Library for MI0283QT-2 LCD
Embed:
(wiki syntax)
Show/hide line numbers
MI0283QTlib.h
00001 /* mbed Graphics LCD library. Library for MI0283QT-2 screen. 00002 00003 Copyright (c) 2011 NXP 3803 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef __MBED_GLCDLIB_H 00025 #define __MBED_GLCDLIB_H 00026 00027 // Comment out if file system functions are not used. 00028 #define _USE_FILE 00029 00030 #ifdef _USE_FILE 00031 #define BUFFER_LINE 10 00032 #endif 00033 00034 // 00035 #define LCD_BLACK (0) 00036 #define LCD_WHITE ( (255<<16)|(255<<8)|(255<<0) ) 00037 #define LCD_RED ( (255<<16)|(0<<8)|(0<<0) ) 00038 #define LCD_GREEN ( (0<<16)|(255<<8)|(0<<0) ) 00039 #define LCD_BLUE ( (0<<16)|(0<<8)|(255<<0) ) 00040 // 00041 #define SCALE_320x240 1 00042 #define SCALE_160x120 2 00043 // 00044 #include "mbed.h" 00045 00046 struct _FONTINFO { 00047 unsigned char *pText; 00048 unsigned int h_size; 00049 unsigned int v_size; 00050 unsigned int h_line; 00051 }; 00052 00053 00054 /** Graphic LCD Library for MI0283QT-2 LCD 00055 * 00056 * Image Format. 00057 * About image format, I choose the way to make the library capable to read the simple RGB format. 00058 * That's: a file with RGB bytes written. This format is very simple to obtain, using the convert.exe software. 00059 * This program is inside the "Imagemagick" installation software. You can download the portable versio for windows 00060 * at this link: http://www.imagemagick.org/download/binaries/ImageMagick-6.6.7-Q16-windows.zip 00061 * 00062 * Icone Format. 00063 * I use some free icons from Internet. I use the bin2h utility to convert this file to .h format. 00064 * (http://www.deadnode.org/sw/bin2h/) 00065 * 00066 * Example: 00067 * @code 00068 * // Init code... 00069 * #include "mbed.h" 00070 * #include "GLCDlib.h" 00071 * 00072 * // Configure the GLCD pin: 00073 * // mosi, miso, clk, cs, rst, bklgh 00074 * GLCD lcd( p11, p12, p13, p14, p17, p26); 00075 * 00076 * int main() { 00077 * 00078 * lcd.lcd_init(); 00079 * lcd.backlightoff(); 00080 * //... do somethings... 00081 * lcd.lcd_clear( LCD_WHITE); 00082 * lcd.backlighton(); 00083 * //... the LCD is ON 00084 * } 00085 * @endcode 00086 00087 */ 00088 00089 class GLCD { 00090 00091 public: 00092 /** Create the GLCD Object and initlaize the I/O pins 00093 * 00094 * @param pin mosi, pin miso, pin sclk, pin cs, pin LCD_reset, pin LCD_backlight 00095 */ 00096 GLCD( PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName bklgh); 00097 00098 /** Configure the SPI port with default parameters. 00099 * 00100 * @param none 00101 */ 00102 void lcd_init( void); 00103 00104 /** Initialize the LCD with an optional SPI freq. Used as FastSpeed 00105 * 00106 * @param clock speed in Hz 00107 */ 00108 void lcd_init( unsigned speedf); 00109 00110 /** Initialize the LCD with two optionals SPI freq: fast and slow speed. 00111 * 00112 * @param speedf The fast freq. used to drive the GLCD 00113 * @param speeds The freq. at wich the SPI is configured after the use. 00114 * 00115 */ 00116 void lcd_init( unsigned int speedf, unsigned int speeds); 00117 00118 /** Clear the entire screen with color 00119 * 00120 * #param color The color we want to use to fill the screen 00121 */ 00122 void lcd_clear(unsigned int color); 00123 00124 /** Clear a specified screen region. Like as a rectangle fill 00125 * @param x0 start x position 00126 * @param y0 start y position 00127 * @param w rectangle width 00128 * @param h rectangle hight 00129 * @param color The color we use to fill the area. 00130 * 00131 */ 00132 void lcd_clear(unsigned int x0, unsigned int y0, unsigned int w, unsigned int h, unsigned int color); 00133 00134 #ifdef _USE_FILE 00135 /** Draw an RGB image file (320x240) starting at x=0, y=0 00136 * The image is read byte per byte. So use this function if you don't care about speed 00137 * 00138 * @param fname The file name we want to display 00139 */ 00140 unsigned int lcd_drawimage(char *fname); 00141 00142 /** Draw an RGB image file (320x240) starting at x=0, y=0 00143 * The image is read using a buffer. You can set the buffer using the define: BUFFER_LINE 00144 * This procedure speedup the draw of the image. At the cost of more RAM. 00145 * The defaullt buffer size is: 320*BUFFER_LINE*3 00146 * 00147 * @param fname The file name we want to display 00148 */ 00149 unsigned int lcd_drawimagebuff(char *fname); 00150 00151 /** Draw an image file (320x240) staring at x=0, y=0 00152 * The image is displayed line-by-line so the main program can do other jobs between the call. 00153 * The buffer size is equivalent to a LCD line, so must be: 320x3 bytes. 00154 * 00155 * @param buffer The pointer at the data to be displayed 00156 * @param lnum The number of the line to display. From 0 to 239. 00157 */ 00158 unsigned int lcd_drawimagebyline(unsigned char *buffer, unsigned int lnum); 00159 00160 /** Draw an image file (less then 320x240) specifying the x and y position and the scale value. 00161 * The image is displayed line-by-line so the main program can do other job during the call. 00162 * It is possible to specify a x and y starting position and a scale value. 00163 * The buffer size is equivalent to a LCD line, so must be: 320x3 bytes. 00164 * 00165 * @param buffer The pointer at the data to be displayed 00166 * @param lnum The number of the line to display. From 0 to 239. 00167 * @param xstart x position 00168 * @param ystart y position 00169 * @param scale Scale factor value. There are two value defined: SCALE_320x240 and SCALE_160x120 00170 * Using the second value it's possible to draw, from a 320x240 image, a 160x120 image. 00171 * at the xy specified. This is the only value supported for now. 00172 */ 00173 unsigned int lcd_drawimagebyline(unsigned char *buffer, unsigned int lnum, unsigned int xstart, unsigned int ystart, unsigned int scale); 00174 00175 /** Play a movie file 80x60 pixel. 00176 * The file is a ready-made array of pictures extracted from a movie, resized to 80x60 and converted to RGB format. 00177 * I use this procedure in a Ubuntu Linux PC: 00178 * 00179 * @code 00180 * mplayer -endpos 10 -nosound -vo png:z=0 perla.avi 00181 * mogrify -resize 80x60 *.png 00182 * mogrify -format rgb *.png 00183 * cat *.rgb > unico_80x60.bin 00184 * @endcode 00185 * 00186 * First I convert 10 sec of my avi files with mplayer, then I use mogrify to resize the 150 images to 80x60 pixel. 00187 * The next step is to convert the PNG format to RGB. At this point I create a single file using a simple cat command. 00188 * With this little format there is enough speed to play the movie at 15fps. 00189 */ 00190 unsigned int lcd_drawmovie(char *fname, unsigned int x_start, unsigned int y_start); 00191 00192 /** Play a 160x120 pixel ready made movie file. 00193 * The file is a ready-made array of pictures extracted from a movie, resized to 160x120 pixel and converted to RGB format. 00194 * I use this procedure in a Ubuntu Linux PC: 00195 * 00196 * @code 00197 * mplayer -endpos 10 -nosound -vo png:z=0 perla.avi 00198 * mogrify -resize 160x120 *.png 00199 * mogrify -format rgb *.png 00200 * cat *.rgb > unico_160x120.bin 00201 * RGBconv_tst unico_160x120.bin unico_160x120_lcd.bin 00202 * @endcode 00203 * 00204 * First I convert 10 sec of my avi files with mplayer, then I use mogrify to resize the 150 images generated to 160x120 pixel. 00205 * The next step is to convert the PNG format to RGB. At this point I create a single file using a simple cat command. 00206 * To speed up the playing procedure, I convert the RGB 24bit file to the LCD format RGB 565bit using a simple programm 00207 * I create. 00208 * The procedure use the USB and Ethernet memory area as a ping pong buffer. The buffer loaded from the SDCard is send to the LCD using the DMA. 00209 * During this time another buffer is read and stored to the second buffer. The single DMA transfer is too short for me and I use 3 linked list 00210 * to chain 3 buffer of memory and send simultaneously more then 12KB if data. 00211 * 00212 * @param fname the file name to display 00213 * @param x_start and y_start, the x and y position where to display the movie. 00214 */ 00215 unsigned int lcd_drawmoviebuff(char *fname, unsigned int x_start, unsigned int y_start); 00216 00217 #endif // End of: #ifdef _USE_FILE 00218 00219 /** Draw an RGB icon at x, y. The size is specified for width and hight. 00220 * 00221 * @param *icon pointer at the array with the RGB value in integer format. That is: 0x00RRGGBB 00222 * @param x x position 00223 * @param y y position 00224 * @param size the width and height 00225 */ 00226 void lcd_drawicon( const unsigned int *icon, unsigned int x, unsigned int y, unsigned int size); 00227 00228 /** Draw an RGB icon at x, y. The size is specified for width and hight. 00229 * 00230 * @param *icon pointer at the array with the RGB value in byte format. That is: 0xRR, 0xGG, 0xBB 00231 * @param x x position 00232 * @param y y position 00233 * @param size the width and height 00234 */ 00235 void lcd_drawicon( const unsigned char *icon, unsigned int x, unsigned int y, unsigned int size); 00236 00237 /** Draw an RGB icon at x, y. The size is specified for width and hight. 00238 * 00239 * @param *icon pointer at the array with the RGB value in byte format. That is: 0xRR, 0xGG, 0xBB 00240 * @param x x position 00241 * @param y y position 00242 * @param xsize the width 00243 * @param ysize the height 00244 */ 00245 void lcd_drawicon( const unsigned char *icon, unsigned int x, unsigned int y, unsigned int xsize, unsigned int ysize); 00246 00247 /** Draw a color line from xy to xy 00248 * 00249 * @param x0 x position 00250 * @param y0 y position 00251 * @param x1 x position 00252 * @param y1 y position 00253 * @param color color to use 00254 */ 00255 void lcd_drawline(unsigned int x0, unsigned int y0, unsigned int x1, unsigned int y1, unsigned int color); 00256 00257 /** Draw a single pixel 00258 * 00259 * @param x0 x position 00260 * @param y0 y position 00261 * @param color color to use 00262 */ 00263 void lcd_drawpixel(unsigned int x0, unsigned int y0, unsigned int color); 00264 00265 /** Draw a character at xy position. 00266 * 00267 * @param ch the character to draw 00268 * @param xpos x position 00269 * @param ypos y position 00270 * @param color the color to use 00271 */ 00272 void lcd_drawch( unsigned ch, unsigned int xpos, unsigned int ypos, unsigned int color); 00273 00274 /** Draw a string at xy position 00275 * 00276 * @param *putstr the string to draw 00277 * @param xpos x position 00278 * @param ypos y position 00279 * @param color the color to use 00280 */ 00281 void lcd_drawstr(char *__putstr, unsigned int xpos, unsigned int ypos, unsigned int color); 00282 00283 /** Set the font type to small: 12x6 00284 */ 00285 void lcd_setfontsmall( void); 00286 00287 /** Set the font type to big: 24x12 00288 */ 00289 void lcd_setfontbig( void); 00290 00291 /** Return the color value use by the LCD. There are two form: 666 and 565 00292 * 00293 * @param color the color in the format: 0x00RRGGBB 00294 * @return the color in the format: 666 or 565 00295 */ 00296 unsigned int lcd_RGB( unsigned int color); 00297 00298 /** Return the color value use by the LCD. There are two form: 666 and 565 00299 * 00300 * @param r the Red color value 00301 * @param g the Green color value 00302 * @param b the Blue color value 00303 * @return the color in the format: 666 or 565 00304 */ 00305 unsigned int lcd_RGB( unsigned int r, unsigned int g, unsigned int b); 00306 00307 /** Set the background color 00308 * 00309 * @param color the color of the background 00310 */ 00311 void lcd_setbackgroundcolor( unsigned int color); 00312 00313 /** Function to start the automated scroll of the screen 00314 */ 00315 void lcd_setverticalarea( unsigned int topf, unsigned int height, unsigned int butf); 00316 void lcd_scrollstartadd( unsigned int ssa); 00317 void lcd_scrollstart( void); 00318 void lcd_scrollstop( void); 00319 00320 /** Set the backlight ON 00321 * 00322 */ 00323 void backlighton( void); 00324 00325 /** Set the backlight OFF 00326 * 00327 */ 00328 void backlightoff( void); 00329 00330 /** Set the backlight at a particular value. 00331 * 00332 * @param val backlight value to set. 00333 */ 00334 void backlightset( double val); 00335 00336 protected: 00337 void lcd_draw(unsigned int color); 00338 void lcd_drawstop(void); 00339 void lcd_drawstart(void); 00340 void lcd_cmd(unsigned int reg, unsigned int param); 00341 void lcd_data(unsigned int c); 00342 void lcd_area(unsigned int x0, unsigned int y0, unsigned int x1, unsigned int y1); 00343 void lcd_reset(void); 00344 00345 // default value for SPIClock speed, SPI mode and backlight freq. 00346 unsigned int SPIClkSpeed; 00347 unsigned int SPISlowClkSpeed; 00348 unsigned int SPIMode; 00349 double LCDBkLight; 00350 // default value for background color 00351 unsigned int BackGroundColor; 00352 // 00353 unsigned char FontIdx; 00354 _FONTINFO FontInfo[2]; 00355 00356 SPI _spi; 00357 DigitalOut _cs; 00358 DigitalOut _rst; 00359 PwmOut _bklgh; 00360 00361 }; 00362 00363 #endif
Generated on Fri Jul 15 2022 19:54:13 by
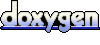