
Initial commit
Dependencies: C12832 MQTT_MbedOS mbed
main.cpp
00001 /** 00002 * Door lock Mechanism which work similar to locking and unlocking 00003 * by using an RFID card to scan nfc tag which is publish onto MQTT 00004 * server by saying in door is locked or unlucked. 00005 * By Kwame Nyantakyi 00006 00007 * Use of MQTT client code created by Daniel Knox and Fred Barnes 00008 **/ 00009 00010 // 00011 #include "mbed.h" 00012 #include "C12832.h" 00013 #include "MQTTEthernet.h" 00014 #include "MQTTClient.h" 00015 00016 /* speed we talk up the [USB] serial-port at */ 00017 #define HOST_SPEED (38400) 00018 00019 // Using Arduino pin notation 00020 C12832 lcd(D11, D13, D12, D7, D10); 00021 00022 static Serial host (USBTX, USBRX); /* connection to host */ 00023 00024 // led display 00025 DigitalOut red_led(D5); 00026 DigitalOut green_led(D9); 00027 00028 // joystick left and right 00029 DigitalIn left(A4); 00030 AnalogIn right(A5); 00031 00032 // sw2 and sw3 00033 InterruptIn sw2_int (PTC6); /* interrupts for the two on-board switches */ 00034 InterruptIn sw3_int (PTA4); 00035 00036 static volatile int sw2_trig; /* switches triggered? */ 00037 static volatile int sw3_trig; 00038 00039 PwmOut spkr(D6); 00040 00041 const char* def = "Unknown"; 00042 const char* stat = "Unknown"; 00043 00044 00045 /* connection stuff for MQTT */ 00046 typedef struct S_mqtt_params { 00047 char *hostname; 00048 int port; 00049 char *topic; 00050 char *clientid; 00051 } mqtt_params_t; 00052 00053 /* configure MQTT with hostname, port, topic and clientid */ 00054 static mqtt_params_t mqtt_config = { 00055 hostname: "129.12.44.120" 00056 , 00057 port: 1883, 00058 topic: "unikent/users/kn241/room/status" 00059 , 00060 clientid: "kn241" 00061 }; 00062 00063 /* 00064 * prints stuffs on the serial host and lcd 00065 */ 00066 static int set_up(void) 00067 { 00068 host.baud (HOST_SPEED); 00069 host.printf ("\r\n\r\nEthernet MQTT client from K64F\r\n"); 00070 00071 lcd.cls (); 00072 lcd.locate (0, 0); 00073 lcd.printf ("Door Lock Mechanism\n"); 00074 00075 return 0; 00076 } 00077 00078 00079 /* 00080 * Display the status of the connection to MQTT aswell as 00081 * the activity of the user. 00082 * 00083 * Roboust function used to print out format,arg of server 00084 * server connection and user interaction on the serial host 00085 * and lcd 00086 */ 00087 static int display_info(const char *format, ...) 00088 { 00089 va_list arg; 00090 int re; 00091 00092 va_start(arg, format); 00093 re = host.vprintf (format, arg); 00094 va_end (arg); 00095 host.printf ("\r\n"); 00096 00097 lcd.fill (0, 20, 128, 12, 0); 00098 lcd.locate (0, 15); 00099 va_start (arg, format); 00100 lcd.vprintf (format, arg); 00101 va_end (arg); 00102 00103 return re; 00104 } 00105 00106 /* plays sounds and sets chars to accepted */ 00107 void accepted() 00108 { 00109 def = "Accepted"; 00110 stat= "Unlocked"; 00111 for (float i=2000.0f; i<10000.0f; i+=100) { 00112 spkr.period(1.0f/i); 00113 spkr=0.5; 00114 wait(0.02); 00115 spkr = 0.0; 00116 } 00117 } 00118 00119 /* function which checks when wrong card is scanned */ 00120 void declined() 00121 { 00122 def = "Rejected"; 00123 stat ="Locked"; 00124 red_led = !left; 00125 00126 for (float i=2000.0f; i<10000.0f; i+=100) { 00127 spkr.period(1.0/150.0); // 500hz period 00128 spkr =0.25; 00129 wait(.02); 00130 spkr=0.0; 00131 } 00132 } 00133 00134 //main code, where the program runs 00135 int main() 00136 { 00137 const char *ip; 00138 int re = 0; 00139 MQTT::Message msg; 00140 char buf[200]; 00141 00142 set_up(); 00143 display_info("Establishing network..."); 00144 00145 /* Brings up the network interface */ 00146 MQTTEthernet eth = MQTTEthernet (); 00147 ip = eth.get_ip_address(); 00148 00149 display_info("IP address is: %s", ip ?: "Not found."); 00150 00151 /* Create Mbed Client Interface */ 00152 MQTT::Client<MQTTEthernet, Countdown> client = MQTT::Client<MQTTEthernet, Countdown> (eth); 00153 00154 /* Create TCP connection */ 00155 eth.open (eth.getEth()); 00156 re = eth.connect (mqtt_config.hostname, mqtt_config.port); 00157 00158 00159 if (!re) { 00160 display_info ("Connected Status: Good"); 00161 00162 /* Wait for a short length of time to allow user to see output messages. */ 00163 Thread::wait(1500); 00164 00165 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00166 data.keepAliveInterval = 20; 00167 data.cleansession = 1; 00168 data.MQTTVersion = 3; 00169 data.clientID.cstring = mqtt_config.clientid; 00170 re = client.connect (data); 00171 00172 if (!re) { 00173 display_info ("MQTT connected!"); 00174 00175 while (!re) { 00176 /* clear screen to setup - set up door status*/ 00177 lcd.cls (); 00178 set_up(); 00179 00180 while(1) { 00181 00182 //Depending on button pressed display a colour 00183 red_led = !left; 00184 green_led = !right; 00185 00186 if(right && !left) { 00187 accepted(); 00188 } else if(left && !right) { 00189 declined(); 00190 } 00191 display_info("Scan :%s \n", def); 00192 sprintf (buf, "%s\n", stat); 00193 // QoS 0 00194 00195 msg.qos = MQTT::QOS0; 00196 msg.retained = false; 00197 msg.dup = false; 00198 msg.payload = (void*)buf; 00199 msg.payloadlen = strlen(buf)+1; 00200 00201 re = client.publish (mqtt_config.topic, msg); 00202 wait(1.0); 00203 00204 00205 if (re) { 00206 //if data can't be publish 00207 display_info ("MQTT publish failed with %d", re); 00208 } else { 00209 Thread::wait(1500); 00210 } 00211 } 00212 } 00213 } else { 00214 // 00215 display_info ("Failed to connect to MQTT"); 00216 } 00217 } else { 00218 display_info ("Failed to connect (TCP)"); 00219 } 00220 00221 // closed ethernet socket 00222 eth.disconnect(); 00223 }
Generated on Tue Jul 12 2022 18:32:58 by
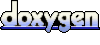