
Time Machine
Dependencies: TextLCD mbed DebounceIn
main.cpp
00001 // Read from I2C slave at address 0x62 00002 #include <iostream> 00003 #include "mbed.h" 00004 00005 #include <math.h> 00006 #include <string> 00007 #define MESSAGE_BUFFER_SIZE 1024 00008 #include "time.h" 00009 #include <stdio.h> 00010 #include <stdlib.h> 00011 00012 00013 Serial pc(USBTX,USBRX); 00014 00015 char line= '\n'; 00016 char c; 00017 char str[40]; 00018 char Hi; 00019 int i; 00020 struct tm t; 00021 00022 00023 void message() //Get character (e.g. Printf the Words from serial) 00024 { 00025 strcpy (str,&Hi); 00026 do { 00027 c=pc.getc(); 00028 pc.putc (c); 00029 strcat (str,&c); 00030 00031 }while (c != ' '); 00032 00033 00034 } 00035 00036 void time_setup() { 00037 pc.baud(9600); 00038 00039 pc.printf("SET TIME\n\r"); 00040 pc.printf("--------\n\r\n\r"); 00041 00042 // get the current time from the terminal 00043 00044 do{ 00045 pc.printf("Enter current date and time:\n\r"); 00046 pc.printf("YYYY MM DD HH MM SS\n\r"); 00047 00048 message(); 00049 t.tm_year = atoi (str); 00050 message(); 00051 t.tm_mon = atoi (str); 00052 message(); 00053 t.tm_mday= atoi (str); 00054 message(); 00055 t.tm_hour = atoi (str); 00056 message(); 00057 t.tm_min = atoi (str); 00058 message(); 00059 t.tm_sec = atoi (str); 00060 00061 pc.printf("\n\r\n\r"); 00062 pc.printf("Is the time correct [y/n]\n\r"); 00063 c=pc.getc(); 00064 pc.printf("\n\r"); 00065 }while(c !='y'); 00066 00067 // adjust for tm structure required values 00068 t.tm_year = t.tm_year - 1900; 00069 t.tm_mon = t.tm_mon - 1; 00070 00071 // set the time 00072 set_time(mktime(&t)); 00073 00074 // display the time 00075 while(1) { 00076 00077 time_t seconds = time(NULL); 00078 pc.printf("Time as a basic string = %s\n\r", ctime(&seconds)); 00079 wait(1); 00080 } 00081 00082 00083 00084 00085 00086 } 00087 int main() 00088 { 00089 time_setup(); 00090 00091 00092 00093 }
Generated on Sat Jul 23 2022 02:40:09 by
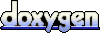