CC3000HostDriver for device TI CC3000 some changes were made due to mbed compiler and the use of void*
Embed:
(wiki syntax)
Show/hide line numbers
cc3000_common.cpp
00001 /***************************************************************************** 00002 * 00003 * cc3000_common.c.c - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 //***************************************************************************** 00036 // 00037 //! \addtogroup common_api 00038 //! @{ 00039 // 00040 //***************************************************************************** 00041 /****************************************************************************** 00042 * 00043 * Include files 00044 * 00045 *****************************************************************************/ 00046 #include "cc3000_common.h" 00047 #include "socket.h" 00048 #include "wlan.h" 00049 #include "evnt_handler.h" 00050 00051 //***************************************************************************** 00052 // 00053 //! __error__ 00054 //! 00055 //! @param pcFilename - file name, where error occurred 00056 //! @param ulLine - line number, where error occurred 00057 //! 00058 //! @return none 00059 //! 00060 //! @brief stub function for ASSERT macro 00061 // 00062 //***************************************************************************** 00063 void 00064 __error__(char *pcFilename, unsigned long ulLine) 00065 { 00066 //TODO full up function 00067 } 00068 00069 00070 00071 //***************************************************************************** 00072 // 00073 //! UINT32_TO_STREAM_f 00074 //! 00075 //! @param p pointer to the new stream 00076 //! @param u32 pointer to the 32 bit 00077 //! 00078 //! @return pointer to the new stream 00079 //! 00080 //! @brief This function is used for copying 32 bit to stream 00081 //! while converting to little endian format. 00082 // 00083 //***************************************************************************** 00084 00085 unsigned char* UINT32_TO_STREAM_f (unsigned char *p, unsigned long u32) 00086 { 00087 *(p)++ = (unsigned char)(u32); 00088 *(p)++ = (unsigned char)((u32) >> 8); 00089 *(p)++ = (unsigned char)((u32) >> 16); 00090 *(p)++ = (unsigned char)((u32) >> 24); 00091 return p; 00092 } 00093 00094 //***************************************************************************** 00095 // 00096 //! UINT16_TO_STREAM_f 00097 //! 00098 //! @param p pointer to the new stream 00099 //! @param u32 pointer to the 16 bit 00100 //! 00101 //! @return pointer to the new stream 00102 //! 00103 //! @brief This function is used for copying 16 bit to stream 00104 //! while converting to little endian format. 00105 // 00106 //***************************************************************************** 00107 00108 unsigned char* UINT16_TO_STREAM_f (unsigned char *p, unsigned short u16) 00109 { 00110 *(p)++ = (unsigned char)(u16); 00111 *(p)++ = (unsigned char)((u16) >> 8); 00112 return p; 00113 } 00114 00115 //***************************************************************************** 00116 // 00117 //! STREAM_TO_UINT16_f 00118 //! 00119 //! @param p pointer to the stream 00120 //! @param offset offset in the stream 00121 //! 00122 //! @return pointer to the new 16 bit 00123 //! 00124 //! @brief This function is used for copying received stream to 00125 //! 16 bit in little endian format. 00126 // 00127 //***************************************************************************** 00128 00129 unsigned short STREAM_TO_UINT16_f(char* p, unsigned short offset) 00130 { 00131 return (unsigned short)((unsigned short)((unsigned short) 00132 (*(p + offset + 1)) << 8) + (unsigned short)(*(p + offset))); 00133 } 00134 00135 //***************************************************************************** 00136 // 00137 //! STREAM_TO_UINT32_f 00138 //! 00139 //! @param p pointer to the stream 00140 //! @param offset offset in the stream 00141 //! 00142 //! @return pointer to the new 32 bit 00143 //! 00144 //! @brief This function is used for copying received stream to 00145 //! 32 bit in little endian format. 00146 // 00147 //***************************************************************************** 00148 00149 unsigned long STREAM_TO_UINT32_f(char* p, unsigned short offset) 00150 { 00151 return (unsigned long)((unsigned long)((unsigned long) 00152 (*(p + offset + 3)) << 24) + (unsigned long)((unsigned long) 00153 (*(p + offset + 2)) << 16) + (unsigned long)((unsigned long) 00154 (*(p + offset + 1)) << 8) + (unsigned long)(*(p + offset))); 00155 } 00156 00157 00158 00159 //***************************************************************************** 00160 // 00161 // Close the Doxygen group. 00162 //! @} 00163 // 00164 //***************************************************************************** 00165
Generated on Tue Jul 12 2022 19:26:44 by
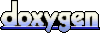