
Testprogram for mRotaryEncoder-os
Dependencies: mRotaryEncoder-os PinDetect
main.cpp
00001 #include "mbed.h" 00002 //#include "USBSerial.h" 00003 #include "mRotaryEncoder.h" 00004 00005 /** Test the Library for mechanical rotary encoders with pushbutton 00006 * like this one from alps http://www.alps.com/WebObjects/catalog.woa/E/HTML/Encoder/Incremental/EC11/EC11E09244BS.shtml 00007 * should work for any other incremental encoder with button 00008 */ 00009 00010 // mbed LEDs 00011 DigitalOut led1(LED1); 00012 DigitalOut led2(LED2); 00013 DigitalOut led3(LED3); 00014 DigitalOut led4(LED4); 00015 00016 //USBSerial pcout; 00017 static BufferedSerial pcout(USBTX, USBRX); // tx, rx 00018 00019 00020 //mRotaryEncoder(PinName pinA, PinName pinB, PinName pinSW, PinMode pullMode=PullUp, int debounceTime_us=1000) 00021 mRotaryEncoder wheel(p16, p17, p18,PullUp,1500); 00022 00023 00024 int lastGet; 00025 int thisGet; 00026 00027 00028 bool enc_pressed = false; // Button of rotaryencoder was pressed 00029 bool enc_rotated = false; // rotary encoder was totaded left or right 00030 00031 00032 //interrup-Handler for button on rotary-encoder 00033 void trigger_sw() { 00034 enc_pressed = true; // just set the flag, rest is done outside isr 00035 } 00036 00037 //interrup-Handler for rotary-encoder rotation 00038 void trigger_rotated() { 00039 enc_rotated = true; // just set the flag, rest is done outside isr 00040 } 00041 00042 00043 // display int-Value on the 4 LEDS 00044 void displayLED(int value) { 00045 switch (abs(value) % 4) { // Get 2 LSBs and display sequence on mbed LEDs 00046 case 0: 00047 led1 = 1; 00048 led2 = 0; 00049 led3 = 0; 00050 led4 = 0; 00051 break; 00052 case 1: 00053 led1 = 0; 00054 led2 = 1; 00055 led3 = 0; 00056 led4 = 0; 00057 break; 00058 case 2: 00059 led1 = 0; 00060 led2 = 0; 00061 led3 = 1; 00062 led4 = 0; 00063 break; 00064 default: 00065 led1 = 0; 00066 led2 = 0; 00067 led3 = 0; 00068 led4 = 1; 00069 break; 00070 } // switch 00071 } 00072 00073 int main() { 00074 00075 pcout.set_baud(115200); 00076 printf("\n\rconnected to mbed...\n\r"); 00077 00078 //Int-Handler 00079 // call trigger_sw() when button of rotary-encoder is pressed 00080 wheel.attachSW(&trigger_sw); 00081 00082 // call trigger_rot() when the shaft is rotaded left or right 00083 wheel.attachROT(&trigger_rotated); 00084 00085 lastGet = 0; 00086 00087 // set encrotated, so position is displayed on startup 00088 enc_rotated = true; 00089 00090 while (1) { 00091 00092 00093 // shaft has been rotated? 00094 if (enc_rotated) { 00095 enc_rotated = false; 00096 00097 thisGet = wheel.Get(); 00098 00099 displayLED(thisGet); 00100 printf ("Pulses is: %i\n\r", thisGet); 00101 00102 } 00103 00104 00105 // Button pressed? 00106 if (enc_pressed) { 00107 enc_pressed = false; 00108 printf("triggered!\n\r"); 00109 00110 wheel.Set(0); 00111 00112 //Update displays 00113 thisGet = wheel.Get(); 00114 displayLED(thisGet); 00115 printf ("\n\rPulses is: %i\n\r", thisGet); 00116 00117 } 00118 //do something else 00119 00120 } 00121 00122 }
Generated on Wed Jul 13 2022 07:18:07 by
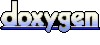