Hexiwear library for communicating with the on-board KW40Z BLE device. KW40Z handles also the touch buttons.
Fork of Hexi_KW40Z by
Hexi_KW40Z.cpp
00001 /** BLE KW40Z Driver for Hexiwear 00002 * This file contains BLE and Touch Buttons driver functionality for Hexiwear 00003 * 00004 * Redistribution and use in source and binary forms, with or without modification, 00005 * are permitted provided that the following conditions are met: 00006 * 00007 * Redistributions of source code must retain the above copyright notice, this list 00008 * of conditions and the following disclaimer. 00009 * 00010 * Redistributions in binary form must reproduce the above copyright notice, this 00011 * list of conditions and the following disclaimer in the documentation and/or 00012 * other materials provided with the distribution. 00013 * 00014 * Neither the name of NXP, nor the names of its 00015 * contributors may be used to endorse or promote products derived from this 00016 * software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00019 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00020 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00021 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00022 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00023 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00024 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00025 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00026 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00027 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00028 * 00029 * visit: http://www.mikroe.com and http://www.nxp.com 00030 * 00031 * get support at: http://www.mikroe.com/forum and https://community.nxp.com 00032 * 00033 * Project HEXIWEAR, 2015 00034 */ 00035 00036 #include "Hexi_KW40Z.h" 00037 00038 #if defined (LIB_DEBUG) 00039 RawSerial pc(USBTX, USBRX); // tx, rx 00040 #endif 00041 00042 KW40Z::KW40Z(PinName txPin,PinName rxPin) : device(txPin, rxPin), mainThread(&KW40Z::mainStarter, this, osPriorityNormal,1024), rxThread(&KW40Z::rxStarter, this, osPriorityNormal,1024) 00043 { 00044 #if defined (LIB_DEBUG) 00045 pc.baud(115200); 00046 pc.printf("Initializing\r\n"); 00047 #endif 00048 00049 device.baud(230400); 00050 device.format(8, Serial::None, 2); 00051 00052 rxBuff = (uint8_t*)&hostInterface_rxPacket; 00053 00054 /* initialize callbacks */ 00055 buttonUpCb = NULL; 00056 buttonDownCb = NULL; 00057 buttonLeftCb = NULL; 00058 buttonRightCb = NULL; 00059 buttonSlideCb = NULL; 00060 alertCb = NULL; 00061 passkeyCb = NULL; 00062 notificationsCb = NULL; 00063 00064 kw40_version.ver_patchNumber = 0; 00065 kw40_version.ver_minorNumber = 0; 00066 kw40_version.ver_majorNumber = 0; 00067 00068 activeTsiGroup = 0; 00069 advertisementMode = 0; 00070 linkState = 0; 00071 bondPassKey = 0; 00072 00073 /* intialization finalized, signal to start the threads */ 00074 mainThread.signal_set(START_THREAD); 00075 rxThread.signal_set(START_THREAD); 00076 } 00077 00078 KW40Z::~KW40Z(void) 00079 { 00080 } 00081 00082 void KW40Z::attach_buttonUp(button_t btnFct) { buttonUpCb = btnFct; } 00083 void KW40Z::attach_buttonDown(button_t btnFct) { buttonDownCb = btnFct; } 00084 void KW40Z::attach_buttonLeft(button_t btnFct) { buttonLeftCb = btnFct; } 00085 void KW40Z::attach_buttonRight(button_t btnFct) { buttonRightCb = btnFct; } 00086 void KW40Z::attach_buttonSlide(button_t btnFct) { buttonSlideCb = btnFct; } 00087 void KW40Z::attach_alert(alert_t alertFct) { alertCb = alertFct; } 00088 void KW40Z::attach_passkey(passkey_t passkeyFct){ passkeyCb = passkeyFct; } 00089 void KW40Z::attach_notifications(notifications_t notFct) { notificationsCb = notFct; } 00090 00091 void KW40Z::rxStarter(void const *p) { 00092 KW40Z *instance = (KW40Z*)p; 00093 instance->rxTask(); 00094 } 00095 00096 void KW40Z::mainStarter(void const *p) { 00097 KW40Z *instance = (KW40Z*)p; 00098 instance->mainTask(); 00099 } 00100 00101 void KW40Z::mainTask(void) 00102 { 00103 mainThread.signal_wait(START_THREAD); 00104 00105 #if defined (LIB_DEBUG) 00106 pc.printf("MainTask Stared\r\n"); 00107 #endif 00108 00109 00110 SendGetActiveTsiGroup(); 00111 SendGetAdvertisementMode(); 00112 SendGetLinkState(); 00113 SendGetVersion(); 00114 00115 00116 while(1) 00117 { 00118 osEvent evt = queue.get(); 00119 if (evt.status == osEventMessage) 00120 { 00121 hostInterface_packet_t *rxPacket = (hostInterface_packet_t*)evt.value.p; 00122 ProcessReceivedPacket(rxPacket); 00123 mpool.free(rxPacket); 00124 } 00125 } 00126 } 00127 00128 void KW40Z::rxTask(void) 00129 { 00130 rxThread.signal_wait(START_THREAD); 00131 00132 #if defined (LIB_DEBUG) 00133 pc.printf("RxTask Stared\r\n"); 00134 #endif 00135 00136 while(1) 00137 { 00138 if(device.readable()) 00139 { 00140 *rxBuff++ = device.getc(); 00141 ProcessBuffer(); 00142 00143 /* check for buffer overflow */ 00144 if(rxBuff >= ((uint8_t*)&hostInterface_rxPacket + sizeof(hostInterface_rxPacket))) 00145 { 00146 rxBuff = (uint8_t*)&hostInterface_rxPacket; 00147 } 00148 } 00149 } 00150 } 00151 00152 #if defined (LIB_DEBUG) 00153 void KW40Z::DebugPrintTxPacket(hostInterface_packet_t * txPacket) 00154 { 00155 char * txBuff = (char *)txPacket; 00156 uint8_t length = txPacket->length + gHostInterface_headerSize + 1; 00157 00158 pc.printf("Tx: "); 00159 for(uint8_t i = 0; i < length; i++) 00160 { 00161 pc.printf("%02X ",*txBuff); 00162 txBuff++; 00163 } 00164 pc.printf("\r\n"); 00165 } 00166 00167 void KW40Z::DebugPrintRxPacket() 00168 { 00169 pc.printf("RX: "); 00170 for(uint8_t * i = (uint8_t*)&hostInterface_rxPacket; i<rxBuff; i++) 00171 { 00172 pc.printf("%02X ",*i); 00173 } 00174 pc.printf("\r\n"); 00175 } 00176 #endif 00177 00178 00179 void KW40Z::SendPacket(hostInterface_packet_t * txPacket, bool confirmRequested) 00180 { 00181 uint8_t retries = 0; 00182 confirmReceived = false; 00183 00184 do 00185 { 00186 char * txBuff = (char *)txPacket; 00187 uint8_t length = txPacket->length + gHostInterface_headerSize + 1; 00188 00189 if(confirmRequested == true) 00190 { 00191 txPacket->start2 |= 0x01; 00192 } 00193 00194 for(uint8_t i = 0; i < length; i++) 00195 { 00196 device.putc(*txBuff); 00197 txBuff++; 00198 } 00199 00200 #if defined (LIB_DEBUG) 00201 DebugPrintTxPacket(txPacket); 00202 #endif 00203 00204 retries++; 00205 00206 #if defined (gHostInterface_RxConfirmationEnable) 00207 if((confirmRequested == true) && (confirmReceived == false)) 00208 { 00209 Thread::wait(gHostInterface_retransmitTimeout); 00210 } 00211 #endif 00212 } 00213 while((confirmRequested == true) && 00214 (confirmReceived == false) && 00215 (retries < gHostInterface_retransmitCount)); 00216 } 00217 00218 void KW40Z::ProcessBuffer() 00219 { 00220 /* check if header has been received */ 00221 if(rxBuff > ((uint8_t*)&hostInterface_rxPacket + gHostInterface_headerSize)) 00222 { 00223 /* check packet header */ 00224 if((gHostInterface_startByte1 != hostInterface_rxPacket.start1)|| 00225 (gHostInterface_startByte2 != (hostInterface_rxPacket.start2 & 0xFE))|| 00226 (hostInterface_rxPacket.length > gHostInterface_dataSize)) 00227 { 00228 #if defined (LIB_DEBUG) 00229 DebugPrintRxPacket(); 00230 pc.printf("check header failed\r\n"); 00231 #endif 00232 00233 SearchStartByte(); 00234 } 00235 else 00236 { 00237 /* check data length */ 00238 if(rxBuff > ((uint8_t*)&hostInterface_rxPacket + gHostInterface_headerSize + hostInterface_rxPacket.length)) 00239 { 00240 /* check trailer byte */ 00241 if(gHostInterface_trailerByte != hostInterface_rxPacket.data[hostInterface_rxPacket.length]) 00242 { 00243 #if defined (LIB_DEBUG) 00244 DebugPrintRxPacket(); 00245 pc.printf("trailer byte failed\r\n"); 00246 #endif 00247 00248 SearchStartByte(); 00249 } 00250 else 00251 { 00252 00253 #if defined (gHostInterface_RxConfirmationEnable) 00254 if(hostInterface_rxPacket.type == packetType_OK) 00255 { 00256 confirmReceived = true; 00257 } 00258 #endif 00259 00260 /* send message to main task */ 00261 hostInterface_packet_t *rxPacket = mpool.alloc(); 00262 memcpy(rxPacket, &hostInterface_rxPacket, sizeof(hostInterface_packet_t)); 00263 queue.put(rxPacket); 00264 00265 #if defined (LIB_DEBUG) 00266 DebugPrintRxPacket(); 00267 #endif 00268 /* reset buffer position */ 00269 rxBuff = (uint8_t*)&hostInterface_rxPacket; 00270 } 00271 } 00272 } 00273 } 00274 } 00275 00276 void KW40Z::SearchStartByte() 00277 { 00278 bool found = false; 00279 uint8_t * rdIdx = (uint8_t*)&hostInterface_rxPacket + 1; 00280 00281 while(rdIdx < rxBuff) 00282 { 00283 if(*rdIdx == gHostInterface_startByte1) 00284 { 00285 uint32_t len = rxBuff - rdIdx; 00286 00287 memcpy(&hostInterface_rxPacket,rdIdx,len); 00288 rxBuff -= len; 00289 found = true; 00290 00291 #if defined (LIB_DEBUG) 00292 pc.printf("moving "); 00293 #endif 00294 break; 00295 } 00296 rdIdx++; 00297 } 00298 00299 if(!found) 00300 { 00301 /* reset buffer position */ 00302 rxBuff = (uint8_t*)&hostInterface_rxPacket; 00303 } 00304 00305 #if defined (LIB_DEBUG) 00306 pc.printf("search done\r\n"); 00307 DebugPrintRxPacket(); 00308 #endif 00309 } 00310 00311 void KW40Z::ProcessReceivedPacket(hostInterface_packet_t * rxPacket) 00312 { 00313 #if defined (LIB_DEBUG) 00314 pc.printf("packet found %d\r\n", rxPacket->type); 00315 #endif 00316 00317 #ifdef gHostInterface_TxConfirmationEnable 00318 // acknowledge the packet reception 00319 if ( 1 == ( rxPacket->start2 & 0x01 ) ) 00320 { 00321 SendPacketOK(); 00322 } 00323 #endif 00324 00325 switch(rxPacket->type) 00326 { 00327 /* button presses */ 00328 case packetType_pressUp: 00329 if(buttonUpCb != NULL) buttonUpCb(); 00330 break; 00331 00332 case packetType_pressDown: 00333 if(buttonDownCb != NULL) buttonDownCb(); 00334 break; 00335 00336 case packetType_pressLeft: 00337 if(buttonLeftCb != NULL) buttonLeftCb(); 00338 break; 00339 00340 case packetType_pressRight: 00341 if(buttonRightCb != NULL) buttonRightCb(); 00342 break; 00343 00344 case packetType_slide: 00345 if(buttonSlideCb != NULL) buttonSlideCb(); 00346 break; 00347 00348 /* Alert Service */ 00349 case packetType_alertIn: 00350 if(alertCb != NULL) alertCb(&rxPacket->data[0], rxPacket->length); 00351 break; 00352 00353 /* Passkey for pairing received */ 00354 case packetType_passDisplay: 00355 if(passkeyCb != NULL) 00356 { 00357 memcpy((uint8_t *)&bondPassKey,&rxPacket->data[0], 3); 00358 passkeyCb(); 00359 } 00360 break; 00361 00362 /* OTAP messages */ 00363 case packetType_otapCompleted: 00364 case packetType_otapFailed: 00365 break; 00366 00367 /* TSI Status */ 00368 case packetType_buttonsGroupSendActive: 00369 activeTsiGroup = rxPacket->data[0]; 00370 break; 00371 00372 /* Advertisement Mode Info */ 00373 case packetType_advModeSend: 00374 advertisementMode = rxPacket->data[0]; 00375 break; 00376 00377 /* Link State */ 00378 case packetType_linkStateSend: 00379 linkState = rxPacket->data[0]; 00380 break; 00381 00382 /* ANCS Service Notification Received */ 00383 case packetType_notification: 00384 if(notificationsCb != NULL) notificationsCb(rxPacket->data[0], rxPacket->data[1]); 00385 break; 00386 00387 /* Build version */ 00388 case packetType_buildVersion: 00389 kw40_version.ver_patchNumber = rxPacket->data[2]; 00390 kw40_version.ver_minorNumber = rxPacket->data[1]; 00391 kw40_version.ver_majorNumber = rxPacket->data[0]; 00392 break; 00393 00394 case packetType_OK: 00395 /* do nothing, the flag is set in the RxTask */ 00396 break; 00397 00398 default: 00399 break; 00400 } 00401 } 00402 00403 void KW40Z::SendBatteryLevel(uint8_t percentage) 00404 { 00405 hostInterface_packet_t txPacket = {0}; 00406 00407 txPacket.start1 = gHostInterface_startByte1; 00408 txPacket.start2 = gHostInterface_startByte2; 00409 txPacket.type = packetType_batteryLevel; 00410 txPacket.length = 1; 00411 txPacket.data[0] = percentage; 00412 txPacket.data[1] = gHostInterface_trailerByte; 00413 00414 SendPacket(&txPacket, true); 00415 } 00416 00417 void KW40Z::SendAccel(uint8_t x, uint8_t y, uint8_t z) 00418 { 00419 hostInterface_packet_t txPacket = {0}; 00420 00421 txPacket.start1 = gHostInterface_startByte1; 00422 txPacket.start2 = gHostInterface_startByte2; 00423 txPacket.type = packetType_accel; 00424 txPacket.length = 3; 00425 txPacket.data[0] = x; 00426 txPacket.data[1] = y; 00427 txPacket.data[2] = z; 00428 txPacket.data[3] = gHostInterface_trailerByte; 00429 00430 SendPacket(&txPacket, true); 00431 } 00432 00433 void KW40Z::SendGyro(uint8_t x, uint8_t y, uint8_t z) 00434 { 00435 hostInterface_packet_t txPacket = {0}; 00436 00437 txPacket.start1 = gHostInterface_startByte1; 00438 txPacket.start2 = gHostInterface_startByte2; 00439 txPacket.type = packetType_gyro; 00440 txPacket.length = 3; 00441 txPacket.data[0] = x; 00442 txPacket.data[1] = y; 00443 txPacket.data[2] = z; 00444 txPacket.data[3] = gHostInterface_trailerByte; 00445 00446 SendPacket(&txPacket, true); 00447 } 00448 00449 void KW40Z::SendMag(uint8_t x, uint8_t y, uint8_t z) 00450 { 00451 hostInterface_packet_t txPacket = {0}; 00452 00453 txPacket.start1 = gHostInterface_startByte1; 00454 txPacket.start2 = gHostInterface_startByte2; 00455 txPacket.type = packetType_magnet; 00456 txPacket.length = 3; 00457 txPacket.data[0] = x; 00458 txPacket.data[1] = y; 00459 txPacket.data[2] = z; 00460 txPacket.data[3] = gHostInterface_trailerByte; 00461 00462 SendPacket(&txPacket, true); 00463 } 00464 00465 void KW40Z::SendAmbientLight(uint8_t percentage) 00466 { 00467 hostInterface_packet_t txPacket = {0}; 00468 00469 txPacket.start1 = gHostInterface_startByte1; 00470 txPacket.start2 = gHostInterface_startByte2; 00471 txPacket.type = packetType_ambiLight; 00472 txPacket.length = 1; 00473 txPacket.data[0] = percentage; 00474 txPacket.data[1] = gHostInterface_trailerByte; 00475 00476 SendPacket(&txPacket, true); 00477 } 00478 00479 void KW40Z::SendTemperature(uint16_t celsius) 00480 { 00481 hostInterface_packet_t txPacket = {0}; 00482 00483 txPacket.start1 = gHostInterface_startByte1; 00484 txPacket.start2 = gHostInterface_startByte2; 00485 txPacket.type = packetType_temperature; 00486 txPacket.length = 2; 00487 memcpy(&txPacket.data[0],(uint8_t*)&celsius,txPacket.length); 00488 txPacket.data[2] = gHostInterface_trailerByte; 00489 00490 SendPacket(&txPacket, true); 00491 } 00492 00493 void KW40Z::SendHumidity(uint16_t percentage) 00494 { 00495 hostInterface_packet_t txPacket = {0}; 00496 00497 txPacket.start1 = gHostInterface_startByte1; 00498 txPacket.start2 = gHostInterface_startByte2; 00499 txPacket.type = packetType_humidity; 00500 txPacket.length = 2; 00501 memcpy(&txPacket.data[0],(uint8_t*)&percentage,txPacket.length); 00502 txPacket.data[2] = gHostInterface_trailerByte; 00503 00504 SendPacket(&txPacket, true); 00505 } 00506 00507 //send iBreathe Data 00508 void KW40Z::SendiBreathe(uint16_t ppm) 00509 { 00510 hostInterface_packet_t txPacket = {0}; 00511 00512 txPacket.start1 = gHostInterface_startByte1; 00513 txPacket.start2 = gHostInterface_startByte2; 00514 txPacket.type = packetType_alc; 00515 txPacket.length = 2; 00516 memcpy(&txPacket.data[0],(uint8_t*)&ppm,txPacket.length); 00517 txPacket.data[2] = gHostInterface_trailerByte; 00518 00519 SendPacket(&txPacket, true); 00520 } 00521 00522 void KW40Z::SendPressure(uint16_t pascal) 00523 { 00524 hostInterface_packet_t txPacket = {0}; 00525 00526 txPacket.start1 = gHostInterface_startByte1; 00527 txPacket.start2 = gHostInterface_startByte2; 00528 txPacket.type = packetType_pressure; 00529 txPacket.length = 2; 00530 memcpy(&txPacket.data[0],(uint8_t*)&pascal,txPacket.length); 00531 txPacket.data[2] = gHostInterface_trailerByte; 00532 00533 SendPacket(&txPacket, true); 00534 } 00535 00536 //void KW40Z::SendHeartRate(uint8_t rate) 00537 //{ 00538 // hostInterface_packet_t txPacket = {0}; 00539 // 00540 // txPacket.start1 = gHostInterface_startByte1; 00541 // txPacket.start2 = gHostInterface_startByte2; 00542 // txPacket.type = packetType_steps; 00543 // txPacket.length = 1; 00544 // txPacket.data[0] = rate; 00545 // txPacket.data[1] = gHostInterface_trailerByte; 00546 00547 // SendPacket(&txPacket, true); 00548 //} 00549 00550 //void KW40Z::SendSteps(uint16_t steps) 00551 //{ 00552 // hostInterface_packet_t txPacket = {0}; 00553 // 00554 // txPacket.start1 = gHostInterface_startByte1; 00555 // txPacket.start2 = gHostInterface_startByte2; 00556 // txPacket.type = packetType_steps; 00557 // txPacket.length = 2; 00558 // memcpy(&txPacket.data[0],(uint8_t*)&steps,txPacket.length); 00559 // txPacket.data[2] = gHostInterface_trailerByte; 00560 // 00561 // SendPacket(&txPacket, true); 00562 //} 00563 00564 void KW40Z::SendCalories(uint16_t calories) 00565 { 00566 hostInterface_packet_t txPacket = {0}; 00567 00568 txPacket.start1 = gHostInterface_startByte1; 00569 txPacket.start2 = gHostInterface_startByte2; 00570 txPacket.type = packetType_calories; 00571 txPacket.length = 2; 00572 memcpy(&txPacket.data[0],(uint8_t*)&calories,txPacket.length); 00573 txPacket.data[2] = gHostInterface_trailerByte; 00574 00575 SendPacket(&txPacket, true); 00576 } 00577 00578 void KW40Z::SendAlert(uint8_t *pData, uint8_t length) 00579 { 00580 hostInterface_packet_t txPacket = {0}; 00581 00582 txPacket.start1 = gHostInterface_startByte1; 00583 txPacket.start2 = gHostInterface_startByte2; 00584 txPacket.type = packetType_alertOut; 00585 txPacket.length = length; 00586 memcpy(&txPacket.data[0],pData,length); 00587 txPacket.data[length] = gHostInterface_trailerByte; 00588 00589 SendPacket(&txPacket, true); 00590 } 00591 00592 void KW40Z::ToggleTsiGroup(void) 00593 { 00594 hostInterface_packet_t txPacket = {0}; 00595 00596 txPacket.start1 = gHostInterface_startByte1; 00597 txPacket.start2 = gHostInterface_startByte2; 00598 txPacket.type = packetType_buttonsGroupToggleActive; 00599 txPacket.length = 0; 00600 txPacket.data[0] = gHostInterface_trailerByte; 00601 00602 SendPacket(&txPacket, true); 00603 } 00604 00605 void KW40Z::ToggleAdvertisementMode(void) 00606 { 00607 hostInterface_packet_t txPacket = {0}; 00608 00609 txPacket.start1 = gHostInterface_startByte1; 00610 txPacket.start2 = gHostInterface_startByte2; 00611 txPacket.type = packetType_advModeToggle; 00612 txPacket.length = 0; 00613 txPacket.data[0] = gHostInterface_trailerByte; 00614 00615 SendPacket(&txPacket, true); 00616 } 00617 00618 void KW40Z::SendSetApplicationMode(gui_current_app_t mode) 00619 { 00620 hostInterface_packet_t txPacket = {0}; 00621 00622 txPacket.start1 = gHostInterface_startByte1; 00623 txPacket.start2 = gHostInterface_startByte2; 00624 txPacket.type = packetType_appMode; 00625 txPacket.length = 1; 00626 txPacket.data[0] = (uint8_t)mode; 00627 txPacket.data[1] = gHostInterface_trailerByte; 00628 00629 SendPacket(&txPacket, true); 00630 } 00631 00632 void KW40Z::SendGetActiveTsiGroup(void) 00633 { 00634 hostInterface_packet_t txPacket = {0}; 00635 00636 txPacket.start1 = gHostInterface_startByte1; 00637 txPacket.start2 = gHostInterface_startByte2; 00638 txPacket.type = packetType_buttonsGroupGetActive; 00639 txPacket.length = 0; 00640 txPacket.data[0] = gHostInterface_trailerByte; 00641 00642 SendPacket(&txPacket, false); 00643 } 00644 00645 void KW40Z::SendGetAdvertisementMode(void) 00646 { 00647 hostInterface_packet_t txPacket = {0}; 00648 00649 txPacket.start1 = gHostInterface_startByte1; 00650 txPacket.start2 = gHostInterface_startByte2; 00651 txPacket.type = packetType_advModeGet; 00652 txPacket.length = 0; 00653 txPacket.data[0] = gHostInterface_trailerByte; 00654 00655 SendPacket(&txPacket, false); 00656 } 00657 00658 void KW40Z::SendGetLinkState(void) 00659 { 00660 hostInterface_packet_t txPacket = {0}; 00661 00662 txPacket.start1 = gHostInterface_startByte1; 00663 txPacket.start2 = gHostInterface_startByte2; 00664 txPacket.type = packetType_linkStateGet; 00665 txPacket.length = 0; 00666 txPacket.data[0] = gHostInterface_trailerByte; 00667 00668 SendPacket(&txPacket, false); 00669 } 00670 00671 void KW40Z::SendGetVersion(void) 00672 { 00673 hostInterface_packet_t txPacket = {0}; 00674 00675 txPacket.start1 = gHostInterface_startByte1; 00676 txPacket.start2 = gHostInterface_startByte2; 00677 txPacket.type = packetType_buildVersion; 00678 txPacket.length = 3; 00679 txPacket.data[0] = HEXIWEAR_VERSION_MAJOR; 00680 txPacket.data[1] = HEXIWEAR_VERSION_MINOR; 00681 txPacket.data[2] = HEXIWEAR_VERSION_PATCH; 00682 txPacket.data[3] = gHostInterface_trailerByte; 00683 00684 SendPacket(&txPacket, false); 00685 } 00686 00687 void KW40Z::SendPacketOK(void) 00688 { 00689 hostInterface_packet_t txPacket = {0}; 00690 00691 txPacket.start1 = gHostInterface_startByte1; 00692 txPacket.start2 = gHostInterface_startByte2; 00693 txPacket.type = packetType_OK; 00694 txPacket.length = 0; 00695 txPacket.data[0] = gHostInterface_trailerByte; 00696 00697 SendPacket(&txPacket, false); 00698 } 00699 00700 uint8_t KW40Z::GetAdvertisementMode(void) 00701 { 00702 return advertisementMode; 00703 } 00704 00705 uint32_t KW40Z::GetPassKey(void) 00706 { 00707 return bondPassKey; 00708 } 00709 00710 uint8_t KW40Z::GetLinkState(void) 00711 { 00712 return linkState; 00713 } 00714 00715 hexiwear_version_t KW40Z::GetVersion(void) 00716 { 00717 return kw40_version; 00718 } 00719 00720 uint8_t KW40Z::GetTsiGroup(void) 00721 { 00722 return activeTsiGroup; 00723 } 00724 00725
Generated on Tue Jul 19 2022 07:05:31 by
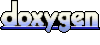