Free (GPLv2) TCP/IP stack developed by TASS Belgium
Dependents: lpc1768-picotcp-demo ZeroMQ_PicoTCP_Publisher_demo TCPSocket_HelloWorld_PicoTCP Pico_TCP_UDP_Test ... more
pico_dev_mbed.cpp
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 Do not redistribute without a written permission by the Copyright 00005 holders. 00006 00007 Authors: Toon Peters, Maxime Vincent 00008 *********************************************************************/ 00009 #include "mbed.h" 00010 extern "C" { 00011 #include "pico_device.h" 00012 #include "pico_dev_mbed.h" 00013 #include "pico_stack.h" 00014 #include "ethernet_api.h" 00015 } 00016 00017 struct pico_device_mbed { 00018 struct pico_device dev; 00019 int bytes_left_in_frame; 00020 }; 00021 00022 #define ETH_MTU 1514 00023 uint8_t buf[ETH_MTU]; 00024 00025 extern "C" { 00026 00027 static int pico_mbed_send(struct pico_device *dev, void *buf, int len) 00028 { 00029 int ret, sent; 00030 00031 if (len > ETH_MTU) 00032 return -1; 00033 00034 /* Write buf content to dev and return amount written */ 00035 ret = ethernet_write((const char *)buf, len); 00036 sent = ethernet_send(); 00037 00038 printf("ETH> sent %d bytes\r\n",ret); 00039 if (len != ret || sent != ret) 00040 return -1; 00041 else 00042 return ret; 00043 } 00044 00045 static int pico_mbed_poll(struct pico_device *dev, int loop_score) 00046 { 00047 int len; 00048 00049 while(loop_score > 0) 00050 { 00051 /* check for new frame(s) */ 00052 len = (int) ethernet_receive(); 00053 00054 /* return if no frame has arrived */ 00055 if (!len) 00056 return loop_score; 00057 00058 /* read and process frame */ 00059 len = ethernet_read((char*)buf, ETH_MTU); 00060 printf("ETH> recv %d bytes: %x:%x\r\n", len, buf[0],buf[1]); 00061 pico_stack_recv(dev, buf, len); 00062 loop_score--; 00063 } 00064 return loop_score; 00065 } 00066 00067 /* Public interface: create/destroy. */ 00068 void pico_mbed_destroy(struct pico_device *dev) 00069 { 00070 ethernet_free(); 00071 pico_device_destroy(dev); 00072 } 00073 00074 struct pico_device *pico_mbed_create(char *name) 00075 { 00076 uint8_t mac[PICO_SIZE_ETH]; 00077 struct pico_device_mbed *mb = (struct pico_device_mbed*) pico_zalloc(sizeof(struct pico_device_mbed)); 00078 00079 if (!mb) 00080 return NULL; 00081 00082 ethernet_address((char *)mac); 00083 printf("ETH> Set MAC address to: %x:%x:%x:%x:%x:%x\r\n", mac[0],mac[1],mac[2],mac[3],mac[4],mac[5]); 00084 00085 if(0 != pico_device_init((struct pico_device *)mb, name, mac)) { 00086 printf ("ETH> Loop init failed.\n"); 00087 //pico_loop_destroy(mb); 00088 return NULL; 00089 } 00090 00091 mb->dev.send = pico_mbed_send; 00092 mb->dev.poll = pico_mbed_poll; 00093 mb->dev.destroy = pico_mbed_destroy; 00094 mb->bytes_left_in_frame = 0; 00095 00096 if(0 != ethernet_init()) { 00097 printf("ETH> Failed to initialize hardware.\r\n"); 00098 pico_device_destroy((struct pico_device *)mb); 00099 return NULL; 00100 } 00101 00102 // future work: make the mac address configurable 00103 00104 printf("ETH> Device %s created.\r\n", mb->dev.name); 00105 00106 return (struct pico_device *)mb; 00107 } 00108 00109 void pico_mbed_get_address(char *mac) 00110 { 00111 ethernet_address(mac); 00112 } 00113 00114 }
Generated on Tue Jul 12 2022 15:59:21 by
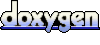