmdExt, uint8_t *pTxRxDescBuff); 00270 00271 // _SlReturnVal_t _SlDrvMsgWrite(void); 00272 00273 _SlReturnVal_t _SlDrvMsgReadCmdCtx(void); 00274 00275 _SlReturnVal_t _SlDrvMsgReadSpawnCtx_(void *pValue); 00276 00277 void _SlDrvClassifyRxMsg(_SlOpcode_t Opcode ); 00278 00279 _SlReturnVal_t _SlDrvRxHdrRead(uint8_t *pBuf, uint8_t *pAlignSize); 00280 00281 void _SlDrvShiftDWord(uint8_t *pBuf); 00282 00283 void _SlAsyncEventGenericHandler(void); 00284 00285 void _SlDrvObjDeInit(void); 00286 00287 void _SlRemoveFromList(uint8_t* ListIndex, uint8_t ItemIndex); 00288 00289 _SlReturnVal_t _SlFindAndSetActiveObj(_SlOpcode_t Opcode, uint8_t Sd); 00290 00291 uint16_t _SlDrvAlignSize(uint16_t msgLen); 00292 void _SlDrvSyncObjWaitForever(_SlSyncObj_t *pSyncObj); 00293 void _SlDrvSyncObjSignal(_SlSyncObj_t *pSyncObj); 00294 void _SlDrvObjLock(_SlLockObj_t *pLockObj, _SlTime_t Timeout); 00295 void _SlDrvObjLockWaitForever(_SlLockObj_t *pLockObj); 00296 void _SlDrvObjUnLock(_SlLockObj_t *pLockObj); 00297 void _SlDrvProtectionObjLockWaitForever(); 00298 void _SlDrvProtectionObjUnLock(); 00299 void _SlDrvMemZero(void* Addr, uint16_t size); 00300 void _SlDrvResetCmdExt(_SlCmdExt_t* pCmdExt); 00301 00302 00303 private: 00304 00305 cc3100_spi &_spi; 00306 #ifndef SL_PLATFORM_MULTI_THREADED 00307 cc3100_nonos &_nonos; 00308 #endif 00309 cc3100_netapp &_netapp; 00310 cc3100_flowcont &_flowcont; 00311 00312 };//class 00313 00314 00315 00316 }//namespace mbed_cc3100 00317 00318 #endif /* __DRIVER_INT_H__ */ 00319
Generated on Wed Jul 13 2022 09:55:38 by
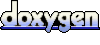