Library for MI0283QT-2 LCD
GLCD Class Reference
Graphic LCD Library for MI0283QT-2 LCD. More...
#include <MI0283QTlib.h>
Public Member Functions | |
GLCD (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName bklgh) | |
Create the GLCD Object and initlaize the I/O pins. | |
void | lcd_init (void) |
Configure the SPI port with default parameters. | |
void | lcd_init (unsigned speedf) |
Initialize the LCD with an optional SPI freq. | |
void | lcd_init (unsigned int speedf, unsigned int speeds) |
Initialize the LCD with two optionals SPI freq: fast and slow speed. | |
void | lcd_clear (unsigned int color) |
Clear the entire screen with color. | |
void | lcd_clear (unsigned int x0, unsigned int y0, unsigned int w, unsigned int h, unsigned int color) |
Clear a specified screen region. | |
unsigned int | lcd_drawimage (char *fname) |
Draw an RGB image file (320x240) starting at x=0, y=0 The image is read byte per byte. | |
unsigned int | lcd_drawimagebuff (char *fname) |
Draw an RGB image file (320x240) starting at x=0, y=0 The image is read using a buffer. | |
unsigned int | lcd_drawimagebyline (unsigned char *buffer, unsigned int lnum) |
Draw an image file (320x240) staring at x=0, y=0 The image is displayed line-by-line so the main program can do other jobs between the call. | |
unsigned int | lcd_drawimagebyline (unsigned char *buffer, unsigned int lnum, unsigned int xstart, unsigned int ystart, unsigned int scale) |
Draw an image file (less then 320x240) specifying the x and y position and the scale value. | |
unsigned int | lcd_drawmovie (char *fname, unsigned int x_start, unsigned int y_start) |
Play a movie file 80x60 pixel. | |
unsigned int | lcd_drawmoviebuff (char *fname, unsigned int x_start, unsigned int y_start) |
Play a 160x120 pixel ready made movie file. | |
void | lcd_drawicon (const unsigned int *icon, unsigned int x, unsigned int y, unsigned int size) |
Draw an RGB icon at x, y. | |
void | lcd_drawicon (const unsigned char *icon, unsigned int x, unsigned int y, unsigned int size) |
Draw an RGB icon at x, y. | |
void | lcd_drawicon (const unsigned char *icon, unsigned int x, unsigned int y, unsigned int xsize, unsigned int ysize) |
Draw an RGB icon at x, y. | |
void | lcd_drawline (unsigned int x0, unsigned int y0, unsigned int x1, unsigned int y1, unsigned int color) |
Draw a color line from xy to xy. | |
void | lcd_drawpixel (unsigned int x0, unsigned int y0, unsigned int color) |
Draw a single pixel. | |
void | lcd_drawch (unsigned ch, unsigned int xpos, unsigned int ypos, unsigned int color) |
Draw a character at xy position. | |
void | lcd_drawstr (char *__putstr, unsigned int xpos, unsigned int ypos, unsigned int color) |
Draw a string at xy position. | |
void | lcd_setfontsmall (void) |
Set the font type to small: 12x6. | |
void | lcd_setfontbig (void) |
Set the font type to big: 24x12. | |
unsigned int | lcd_RGB (unsigned int color) |
Return the color value use by the LCD. | |
unsigned int | lcd_RGB (unsigned int r, unsigned int g, unsigned int b) |
Return the color value use by the LCD. | |
void | lcd_setbackgroundcolor (unsigned int color) |
Set the background color. | |
void | lcd_setverticalarea (unsigned int topf, unsigned int height, unsigned int butf) |
Function to start the automated scroll of the screen. | |
void | backlighton (void) |
Set the backlight ON. | |
void | backlightoff (void) |
Set the backlight OFF. | |
void | backlightset (double val) |
Set the backlight at a particular value. |
Detailed Description
Graphic LCD Library for MI0283QT-2 LCD.
Image Format. About image format, I choose the way to make the library capable to read the simple RGB format. That's: a file with RGB bytes written. This format is very simple to obtain, using the convert.exe software. This program is inside the "Imagemagick" installation software. You can download the portable versio for windows at this link: http://www.imagemagick.org/download/binaries/ImageMagick-6.6.7-Q16-windows.zip
Icone Format. I use some free icons from Internet. I use the bin2h utility to convert this file to .h format. (http://www.deadnode.org/sw/bin2h/)
Example:
// Init code... #include "mbed.h" #include "GLCDlib.h" // Configure the GLCD pin: // mosi, miso, clk, cs, rst, bklgh GLCD lcd( p11, p12, p13, p14, p17, p26); int main() { lcd.lcd_init(); lcd.backlightoff(); //... do somethings... lcd.lcd_clear( LCD_WHITE); lcd.backlighton(); //... the LCD is ON }
Definition at line 89 of file MI0283QTlib.h.
Constructor & Destructor Documentation
GLCD | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | rst, | ||
PinName | bklgh | ||
) |
Create the GLCD Object and initlaize the I/O pins.
- Parameters:
-
pin mosi, pin miso, pin sclk, pin cs, pin LCD_reset, pin LCD_backlight
Definition at line 60 of file MI0283QTlib.cpp.
Member Function Documentation
void backlightoff | ( | void | ) |
Set the backlight OFF.
Definition at line 99 of file MI0283QTlib.cpp.
void backlighton | ( | void | ) |
Set the backlight ON.
Definition at line 94 of file MI0283QTlib.cpp.
void backlightset | ( | double | val ) |
Set the backlight at a particular value.
- Parameters:
-
val backlight value to set.
Definition at line 104 of file MI0283QTlib.cpp.
void lcd_clear | ( | unsigned int | x0, |
unsigned int | y0, | ||
unsigned int | w, | ||
unsigned int | h, | ||
unsigned int | color | ||
) |
Clear a specified screen region.
Like as a rectangle fill
- Parameters:
-
x0 start x position y0 start y position w rectangle width h rectangle hight color The color we use to fill the area.
Definition at line 386 of file MI0283QTlib.cpp.
void lcd_clear | ( | unsigned int | color ) |
Clear the entire screen with color.
param color The color we want to use to fill the screen
Definition at line 348 of file MI0283QTlib.cpp.
void lcd_drawch | ( | unsigned | ch, |
unsigned int | xpos, | ||
unsigned int | ypos, | ||
unsigned int | color | ||
) |
Draw a character at xy position.
- Parameters:
-
ch the character to draw xpos x position ypos y position color the color to use
Definition at line 178 of file MI0283QTlib.cpp.
void lcd_drawicon | ( | const unsigned char * | icon, |
unsigned int | x, | ||
unsigned int | y, | ||
unsigned int | size | ||
) |
Draw an RGB icon at x, y.
The size is specified for width and hight.
- Parameters:
-
*icon pointer at the array with the RGB value in byte format. That is: 0xRR, 0xGG, 0xBB x x position y y position size the width and height
Definition at line 1035 of file MI0283QTlib.cpp.
void lcd_drawicon | ( | const unsigned int * | icon, |
unsigned int | x, | ||
unsigned int | y, | ||
unsigned int | size | ||
) |
Draw an RGB icon at x, y.
The size is specified for width and hight.
- Parameters:
-
*icon pointer at the array with the RGB value in integer format. That is: 0x00RRGGBB x x position y y position size the width and height
Definition at line 1114 of file MI0283QTlib.cpp.
void lcd_drawicon | ( | const unsigned char * | icon, |
unsigned int | x, | ||
unsigned int | y, | ||
unsigned int | xsize, | ||
unsigned int | ysize | ||
) |
Draw an RGB icon at x, y.
The size is specified for width and hight.
- Parameters:
-
*icon pointer at the array with the RGB value in byte format. That is: 0xRR, 0xGG, 0xBB x x position y y position xsize the width ysize the height
Definition at line 1074 of file MI0283QTlib.cpp.
unsigned int lcd_drawimage | ( | char * | fname ) |
Draw an RGB image file (320x240) starting at x=0, y=0 The image is read byte per byte.
So use this function if you don't care about speed
- Parameters:
-
fname The file name we want to display
Definition at line 426 of file MI0283QTlib.cpp.
unsigned int lcd_drawimagebuff | ( | char * | fname ) |
Draw an RGB image file (320x240) starting at x=0, y=0 The image is read using a buffer.
You can set the buffer using the define: BUFFER_LINE This procedure speedup the draw of the image. At the cost of more RAM. The defaullt buffer size is: 320*BUFFER_LINE*3
- Parameters:
-
fname The file name we want to display
Definition at line 481 of file MI0283QTlib.cpp.
unsigned int lcd_drawimagebyline | ( | unsigned char * | buffer, |
unsigned int | lnum | ||
) |
Draw an image file (320x240) staring at x=0, y=0 The image is displayed line-by-line so the main program can do other jobs between the call.
The buffer size is equivalent to a LCD line, so must be: 320x3 bytes.
- Parameters:
-
buffer The pointer at the data to be displayed lnum The number of the line to display. From 0 to 239.
Definition at line 609 of file MI0283QTlib.cpp.
unsigned int lcd_drawimagebyline | ( | unsigned char * | buffer, |
unsigned int | lnum, | ||
unsigned int | xstart, | ||
unsigned int | ystart, | ||
unsigned int | scale | ||
) |
Draw an image file (less then 320x240) specifying the x and y position and the scale value.
The image is displayed line-by-line so the main program can do other job during the call. It is possible to specify a x and y starting position and a scale value. The buffer size is equivalent to a LCD line, so must be: 320x3 bytes.
- Parameters:
-
buffer The pointer at the data to be displayed lnum The number of the line to display. From 0 to 239. xstart x position ystart y position scale Scale factor value. There are two value defined: SCALE_320x240 and SCALE_160x120 Using the second value it's possible to draw, from a 320x240 image, a 160x120 image. at the xy specified. This is the only value supported for now.
Definition at line 561 of file MI0283QTlib.cpp.
void lcd_drawline | ( | unsigned int | x0, |
unsigned int | y0, | ||
unsigned int | x1, | ||
unsigned int | y1, | ||
unsigned int | color | ||
) |
Draw a color line from xy to xy.
- Parameters:
-
x0 x position y0 y position x1 x position y1 y position color color to use
Definition at line 1173 of file MI0283QTlib.cpp.
unsigned int lcd_drawmovie | ( | char * | fname, |
unsigned int | x_start, | ||
unsigned int | y_start | ||
) |
Play a movie file 80x60 pixel.
The file is a ready-made array of pictures extracted from a movie, resized to 80x60 and converted to RGB format. I use this procedure in a Ubuntu Linux PC:
mplayer -endpos 10 -nosound -vo png:z=0 perla.avi mogrify -resize 80x60 *.png mogrify -format rgb *.png cat *.rgb > unico_80x60.bin
First I convert 10 sec of my avi files with mplayer, then I use mogrify to resize the 150 images to 80x60 pixel. The next step is to convert the PNG format to RGB. At this point I create a single file using a simple cat command. With this little format there is enough speed to play the movie at 15fps.
Definition at line 656 of file MI0283QTlib.cpp.
unsigned int lcd_drawmoviebuff | ( | char * | fname, |
unsigned int | x_start, | ||
unsigned int | y_start | ||
) |
Play a 160x120 pixel ready made movie file.
Display a movie file.
The file is a ready-made array of pictures extracted from a movie, resized to 160x120 pixel and converted to RGB format. I use this procedure in a Ubuntu Linux PC:
mplayer -endpos 10 -nosound -vo png:z=0 perla.avi mogrify -resize 160x120 *.png mogrify -format rgb *.png cat *.rgb > unico_160x120.bin RGBconv_tst unico_160x120.bin unico_160x120_lcd.bin
First I convert 10 sec of my avi files with mplayer, then I use mogrify to resize the 150 images generated to 160x120 pixel. The next step is to convert the PNG format to RGB. At this point I create a single file using a simple cat command. To speed up the playing procedure, I convert the RGB 24bit file to the LCD format RGB 565bit using a simple programm I create. The procedure use the USB and Ethernet memory area as a ping pong buffer. The buffer loaded from the SDCard is send to the LCD using the DMA. During this time another buffer is read and stored to the second buffer. The single DMA transfer is too short for me and I use 3 linked list to chain 3 buffer of memory and send simultaneously more then 12KB if data.
- Parameters:
-
fname the file name to display x_start and y_start, the x and y position where to display the movie.
The file is ready made to speed up the entire process.
- Parameters:
-
the name file.
Definition at line 772 of file MI0283QTlib.cpp.
void lcd_drawpixel | ( | unsigned int | x0, |
unsigned int | y0, | ||
unsigned int | color | ||
) |
Draw a single pixel.
- Parameters:
-
x0 x position y0 y position color color to use
Definition at line 1149 of file MI0283QTlib.cpp.
void lcd_drawstr | ( | char * | __putstr, |
unsigned int | xpos, | ||
unsigned int | ypos, | ||
unsigned int | color | ||
) |
Draw a string at xy position.
- Parameters:
-
*putstr the string to draw xpos x position ypos y position color the color to use
Definition at line 157 of file MI0283QTlib.cpp.
void lcd_init | ( | unsigned | speedf ) |
Initialize the LCD with an optional SPI freq.
Used as FastSpeed
- Parameters:
-
clock speed in Hz
void lcd_init | ( | void | ) |
Configure the SPI port with default parameters.
- Parameters:
-
none
Definition at line 110 of file MI0283QTlib.cpp.
void lcd_init | ( | unsigned int | speedf, |
unsigned int | speeds | ||
) |
Initialize the LCD with two optionals SPI freq: fast and slow speed.
- Parameters:
-
speedf The fast freq. used to drive the GLCD speeds The freq. at wich the SPI is configured after the use.
Definition at line 131 of file MI0283QTlib.cpp.
unsigned int lcd_RGB | ( | unsigned int | r, |
unsigned int | g, | ||
unsigned int | b | ||
) |
Return the color value use by the LCD.
There are two form: 666 and 565
- Parameters:
-
r the Red color value g the Green color value b the Blue color value
- Returns:
- the color in the format: 666 or 565
Definition at line 342 of file MI0283QTlib.cpp.
unsigned int lcd_RGB | ( | unsigned int | color ) |
Return the color value use by the LCD.
There are two form: 666 and 565
- Parameters:
-
color the color in the format: 0x00RRGGBB
- Returns:
- the color in the format: 666 or 565
Definition at line 335 of file MI0283QTlib.cpp.
void lcd_setbackgroundcolor | ( | unsigned int | color ) |
Set the background color.
- Parameters:
-
color the color of the background
Definition at line 153 of file MI0283QTlib.cpp.
void lcd_setfontbig | ( | void | ) |
Set the font type to big: 24x12.
Definition at line 148 of file MI0283QTlib.cpp.
void lcd_setfontsmall | ( | void | ) |
Set the font type to small: 12x6.
Definition at line 143 of file MI0283QTlib.cpp.
void lcd_setverticalarea | ( | unsigned int | topf, |
unsigned int | height, | ||
unsigned int | butf | ||
) |
Function to start the automated scroll of the screen.
Definition at line 306 of file MI0283QTlib.cpp.
Generated on Fri Jul 15 2022 19:54:13 by
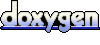