
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
portmacro.h
00001 /* 00002 FreeRTOS V8.2.1 - Copyright (C) 2015 Real Time Engineers Ltd. 00003 All rights reserved 00004 00005 VISIT http://www.FreeRTOS.org TO ENSURE YOU ARE USING THE LATEST VERSION. 00006 00007 This file is part of the FreeRTOS distribution. 00008 00009 FreeRTOS is free software; you can redistribute it and/or modify it under 00010 the terms of the GNU General Public License (version 2) as published by the 00011 Free Software Foundation >>!AND MODIFIED BY!<< the FreeRTOS exception. 00012 00013 *************************************************************************** 00014 >>! NOTE: The modification to the GPL is included to allow you to !<< 00015 >>! distribute a combined work that includes FreeRTOS without being !<< 00016 >>! obliged to provide the source code for proprietary components !<< 00017 >>! outside of the FreeRTOS kernel. !<< 00018 *************************************************************************** 00019 00020 FreeRTOS is distributed in the hope that it will be useful, but WITHOUT ANY 00021 WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS 00022 FOR A PARTICULAR PURPOSE. Full license text is available on the following 00023 link: http://www.freertos.org/a00114.html 00024 00025 *************************************************************************** 00026 * * 00027 * FreeRTOS provides completely free yet professionally developed, * 00028 * robust, strictly quality controlled, supported, and cross * 00029 * platform software that is more than just the market leader, it * 00030 * is the industry's de facto standard. * 00031 * * 00032 * Help yourself get started quickly while simultaneously helping * 00033 * to support the FreeRTOS project by purchasing a FreeRTOS * 00034 * tutorial book, reference manual, or both: * 00035 * http://www.FreeRTOS.org/Documentation * 00036 * * 00037 *************************************************************************** 00038 00039 http://www.FreeRTOS.org/FAQHelp.html - Having a problem? Start by reading 00040 the FAQ page "My application does not run, what could be wrong?". Have you 00041 defined configASSERT()? 00042 00043 http://www.FreeRTOS.org/support - In return for receiving this top quality 00044 embedded software for free we request you assist our global community by 00045 participating in the support forum. 00046 00047 http://www.FreeRTOS.org/training - Investing in training allows your team to 00048 be as productive as possible as early as possible. Now you can receive 00049 FreeRTOS training directly from Richard Barry, CEO of Real Time Engineers 00050 Ltd, and the world's leading authority on the world's leading RTOS. 00051 00052 http://www.FreeRTOS.org/plus - A selection of FreeRTOS ecosystem products, 00053 including FreeRTOS+Trace - an indispensable productivity tool, a DOS 00054 compatible FAT file system, and our tiny thread aware UDP/IP stack. 00055 00056 http://www.FreeRTOS.org/labs - Where new FreeRTOS products go to incubate. 00057 Come and try FreeRTOS+TCP, our new open source TCP/IP stack for FreeRTOS. 00058 00059 http://www.OpenRTOS.com - Real Time Engineers ltd. license FreeRTOS to High 00060 Integrity Systems ltd. to sell under the OpenRTOS brand. Low cost OpenRTOS 00061 licenses offer ticketed support, indemnification and commercial middleware. 00062 00063 http://www.SafeRTOS.com - High Integrity Systems also provide a safety 00064 engineered and independently SIL3 certified version for use in safety and 00065 mission critical applications that require provable dependability. 00066 00067 1 tab == 4 spaces! 00068 */ 00069 00070 00071 #ifndef PORTMACRO_H 00072 #define PORTMACRO_H 00073 00074 #ifdef __cplusplus 00075 extern "C" { 00076 #endif 00077 00078 /*----------------------------------------------------------- 00079 * Port specific definitions. 00080 * 00081 * The settings in this file configure FreeRTOS correctly for the 00082 * given hardware and compiler. 00083 * 00084 * These settings should not be altered. 00085 *----------------------------------------------------------- 00086 */ 00087 00088 /* Type definitions. */ 00089 #define portCHAR char 00090 #define portFLOAT float 00091 #define portDOUBLE double 00092 #define portLONG long 00093 #define portSHORT short 00094 #define portSTACK_TYPE uint32_t 00095 #define portBASE_TYPE long 00096 00097 typedef portSTACK_TYPE StackType_t; 00098 typedef long BaseType_t; 00099 typedef unsigned long UBaseType_t; 00100 00101 #if( configUSE_16_BIT_TICKS == 1 ) 00102 typedef uint16_t TickType_t; 00103 #define portMAX_DELAY ( TickType_t ) 0xffff 00104 #else 00105 typedef uint32_t TickType_t; 00106 #define portMAX_DELAY ( TickType_t ) 0xffffffffUL 00107 00108 /* 32-bit tick type on a 32-bit architecture, so reads of the tick count do 00109 not need to be guarded with a critical section. */ 00110 #define portTICK_TYPE_IS_ATOMIC 1 00111 #endif 00112 /*-----------------------------------------------------------*/ 00113 00114 /* Architecture specifics. */ 00115 #define portSTACK_GROWTH ( -1 ) 00116 #define portTICK_PERIOD_MS ( ( TickType_t ) 1000 / configTICK_RATE_HZ ) 00117 #define portBYTE_ALIGNMENT 8 00118 /*-----------------------------------------------------------*/ 00119 00120 /* Scheduler utilities. */ 00121 extern void vPortYield( void ); 00122 #define portNVIC_INT_CTRL_REG ( * ( ( volatile uint32_t * ) 0xe000ed04 ) ) 00123 #define portNVIC_PENDSVSET_BIT ( 1UL << 28UL ) 00124 #define portYIELD() vPortYield() 00125 #define portEND_SWITCHING_ISR( xSwitchRequired ) if( xSwitchRequired ) portNVIC_INT_CTRL_REG = portNVIC_PENDSVSET_BIT 00126 #define portYIELD_FROM_ISR( x ) portEND_SWITCHING_ISR( x ) 00127 /*-----------------------------------------------------------*/ 00128 00129 /* Critical section management. */ 00130 extern uint32_t ulPortSetInterruptMask( void ); 00131 extern void vPortClearInterruptMask( uint32_t ulNewMask ); 00132 extern void vPortEnterCritical( void ); 00133 extern void vPortExitCritical( void ); 00134 00135 #define portDISABLE_INTERRUPTS() ulPortSetInterruptMask() 00136 #define portENABLE_INTERRUPTS() vPortClearInterruptMask( 0 ) 00137 #define portENTER_CRITICAL() vPortEnterCritical() 00138 #define portEXIT_CRITICAL() vPortExitCritical() 00139 #define portSET_INTERRUPT_MASK_FROM_ISR() ulPortSetInterruptMask() 00140 #define portCLEAR_INTERRUPT_MASK_FROM_ISR(x) vPortClearInterruptMask(x) 00141 /*-----------------------------------------------------------*/ 00142 00143 /* Tickless idle/low power functionality. */ 00144 #ifndef portSUPPRESS_TICKS_AND_SLEEP 00145 extern void vPortSuppressTicksAndSleep( TickType_t xExpectedIdleTime ); 00146 #define portSUPPRESS_TICKS_AND_SLEEP( xExpectedIdleTime ) vPortSuppressTicksAndSleep( xExpectedIdleTime ) 00147 #endif 00148 /*-----------------------------------------------------------*/ 00149 00150 /* Port specific optimisations. */ 00151 //#ifndef configUSE_PORT_OPTIMISED_TASK_SELECTION 00152 // #define configUSE_PORT_OPTIMISED_TASK_SELECTION 1 00153 //#endif 00154 00155 #if configUSE_PORT_OPTIMISED_TASK_SELECTION == 1 00156 00157 /* Check the configuration. */ 00158 #if( configMAX_PRIORITIES > 32 ) 00159 #error configUSE_PORT_OPTIMISED_TASK_SELECTION can only be set to 1 when configMAX_PRIORITIES is less than or equal to 32. It is very rare that a system requires more than 10 to 15 difference priorities as tasks that share a priority will time slice. 00160 #endif 00161 00162 /* Store/clear the ready priorities in a bit map. */ 00163 #define portRECORD_READY_PRIORITY( uxPriority, uxReadyPriorities ) ( uxReadyPriorities ) |= ( 1UL << ( uxPriority ) ) 00164 #define portRESET_READY_PRIORITY( uxPriority, uxReadyPriorities ) ( uxReadyPriorities ) &= ~( 1UL << ( uxPriority ) ) 00165 00166 /*-----------------------------------------------------------*/ 00167 00168 #define portGET_HIGHEST_PRIORITY( uxTopPriority, uxReadyPriorities ) uxTopPriority = ( 31 - __clz( ( uxReadyPriorities ) ) ) 00169 00170 #endif /* taskRECORD_READY_PRIORITY */ 00171 /*-----------------------------------------------------------*/ 00172 00173 /* Task function macros as described on the FreeRTOS.org WEB site. These are 00174 not necessary for to use this port. They are defined so the common demo files 00175 (which build with all the ports) will build. */ 00176 #define portTASK_FUNCTION_PROTO( vFunction, pvParameters ) void vFunction( void *pvParameters ) 00177 #define portTASK_FUNCTION( vFunction, pvParameters ) void vFunction( void *pvParameters ) 00178 /*-----------------------------------------------------------*/ 00179 00180 #ifdef configASSERT 00181 void vPortValidateInterruptPriority( void ); 00182 #define portASSERT_IF_INTERRUPT_PRIORITY_INVALID() vPortValidateInterruptPriority() 00183 #endif 00184 00185 /* portNOP() is not required by this port. */ 00186 #define portNOP() 00187 00188 #ifdef __cplusplus 00189 } 00190 #endif 00191 00192 #endif /* PORTMACRO_H */ 00193 00194
Generated on Tue Jul 12 2022 18:55:10 by
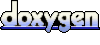