
Update revision to use TI's mqtt and Freertos.
Dependencies: mbed client server
Fork of cc3100_Test_mqtt_CM3 by
cc3100_trace.h
00001 /* 00002 * trace.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2014 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 #ifndef SIMPLELINK_TRACE_H_ 00037 #define SIMPLELINK_TRACE_H_ 00038 00039 00040 #include "cc3100_simplelink.h" 00041 00042 /*****************************************************************************/ 00043 /* Macro declarations */ 00044 /*****************************************************************************/ 00045 00046 /* This macro is for use by other macros to form a fully valid C statement. */ 00047 #define st(x) do { x } while (__LINE__ == -1) 00048 00049 #define SL_SYNC_SCAN_THRESHOLD (( uint32_t )2000) 00050 00051 #define _SlDrvAssert(line ) { while(1); } 00052 00053 #define _SL_ASSERT(expr) { if(!(expr)){_SlDrvAssert(__LINE__); } } 00054 #define _SL_ERROR(expr, error) { if(!(expr)){return (error); } } 00055 00056 #define SL_HANDLING_ASSERT 2 00057 #define SL_HANDLING_ERROR 1 00058 #define SL_HANDLING_NONE 0 00059 00060 #ifndef SL_TINY_EXT 00061 #define SL_SELF_COND_HANDLING SL_HANDLING_ASSERT 00062 #define SL_PROTOCOL_HANDLING SL_HANDLING_ASSERT 00063 #define SL_DRV_RET_CODE_HANDLING SL_HANDLING_ASSERT 00064 #define SL_NWP_IF_HANDLING SL_HANDLING_ASSERT 00065 #define SL_OSI_RET_OK_HANDLING SL_HANDLING_ASSERT 00066 #define SL_MALLOC_OK_HANDLING SL_HANDLING_ASSERT 00067 #define SL_USER_ARGS_HANDLING SL_HANDLING_ASSERT 00068 #else 00069 #define SL_SELF_COND_HANDLING SL_HANDLING_NONE 00070 #define SL_PROTOCOL_HANDLING SL_HANDLING_NONE 00071 #define SL_DRV_RET_CODE_HANDLING SL_HANDLING_NONE 00072 #define SL_NWP_IF_HANDLING SL_HANDLING_NONE 00073 #define SL_OSI_RET_OK_HANDLING SL_HANDLING_NONE 00074 #define SL_MALLOC_OK_HANDLING SL_HANDLING_NONE 00075 #define SL_USER_ARGS_HANDLING SL_HANDLING_NONE 00076 #endif 00077 00078 #if (SL_DRV_RET_CODE_HANDLING == SL_HANDLING_ASSERT) 00079 #define VERIFY_RET_OK(Func) {_SlReturnVal_t _RetVal = (Func); _SL_ASSERT((_SlReturnVal_t)SL_OS_RET_CODE_OK == _RetVal)} 00080 #elif (SL_DRV_RET_CODE_HANDLING == SL_HANDLING_ERROR) 00081 #define VERIFY_RET_OK(Func) {_SlReturnVal_t _RetVal = (Func); if (SL_OS_RET_CODE_OK != _RetVal) return _RetVal;} 00082 #else 00083 #define VERIFY_RET_OK(Func) (Func); 00084 #endif 00085 00086 #if (SL_PROTOCOL_HANDLING == SL_HANDLING_ASSERT) 00087 #define VERIFY_PROTOCOL(expr) _SL_ASSERT(expr) 00088 #elif (SL_PROTOCOL_HANDLING == SL_HANDLING_ERROR) 00089 #define VERIFY_PROTOCOL(expr) _SL_ERROR(expr, SL_RET_CODE_PROTOCOL_ERROR) 00090 #else 00091 #define VERIFY_PROTOCOL(expr) 00092 #endif 00093 00094 #if (defined(PROTECT_SOCKET_ASYNC_RESP) && (SL_SELF_COND_HANDLING == SL_HANDLING_ASSERT)) 00095 #define VERIFY_SOCKET_CB(expr) _SL_ASSERT(expr) 00096 #elif (defined(PROTECT_SOCKET_ASYNC_RESP) && (SL_SELF_COND_HANDLING == SL_HANDLING_ERROR)) 00097 #define VERIFY_SOCKET_CB(expr) _SL_ERROR(expr, SL_RET_CODE_SELF_ERROR) 00098 #else 00099 #define VERIFY_SOCKET_CB(expr) 00100 #endif 00101 00102 #if (SL_NWP_IF_HANDLING == SL_HANDLING_ASSERT) 00103 //#define NWP_IF_WRITE_CHECK(fd,pBuff,len) { int16_t RetSize, ExpSize = (len); RetSize = sl_IfWrite((fd),(pBuff),ExpSize); _SL_ASSERT(ExpSize == RetSize)} 00104 //#define NWP_IF_READ_CHECK(fd,pBuff,len) { int16_t RetSize, ExpSize = (len); RetSize = sl_IfRead((fd),(pBuff),ExpSize); _SL_ASSERT(ExpSize == RetSize)} 00105 #elif (SL_NWP_IF_HANDLING == SL_HANDLING_ERROR) 00106 //#define NWP_IF_WRITE_CHECK(fd,pBuff,len) { _SL_ERROR((len == sl_IfWrite((fd),(pBuff),(len))), SL_RET_CODE_NWP_IF_ERROR);} 00107 //#define NWP_IF_READ_CHECK(fd,pBuff,len) { _SL_ERROR((len == sl_IfRead((fd),(pBuff),(len))), SL_RET_CODE_NWP_IF_ERROR);} 00108 #else 00109 //#define NWP_IF_WRITE_CHECK(fd,pBuff,len) { sl_IfWrite((fd),(pBuff),(len));} 00110 //#define NWP_IF_READ_CHECK(fd,pBuff,len) { sl_IfRead((fd),(pBuff),(len));} 00111 #endif 00112 00113 #if (SL_OSI_RET_OK_HANDLING == SL_HANDLING_ASSERT) 00114 #define OSI_RET_OK_CHECK(Func) {_SlReturnVal_t _RetVal = (Func); _SL_ASSERT((_SlReturnVal_t)SL_OS_RET_CODE_OK == _RetVal)} 00115 #elif (SL_OSI_RET_OK_HANDLING == SL_HANDLING_ERROR) 00116 #define OSI_RET_OK_CHECK(Func) {_SlReturnVal_t _RetVal = (Func); if (SL_OS_RET_CODE_OK != _RetVal) return _RetVal;} 00117 #else 00118 #define OSI_RET_OK_CHECK(Func) (Func); 00119 #endif 00120 00121 #if (SL_MALLOC_OK_HANDLING == SL_HANDLING_ASSERT) 00122 #define MALLOC_OK_CHECK(Ptr) _SL_ASSERT(NULL != Ptr) 00123 #elif (SL_MALLOC_OK_HANDLING == SL_HANDLING_ERROR) 00124 #define MALLOC_OK_CHECK(Ptr) _SL_ERROR((NULL != Ptr), SL_RET_CODE_MALLOC_ERROR) 00125 #else 00126 #define MALLOC_OK_CHECK(Ptr) 00127 #endif 00128 00129 #ifdef SL_INC_ARG_CHECK 00130 00131 #if (SL_USER_ARGS_HANDLING == SL_HANDLING_ASSERT) 00132 #define ARG_CHECK_PTR(Ptr) _SL_ASSERT(NULL != Ptr) 00133 #elif (SL_USER_ARGS_HANDLING == SL_HANDLING_ERROR) 00134 #define ARG_CHECK_PTR(Ptr) _SL_ERROR((NULL != Ptr), SL_RET_CODE_INVALID_INPUT) 00135 #else 00136 #define ARG_CHECK_PTR(Ptr) 00137 #endif 00138 00139 #else 00140 #define ARG_CHECK_PTR(Ptr) 00141 #endif 00142 00143 //#define SL_DBG_TRACE_ENABLE 00144 #ifdef SL_DBG_TRACE_ENABLE 00145 #define SL_TRACE0(level,msg_id,str) printf(str) 00146 #define SL_TRACE1(level,msg_id,str,p1) printf(str,(p1)) 00147 #define SL_TRACE2(level,msg_id,str,p1,p2) printf(str,(p1),(p2)) 00148 #define SL_TRACE3(level,msg_id,str,p1,p2,p3) printf(str,(p1),(p2),(p3)) 00149 #define SL_TRACE4(level,msg_id,str,p1,p2,p3,p4) printf(str,(p1),(p2),(p3),(p4)) 00150 #define SL_ERROR_TRACE(msg_id,str) printf(str) 00151 #define SL_ERROR_TRACE1(msg_id,str,p1) printf(str,(p1)) 00152 #define SL_ERROR_TRACE2(msg_id,str,p1,p2) printf(str,(p1),(p2)) 00153 #define SL_ERROR_TRACE3(msg_id,str,p1,p2,p3) printf(str,(p1),(p2),(p3)) 00154 #define SL_ERROR_TRACE4(msg_id,str,p1,p2,p3,p4) printf(str,(p1),(p2),(p3),(p4)) 00155 #define SL_TRACE_FLUSH() 00156 #else 00157 #define SL_TRACE0(level,msg_id,str) 00158 #define SL_TRACE1(level,msg_id,str,p1) 00159 #define SL_TRACE2(level,msg_id,str,p1,p2) 00160 #define SL_TRACE3(level,msg_id,str,p1,p2,p3) 00161 #define SL_TRACE4(level,msg_id,str,p1,p2,p3,p4) 00162 #define SL_ERROR_TRACE(msg_id,str) 00163 #define SL_ERROR_TRACE1(msg_id,str,p1) 00164 #define SL_ERROR_TRACE2(msg_id,str,p1,p2) 00165 #define SL_ERROR_TRACE3(msg_id,str,p1,p2,p3) 00166 #define SL_ERROR_TRACE4(msg_id,str,p1,p2,p3,p4) 00167 #define SL_TRACE_FLUSH() 00168 #endif 00169 00170 //#define SL_DBG_CNT_ENABLE 00171 #ifdef SL_DBG_CNT_ENABLE 00172 #define _SL_DBG_CNT_INC(Cnt) g_DbgCnt. ## Cnt++ 00173 #define _SL_DBG_SYNC_LOG(index,value) {if(index < SL_DBG_SYNC_LOG_SIZE){*(uint32_t *)&g_DbgCnt.SyncLog[index] = *(uint32_t *)(value);}} 00174 00175 #else 00176 #define _SL_DBG_CNT_INC(Cnt) 00177 #define _SL_DBG_SYNC_LOG(index,value) 00178 #endif 00179 00180 #define SL_DBG_LEVEL_1 1 00181 #define SL_DBG_LEVEL_2 2 00182 #define SL_DBG_LEVEL_3 4 00183 #define SL_DBG_LEVEL_MASK (SL_DBG_LEVEL_2|SL_DBG_LEVEL_3) 00184 00185 #define SL_INCLUDE_DBG_FUNC(Name) ((Name ## _DBG_LEVEL) & SL_DBG_LEVEL_MASK) 00186 00187 #define _SlDrvPrintStat_DBG_LEVEL SL_DBG_LEVEL_3 00188 #define _SlDrvOtherFunc_DBG_LEVEL SL_DBG_LEVEL_1 00189 00190 00191 #endif /*__SIMPLELINK_TRACE_H__*/ 00192 00193
Generated on Tue Jul 12 2022 18:55:10 by
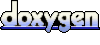