Free (GPLv2) TCP/IP stack developed by TASS Belgium
Dependents: lpc1768-picotcp-demo ZeroMQ_PicoTCP_Publisher_demo TCPSocket_HelloWorld_PicoTCP Pico_TCP_UDP_Test ... more
pico_socket.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012-2015 Altran Intelligent Systems. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 *********************************************************************/ 00006 #ifndef INCLUDE_PICO_SOCKET 00007 #define INCLUDE_PICO_SOCKET 00008 #include "pico_queue.h" 00009 #include "pico_addressing.h" 00010 #include "pico_config.h" 00011 #include "pico_protocol.h" 00012 #include "pico_tree.h" 00013 00014 #ifdef __linux__ 00015 #define PICO_DEFAULT_SOCKETQ (16 * 1024) /* Linux host, so we want full throttle */ 00016 #else 00017 #define PICO_DEFAULT_SOCKETQ (6 * 1024) /* seems like an acceptable default for small embedded systems */ 00018 #endif 00019 00020 #define PICO_SHUT_RD 1 00021 #define PICO_SHUT_WR 2 00022 #define PICO_SHUT_RDWR 3 00023 00024 #ifdef PICO_SUPPORT_IPV4 00025 # define IS_SOCK_IPV4(s) ((s->net == &pico_proto_ipv4)) 00026 #else 00027 # define IS_SOCK_IPV4(s) (0) 00028 #endif 00029 00030 #ifdef PICO_SUPPORT_IPV6 00031 # define IS_SOCK_IPV6(s) ((s->net == &pico_proto_ipv6)) 00032 #else 00033 # define IS_SOCK_IPV6(s) (0) 00034 #endif 00035 00036 00037 struct pico_sockport 00038 { 00039 struct pico_tree socks; /* how you make the connection ? */ 00040 uint16_t number; 00041 uint16_t proto; 00042 }; 00043 00044 00045 struct pico_socket { 00046 struct pico_protocol *proto; 00047 struct pico_protocol *net; 00048 00049 union pico_address local_addr; 00050 union pico_address remote_addr; 00051 00052 uint16_t local_port; 00053 uint16_t remote_port; 00054 00055 struct pico_queue q_in; 00056 struct pico_queue q_out; 00057 00058 void (*wakeup)(uint16_t ev, struct pico_socket *s); 00059 00060 00061 #ifdef PICO_SUPPORT_TCP 00062 /* For the TCP backlog queue */ 00063 struct pico_socket *backlog; 00064 struct pico_socket *next; 00065 struct pico_socket *parent; 00066 uint16_t max_backlog; 00067 uint16_t number_of_pending_conn; 00068 #endif 00069 #ifdef PICO_SUPPORT_MCAST 00070 struct pico_tree *MCASTListen; 00071 #ifdef PICO_SUPPORT_IPV6 00072 struct pico_tree *MCASTListen_ipv6; 00073 #endif 00074 #endif 00075 uint16_t ev_pending; 00076 00077 struct pico_device *dev; 00078 00079 /* Private field. */ 00080 int id; 00081 uint16_t state; 00082 uint16_t opt_flags; 00083 pico_time timestamp; 00084 void *priv; 00085 }; 00086 00087 struct pico_remote_endpoint { 00088 union pico_address remote_addr; 00089 uint16_t remote_port; 00090 }; 00091 00092 00093 struct pico_ip_mreq { 00094 union pico_address mcast_group_addr; 00095 union pico_address mcast_link_addr; 00096 }; 00097 struct pico_ip_mreq_source { 00098 union pico_address mcast_group_addr; 00099 union pico_address mcast_source_addr; 00100 union pico_address mcast_link_addr; 00101 }; 00102 00103 00104 #define PICO_SOCKET_STATE_UNDEFINED 0x0000u 00105 #define PICO_SOCKET_STATE_SHUT_LOCAL 0x0001u 00106 #define PICO_SOCKET_STATE_SHUT_REMOTE 0x0002u 00107 #define PICO_SOCKET_STATE_BOUND 0x0004u 00108 #define PICO_SOCKET_STATE_CONNECTED 0x0008u 00109 #define PICO_SOCKET_STATE_CLOSING 0x0010u 00110 #define PICO_SOCKET_STATE_CLOSED 0x0020u 00111 00112 # define PICO_SOCKET_STATE_TCP 0xFF00u 00113 # define PICO_SOCKET_STATE_TCP_UNDEF 0x00FFu 00114 # define PICO_SOCKET_STATE_TCP_CLOSED 0x0100u 00115 # define PICO_SOCKET_STATE_TCP_LISTEN 0x0200u 00116 # define PICO_SOCKET_STATE_TCP_SYN_SENT 0x0300u 00117 # define PICO_SOCKET_STATE_TCP_SYN_RECV 0x0400u 00118 # define PICO_SOCKET_STATE_TCP_ESTABLISHED 0x0500u 00119 # define PICO_SOCKET_STATE_TCP_CLOSE_WAIT 0x0600u 00120 # define PICO_SOCKET_STATE_TCP_LAST_ACK 0x0700u 00121 # define PICO_SOCKET_STATE_TCP_FIN_WAIT1 0x0800u 00122 # define PICO_SOCKET_STATE_TCP_FIN_WAIT2 0x0900u 00123 # define PICO_SOCKET_STATE_TCP_CLOSING 0x0a00u 00124 # define PICO_SOCKET_STATE_TCP_TIME_WAIT 0x0b00u 00125 # define PICO_SOCKET_STATE_TCP_ARRAYSIZ 0x0cu 00126 00127 00128 /* Socket options */ 00129 # define PICO_TCP_NODELAY 1 00130 # define PICO_SOCKET_OPT_TCPNODELAY 0x0000u 00131 00132 # define PICO_IP_MULTICAST_EXCLUDE 0 00133 # define PICO_IP_MULTICAST_INCLUDE 1 00134 # define PICO_IP_MULTICAST_IF 32 00135 # define PICO_IP_MULTICAST_TTL 33 00136 # define PICO_IP_MULTICAST_LOOP 34 00137 # define PICO_IP_ADD_MEMBERSHIP 35 00138 # define PICO_IP_DROP_MEMBERSHIP 36 00139 # define PICO_IP_UNBLOCK_SOURCE 37 00140 # define PICO_IP_BLOCK_SOURCE 38 00141 # define PICO_IP_ADD_SOURCE_MEMBERSHIP 39 00142 # define PICO_IP_DROP_SOURCE_MEMBERSHIP 40 00143 00144 # define PICO_SOCKET_OPT_MULTICAST_LOOP 1 00145 # define PICO_SOCKET_OPT_KEEPIDLE 4 00146 # define PICO_SOCKET_OPT_KEEPINTVL 5 00147 # define PICO_SOCKET_OPT_KEEPCNT 6 00148 00149 #define PICO_SOCKET_OPT_LINGER 13 00150 00151 # define PICO_SOCKET_OPT_RCVBUF 52 00152 # define PICO_SOCKET_OPT_SNDBUF 53 00153 00154 00155 /* Constants */ 00156 # define PICO_IP_DEFAULT_MULTICAST_TTL 1 00157 # define PICO_IP_DEFAULT_MULTICAST_LOOP 1 00158 00159 #define PICO_SOCKET_TIMEOUT 5000u /* 5 seconds */ 00160 #define PICO_SOCKET_LINGER_TIMEOUT 3000u /* 3 seconds */ 00161 #define PICO_SOCKET_BOUND_TIMEOUT 30000u /* 30 seconds */ 00162 00163 #define PICO_SOCKET_SHUTDOWN_WRITE 0x01u 00164 #define PICO_SOCKET_SHUTDOWN_READ 0x02u 00165 #define TCPSTATE(s) ((s)->state & PICO_SOCKET_STATE_TCP) 00166 00167 #define PICO_SOCK_EV_RD 1u 00168 #define PICO_SOCK_EV_WR 2u 00169 #define PICO_SOCK_EV_CONN 4u 00170 #define PICO_SOCK_EV_CLOSE 8u 00171 #define PICO_SOCK_EV_FIN 0x10u 00172 #define PICO_SOCK_EV_ERR 0x80u 00173 00174 struct pico_msginfo { 00175 struct pico_device *dev; 00176 uint8_t ttl; 00177 uint8_t tos; 00178 }; 00179 00180 struct pico_socket *pico_socket_open(uint16_t net, uint16_t proto, void (*wakeup)(uint16_t ev, struct pico_socket *s)); 00181 00182 int pico_socket_read(struct pico_socket *s, void *buf, int len); 00183 int pico_socket_write(struct pico_socket *s, const void *buf, int len); 00184 00185 int pico_socket_sendto(struct pico_socket *s, const void *buf, int len, void *dst, uint16_t remote_port); 00186 int pico_socket_sendto_extended(struct pico_socket *s, const void *buf, const int len, 00187 void *dst, uint16_t remote_port, struct pico_msginfo *msginfo); 00188 00189 int pico_socket_recvfrom(struct pico_socket *s, void *buf, int len, void *orig, uint16_t *local_port); 00190 int pico_socket_recvfrom_extended(struct pico_socket *s, void *buf, int len, void *orig, 00191 uint16_t *remote_port, struct pico_msginfo *msginfo); 00192 00193 int pico_socket_send(struct pico_socket *s, const void *buf, int len); 00194 int pico_socket_recv(struct pico_socket *s, void *buf, int len); 00195 00196 int pico_socket_bind(struct pico_socket *s, void *local_addr, uint16_t *port); 00197 int pico_socket_getname(struct pico_socket *s, void *local_addr, uint16_t *port, uint16_t *proto); 00198 int pico_socket_getpeername(struct pico_socket *s, void *remote_addr, uint16_t *port, uint16_t *proto); 00199 00200 int pico_socket_connect(struct pico_socket *s, const void *srv_addr, uint16_t remote_port); 00201 int pico_socket_listen(struct pico_socket *s, const int backlog); 00202 struct pico_socket *pico_socket_accept(struct pico_socket *s, void *orig, uint16_t *port); 00203 int8_t pico_socket_del(struct pico_socket *s); 00204 00205 int pico_socket_setoption(struct pico_socket *s, int option, void *value); 00206 int pico_socket_getoption(struct pico_socket *s, int option, void *value); 00207 00208 int pico_socket_shutdown(struct pico_socket *s, int mode); 00209 int pico_socket_close(struct pico_socket *s); 00210 00211 struct pico_frame *pico_socket_frame_alloc(struct pico_socket *s, uint16_t len); 00212 00213 #ifdef PICO_SUPPORT_IPV4 00214 # define is_sock_ipv4(x) (x->net == &pico_proto_ipv4) 00215 #else 00216 # define is_sock_ipv4(x) (0) 00217 #endif 00218 00219 #ifdef PICO_SUPPORT_IPV6 00220 # define is_sock_ipv6(x) (x->net == &pico_proto_ipv6) 00221 #else 00222 # define is_sock_ipv6(x) (0) 00223 #endif 00224 00225 #ifdef PICO_SUPPORT_UDP 00226 # define is_sock_udp(x) (x->proto == &pico_proto_udp) 00227 #else 00228 # define is_sock_udp(x) (0) 00229 #endif 00230 00231 #ifdef PICO_SUPPORT_TCP 00232 # define is_sock_tcp(x) (x->proto == &pico_proto_tcp) 00233 #else 00234 # define is_sock_tcp(x) (0) 00235 #endif 00236 00237 /* Interface towards transport protocol */ 00238 int pico_transport_process_in(struct pico_protocol *self, struct pico_frame *f); 00239 struct pico_socket *pico_socket_clone(struct pico_socket *facsimile); 00240 int8_t pico_socket_add(struct pico_socket *s); 00241 int pico_transport_error(struct pico_frame *f, uint8_t proto, int code); 00242 00243 /* Socket loop */ 00244 int pico_sockets_loop(int loop_score); 00245 struct pico_socket*pico_sockets_find(uint16_t local, uint16_t remote); 00246 /* Port check */ 00247 int pico_is_port_free(uint16_t proto, uint16_t port, void *addr, void *net); 00248 00249 struct pico_sockport *pico_get_sockport(uint16_t proto, uint16_t port); 00250 00251 uint32_t pico_socket_get_mss(struct pico_socket *s); 00252 int pico_socket_set_family(struct pico_socket *s, uint16_t family); 00253 00254 int pico_count_sockets(uint8_t proto); 00255 00256 #define PICO_SOCKET_SETOPT_EN(socket, index) (socket->opt_flags |= (1 << index)) 00257 #define PICO_SOCKET_SETOPT_DIS(socket, index) (socket->opt_flags &= (uint16_t) ~(1 << index)) 00258 #define PICO_SOCKET_GETOPT(socket, index) ((socket->opt_flags & (1u << index)) != 0) 00259 00260 00261 #endif
Generated on Tue Jul 12 2022 15:59:22 by
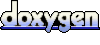