Library for Texas Instruments TLV320AIC23B hi-def audio chip note: requires I2SSlave abstraction library
Dependents: playback FTSESpeech i2s_audio_echo i2s_audio_sampler ... more
TLV320.h
00001 /** 00002 * @author Ioannis Kedros, Daniel Worrall 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2011 mbed 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * Library for Texas instruments TLV320AIC23B library NXP LPC1768 00028 * 00029 */ 00030 00031 #ifndef MBED_TLV320_H 00032 #define MBED_TLV320_H 00033 00034 #include "mbed.h" 00035 #include "I2SSlave.h" 00036 00037 /** TLV320 class, defined on the I2C master bus 00038 * 00039 */ 00040 00041 class TLV320 00042 { 00043 public: 00044 //constructor 00045 /** Create a TLV320 object defined on the I2C port 00046 * 00047 * @param sda Serial data pin (p9 or p28) 00048 * @param scl Serial clock pin (p10 or p27) 00049 * @param addr Object address 00050 */ 00051 TLV320(PinName sda, PinName scl, int addr, PinName tx_sda, PinName tx_ws, PinName clk, PinName rx_sda, PinName rx_ws); 00052 /** Power up/down 00053 * 00054 * @param powerUp 0 = power down, 1 = power up 00055 */ 00056 void power(bool powerUp); 00057 /** Overloaded power() function default = 0x07, record requires 0x02 00058 * 00059 * @param device Call individual devices to power up/down 00060 * Device power 0x00 = On 0x80 = Off 00061 * Clock 0x00 = On 0x40 = Off 00062 * Oscillator 0x00 = On 0x20 = Off 00063 * Outputs 0x00 = On 0x10 = Off 00064 * DAC 0x00 = On 0x08 = Off 00065 * ADC 0x00 = On 0x04 = Off 00066 * Microphone input 0x00 = On 0x02 = Off 00067 * Line input 0x00 = On 0x01 = Off 00068 */ 00069 void power(int device); 00070 /** Set I2S interface bit length and mode 00071 * 00072 * @param length Set bit length to 16, 20, 24 or 32 bits 00073 * @param mode Set STEREO (0), MONO (1) 00074 */ 00075 void format(char length, bool mode); 00076 /** Set sample frequency 00077 * 00078 * @param frequency Sample frequency of data in Hz 00079 * @return Returns an integer 0 = success, -1 = unrecognnised frequency 00080 * 00081 * The TLV320 supports the following frequencies: 8kHz, 8.021kHz, 32kHz, 44.1kHz, 48kHz, 88.2kHz, 96kHz 00082 * Default is 44.1kHz 00083 */ 00084 int frequency(int hz); 00085 /** Reset TLV320 00086 * 00087 */ 00088 void reset(void); 00089 /** Start streaming i.e. enable interrupts 00090 * 00091 * @param mode Enable interrupts for NONE, TRANSMIT only, RECEIVE only, BOTH 00092 */ 00093 void start(int mode); 00094 /** Stop streaming i.e. disable all interrupts 00095 * 00096 */ 00097 void stop(void); 00098 /** Write [length] 32 bit words in buffer to I2S port 00099 * 00100 * @param *buffer Address of buffer to be written 00101 * @param from Start position in buffer to read from 00102 * @param length Number of words to be written (MUST not exceed 4) 00103 */ 00104 void write(int *buffer, int from, int length); 00105 /** Read 4 x (32bit) words into rxBuffer 00106 * 00107 */ 00108 void read(void); 00109 /** Attach a void/void function or void/void static member function to an interrupt generated by the I2SxxFIFOs 00110 * 00111 * @param function Function to attach 00112 * 00113 * e.g. <code> myTlv320Object.attach(&myfunction);</code> 00114 * OR <code> myTlv320Object.attach(&myClass::myStaticMemberFunction);</code> 00115 */ 00116 void attach(void(*fptr)(void)); 00117 /** Attach a nonstatic void/void member function to an interrupt generated by the I2SxxFIFOs 00118 * 00119 * @param tptr Object pointer 00120 * @param mptr Member function pointer 00121 * 00122 * e.g. <code>myTlv320Object.attach(&myObject, &myClass::myNonstaticMemberFunction);</code> where myObject is an object of myClass 00123 */ 00124 template<typename T> 00125 void attach(T *tptr, void(T::*mptr)(void)){ 00126 mI2s_.attach(tptr, mptr); 00127 } 00128 /** Line in volume control i.e. record volume 00129 * 00130 * @param leftVolumeIn Left line-in volume 00131 * @param rightVolumeIn Right line-in volume 00132 * @return Returns 0 for success, -1 if parameters are out of range 00133 * Parameters accept a value, where 0.0 < parameter < 1.0 and where 0.0 maps to -34.5dB 00134 * and 1.0 maps to +12dB (0.74 = 0 dB default). 00135 */ 00136 int inputVolume(float leftVolumeIn, float rightVolumeIn); 00137 /** Headphone out volume control 00138 * 00139 * @param leftVolumeOut Left line-out volume 00140 * @param rightVolumeOut Right line-out volume 00141 * @return Returns 0 for success, -1 if parameters are out of range 00142 * Parameters accept a value, where 0.0 < parameter < 1.0 and where 0.0 maps to -73dB (mute) 00143 * and 1.0 maps to +6dB (0.5 = default) 00144 */ 00145 int outputVolume(float leftVolumeOut, float rightVolumeOut); 00146 /** Analog audio path control 00147 * 00148 * @param bypassVar Route analogue audio direct from line in to headphone out 00149 */ 00150 void bypass(bool bypassVar); 00151 /**Digital audio path control 00152 * 00153 * @param softMute Mute output 00154 */ 00155 void mute(bool softMute); 00156 //Receive buffer 00157 00158 int *rxBuffer; 00159 00160 protected: 00161 char cmd[2]; //the address and command for TLV320 internal registers 00162 int mAddr; //register write address 00163 private: 00164 I2C mI2c_; //MUST use the I2C port 00165 I2SSlave mI2s_; 00166 Ticker I2sTick; 00167 void io(void); 00168 /** Sample rate control 00169 * 00170 * @param rate Set the sampling rate as per datasheet section 3.3.2 00171 * @param clockIn Set the clock in divider MCLK, MCLK_DIV2 00172 * @param clockMode Set clock mode CLOCK_NORMAL, CLOCK_USB 00173 */ 00174 void setSampleRate_(char rate, bool clockIn, bool mode, bool bOSR); 00175 /** Digital interface activation 00176 * 00177 */ 00178 void activateDigitalInterface_(void); 00179 /** Digital interface deactivation 00180 * 00181 */ 00182 void deactivateDigitalInterface_(void); 00183 00184 //TLV320AIC23B register addresses as defined in the TLV320AIC23B datasheet 00185 #define LEFT_LINE_INPUT_CHANNEL_VOLUME_CONTROL (0x00 << 1) 00186 #define RIGHT_LINE_INPUT_CHANNEL_VOLUME_CONTROL (0x01 << 1) 00187 #define LEFT_CHANNEL_HEADPHONE_VOLUME_CONTROL (0x02 << 1) 00188 #define RIGHT_CHANNEL_HEADPHONE_VOLUME_CONTROL (0x03 << 1) 00189 #define ANALOG_AUDIO_PATH_CONTROL (0x04 << 1) 00190 #define DIGITAL_AUDIO_PATH_CONTROL (0x05 << 1) 00191 #define POWER_DOWN_CONTROL (0x06 << 1) 00192 #define DIGITAL_AUDIO_INTERFACE_FORMAT (0x07 << 1) 00193 #define SAMPLE_RATE_CONTROL (0x08 << 1) 00194 #define DIGITAL_INTERFACE_ACTIVATION (0x09 << 1) 00195 #define RESET_REGISTER (0x0F << 1) 00196 00197 #define CLOCK_NORMAL 0 00198 #define CLOCK_USB 1 00199 #define MCLK 0 00200 #define MCLK_DIV2 1 00201 }; 00202 00203 #endif
Generated on Tue Jul 12 2022 12:04:03 by
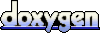