Free (GPLv2) TCP/IP stack developed by TASS Belgium
Dependents: lpc1768-picotcp-demo ZeroMQ_PicoTCP_Publisher_demo TCPSocket_HelloWorld_PicoTCP Pico_TCP_UDP_Test ... more
pico_mbed.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 Do not redistribute without a written permission by the Copyright 00005 holders. 00006 00007 File: pico_mbed.h 00008 Author: Toon Peters 00009 *********************************************************************/ 00010 00011 #ifndef PICO_SUPPORT_MBED 00012 #define PICO_SUPPORT_MBED 00013 #include <stdio.h> 00014 //#include "mbed.h" 00015 //#include "serial_api.h" 00016 00017 //#define TIME_PRESCALE 00018 //#define PICO_MEASURE_STACK 00019 //#define MEMORY_MEASURE 00020 /* 00021 Debug needs initialization: 00022 * void serial_init (serial_t *obj, PinName tx, PinName rx); 00023 * void serial_baud (serial_t *obj, int baudrate); 00024 * void serial_format (serial_t *obj, int data_bits, SerialParity parity, int stop_bits); 00025 */ 00026 00027 #define dbg(...) 00028 00029 #ifdef PICO_MEASURE_STACK 00030 00031 extern int freeStack; 00032 #define STACK_TOTAL_WORDS 1000u 00033 #define STACK_PATTERN (0xC0CAC01A) 00034 00035 void stack_fill_pattern(void *ptr); 00036 void stack_count_free_words(void *ptr); 00037 int stack_get_free_words(void); 00038 #else 00039 #define stack_fill_pattern(...) do{}while(0) 00040 #define stack_count_free_words(...) do{}while(0) 00041 #define stack_get_free_words() (0) 00042 #endif 00043 00044 #ifdef MEMORY_MEASURE // in case, comment out the two defines above me. 00045 extern uint32_t max_mem; 00046 extern uint32_t cur_mem; 00047 00048 static inline void * pico_zalloc(int x) 00049 { 00050 uint32_t *ptr; 00051 if ((cur_mem + x )> (10 * 1024)) 00052 return NULL; 00053 00054 ptr = (uint32_t *)calloc(x + 4, 1); 00055 *ptr = (uint32_t)x; 00056 cur_mem += x; 00057 if (cur_mem > max_mem) { 00058 max_mem = cur_mem; 00059 // printf("max mem: %lu\n", max_mem); 00060 } 00061 return (void*)(ptr + 1); 00062 } 00063 00064 static inline void pico_free(void *x) 00065 { 00066 uint32_t *ptr = (uint32_t*)(((uint8_t *)x) - 4); 00067 cur_mem -= *ptr; 00068 free(ptr); 00069 } 00070 #else 00071 00072 #define pico_zalloc(x) calloc(x, 1) 00073 #define pico_free(x) free(x) 00074 00075 #endif 00076 00077 #define PICO_SUPPORT_MUTEX 00078 extern void *pico_mutex_init(void); 00079 extern void pico_mutex_lock(void*); 00080 extern void pico_mutex_unlock(void*); 00081 extern void pico_mutex_deinit(void*); 00082 00083 extern uint32_t os_time; 00084 extern uint64_t local_time; 00085 extern uint32_t last_os_time; 00086 00087 #ifdef TIME_PRESCALE 00088 extern int32_t prescale_time; 00089 #endif 00090 00091 #define UPDATE_LOCAL_TIME() do {local_time = local_time + ((pico_time)os_time - (pico_time)last_os_time);last_os_time = os_time;} while(0) 00092 00093 static inline uint64_t PICO_TIME(void) 00094 { 00095 UPDATE_LOCAL_TIME(); 00096 #ifdef TIME_PRESCALE 00097 return (prescale_time < 0) ? (uint64_t)(local_time / 1000 << (-prescale_time)) : \ 00098 (uint64_t)(local_time / 1000 >> prescale_time); 00099 #else 00100 return (uint64_t)(local_time / 1000); 00101 #endif 00102 } 00103 00104 static inline uint64_t PICO_TIME_MS(void) 00105 { 00106 UPDATE_LOCAL_TIME(); 00107 #ifdef TIME_PRESCALE 00108 return (prescale_time < 0) ? (uint64_t)(local_time << (-prescale_time)) : \ 00109 (uint64_t)(local_time >> prescale_time); 00110 #else 00111 return (uint64_t)local_time; 00112 #endif 00113 } 00114 00115 static inline void PICO_IDLE(void) 00116 { 00117 // TODO needs implementation 00118 } 00119 /* 00120 static inline void PICO_DEBUG(const char * formatter, ... ) 00121 { 00122 char buffer[256]; 00123 char *ptr; 00124 va_list args; 00125 va_start(args, formatter); 00126 vsnprintf(buffer, 256, formatter, args); 00127 ptr = buffer; 00128 while(*ptr != '\0') 00129 serial_putc(serial_t *obj, (int) (*(ptr++))); 00130 va_end(args); 00131 //TODO implement serial_t 00132 }*/ 00133 00134 #endif
Generated on Tue Jul 12 2022 15:59:22 by
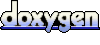