CC3000HostDriver for device TI CC3000 some changes were made due to mbed compiler and the use of void*
Embed:
(wiki syntax)
Show/hide line numbers
evnt_handler.cpp
00001 /***************************************************************************** 00002 * 00003 * evnt_handler.c - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 //***************************************************************************** 00036 // 00037 //! \addtogroup evnt_handler_api 00038 //! @{ 00039 // 00040 //****************************************************************************** 00041 00042 //****************************************************************************** 00043 // INCLUDE FILES 00044 //****************************************************************************** 00045 00046 #include "cc3000_common.h" 00047 #include "string.h" 00048 #include "hci.h" 00049 #include "evnt_handler.h" 00050 #include "wlan.h" 00051 #include "socket.h" 00052 #include "netapp.h" 00053 #include "mbed.h" 00054 #include "spi.h" 00055 00056 00057 00058 //***************************************************************************** 00059 // COMMON DEFINES 00060 //***************************************************************************** 00061 00062 #define FLOW_CONTROL_EVENT_HANDLE_OFFSET (0) 00063 #define FLOW_CONTROL_EVENT_BLOCK_MODE_OFFSET (1) 00064 #define FLOW_CONTROL_EVENT_FREE_BUFFS_OFFSET (2) 00065 #define FLOW_CONTROL_EVENT_SIZE (4) 00066 00067 #define BSD_RSP_PARAMS_SOCKET_OFFSET (0) 00068 #define BSD_RSP_PARAMS_STATUS_OFFSET (4) 00069 00070 #define GET_HOST_BY_NAME_RETVAL_OFFSET (0) 00071 #define GET_HOST_BY_NAME_ADDR_OFFSET (4) 00072 00073 #define ACCEPT_SD_OFFSET (0) 00074 #define ACCEPT_RETURN_STATUS_OFFSET (4) 00075 #define ACCEPT_ADDRESS__OFFSET (8) 00076 00077 #define SL_RECEIVE_SD_OFFSET (0) 00078 #define SL_RECEIVE_NUM_BYTES_OFFSET (4) 00079 #define SL_RECEIVE__FLAGS__OFFSET (8) 00080 00081 00082 #define SELECT_STATUS_OFFSET (0) 00083 #define SELECT_READFD_OFFSET (4) 00084 #define SELECT_WRITEFD_OFFSET (8) 00085 #define SELECT_EXFD_OFFSET (12) 00086 00087 00088 #define NETAPP_IPCONFIG_IP_OFFSET (0) 00089 #define NETAPP_IPCONFIG_SUBNET_OFFSET (4) 00090 #define NETAPP_IPCONFIG_GW_OFFSET (8) 00091 #define NETAPP_IPCONFIG_DHCP_OFFSET (12) 00092 #define NETAPP_IPCONFIG_DNS_OFFSET (16) 00093 #define NETAPP_IPCONFIG_MAC_OFFSET (20) 00094 #define NETAPP_IPCONFIG_SSID_OFFSET (26) 00095 00096 #define NETAPP_IPCONFIG_IP_LENGTH (4) 00097 #define NETAPP_IPCONFIG_MAC_LENGTH (6) 00098 #define NETAPP_IPCONFIG_SSID_LENGTH (32) 00099 00100 00101 #define NETAPP_PING_PACKETS_SENT_OFFSET (0) 00102 #define NETAPP_PING_PACKETS_RCVD_OFFSET (4) 00103 #define NETAPP_PING_MIN_RTT_OFFSET (8) 00104 #define NETAPP_PING_MAX_RTT_OFFSET (12) 00105 #define NETAPP_PING_AVG_RTT_OFFSET (16) 00106 00107 #define GET_SCAN_RESULTS_TABlE_COUNT_OFFSET (0) 00108 #define GET_SCAN_RESULTS_SCANRESULT_STATUS_OFFSET (4) 00109 #define GET_SCAN_RESULTS_ISVALID_TO_SSIDLEN_OFFSET (8) 00110 #define GET_SCAN_RESULTS_FRAME_TIME_OFFSET (10) 00111 #define GET_SCAN_RESULTS_SSID_MAC_LENGTH (38) 00112 00113 00114 00115 //***************************************************************************** 00116 // GLOBAL VARAIABLES 00117 //***************************************************************************** 00118 00119 unsigned long socket_active_status = SOCKET_STATUS_INIT_VAL; 00120 00121 00122 //***************************************************************************** 00123 // Prototypes for the static functions 00124 //***************************************************************************** 00125 00126 static long hci_event_unsol_flowcontrol_handler(char *pEvent); 00127 00128 static void update_socket_active_status(char *resp_params); 00129 00130 00131 //***************************************************************************** 00132 // 00133 //! hci_unsol_handle_patch_request 00134 //! 00135 //! @param event_hdr event header 00136 //! 00137 //! @return none 00138 //! 00139 //! @brief Handle unsolicited event from type patch request 00140 // 00141 //***************************************************************************** 00142 void hci_unsol_handle_patch_request(char *event_hdr) 00143 { 00144 char *params = (char *)(event_hdr) + HCI_EVENT_HEADER_SIZE; 00145 unsigned long ucLength = 0; 00146 char *patch; 00147 00148 switch (*params) 00149 { 00150 case HCI_EVENT_PATCHES_DRV_REQ: 00151 00152 if (tSLInformation.sDriverPatches) 00153 { 00154 patch = tSLInformation.sDriverPatches(&ucLength); 00155 00156 if (patch) 00157 { 00158 hci_patch_send(HCI_EVENT_PATCHES_DRV_REQ, tSLInformation.pucTxCommandBuffer, patch, ucLength); 00159 return; 00160 } 00161 } 00162 00163 // Send 0 length Patches response event 00164 hci_patch_send(HCI_EVENT_PATCHES_DRV_REQ, tSLInformation.pucTxCommandBuffer, 0, 0); 00165 break; 00166 00167 case HCI_EVENT_PATCHES_FW_REQ: 00168 00169 if (tSLInformation.sFWPatches) 00170 { 00171 patch = tSLInformation.sFWPatches(&ucLength); 00172 00173 // Build and send a patch 00174 if (patch) 00175 { 00176 hci_patch_send(HCI_EVENT_PATCHES_FW_REQ, tSLInformation.pucTxCommandBuffer, patch, ucLength); 00177 return; 00178 } 00179 } 00180 00181 // Send 0 length Patches response event 00182 hci_patch_send(HCI_EVENT_PATCHES_FW_REQ, tSLInformation.pucTxCommandBuffer, 0, 0); 00183 break; 00184 00185 case HCI_EVENT_PATCHES_BOOTLOAD_REQ: 00186 00187 if (tSLInformation.sBootLoaderPatches) 00188 { 00189 patch = tSLInformation.sBootLoaderPatches(&ucLength); 00190 00191 if (patch) 00192 { 00193 hci_patch_send(HCI_EVENT_PATCHES_BOOTLOAD_REQ, tSLInformation.pucTxCommandBuffer, patch, ucLength); 00194 return; 00195 } 00196 } 00197 00198 // Send 0 length Patches response event 00199 hci_patch_send(HCI_EVENT_PATCHES_BOOTLOAD_REQ, tSLInformation.pucTxCommandBuffer, 0, 0); 00200 break; 00201 } 00202 } 00203 00204 00205 00206 //***************************************************************************** 00207 // 00208 //! hci_event_handler 00209 //! 00210 //! @param pRetParams incoming data buffer 00211 //! @param from from information (in case of data received) 00212 //! @param fromlen from information length (in case of data received) 00213 //! 00214 //! @return none 00215 //! 00216 //! @brief Parse the incoming events packets and issues corresponding 00217 //! event handler from global array of handlers pointers 00218 // 00219 //***************************************************************************** 00220 00221 //unsigned char * hci_event_handler(void *pRetParams, unsigned char *from, unsigned char *fromlen) 00222 unsigned char * 00223 hci_event_handler(char *pRetParams, unsigned char *from, unsigned char *fromlen) 00224 { 00225 unsigned char *pucReceivedData, ucArgsize; 00226 unsigned short usLength = 0; 00227 unsigned char *pucReceivedParams; 00228 unsigned short usReceivedEventOpcode = 0; 00229 unsigned long retValue32; 00230 unsigned char * RecvParams; 00231 char *RetParams; 00232 00233 00234 while (1) 00235 { 00236 00237 if (tSLInformation.usEventOrDataReceived != 0) 00238 { 00239 00240 pucReceivedData = (tSLInformation.pucReceivedData); 00241 00242 00243 if (*pucReceivedData == HCI_TYPE_EVNT) 00244 { 00245 00246 // Event Received 00247 STREAM_TO_UINT16((char *)pucReceivedData, HCI_EVENT_OPCODE_OFFSET, usReceivedEventOpcode); 00248 pucReceivedParams = pucReceivedData + HCI_EVENT_HEADER_SIZE; 00249 RecvParams = pucReceivedParams; 00250 RetParams = pRetParams; 00251 00252 // In case unsolicited event received - here the handling finished 00253 00254 if (hci_unsol_event_handler((char *)pucReceivedData) == 0) 00255 { 00256 00257 STREAM_TO_UINT8(pucReceivedData, HCI_DATA_LENGTH_OFFSET, usLength); 00258 00259 switch(usReceivedEventOpcode) 00260 { 00261 case HCI_CMND_READ_BUFFER_SIZE: 00262 { 00263 00264 STREAM_TO_UINT8((char *)pucReceivedParams, 0, 00265 tSLInformation.usNumberOfFreeBuffers); 00266 STREAM_TO_UINT16((char *)pucReceivedParams, 1, 00267 tSLInformation.usSlBufferLength); 00268 } 00269 00270 break; 00271 00272 case HCI_CMND_WLAN_CONFIGURE_PATCH: 00273 case HCI_NETAPP_DHCP: 00274 case HCI_NETAPP_PING_SEND: 00275 case HCI_NETAPP_PING_STOP: 00276 case HCI_NETAPP_ARP_FLUSH: 00277 case HCI_NETAPP_SET_DEBUG_LEVEL: 00278 case HCI_NETAPP_SET_TIMERS: 00279 case HCI_EVNT_NVMEM_READ: 00280 case HCI_EVNT_NVMEM_CREATE_ENTRY: 00281 case HCI_CMND_NVMEM_WRITE_PATCH: 00282 case HCI_NETAPP_PING_REPORT: 00283 case HCI_EVNT_MDNS_ADVERTISE: 00284 00285 STREAM_TO_UINT8(pucReceivedData, HCI_EVENT_STATUS_OFFSET, *(unsigned char *)pRetParams); 00286 00287 break; 00288 00289 case HCI_CMND_SETSOCKOPT: 00290 case HCI_CMND_WLAN_CONNECT: 00291 case HCI_CMND_WLAN_IOCTL_STATUSGET: 00292 case HCI_EVNT_WLAN_IOCTL_ADD_PROFILE: 00293 case HCI_CMND_WLAN_IOCTL_DEL_PROFILE: 00294 case HCI_CMND_WLAN_IOCTL_SET_CONNECTION_POLICY: 00295 case HCI_CMND_WLAN_IOCTL_SET_SCANPARAM: 00296 case HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_START: 00297 case HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_STOP: 00298 case HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_SET_PREFIX: 00299 case HCI_CMND_EVENT_MASK: 00300 case HCI_EVNT_WLAN_DISCONNECT: 00301 case HCI_EVNT_SOCKET: 00302 case HCI_EVNT_BIND: 00303 case HCI_CMND_LISTEN: 00304 case HCI_EVNT_CLOSE_SOCKET: 00305 case HCI_EVNT_CONNECT: 00306 case HCI_EVNT_NVMEM_WRITE: 00307 00308 STREAM_TO_UINT32((char *)pucReceivedParams, 0, *(unsigned long *)pRetParams); 00309 00310 break; 00311 00312 case HCI_EVNT_READ_SP_VERSION: 00313 00314 STREAM_TO_UINT8(pucReceivedData, HCI_EVENT_STATUS_OFFSET, *(unsigned char *)pRetParams); 00315 pRetParams = ((char *)pRetParams) + 1; 00316 STREAM_TO_UINT32((char *)pucReceivedParams, 0, retValue32); 00317 UINT32_TO_STREAM((unsigned char *)pRetParams, retValue32); 00318 00319 break; 00320 00321 case HCI_EVNT_BSD_GETHOSTBYNAME: 00322 00323 STREAM_TO_UINT32((char *)pucReceivedParams, GET_HOST_BY_NAME_RETVAL_OFFSET,*(unsigned long *)pRetParams); 00324 pRetParams = ((char *)pRetParams) + 4; 00325 STREAM_TO_UINT32((char *)pucReceivedParams, GET_HOST_BY_NAME_ADDR_OFFSET,*(unsigned long *)pRetParams); 00326 00327 break; 00328 00329 case HCI_EVNT_ACCEPT: 00330 { 00331 STREAM_TO_UINT32((char *)pucReceivedParams,ACCEPT_SD_OFFSET,*(unsigned long *)pRetParams); 00332 pRetParams = ((char *)pRetParams) + 4; 00333 STREAM_TO_UINT32((char *)pucReceivedParams,ACCEPT_RETURN_STATUS_OFFSET,*(unsigned long *)pRetParams); 00334 pRetParams = ((char *)pRetParams) + 4; 00335 00336 //This argument returns in network order 00337 memcpy((unsigned char *)pRetParams, pucReceivedParams + ACCEPT_ADDRESS__OFFSET, sizeof(sockaddr)); 00338 00339 break; 00340 } 00341 00342 case HCI_EVNT_RECV: 00343 case HCI_EVNT_RECVFROM: 00344 { 00345 STREAM_TO_UINT32((char *)pucReceivedParams,SL_RECEIVE_SD_OFFSET ,*(unsigned long *)pRetParams); 00346 pRetParams = ((char *)pRetParams) + 4; 00347 STREAM_TO_UINT32((char *)pucReceivedParams,SL_RECEIVE_NUM_BYTES_OFFSET,*(unsigned long *)pRetParams); 00348 pRetParams = ((char *)pRetParams) + 4; 00349 STREAM_TO_UINT32((char *)pucReceivedParams,SL_RECEIVE__FLAGS__OFFSET,*(unsigned long *)pRetParams); 00350 00351 if(((tBsdReadReturnParams *)pRetParams)->iNumberOfBytes == ERROR_SOCKET_INACTIVE) 00352 { 00353 00354 set_socket_active_status(((tBsdReadReturnParams *)pRetParams)->iSocketDescriptor,SOCKET_STATUS_INACTIVE); 00355 00356 } 00357 00358 break; 00359 } 00360 00361 case HCI_EVNT_SEND: 00362 case HCI_EVNT_SENDTO: 00363 { 00364 STREAM_TO_UINT32((char *)pucReceivedParams,SL_RECEIVE_SD_OFFSET ,*(unsigned long *)pRetParams); 00365 pRetParams = ((char *)pRetParams) + 4; 00366 STREAM_TO_UINT32((char *)pucReceivedParams,SL_RECEIVE_NUM_BYTES_OFFSET,*(unsigned long *)pRetParams); 00367 pRetParams = ((char *)pRetParams) + 4; 00368 00369 break; 00370 } 00371 00372 case HCI_EVNT_SELECT: 00373 { 00374 STREAM_TO_UINT32((char *)pucReceivedParams,SELECT_STATUS_OFFSET,*(unsigned long *)pRetParams); 00375 pRetParams = ((char *)pRetParams) + 4; 00376 STREAM_TO_UINT32((char *)pucReceivedParams,SELECT_READFD_OFFSET,*(unsigned long *)pRetParams); 00377 pRetParams = ((char *)pRetParams) + 4; 00378 STREAM_TO_UINT32((char *)pucReceivedParams,SELECT_WRITEFD_OFFSET,*(unsigned long *)pRetParams); 00379 pRetParams = ((char *)pRetParams) + 4; 00380 STREAM_TO_UINT32((char *)pucReceivedParams,SELECT_EXFD_OFFSET,*(unsigned long *)pRetParams); 00381 00382 break; 00383 } 00384 00385 case HCI_CMND_GETSOCKOPT: 00386 00387 STREAM_TO_UINT8(pucReceivedData, HCI_EVENT_STATUS_OFFSET,((tBsdGetSockOptReturnParams *)pRetParams)->iStatus); 00388 //This argument returns in network order 00389 memcpy((unsigned char *)pRetParams, pucReceivedParams, 4); 00390 00391 break; 00392 00393 case HCI_CMND_WLAN_IOCTL_GET_SCAN_RESULTS: 00394 00395 STREAM_TO_UINT32((char *)pucReceivedParams,GET_SCAN_RESULTS_TABlE_COUNT_OFFSET,*(unsigned long *)pRetParams); 00396 pRetParams = ((char *)pRetParams) + 4; 00397 STREAM_TO_UINT32((char *)pucReceivedParams,GET_SCAN_RESULTS_SCANRESULT_STATUS_OFFSET,*(unsigned long *)pRetParams); 00398 pRetParams = ((char *)pRetParams) + 4; 00399 STREAM_TO_UINT16((char *)pucReceivedParams,GET_SCAN_RESULTS_ISVALID_TO_SSIDLEN_OFFSET,*(unsigned long *)pRetParams); 00400 pRetParams = ((char *)pRetParams) + 2; 00401 STREAM_TO_UINT16((char *)pucReceivedParams,GET_SCAN_RESULTS_FRAME_TIME_OFFSET,*(unsigned long *)pRetParams); 00402 pRetParams = ((char *)pRetParams) + 2; 00403 memcpy((unsigned char *)pRetParams, (char *)(pucReceivedParams + GET_SCAN_RESULTS_FRAME_TIME_OFFSET + 2), GET_SCAN_RESULTS_SSID_MAC_LENGTH); 00404 00405 break; 00406 00407 case HCI_CMND_SIMPLE_LINK_START: 00408 00409 break; 00410 00411 case HCI_NETAPP_IPCONFIG: 00412 00413 //Read IP address 00414 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_IP_LENGTH); 00415 RecvParams += 4; 00416 00417 //Read subnet 00418 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_IP_LENGTH); 00419 RecvParams += 4; 00420 00421 //Read default GW 00422 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_IP_LENGTH); 00423 RecvParams += 4; 00424 00425 //Read DHCP server 00426 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_IP_LENGTH); 00427 RecvParams += 4; 00428 00429 //Read DNS server 00430 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_IP_LENGTH); 00431 RecvParams += 4; 00432 00433 //Read Mac address 00434 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_MAC_LENGTH); 00435 RecvParams += 6; 00436 00437 //Read SSID 00438 STREAM_TO_STREAM(RecvParams,RetParams,NETAPP_IPCONFIG_SSID_LENGTH); 00439 00440 } 00441 } 00442 00443 if (usReceivedEventOpcode == tSLInformation.usRxEventOpcode) 00444 00445 { 00446 tSLInformation.usRxEventOpcode = 0; 00447 } 00448 } 00449 else 00450 { 00451 00452 pucReceivedParams = pucReceivedData; 00453 STREAM_TO_UINT8((char *)pucReceivedData, HCI_PACKET_ARGSIZE_OFFSET, ucArgsize); 00454 00455 STREAM_TO_UINT16((char *)pucReceivedData, HCI_PACKET_LENGTH_OFFSET, usLength); 00456 00457 // Data received: note that the only case where from and from length 00458 // are not null is in recv from, so fill the args accordingly 00459 if (from) 00460 { 00461 00462 STREAM_TO_UINT32((char *)(pucReceivedData + HCI_DATA_HEADER_SIZE), BSD_RECV_FROM_FROMLEN_OFFSET, *(unsigned long *)fromlen); 00463 00464 memcpy(from, (pucReceivedData + HCI_DATA_HEADER_SIZE + BSD_RECV_FROM_FROM_OFFSET) ,*fromlen); 00465 } 00466 00467 00468 00469 memcpy(pRetParams, pucReceivedParams + HCI_DATA_HEADER_SIZE + ucArgsize, usLength - ucArgsize); 00470 00471 tSLInformation.usRxDataPending = 0; 00472 00473 } 00474 00475 tSLInformation.usEventOrDataReceived = 0; 00476 00477 SpiResumeSpi(); 00478 00479 // Since we are going to TX - we need to handle this event after the 00480 // ResumeSPi since we need interrupts 00481 if ((*pucReceivedData == HCI_TYPE_EVNT) && (usReceivedEventOpcode == HCI_EVNT_PATCHES_REQ)) 00482 { 00483 hci_unsol_handle_patch_request((char *)pucReceivedData); 00484 00485 } 00486 00487 if ((tSLInformation.usRxEventOpcode == 0) && (tSLInformation.usRxDataPending == 0)) 00488 { 00489 00490 return NULL; 00491 } 00492 } 00493 } 00494 00495 } 00496 00497 //***************************************************************************** 00498 // 00499 //! hci_unsol_event_handler 00500 //! 00501 //! @param event_hdr event header 00502 //! 00503 //! @return 1 if event supported and handled 00504 //! 0 if event is not supported 00505 //! 00506 //! @brief Handle unsolicited events 00507 // 00508 //***************************************************************************** 00509 long 00510 hci_unsol_event_handler(char *event_hdr) 00511 { 00512 char * data = NULL; 00513 long event_type; 00514 unsigned long NumberOfReleasedPackets; 00515 unsigned long NumberOfSentPackets; 00516 00517 STREAM_TO_UINT16(event_hdr, HCI_EVENT_OPCODE_OFFSET,event_type); 00518 00519 if (event_type & HCI_EVNT_UNSOL_BASE) 00520 { 00521 00522 switch(event_type) 00523 { 00524 00525 case HCI_EVNT_DATA_UNSOL_FREE_BUFF: 00526 { 00527 hci_event_unsol_flowcontrol_handler(event_hdr); 00528 00529 NumberOfReleasedPackets = tSLInformation.NumberOfReleasedPackets; 00530 NumberOfSentPackets = tSLInformation.NumberOfSentPackets; 00531 00532 if (NumberOfReleasedPackets == NumberOfSentPackets) 00533 { 00534 if (tSLInformation.InformHostOnTxComplete) 00535 { 00536 tSLInformation.sWlanCB(HCI_EVENT_CC3000_CAN_SHUT_DOWN, NULL, 0); 00537 } 00538 } 00539 return 1; 00540 00541 } 00542 } 00543 } 00544 00545 if(event_type & HCI_EVNT_WLAN_UNSOL_BASE) 00546 { 00547 switch(event_type) 00548 { 00549 case HCI_EVNT_WLAN_KEEPALIVE: 00550 case HCI_EVNT_WLAN_UNSOL_CONNECT: 00551 case HCI_EVNT_WLAN_UNSOL_DISCONNECT: 00552 case HCI_EVNT_WLAN_UNSOL_INIT: 00553 case HCI_EVNT_WLAN_ASYNC_SIMPLE_CONFIG_DONE: 00554 00555 if( tSLInformation.sWlanCB ) 00556 { 00557 tSLInformation.sWlanCB(event_type, 0, 0); 00558 } 00559 break; 00560 00561 case HCI_EVNT_WLAN_UNSOL_DHCP: 00562 { 00563 unsigned char params[NETAPP_IPCONFIG_MAC_OFFSET + 1]; // extra byte is for the status 00564 unsigned char *recParams = params; 00565 00566 data = (char*)(event_hdr) + HCI_EVENT_HEADER_SIZE; 00567 00568 //Read IP address 00569 STREAM_TO_STREAM(data,recParams,NETAPP_IPCONFIG_IP_LENGTH); 00570 data += 4; 00571 //Read subnet 00572 STREAM_TO_STREAM(data,recParams,NETAPP_IPCONFIG_IP_LENGTH); 00573 data += 4; 00574 //Read default GW 00575 STREAM_TO_STREAM(data,recParams,NETAPP_IPCONFIG_IP_LENGTH); 00576 data += 4; 00577 //Read DHCP server 00578 STREAM_TO_STREAM(data,recParams,NETAPP_IPCONFIG_IP_LENGTH); 00579 data += 4; 00580 //Read DNS server 00581 STREAM_TO_STREAM(data,recParams,NETAPP_IPCONFIG_IP_LENGTH); 00582 // read the status 00583 STREAM_TO_UINT8(event_hdr, HCI_EVENT_STATUS_OFFSET, *recParams); 00584 00585 00586 if( tSLInformation.sWlanCB ) 00587 { 00588 tSLInformation.sWlanCB(event_type, (char *)params, sizeof(params)); 00589 } 00590 } 00591 break; 00592 00593 case HCI_EVNT_WLAN_ASYNC_PING_REPORT: 00594 { 00595 netapp_pingreport_args_t params; 00596 data = (char*)(event_hdr) + HCI_EVENT_HEADER_SIZE; 00597 STREAM_TO_UINT32(data, NETAPP_PING_PACKETS_SENT_OFFSET, params.packets_sent); 00598 STREAM_TO_UINT32(data, NETAPP_PING_PACKETS_RCVD_OFFSET, params.packets_received); 00599 STREAM_TO_UINT32(data, NETAPP_PING_MIN_RTT_OFFSET, params.min_round_time); 00600 STREAM_TO_UINT32(data, NETAPP_PING_MAX_RTT_OFFSET, params.max_round_time); 00601 STREAM_TO_UINT32(data, NETAPP_PING_AVG_RTT_OFFSET, params.avg_round_time); 00602 00603 if( tSLInformation.sWlanCB ) 00604 { 00605 tSLInformation.sWlanCB(event_type, (char *)¶ms, sizeof(params)); 00606 } 00607 } 00608 break; 00609 case HCI_EVNT_BSD_TCP_CLOSE_WAIT: 00610 { 00611 data = (char *)(event_hdr) + HCI_EVENT_HEADER_SIZE; 00612 if( tSLInformation.sWlanCB ) 00613 { 00614 tSLInformation.sWlanCB(event_type, data, 0); 00615 } 00616 } 00617 break; 00618 00619 //'default' case which means "event not supported" 00620 default: 00621 return (0); 00622 } 00623 return(1); 00624 } 00625 00626 if ((event_type == HCI_EVNT_SEND) || (event_type == HCI_EVNT_SENDTO) || (event_type == HCI_EVNT_WRITE)) 00627 { 00628 char *pArg; 00629 long status; 00630 00631 pArg = M_BSD_RESP_PARAMS_OFFSET(event_hdr); 00632 STREAM_TO_UINT32(pArg, BSD_RSP_PARAMS_STATUS_OFFSET,status); 00633 00634 if (ERROR_SOCKET_INACTIVE == status) 00635 { 00636 // The only synchronous event that can come from SL device in form of 00637 // command complete is "Command Complete" on data sent, in case SL device 00638 // was unable to transmit 00639 STREAM_TO_UINT8(event_hdr, HCI_EVENT_STATUS_OFFSET, tSLInformation.slTransmitDataError); 00640 update_socket_active_status(M_BSD_RESP_PARAMS_OFFSET(event_hdr)); 00641 00642 return (1); 00643 } 00644 else 00645 return (0); 00646 } 00647 00648 return(0); 00649 } 00650 00651 //***************************************************************************** 00652 // 00653 //! hci_unsolicited_event_handler 00654 //! 00655 //! @param None 00656 //! 00657 //! @return ESUCCESS if successful, EFAIL if an error occurred 00658 //! 00659 //! @brief Parse the incoming unsolicited event packets and issues 00660 //! corresponding event handler. 00661 // 00662 //***************************************************************************** 00663 long 00664 hci_unsolicited_event_handler(void) 00665 { 00666 unsigned long res = 0; 00667 unsigned char *pucReceivedData; 00668 00669 if (tSLInformation.usEventOrDataReceived != 0) 00670 { 00671 pucReceivedData = (tSLInformation.pucReceivedData); 00672 00673 if (*pucReceivedData == HCI_TYPE_EVNT) 00674 { 00675 00676 // In case unsolicited event received - here the handling finished 00677 if (hci_unsol_event_handler((char *)pucReceivedData) == 1) 00678 { 00679 00680 // There was an unsolicited event received - we can release the buffer 00681 // and clean the event received 00682 tSLInformation.usEventOrDataReceived = 0; 00683 00684 res = 1; 00685 SpiResumeSpi(); 00686 } 00687 } 00688 } 00689 00690 return res; 00691 } 00692 00693 //***************************************************************************** 00694 // 00695 //! set_socket_active_status 00696 //! 00697 //! @param Sd 00698 //! @param Status 00699 //! @return none 00700 //! 00701 //! @brief Check if the socket ID and status are valid and set 00702 //! accordingly the global socket status 00703 // 00704 //***************************************************************************** 00705 void set_socket_active_status(long Sd, long Status) 00706 { 00707 if(M_IS_VALID_SD(Sd) && M_IS_VALID_STATUS(Status)) 00708 { 00709 socket_active_status &= ~(1 << Sd); /* clean socket's mask */ 00710 socket_active_status |= (Status << Sd); /* set new socket's mask */ 00711 } 00712 } 00713 00714 00715 //***************************************************************************** 00716 // 00717 //! hci_event_unsol_flowcontrol_handler 00718 //! 00719 //! @param pEvent pointer to the string contains parameters for IPERF 00720 //! @return ESUCCESS if successful, EFAIL if an error occurred 00721 //! 00722 //! @brief Called in case unsolicited event from type 00723 //! HCI_EVNT_DATA_UNSOL_FREE_BUFF has received. 00724 //! Keep track on the number of packets transmitted and update the 00725 //! number of free buffer in the SL device. 00726 // 00727 //***************************************************************************** 00728 long 00729 hci_event_unsol_flowcontrol_handler(char *pEvent) 00730 { 00731 00732 long temp, value; 00733 unsigned short i; 00734 unsigned short pusNumberOfHandles=0; 00735 char *pReadPayload; 00736 00737 STREAM_TO_UINT16((char *)pEvent,HCI_EVENT_HEADER_SIZE,pusNumberOfHandles); 00738 pReadPayload = ((char *)pEvent + HCI_EVENT_HEADER_SIZE + sizeof(pusNumberOfHandles)); 00739 temp = 0; 00740 00741 for(i = 0; i < pusNumberOfHandles ; i++) 00742 { 00743 STREAM_TO_UINT16(pReadPayload, FLOW_CONTROL_EVENT_FREE_BUFFS_OFFSET, value); 00744 temp += value; 00745 pReadPayload += FLOW_CONTROL_EVENT_SIZE; 00746 } 00747 00748 tSLInformation.usNumberOfFreeBuffers += temp; 00749 tSLInformation.NumberOfReleasedPackets += temp; 00750 00751 return(ESUCCESS); 00752 } 00753 00754 //***************************************************************************** 00755 // 00756 //! get_socket_active_status 00757 //! 00758 //! @param Sd Socket IS 00759 //! @return Current status of the socket. 00760 //! 00761 //! @brief Retrieve socket status 00762 // 00763 //***************************************************************************** 00764 00765 long 00766 get_socket_active_status(long Sd) 00767 { 00768 if(M_IS_VALID_SD(Sd)) 00769 { 00770 return (socket_active_status & (1 << Sd)) ? SOCKET_STATUS_INACTIVE : SOCKET_STATUS_ACTIVE; 00771 } 00772 return SOCKET_STATUS_INACTIVE; 00773 } 00774 00775 //***************************************************************************** 00776 // 00777 //! update_socket_active_status 00778 //! 00779 //! @param resp_params Socket IS 00780 //! @return Current status of the socket. 00781 //! 00782 //! @brief Retrieve socket status 00783 // 00784 //***************************************************************************** 00785 void 00786 update_socket_active_status(char *resp_params) 00787 { 00788 long status, sd; 00789 00790 STREAM_TO_UINT32(resp_params, BSD_RSP_PARAMS_SOCKET_OFFSET,sd); 00791 STREAM_TO_UINT32(resp_params, BSD_RSP_PARAMS_STATUS_OFFSET,status); 00792 00793 if(ERROR_SOCKET_INACTIVE == status) 00794 { 00795 set_socket_active_status(sd, SOCKET_STATUS_INACTIVE); 00796 } 00797 } 00798 00799 00800 //***************************************************************************** 00801 // 00802 //! SimpleLinkWaitEvent 00803 //! 00804 //! @param usOpcode command operation code 00805 //! @param pRetParams command return parameters 00806 //! 00807 //! @return none 00808 //! 00809 //! @brief Wait for event, pass it to the hci_event_handler and 00810 //! update the event opcode in a global variable. 00811 // 00812 //***************************************************************************** 00813 00814 void 00815 SimpleLinkWaitEvent(unsigned short usOpcode, long* pRetParams) 00816 { 00817 // In the blocking implementation the control to caller will be returned only 00818 // after the end of current transaction 00819 tSLInformation.usRxEventOpcode = usOpcode; 00820 00821 hci_event_handler((char*)pRetParams, 0, 0); 00822 00823 } 00824 00825 //***************************************************************************** 00826 // 00827 //! SimpleLinkWaitData 00828 //! 00829 //! @param pBuf data buffer 00830 //! @param from from information 00831 //! @param fromlen from information length 00832 //! 00833 //! @return none 00834 //! 00835 //! @brief Wait for data, pass it to the hci_event_handler 00836 //! and update in a global variable that there is 00837 //! data to read. 00838 // 00839 //***************************************************************************** 00840 00841 void 00842 SimpleLinkWaitData(unsigned char *pBuf, unsigned char *from, 00843 unsigned char *fromlen) 00844 { 00845 // In the blocking implementation the control to caller will be returned only 00846 // after the end of current transaction, i.e. only after data will be received 00847 tSLInformation.usRxDataPending = 1; 00848 hci_event_handler((char*)pBuf, from, fromlen); 00849 } 00850 00851 //***************************************************************************** 00852 // 00853 // Close the Doxygen group. 00854 //! @} 00855 // 00856 //***************************************************************************** 00857
Generated on Tue Jul 12 2022 19:26:44 by
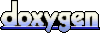