private Library copy of TextLCD
Dependents: eBible ej_RSSfeeder erg y_HM55B ... more
TextLCD.cpp
00001 /* mbed TextLCD Library, for a 4-bit LCD based on HD44780 00002 * Copyright (c) 2007-2010, sford, http://mbed.org 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 /* 00024 * Comment: Added support for LCD40x2 and LCD8x2 00025 * Name: davervw 00026 * Date: 02/26/2011 00027 */ 00028 00029 #include "TextLCD.h" 00030 #include "mbed.h" 00031 00032 TextLCD::TextLCD(PinName rs, PinName e, PinName d4, PinName d5, 00033 PinName d6, PinName d7, LCDType type) : _rs(rs), 00034 _e(e), _d(d4, d5, d6, d7), 00035 _type(type) { 00036 00037 _e = 1; 00038 _rs = 0; // command mode 00039 00040 wait(0.015); // Wait 15ms to ensure powered up 00041 00042 // send "Display Settings" 3 times (Only top nibble of 0x30 as we've got 4-bit bus) 00043 for (int i=0; i<3; i++) { 00044 writeByte(0x3); 00045 wait(0.00164); // this command takes 1.64ms, so wait for it 00046 } 00047 writeByte(0x2); // 4-bit mode 00048 wait(0.000040f); // most instructions take 40us 00049 00050 writeCommand(0x28); // Function set 001 BW N F - - 00051 writeCommand(0x0C); 00052 writeCommand(0x6); // Cursor Direction and Display Shift : 0000 01 CD S (CD 0-left, 1-right S(hift) 0-no, 1-yes 00053 cls(); 00054 } 00055 00056 void TextLCD::character(int column, int row, int c) { 00057 int a = address(column, row); 00058 writeCommand(a); 00059 writeData(c); 00060 } 00061 00062 void TextLCD::cls() { 00063 writeCommand(0x01); // cls, and set cursor to 0 00064 wait(0.00164f); // This command takes 1.64 ms 00065 locate(0, 0); 00066 } 00067 00068 void TextLCD::locate(int column, int row) { 00069 _column = column; 00070 _row = row; 00071 } 00072 00073 int TextLCD::_putc(int value) { 00074 if (value == '\n') { 00075 _column = 0; 00076 _row++; 00077 if (_row >= rows()) { 00078 _row = 0; 00079 } 00080 } else { 00081 character(_column, _row, value); 00082 _column++; 00083 if (_column >= columns()) { 00084 _column = 0; 00085 _row++; 00086 if (_row >= rows()) { 00087 _row = 0; 00088 } 00089 } 00090 } 00091 return value; 00092 } 00093 00094 int TextLCD::_getc() { 00095 return -1; 00096 } 00097 00098 void TextLCD::writeByte(int value) { 00099 _d = value >> 4; 00100 wait(0.000040f); // most instructions take 40us 00101 _e = 0; 00102 wait(0.000040f); 00103 _e = 1; 00104 _d = value >> 0; 00105 wait(0.000040f); 00106 _e = 0; 00107 wait(0.000040f); // most instructions take 40us 00108 _e = 1; 00109 } 00110 00111 void TextLCD::writeCommand(int command) { 00112 _rs = 0; 00113 writeByte(command); 00114 } 00115 00116 void TextLCD::writeData(int data) { 00117 _rs = 1; 00118 writeByte(data); 00119 } 00120 00121 int TextLCD::address(int column, int row) { 00122 switch (_type) { 00123 case LCD20x4: 00124 switch (row) { 00125 case 0: 00126 return 0x80 + column; 00127 case 1: 00128 return 0xc0 + column; 00129 case 2: 00130 return 0x94 + column; 00131 case 3: 00132 return 0xd4 + column; 00133 } 00134 case LCD16x2B: 00135 return 0x80 + (row * 40) + column; 00136 case LCD16x2: 00137 case LCD40x2: 00138 case LCD20x2: 00139 case LCD8x2: 00140 default: 00141 return 0x80 + (row * 0x40) + column; 00142 } 00143 } 00144 00145 int TextLCD::columns() { 00146 switch (_type) { 00147 case LCD20x4: 00148 case LCD20x2: 00149 return 20; 00150 case LCD40x2: 00151 return 40; 00152 case LCD8x2: 00153 return 8; 00154 case LCD16x2: 00155 case LCD16x2B: 00156 default: 00157 return 16; 00158 } 00159 } 00160 00161 int TextLCD::rows() { 00162 switch (_type) { 00163 case LCD20x4: 00164 return 4; 00165 case LCD8x2: 00166 case LCD16x2: 00167 case LCD16x2B: 00168 case LCD20x2: 00169 case LCD40x2: 00170 default: 00171 return 2; 00172 } 00173 }
Generated on Tue Jul 12 2022 15:30:52 by
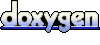