CC3000HostDriver for device TI CC3000 some changes were made due to mbed compiler and the use of void*
Embed:
(wiki syntax)
Show/hide line numbers
socket.h
00001 /***************************************************************************** 00002 * 00003 * socket.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __SOCKET_H__ 00036 #define __SOCKET_H__ 00037 00038 00039 //***************************************************************************** 00040 // 00041 //! \addtogroup socket_api 00042 //! @{ 00043 // 00044 //***************************************************************************** 00045 00046 00047 //***************************************************************************** 00048 // 00049 // If building with a C++ compiler, make all of the definitions in this header 00050 // have a C binding. 00051 // 00052 //***************************************************************************** 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 #define HOSTNAME_MAX_LENGTH (230) // 230 bytes + header shouldn't exceed 8 bit value 00058 00059 //--------- Address Families -------- 00060 00061 #define AF_INET 2 00062 #define AF_INET6 23 00063 00064 //------------ Socket Types ------------ 00065 00066 #define SOCK_STREAM 1 00067 #define SOCK_DGRAM 2 00068 #define SOCK_RAW 3 // Raw sockets allow new IPv4 protocols to be implemented in user space. A raw socket receives or sends the raw datagram not including link level headers 00069 #define SOCK_RDM 4 00070 #define SOCK_SEQPACKET 5 00071 00072 //----------- Socket Protocol ---------- 00073 00074 #define IPPROTO_IP 0 // dummy for IP 00075 #define IPPROTO_ICMP 1 // control message protocol 00076 #define IPPROTO_IPV4 IPPROTO_IP // IP inside IP 00077 #define IPPROTO_TCP 6 // tcp 00078 #define IPPROTO_UDP 17 // user datagram protocol 00079 #define IPPROTO_IPV6 41 // IPv6 in IPv6 00080 #define IPPROTO_NONE 59 // No next header 00081 #define IPPROTO_RAW 255 // raw IP packet 00082 #define IPPROTO_MAX 256 00083 00084 //----------- Socket retunr codes ----------- 00085 00086 #define SOC_ERROR (-1) // error 00087 #define SOC_IN_PROGRESS (-2) // socket in progress 00088 00089 //----------- Socket Options ----------- 00090 #define SOL_SOCKET 0xffff // socket level 00091 #define SOCKOPT_RECV_NONBLOCK 0 // recv non block mode, set SOCK_ON or SOCK_OFF (default block mode) 00092 #define SOCKOPT_RECV_TIMEOUT 1 // optname to configure recv and recvfromtimeout 00093 #define SOCKOPT_ACCEPT_NONBLOCK 2 // accept non block mode, set SOCK_ON or SOCK_OFF (default block mode) 00094 #define SOCK_ON 0 // socket non-blocking mode is enabled 00095 #define SOCK_OFF 1 // socket blocking mode is enabled 00096 00097 #define TCP_NODELAY 0x0001 00098 #define TCP_BSDURGENT 0x7000 00099 00100 #define MAX_PACKET_SIZE 1500 00101 #define MAX_LISTEN_QUEUE 4 00102 00103 #define IOCTL_SOCKET_EVENTMASK 00104 00105 #define ENOBUFS 55 // No buffer space available 00106 00107 #define __FD_SETSIZE 32 00108 00109 #define ASIC_ADDR_LEN 8 00110 00111 #define NO_QUERY_RECIVED -3 00112 00113 00114 typedef struct _in_addr_t 00115 { 00116 unsigned long s_addr; // load with inet_aton() 00117 } in_addr; 00118 00119 typedef struct _sockaddr_t 00120 { 00121 unsigned short int sa_family; 00122 unsigned char sa_data[14]; 00123 } sockaddr; 00124 00125 typedef struct _sockaddr_in_t 00126 { 00127 short sin_family; // e.g. AF_INET 00128 unsigned short sin_port; // e.g. htons(3490) 00129 in_addr sin_addr; // see struct in_addr, below 00130 char sin_zero[8]; // zero this if you want to 00131 } sockaddr_in; 00132 00133 typedef unsigned long socklen_t; 00134 00135 // The fd_set member is required to be an array of longs. 00136 typedef long int __fd_mask; 00137 00138 // It's easier to assume 8-bit bytes than to get CHAR_BIT. 00139 #define __NFDBITS (8 * sizeof (__fd_mask)) 00140 #define __FDELT(d) ((d) / __NFDBITS) 00141 #define __FDMASK(d) ((__fd_mask) 1 << ((d) % __NFDBITS)) 00142 00143 // fd_set for select and pselect. 00144 typedef struct 00145 { 00146 __fd_mask fds_bits[__FD_SETSIZE / __NFDBITS]; 00147 #define __FDS_BITS(set) ((set)->fds_bits) 00148 } fd_set; 00149 00150 // We don't use `memset' because this would require a prototype and 00151 // the array isn't too big. 00152 #define __FD_ZERO(set) \ 00153 do { \ 00154 unsigned int __i; \ 00155 fd_set *__arr = (set); \ 00156 for (__i = 0; __i < sizeof (fd_set) / sizeof (__fd_mask); ++__i) \ 00157 __FDS_BITS (__arr)[__i] = 0; \ 00158 } while (0) 00159 #define __FD_SET(d, set) (__FDS_BITS (set)[__FDELT (d)] |= __FDMASK (d)) 00160 #define __FD_CLR(d, set) (__FDS_BITS (set)[__FDELT (d)] &= ~__FDMASK (d)) 00161 #define __FD_ISSET(d, set) (__FDS_BITS (set)[__FDELT (d)] & __FDMASK (d)) 00162 00163 // Access macros for 'fd_set'. 00164 #define FD_SET(fd, fdsetp) __FD_SET (fd, fdsetp) 00165 #define FD_CLR(fd, fdsetp) __FD_CLR (fd, fdsetp) 00166 #define FD_ISSET(fd, fdsetp) __FD_ISSET (fd, fdsetp) 00167 #define FD_ZERO(fdsetp) __FD_ZERO (fdsetp) 00168 00169 //Use in case of Big Endian only 00170 00171 #define htonl(A) ((((unsigned long)(A) & 0xff000000) >> 24) | \ 00172 (((unsigned long)(A) & 0x00ff0000) >> 8) | \ 00173 (((unsigned long)(A) & 0x0000ff00) << 8) | \ 00174 (((unsigned long)(A) & 0x000000ff) << 24)) 00175 00176 #define ntohl htonl 00177 00178 //Use in case of Big Endian only 00179 #define htons(A) ((((unsigned long)(A) & 0xff00) >> 8) | \ 00180 (((unsigned long)(A) & 0x00ff) << 8)) 00181 00182 00183 #define ntohs htons 00184 00185 // mDNS port - 5353 mDNS multicast address - 224.0.0.251 00186 #define SET_mDNS_ADD(sockaddr) sockaddr.sa_data[0] = 0x14; \ 00187 sockaddr.sa_data[1] = 0xe9; \ 00188 sockaddr.sa_data[2] = 0xe0; \ 00189 sockaddr.sa_data[3] = 0x0; \ 00190 sockaddr.sa_data[4] = 0x0; \ 00191 sockaddr.sa_data[5] = 0xfb; 00192 00193 00194 //***************************************************************************** 00195 // 00196 // Prototypes for the APIs. 00197 // 00198 //***************************************************************************** 00199 00200 //***************************************************************************** 00201 // 00202 //! socket 00203 //! 00204 //! @param domain selects the protocol family which will be used for 00205 //! communication. On this version only AF_INET is supported 00206 //! @param type specifies the communication semantics. On this version 00207 //! only SOCK_STREAM, SOCK_DGRAM, SOCK_RAW are supported 00208 //! @param protocol specifies a particular protocol to be used with the 00209 //! socket IPPROTO_TCP, IPPROTO_UDP or IPPROTO_RAW are 00210 //! supported. 00211 //! 00212 //! @return On success, socket handle that is used for consequent socket 00213 //! operations. On error, -1 is returned. 00214 //! 00215 //! @brief create an endpoint for communication 00216 //! The socket function creates a socket that is bound to a specific 00217 //! transport service provider. This function is called by the 00218 //! application layer to obtain a socket handle. 00219 // 00220 //***************************************************************************** 00221 extern int socket(long domain, long type, long protocol); 00222 00223 //***************************************************************************** 00224 // 00225 //! closesocket 00226 //! 00227 //! @param sd socket handle. 00228 //! 00229 //! @return On success, zero is returned. On error, -1 is returned. 00230 //! 00231 //! @brief The socket function closes a created socket. 00232 // 00233 //***************************************************************************** 00234 extern long closesocket(long sd); 00235 00236 //***************************************************************************** 00237 // 00238 //! accept 00239 //! 00240 //! @param[in] sd socket descriptor (handle) 00241 //! @param[out] addr the argument addr is a pointer to a sockaddr structure 00242 //! This structure is filled in with the address of the 00243 //! peer socket, as known to the communications layer. 00244 //! determined. The exact format of the address returned 00245 //! addr is by the socket's address sockaddr. 00246 //! On this version only AF_INET is supported. 00247 //! This argument returns in network order. 00248 //! @param[out] addrlen the addrlen argument is a value-result argument: 00249 //! it should initially contain the size of the structure 00250 //! pointed to by addr. 00251 //! 00252 //! @return For socket in blocking mode: 00253 //! On success, socket handle. on failure negative 00254 //! For socket in non-blocking mode: 00255 //! - On connection establishment, socket handle 00256 //! - On connection pending, SOC_IN_PROGRESS (-2) 00257 //! - On failure, SOC_ERROR (-1) 00258 //! 00259 //! @brief accept a connection on a socket: 00260 //! This function is used with connection-based socket types 00261 //! (SOCK_STREAM). It extracts the first connection request on the 00262 //! queue of pending connections, creates a new connected socket, and 00263 //! returns a new file descriptor referring to that socket. 00264 //! The newly created socket is not in the listening state. 00265 //! The original socket sd is unaffected by this call. 00266 //! The argument sd is a socket that has been created with socket(), 00267 //! bound to a local address with bind(), and is listening for 00268 //! connections after a listen(). The argument addr is a pointer 00269 //! to a sockaddr structure. This structure is filled in with the 00270 //! address of the peer socket, as known to the communications layer. 00271 //! The exact format of the address returned addr is determined by the 00272 //! socket's address family. The addrlen argument is a value-result 00273 //! argument: it should initially contain the size of the structure 00274 //! pointed to by addr, on return it will contain the actual 00275 //! length (in bytes) of the address returned. 00276 //! 00277 //! @sa socket ; bind ; listen 00278 // 00279 //***************************************************************************** 00280 extern long accept(long sd, sockaddr *addr, socklen_t *addrlen); 00281 00282 //***************************************************************************** 00283 // 00284 //! bind 00285 //! 00286 //! @param[in] sd socket descriptor (handle) 00287 //! @param[out] addr specifies the destination address. On this version 00288 //! only AF_INET is supported. 00289 //! @param[out] addrlen contains the size of the structure pointed to by addr. 00290 //! 00291 //! @return On success, zero is returned. On error, -1 is returned. 00292 //! 00293 //! @brief assign a name to a socket 00294 //! This function gives the socket the local address addr. 00295 //! addr is addrlen bytes long. Traditionally, this is called when a 00296 //! socket is created with socket, it exists in a name space (address 00297 //! family) but has no name assigned. 00298 //! It is necessary to assign a local address before a SOCK_STREAM 00299 //! socket may receive connections. 00300 //! 00301 //! @sa socket ; accept ; listen 00302 // 00303 //***************************************************************************** 00304 extern long bind(long sd, const sockaddr *addr, long addrlen); 00305 00306 //***************************************************************************** 00307 // 00308 //! listen 00309 //! 00310 //! @param[in] sd socket descriptor (handle) 00311 //! @param[in] backlog specifies the listen queue depth. On this version 00312 //! backlog is not supported. 00313 //! @return On success, zero is returned. On error, -1 is returned. 00314 //! 00315 //! @brief listen for connections on a socket 00316 //! The willingness to accept incoming connections and a queue 00317 //! limit for incoming connections are specified with listen(), 00318 //! and then the connections are accepted with accept. 00319 //! The listen() call applies only to sockets of type SOCK_STREAM 00320 //! The backlog parameter defines the maximum length the queue of 00321 //! pending connections may grow to. 00322 //! 00323 //! @sa socket ; accept ; bind 00324 //! 00325 //! @note On this version, backlog is not supported 00326 // 00327 //***************************************************************************** 00328 extern long listen(long sd, long backlog); 00329 00330 //***************************************************************************** 00331 // 00332 //! gethostbyname 00333 //! 00334 //! @param[in] hostname host name 00335 //! @param[in] usNameLen name length 00336 //! @param[out] out_ip_addr This parameter is filled in with host IP address. 00337 //! In case that host name is not resolved, 00338 //! out_ip_addr is zero. 00339 //! @return On success, positive is returned. On error, negative is returned 00340 //! 00341 //! @brief Get host IP by name. Obtain the IP Address of machine on network, 00342 //! by its name. 00343 //! 00344 //! @note On this version, only blocking mode is supported. Also note that 00345 //! the function requires DNS server to be configured prior to its usage. 00346 // 00347 //***************************************************************************** 00348 #ifndef CC3000_TINY_DRIVER 00349 extern int gethostbyname(char * hostname, unsigned short usNameLen, unsigned long* out_ip_addr); 00350 #endif 00351 00352 00353 //***************************************************************************** 00354 // 00355 //! connect 00356 //! 00357 //! @param[in] sd socket descriptor (handle) 00358 //! @param[in] addr specifies the destination addr. On this version 00359 //! only AF_INET is supported. 00360 //! @param[out] addrlen contains the size of the structure pointed to by addr 00361 //! @return On success, zero is returned. On error, -1 is returned 00362 //! 00363 //! @brief initiate a connection on a socket 00364 //! Function connects the socket referred to by the socket descriptor 00365 //! sd, to the address specified by addr. The addrlen argument 00366 //! specifies the size of addr. The format of the address in addr is 00367 //! determined by the address space of the socket. If it is of type 00368 //! SOCK_DGRAM, this call specifies the peer with which the socket is 00369 //! to be associated; this address is that to which datagrams are to be 00370 //! sent, and the only address from which datagrams are to be received. 00371 //! If the socket is of type SOCK_STREAM, this call attempts to make a 00372 //! connection to another socket. The other socket is specified by 00373 //! address, which is an address in the communications space of the 00374 //! socket. Note that the function implements only blocking behavior 00375 //! thus the caller will be waiting either for the connection 00376 //! establishment or for the connection establishment failure. 00377 //! 00378 //! @sa socket 00379 // 00380 //***************************************************************************** 00381 extern long connect(long sd, const sockaddr *addr, long addrlen); 00382 00383 //***************************************************************************** 00384 // 00385 //! select 00386 //! 00387 //! @param[in] nfds the highest-numbered file descriptor in any of the 00388 //! three sets, plus 1. 00389 //! @param[out] writesds socket descriptors list for write monitoring 00390 //! @param[out] readsds socket descriptors list for read monitoring 00391 //! @param[out] exceptsds socket descriptors list for exception monitoring 00392 //! @param[in] timeout is an upper bound on the amount of time elapsed 00393 //! before select() returns. Null means infinity 00394 //! timeout. The minimum timeout is 5 milliseconds, 00395 //! less than 5 milliseconds will be set 00396 //! automatically to 5 milliseconds. 00397 //! @return On success, select() returns the number of file descriptors 00398 //! contained in the three returned descriptor sets (that is, the 00399 //! total number of bits that are set in readfds, writefds, 00400 //! exceptfds) which may be zero if the timeout expires before 00401 //! anything interesting happens. 00402 //! On error, -1 is returned. 00403 //! *readsds - return the sockets on which Read request will 00404 //! return without delay with valid data. 00405 //! *writesds - return the sockets on which Write request 00406 //! will return without delay. 00407 //! *exceptsds - return the sockets which closed recently. 00408 //! 00409 //! @brief Monitor socket activity 00410 //! Select allow a program to monitor multiple file descriptors, 00411 //! waiting until one or more of the file descriptors become 00412 //! "ready" for some class of I/O operation 00413 //! 00414 //! @Note If the timeout value set to less than 5ms it will automatically set 00415 //! to 5ms to prevent overload of the system 00416 //! 00417 //! @sa socket 00418 // 00419 //***************************************************************************** 00420 extern int select(long nfds, fd_set *readsds, fd_set *writesds, 00421 fd_set *exceptsds, struct timeval *timeout); 00422 00423 //***************************************************************************** 00424 // 00425 //! setsockopt 00426 //! 00427 //! @param[in] sd socket handle 00428 //! @param[in] level defines the protocol level for this option 00429 //! @param[in] optname defines the option name to Interrogate 00430 //! @param[in] optval specifies a value for the option 00431 //! @param[in] optlen specifies the length of the option value 00432 //! @return On success, zero is returned. On error, -1 is returned 00433 //! 00434 //! @brief set socket options 00435 //! This function manipulate the options associated with a socket. 00436 //! Options may exist at multiple protocol levels; they are always 00437 //! present at the uppermost socket level. 00438 //! When manipulating socket options the level at which the option 00439 //! resides and the name of the option must be specified. 00440 //! To manipulate options at the socket level, level is specified as 00441 //! SOL_SOCKET. To manipulate options at any other level the protocol 00442 //! number of the appropriate protocol controlling the option is 00443 //! supplied. For example, to indicate that an option is to be 00444 //! interpreted by the TCP protocol, level should be set to the 00445 //! protocol number of TCP; 00446 //! The parameters optval and optlen are used to access optval - 00447 //! use for setsockopt(). For getsockopt() they identify a buffer 00448 //! in which the value for the requested option(s) are to 00449 //! be returned. For getsockopt(), optlen is a value-result 00450 //! parameter, initially containing the size of the buffer 00451 //! pointed to by option_value, and modified on return to 00452 //! indicate the actual size of the value returned. If no option 00453 //! value is to be supplied or returned, option_value may be NULL. 00454 //! 00455 //! @Note On this version the following two socket options are enabled: 00456 //! The only protocol level supported in this version 00457 //! is SOL_SOCKET (level). 00458 //! 1. SOCKOPT_RECV_TIMEOUT (optname) 00459 //! SOCKOPT_RECV_TIMEOUT configures recv and recvfrom timeout 00460 //! in milliseconds. 00461 //! In that case optval should be pointer to unsigned long. 00462 //! 2. SOCKOPT_NONBLOCK (optname). sets the socket non-blocking mode on 00463 //! or off. 00464 //! In that case optval should be SOCK_ON or SOCK_OFF (optval). 00465 //! 00466 //! @sa getsockopt 00467 // 00468 //***************************************************************************** 00469 #ifndef CC3000_TINY_DRIVER 00470 extern int setsockopt(long sd, long level, long optname, const void *optval, 00471 socklen_t optlen); 00472 #endif 00473 //***************************************************************************** 00474 // 00475 //! getsockopt 00476 //! 00477 //! @param[in] sd socket handle 00478 //! @param[in] level defines the protocol level for this option 00479 //! @param[in] optname defines the option name to Interrogate 00480 //! @param[out] optval specifies a value for the option 00481 //! @param[out] optlen specifies the length of the option value 00482 //! @return On success, zero is returned. On error, -1 is returned 00483 //! 00484 //! @brief set socket options 00485 //! This function manipulate the options associated with a socket. 00486 //! Options may exist at multiple protocol levels; they are always 00487 //! present at the uppermost socket level. 00488 //! When manipulating socket options the level at which the option 00489 //! resides and the name of the option must be specified. 00490 //! To manipulate options at the socket level, level is specified as 00491 //! SOL_SOCKET. To manipulate options at any other level the protocol 00492 //! number of the appropriate protocol controlling the option is 00493 //! supplied. For example, to indicate that an option is to be 00494 //! interpreted by the TCP protocol, level should be set to the 00495 //! protocol number of TCP; 00496 //! The parameters optval and optlen are used to access optval - 00497 //! use for setsockopt(). For getsockopt() they identify a buffer 00498 //! in which the value for the requested option(s) are to 00499 //! be returned. For getsockopt(), optlen is a value-result 00500 //! parameter, initially containing the size of the buffer 00501 //! pointed to by option_value, and modified on return to 00502 //! indicate the actual size of the value returned. If no option 00503 //! value is to be supplied or returned, option_value may be NULL. 00504 //! 00505 //! @Note On this version the following two socket options are enabled: 00506 //! The only protocol level supported in this version 00507 //! is SOL_SOCKET (level). 00508 //! 1. SOCKOPT_RECV_TIMEOUT (optname) 00509 //! SOCKOPT_RECV_TIMEOUT configures recv and recvfrom timeout 00510 //! in milliseconds. 00511 //! In that case optval should be pointer to unsigned long. 00512 //! 2. SOCKOPT_NONBLOCK (optname). sets the socket non-blocking mode on 00513 //! or off. 00514 //! In that case optval should be SOCK_ON or SOCK_OFF (optval). 00515 //! 00516 //! @sa setsockopt 00517 // 00518 //***************************************************************************** 00519 extern int getsockopt(long sd, long level, long optname, void *optval, 00520 socklen_t *optlen); 00521 00522 //***************************************************************************** 00523 // 00524 //! recv 00525 //! 00526 //! @param[in] sd socket handle 00527 //! @param[out] buf Points to the buffer where the message should be stored 00528 //! @param[in] len Specifies the length in bytes of the buffer pointed to 00529 //! by the buffer argument. 00530 //! @param[in] flags Specifies the type of message reception. 00531 //! On this version, this parameter is not supported. 00532 //! 00533 //! @return Return the number of bytes received, or -1 if an error 00534 //! occurred 00535 //! 00536 //! @brief function receives a message from a connection-mode socket 00537 //! 00538 //! @sa recvfrom 00539 //! 00540 //! @Note On this version, only blocking mode is supported. 00541 // 00542 //***************************************************************************** 00543 extern int recv(long sd, unsigned char *buf, long len, long flags); 00544 00545 //***************************************************************************** 00546 // 00547 //! recvfrom 00548 //! 00549 //! @param[in] sd socket handle 00550 //! @param[out] buf Points to the buffer where the message should be stored 00551 //! @param[in] len Specifies the length in bytes of the buffer pointed to 00552 //! by the buffer argument. 00553 //! @param[in] flags Specifies the type of message reception. 00554 //! On this version, this parameter is not supported. 00555 //! @param[in] from pointer to an address structure indicating the source 00556 //! address: sockaddr. On this version only AF_INET is 00557 //! supported. 00558 //! @param[in] fromlen source address structure size 00559 //! 00560 //! @return Return the number of bytes received, or -1 if an error 00561 //! occurred 00562 //! 00563 //! @brief read data from socket 00564 //! function receives a message from a connection-mode or 00565 //! connectionless-mode socket. Note that raw sockets are not 00566 //! supported. 00567 //! 00568 //! @sa recv 00569 //! 00570 //! @Note On this version, only blocking mode is supported. 00571 // 00572 //***************************************************************************** 00573 extern int recvfrom(long sd, void *buf, long len, long flags, sockaddr *from, 00574 socklen_t *fromlen); 00575 00576 //***************************************************************************** 00577 // 00578 //! send 00579 //! 00580 //! @param sd socket handle 00581 //! @param buf Points to a buffer containing the message to be sent 00582 //! @param len message size in bytes 00583 //! @param flags On this version, this parameter is not supported 00584 //! 00585 //! @return Return the number of bytes transmitted, or -1 if an 00586 //! error occurred 00587 //! 00588 //! @brief Write data to TCP socket 00589 //! This function is used to transmit a message to another 00590 //! socket. 00591 //! 00592 //! @Note On this version, only blocking mode is supported. 00593 //! 00594 //! @sa sendto 00595 // 00596 //***************************************************************************** 00597 00598 extern int send(long sd, const void *buf, long len, long flags); 00599 00600 //***************************************************************************** 00601 // 00602 //! sendto 00603 //! 00604 //! @param sd socket handle 00605 //! @param buf Points to a buffer containing the message to be sent 00606 //! @param len message size in bytes 00607 //! @param flags On this version, this parameter is not supported 00608 //! @param to pointer to an address structure indicating the destination 00609 //! address: sockaddr. On this version only AF_INET is 00610 //! supported. 00611 //! @param tolen destination address structure size 00612 //! 00613 //! @return Return the number of bytes transmitted, or -1 if an 00614 //! error occurred 00615 //! 00616 //! @brief Write data to TCP socket 00617 //! This function is used to transmit a message to another 00618 //! socket. 00619 //! 00620 //! @Note On this version, only blocking mode is supported. 00621 //! 00622 //! @sa send 00623 // 00624 //***************************************************************************** 00625 00626 extern int sendto(long sd, const void *buf, long len, long flags, 00627 const sockaddr *to, socklen_t tolen); 00628 00629 //***************************************************************************** 00630 // 00631 //! mdnsAdvertiser 00632 //! 00633 //! @param[in] mdnsEnabled flag to enable/disable the mDNS feature 00634 //! @param[in] deviceServiceName Service name as part of the published 00635 //! canonical domain name 00636 //! @param[in] deviceServiceNameLength Length of the service name 00637 //! 00638 //! 00639 //! @return On success, zero is returned, return SOC_ERROR if socket was not 00640 //! opened successfully, or if an error occurred. 00641 //! 00642 //! @brief Set CC3000 in mDNS advertiser mode in order to advertise itself. 00643 // 00644 //***************************************************************************** 00645 extern int mdnsAdvertiser(unsigned short mdnsEnabled, char * deviceServiceName, unsigned short deviceServiceNameLength); 00646 00647 //***************************************************************************** 00648 // 00649 // Close the Doxygen group. 00650 //! @} 00651 // 00652 //***************************************************************************** 00653 00654 00655 //***************************************************************************** 00656 // 00657 // Mark the end of the C bindings section for C++ compilers. 00658 // 00659 //***************************************************************************** 00660 #ifdef __cplusplus 00661 } 00662 #endif // __cplusplus 00663 00664 #endif // __SOCKET_H__ 00665
Generated on Tue Jul 12 2022 19:26:44 by
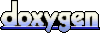