Dependents: playback FTSESpeech i2s_audio_echo i2s_audio_sampler ... more
I2SSlave Class Reference
I2S class defined on the LPC1768 port. More...
#include <I2SSlave.h>
Public Member Functions | |
I2SSlave (PinName tx_sda, PinName tx_ws, PinName clk, PinName rx_sda, PinName rx_ws) | |
Create an I2S object. | |
void | format (int bit, bool mode) |
Set the data transmission format. | |
void | write (int *buffer, int from, int length) |
Write a buffer to the I2S port. | |
void | start (int mode) |
Activate I2S port for data streaming. | |
void | stop (void) |
Deactivate I2S port from data streaming. | |
void | read (void) |
Load receive FIFO data into receiver buffer. | |
void | attach (void(*fptr)(void)) |
Attach a void/void function or void/void static memeber function to an interrupt generated by the I2SxxFIFOs. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void)) |
Attach a nonstatic void/void member function to an interrupt generated by the I2SxxFIFOs. | |
int | status (void) |
Return contents of I2S status register. |
Detailed Description
I2S class defined on the LPC1768 port.
Definition at line 42 of file I2SSlave.h.
Constructor & Destructor Documentation
I2SSlave | ( | PinName | tx_sda, |
PinName | tx_ws, | ||
PinName | clk, | ||
PinName | rx_sda, | ||
PinName | rx_ws | ||
) |
Create an I2S object.
- Parameters:
-
tx_sda Transmitter serial data line tx_ws Transmitter word select line clk Shared transmitter/receiver clock line rx_sda Receiver serial data line rx_ws Receiver word select line
Definition at line 46 of file I2SSlave.cpp.
Member Function Documentation
void attach | ( | void(*)(void) | fptr ) |
Attach a void/void function or void/void static memeber function to an interrupt generated by the I2SxxFIFOs.
- Parameters:
-
function Function to attach
myI2sObject.attach(&myfunction);
OR myI2sObject.attach(&myClass::myStaticMemberFunction);
Definition at line 92 of file I2SSlave.h.
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
Attach a nonstatic void/void member function to an interrupt generated by the I2SxxFIFOs.
- Parameters:
-
tptr Object pointer mptr Member function pointer
e.g. myI2sObject.attach(&myObject, &myClass::myNonstaticMemberFunction);
where myObject is an object of myClass
Definition at line 103 of file I2SSlave.h.
void format | ( | int | bit, |
bool | mode | ||
) |
Set the data transmission format.
- Parameters:
-
bit Set the number of bits per write mode Set STEREO (0) or MONO (1) mode
Definition at line 59 of file I2SSlave.cpp.
void read | ( | void | ) |
Load receive FIFO data into receiver buffer.
Definition at line 133 of file I2SSlave.cpp.
void start | ( | int | mode ) |
Activate I2S port for data streaming.
- Parameters:
-
mode Mode to enable - NONE, TRANSMIT only, RECEIVE only, BOTH Enables tx/rx interrupts
Definition at line 86 of file I2SSlave.cpp.
int status | ( | void | ) |
Return contents of I2S status register.
- Returns:
- Content of I2SSTATE register
bit0: receive/transmit interrupt active bit1: receive/transmit DMA request 1 bit2: receive/transmit DMA request 2 bit[11:8]: receive FIFO level bit[19:16]: transmit FIFO level
Definition at line 147 of file I2SSlave.cpp.
void stop | ( | void | ) |
Deactivate I2S port from data streaming.
Disable all interrupts
Definition at line 122 of file I2SSlave.cpp.
void write | ( | int * | buffer, |
int | from, | ||
int | length | ||
) |
Write a buffer to the I2S port.
- Parameters:
-
buffer Address of buffer to pass to I2S port from Start position in buffer to read from length Length of buffer (MUST not exceed 8 words, each 32 bits long)
Note: sending 8 words to the TXFIFO will trigger an interrupt!
Definition at line 72 of file I2SSlave.cpp.
Generated on Tue Jul 12 2022 18:43:37 by
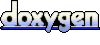