This is a bitbang library for WS2812. I test it with STM32F411RE (Nucleo 441RE). If you want to use another board, u need to adjust the sum of asm("nop"). In the example program i put some code to measure how many asm("nop") do we need. See more detail about WS2812 timing https://cdn-shop.adafruit.com/datasheets/WS2812.pdf
Dependents: STM32FC_RGB_WS2812
WS2812.h
00001 #ifndef WS2812_H 00002 #define WS2812_H 00003 #include "mbed.h" 00004 #include "USBSerial.h" 00005 00006 class WS2812 00007 { 00008 public: 00009 00010 uint8_t Red; 00011 uint8_t Green; 00012 uint8_t Blue; 00013 00014 WS2812(PinName pin, int qty); 00015 ~WS2812(); 00016 void writeColor(uint32_t RGB); 00017 void send1Color(uint32_t RGB); 00018 void sendColors(uint32_t * ColorBuffer); 00019 void sendReset(); 00020 00021 private: 00022 int LED_Qty; 00023 PinName dataPin; 00024 DigitalOut dataOut; 00025 00026 void writeByte(uint8_t data); 00027 void send0(); 00028 void send1(); 00029 }; 00030 00031 #endif
Generated on Tue Jul 19 2022 03:57:51 by
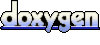