Free (GPLv2) TCP/IP stack developed by TASS Belgium
Dependents: lpc1768-picotcp-demo ZeroMQ_PicoTCP_Publisher_demo TCPSocket_HelloWorld_PicoTCP Pico_TCP_UDP_Test ... more
heap.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012-2015 Altran Intelligent Systems. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 *********************************************************************/ 00006 00007 #define DECLARE_HEAP(type, orderby) \ 00008 struct heap_ ## type { \ 00009 uint32_t size; \ 00010 uint32_t n; \ 00011 type *top; \ 00012 }; \ 00013 typedef struct heap_ ## type heap_ ## type; \ 00014 static inline int heap_insert(struct heap_ ## type *heap, type * el) \ 00015 { \ 00016 uint32_t i; \ 00017 type *newTop; \ 00018 if (++heap->n >= heap->size) { \ 00019 newTop = PICO_ZALLOC((heap->n + 1) * sizeof(type)); \ 00020 if(!newTop) { \ 00021 heap->n--; \ 00022 return -1; \ 00023 } \ 00024 if (heap->top) { \ 00025 memcpy(newTop, heap->top, heap->n * sizeof(type)); \ 00026 PICO_FREE(heap->top); \ 00027 } \ 00028 heap->top = newTop; \ 00029 heap->size++; \ 00030 } \ 00031 if (heap->n == 1) { \ 00032 memcpy(&heap->top[1], el, sizeof(type)); \ 00033 return 0; \ 00034 } \ 00035 for (i = heap->n; ((i > 1) && (heap->top[i / 2].orderby > el->orderby)); i /= 2) { \ 00036 memcpy(&heap->top[i], &heap->top[i / 2], sizeof(type)); \ 00037 } \ 00038 memcpy(&heap->top[i], el, sizeof(type)); \ 00039 return 0; \ 00040 } \ 00041 static inline int heap_peek(struct heap_ ## type *heap, type * first) \ 00042 { \ 00043 type *last; \ 00044 uint32_t i, child; \ 00045 if(heap->n == 0) { \ 00046 return -1; \ 00047 } \ 00048 memcpy(first, &heap->top[1], sizeof(type)); \ 00049 last = &heap->top[heap->n--]; \ 00050 for(i = 1; (i * 2u) <= heap->n; i = child) { \ 00051 child = 2u * i; \ 00052 if ((child != heap->n) && \ 00053 (heap->top[child + 1]).orderby \ 00054 < (heap->top[child]).orderby) \ 00055 child++; \ 00056 if (last->orderby > \ 00057 heap->top[child].orderby) \ 00058 memcpy(&heap->top[i], &heap->top[child], \ 00059 sizeof(type)); \ 00060 else \ 00061 break; \ 00062 } \ 00063 memcpy(&heap->top[i], last, sizeof(type)); \ 00064 return 0; \ 00065 } \ 00066 static inline type *heap_first(heap_ ## type * heap) \ 00067 { \ 00068 if (heap->n == 0) \ 00069 return NULL; \ 00070 return &heap->top[1]; \ 00071 } \ 00072 static inline heap_ ## type *heap_init(void) \ 00073 { \ 00074 heap_ ## type * p = (heap_ ## type *)PICO_ZALLOC(sizeof(heap_ ## type)); \ 00075 return p; \ 00076 } \ 00077 /*static inline void heap_destroy(heap_ ## type * h) \ 00078 { \ 00079 PICO_FREE(h->top); \ 00080 PICO_FREE(h); \ 00081 } \*/ 00082 00083
Generated on Tue Jul 12 2022 15:59:21 by
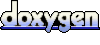