
Bus comms (external protocol) with xbee code for end device
Dependencies: C12832_lcd mbed xbee_lib
main.cpp
00001 #include "mbed.h" 00002 #include "xbee.h" // Include for xbee code 00003 #include "C12832_lcd.h" // Include for LCD code 00004 #include <stdio.h> //Include for sprintf 00005 00006 xbee xbee1(p9,p10,p30); //Initalise xbee_lib varName(rx,tx,reset) 00007 DigitalOut rst1(p30); 00008 Serial pc(USBTX, USBRX); //Initalise PC serial comms 00009 C12832_LCD lcd; //Initialize LCD Screen 00010 00011 //Initialize the joystick on the appBoard as a Bus 00012 BusIn joy(p15,p12,p13,p16); 00013 //Set the button as a Digital In 00014 DigitalIn fire(p14); 00015 00016 int main() 00017 { 00018 // reset the xbees (at least 200ns) 00019 rst1 = 0; 00020 wait_ms(1); 00021 rst1 = 1; 00022 wait_ms(1); 00023 //Setup LCD screen 00024 lcd.cls(); 00025 lcd.locate(0,1); 00026 00027 char sendData[1]; //Buffer to send value of bus 00028 00029 while(1) { 00030 00031 //Digital pin to check for button press 00032 int yes = 0; 00033 00034 //If pressed send 9 00035 if(fire) { 00036 yes = 1; 00037 sprintf (sendData, "%d", 9); 00038 } 00039 //Else send value of the Bus 00040 else{ 00041 sprintf (sendData, "%d", joy.read()); 00042 } 00043 00044 xbee1.SendData(sendData); //Send data to XBee 00045 00046 //Local of value sent 00047 lcd.printf("Value = %s \n", sendData); 00048 wait(0.1); 00049 00050 00051 00052 } 00053 }
Generated on Sat Jul 16 2022 01:46:27 by
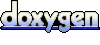