Hexiwear library for communicating with the on-board KW40Z BLE device. KW40Z handles also the touch buttons.
Fork of Hexi_KW40Z by
Hexi_KW40Z.h
00001 /** BLE KW40Z Driver for Hexiwear 00002 * This file contains BLE and Touch Buttons driver functionality for Hexiwear 00003 * 00004 * Redistribution and use in source and binary forms, with or without modification, 00005 * are permitted provided that the following conditions are met: 00006 * 00007 * Redistributions of source code must retain the above copyright notice, this list 00008 * of conditions and the following disclaimer. 00009 * 00010 * Redistributions in binary form must reproduce the above copyright notice, this 00011 * list of conditions and the following disclaimer in the documentation and/or 00012 * other materials provided with the distribution. 00013 * 00014 * Neither the name of NXP, nor the names of its 00015 * contributors may be used to endorse or promote products derived from this 00016 * software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00019 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00020 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00021 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00022 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00023 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00024 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00025 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00026 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00027 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00028 * 00029 * visit: http://www.mikroe.com and http://www.nxp.com 00030 * 00031 * get support at: http://www.mikroe.com/forum and https://community.nxp.com 00032 * 00033 * Project HEXIWEAR, 2015 00034 */ 00035 00036 #ifndef HG_HEXI_KW40Z 00037 #define HG_HEXI_KW40Z 00038 00039 #include "mbed.h" 00040 #include "rtos.h" 00041 00042 //#define LIB_DEBUG 1 00043 00044 #define START_THREAD 1 00045 00046 #define gHostInterface_startByte1 0x55 00047 #define gHostInterface_startByte2 0xAA 00048 #define gHostInterface_trailerByte 0x45 00049 00050 #define gHostInterface_dataSize 23 00051 #define gHostInterface_headerSize 4 00052 00053 #define gHostInterface_retransmitCount 3 00054 #define gHostInterface_retransmitTimeout 100 00055 00056 #define gHostInterface_TxConfirmationEnable 1 // send confirmation when receive packet 00057 #define gHostInterface_RxConfirmationEnable 1 // wait on confirmation from remote side (do retransmit) 00058 00059 /** HEXIWEAR firmware version */ 00060 #define HEXIWEAR_VERSION_PATCH ( 2 ) 00061 #define HEXIWEAR_VERSION_MINOR ( 0 ) 00062 #define HEXIWEAR_VERSION_MAJOR ( 1 ) 00063 00064 /** packet types */ 00065 typedef enum 00066 { 00067 packetType_pressUp = 0, /**< touch press up */ 00068 packetType_pressDown = 1, /**< touch press down */ 00069 packetType_pressLeft = 2, /**< touch press left */ 00070 packetType_pressRight = 3, /**< touch press right */ 00071 packetType_slide = 4, /**< touch slide */ 00072 00073 packetType_batteryLevel = 5, /**< battery Service */ 00074 00075 packetType_accel = 6, /**< motion service */ 00076 packetType_ambiLight = 7, /**< weather service */ 00077 packetType_pressure = 8, /**< weather service */ 00078 00079 00080 packetType_gyro = 9, /**< motion service */ 00081 packetType_temperature = 10, /**< weather service */ 00082 packetType_humidity = 11, /**< weather service */ 00083 packetType_magnet = 12, /**< motion service */ 00084 00085 // packetType_heartRate = 13, /**< health service */ 00086 // packetType_steps = 14, /**< health service */ 00087 packetType_calories = 15, /**< health service */ 00088 packetType_alc = 14, /* add ibreathe packet identifier*/ 00089 00090 /* Alert Service */ 00091 packetType_alertIn = 16, /**< incoming alerts */ 00092 packetType_alertOut = 17, /**< outcoming alerts */ 00093 00094 packetType_passDisplay = 18, /**< key display type */ 00095 00096 /* OTAP procedure types */ 00097 packetType_otapKW40Started = 19, 00098 packetType_otapMK64Started = 20, 00099 packetType_otapCompleted = 21, 00100 packetType_otapFailed = 22, 00101 00102 /* active buttons types */ 00103 packetType_buttonsGroupToggleActive = 23, 00104 packetType_buttonsGroupGetActive = 24, 00105 packetType_buttonsGroupSendActive = 25, 00106 00107 /* Turn off/on bluetooth advertising */ 00108 packetType_advModeGet = 26, 00109 packetType_advModeSend = 27, 00110 packetType_advModeToggle = 28, 00111 00112 packetType_appMode = 29, /**< app mode service */ 00113 00114 /* Link State */ 00115 packetType_linkStateGet = 30, /**< connected */ 00116 packetType_linkStateSend = 31, /**< disconnected */ 00117 00118 packetType_notification = 32, /**< notifications */ 00119 00120 packetType_buildVersion = 33, /**< build version */ 00121 00122 packetType_sleepON = 34, /**< sleep ON */ 00123 packetType_sleepOFF = 35, /**< sleep OFF */ 00124 00125 packetType_OK = 255 /**< OK packet */ 00126 } hostInterface_packetType_t; 00127 00128 /** data-packet structure */ 00129 typedef struct 00130 { 00131 /* NOTE: Size of struct must be multiplier of 4! */ 00132 uint8_t start1; 00133 uint8_t start2; 00134 hostInterface_packetType_t type; 00135 uint8_t length; 00136 uint8_t data[gHostInterface_dataSize + 1]; 00137 } hostInterface_packet_t; 00138 00139 /** incoming alert types */ 00140 typedef enum 00141 { 00142 alertIn_type_notification = 1, 00143 alertIn_type_settings = 2, 00144 alertIn_type_timeUpdate = 3, 00145 } hostInterface_alertIn_type_t; 00146 00147 /** current app enum */ 00148 typedef enum 00149 { 00150 GUI_CURRENT_APP_IDLE = 0, /**< no app active */ 00151 GUI_CURRENT_APP_SENSOR_TAG = 2, /**< sensor tag */ 00152 GUI_CURRENT_APP_HEART_RATE = 5, /**< heart rate */ 00153 GUI_CURRENT_APP_PEDOMETER = 6 /**< Pedometer */ 00154 } gui_current_app_t; 00155 00156 typedef void (*button_t)(void); 00157 typedef void (*alert_t)(uint8_t *data, uint8_t length); 00158 //typedef void (*passkey_t)(uint8_t *data); 00159 typedef void (*passkey_t)(void); 00160 typedef void (*notifications_t)(uint8_t eventId, uint8_t categoryId); 00161 00162 typedef struct name 00163 { 00164 uint8_t ver_patchNumber; 00165 uint8_t ver_minorNumber; 00166 uint8_t ver_majorNumber; 00167 00168 } hexiwear_version_t; 00169 00170 class KW40Z{ 00171 00172 public: 00173 00174 /** 00175 * Create a Hexiwear BLE KW40Z Driver connected to the UART pins 00176 * 00177 * @param txPin UART TX pin 00178 * @param rxPin UART RX pin 00179 */ 00180 KW40Z(PinName txPin,PinName rxPin); 00181 00182 /** 00183 * Destroy the Hexiwear instance 00184 */ 00185 ~KW40Z(); 00186 00187 void attach_buttonUp(button_t btnFct); 00188 void attach_buttonDown(button_t btnFct); 00189 void attach_buttonLeft(button_t btnFct); 00190 void attach_buttonRight(button_t btnFct); 00191 void attach_buttonSlide(button_t btnFct); 00192 00193 void attach_alert(alert_t alertFct); 00194 void attach_passkey(passkey_t passkeyFct); 00195 void attach_notifications(notifications_t notFct); 00196 00197 void SendBatteryLevel(uint8_t percentage); 00198 void SendAccel(uint8_t x, uint8_t y, uint8_t z); 00199 void SendGyro(uint8_t x, uint8_t y, uint8_t z); 00200 void SendMag(uint8_t x, uint8_t y, uint8_t z); 00201 void SendAmbientLight(uint8_t percentage); 00202 void SendTemperature(uint16_t celsius); 00203 void SendHumidity(uint16_t percentage); 00204 void SendPressure(uint16_t pascal); 00205 // add iBreathe 00206 void SendiBreathe(uint16_t ppm); 00207 void SendHeartRate(uint8_t rate); 00208 void SendSteps(uint16_t steps); 00209 void SendCalories(uint16_t calories); 00210 void SendAlert(uint8_t *pData, uint8_t length); 00211 void SendSetApplicationMode(gui_current_app_t mode); 00212 00213 void ToggleTsiGroup(void); 00214 void ToggleAdvertisementMode(void); 00215 00216 uint8_t GetTsiGroup(void); 00217 uint8_t GetAdvertisementMode(void); 00218 uint8_t GetLinkState(void); 00219 hexiwear_version_t GetVersion(void); 00220 00221 uint32_t GetPassKey(void); 00222 00223 00224 00225 00226 00227 private: 00228 RawSerial device; 00229 Thread mainThread; 00230 Thread rxThread; 00231 00232 hostInterface_packet_t hostInterface_rxPacket; 00233 hostInterface_packet_t hostInterface_txPacket; 00234 00235 button_t buttonUpCb; 00236 button_t buttonDownCb; 00237 button_t buttonLeftCb; 00238 button_t buttonRightCb; 00239 button_t buttonSlideCb; 00240 00241 alert_t alertCb; 00242 passkey_t passkeyCb; 00243 notifications_t notificationsCb; 00244 00245 uint8_t * rxBuff; 00246 bool confirmReceived; 00247 00248 hexiwear_version_t kw40_version; 00249 uint8_t activeTsiGroup; 00250 uint8_t advertisementMode; 00251 uint8_t linkState; 00252 uint32_t bondPassKey; 00253 00254 MemoryPool<hostInterface_packet_t, 16> mpool; 00255 Queue<hostInterface_packet_t, 16> queue; 00256 00257 void mainTask(void); 00258 void rxTask(void); 00259 00260 void ProcessBuffer(); 00261 void ProcessReceivedPacket(hostInterface_packet_t * rxPacket); 00262 void SendPacket(hostInterface_packet_t * txPacket, bool confirmRequested); 00263 void SearchStartByte(); 00264 00265 void SendPacketOK(void); 00266 void SendGetActiveTsiGroup(void); 00267 void SendGetAdvertisementMode(void); 00268 void SendGetLinkState(void); 00269 void SendGetVersion(void); 00270 00271 #if defined (LIB_DEBUG) 00272 void DebugPrintRxPacket(); 00273 void DebugPrintTxPacket(hostInterface_packet_t * txPacket); 00274 #endif 00275 00276 static void rxStarter(void const *p); 00277 static void mainStarter(void const *p); 00278 }; 00279 00280 #endif
Generated on Tue Jul 19 2022 07:05:31 by
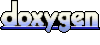