Class mRotaryEncoder for mechanical incremental rotary encoders with pushbuttons. Use debouncing and callback-functions for rotation and pressing of button. This version is for old mbed. New version for mbed-os see https://os.mbed.com/users/charly/code/mRotaryEncoder-os/
Dependents: SimplePIDBot FinalProgram VS1053Player SPK-DVIMXR ... more
mRotaryEncoder.h
00001 #ifndef MROTENC_H_INCLUDED 00002 #define MROTENC_H_INCLUDED 00003 00004 #include "mbed.h" 00005 #include "PinDetect.h" 00006 00007 00008 /** This Class handles a rotary encoder with mechanical switches and an integrated pushbutton 00009 * It uses two pins, one creating an interrupt on change. 00010 * Rotation direction is determined by checking the state of the other pin. 00011 * Additionally a pushbutton switch is detected 00012 * 00013 * Operating the encoder changes an internal integer value that can be read 00014 * by Get() or the operator int() functions. 00015 * A new value can be set by Set(value) or opperator=. 00016 * 00017 * Autor: Thomas Raab (Raabinator) 00018 * Extended by Karl Zweimueller (charly) 00019 * 00020 * Dent steady point ! ! ! 00021 * +-----+ +-----+ 00022 * pinA (interrupt) | | | | 00023 * --+ +-----+ +--- 00024 * +-----+ +-----+ 00025 * pinB | | | | 00026 * ----+ +-----+ +- 00027 * --> C.W 00028 * CW: increases position value 00029 * CCW: decreases position value 00030 * 00031 * changelog: 00032 * 00033 * 09. Nov. 2010 00034 * First version published Thomas Raab raabinator 00035 * 26.11.2010 extended by charly - pushbutton, pullmode, debounce, callback-system 00036 * Feb2011 Changes InterruptIn to PinDetect which does the debounce of mechanical switches 00037 * Mar2020 Configurable detection of rise/fall events to account for different types of encoders (half as much dent points) 00038 * 00039 */ 00040 class mRotaryEncoder { 00041 public: 00042 /** Create a mechanical rotary encoder object connected to the specified pins 00043 * 00044 * @param pinA Switch A of quadrature encoder 00045 * @param pinB Switch B of quadrature encoder 00046 * @param pinSW Pin for push-button switch 00047 * @param pullmode mode for pinA pinB and pinSW like DigitalIn.mode 00048 * @param debounceTime_us time in micro-seconds to wait for bouncing of mechanical switches to end 00049 * @param detectRise Detect rise event as new rotation 00050 * @param detectFall Detect fall event as new rotation 00051 */ 00052 mRotaryEncoder(PinName pinA, PinName pinB, PinName pinSW, PinMode pullMode=PullUp, int debounceTime_us=1000, int detectRise=1, int detectFall=1); 00053 00054 /** destroy object 00055 * 00056 */ 00057 ~mRotaryEncoder(); 00058 00059 /** Get the actual value of the rotary position 00060 * 00061 * @return position int value of position 00062 */ 00063 int Get(void); 00064 inline operator int() { 00065 return Get(); 00066 } 00067 00068 /** Set the current position value 00069 * 00070 * @param value the new position to set 00071 * 00072 */ 00073 void Set(int value); 00074 inline mRotaryEncoder& operator= ( int value ) { 00075 Set(value); 00076 return *this; 00077 } 00078 00079 /** attach a function to be called when switch is pressed 00080 * 00081 * keep this function short, as no interrrupts can occour within 00082 * 00083 * @param fptr Pointer to callback-function 00084 */ 00085 void attachSW(void (*fptr)(void)) { 00086 m_pinSW->attach_deasserted(fptr); 00087 } 00088 00089 template<typename T> 00090 /** attach an object member function to be called when switch is pressed 00091 * 00092 * @param tptr pointer to object 00093 * @param mprt pointer ro member function 00094 * 00095 */ 00096 void attachSW(T* tptr, void (T::*mptr)(void)) { 00097 if ((mptr != NULL) && (tptr != NULL)) { 00098 m_pinSW->attach_deasserted(tptr, mptr); 00099 } 00100 } 00101 00102 /** callback-System for rotation of shaft 00103 * 00104 * attach a function to be called when the shaft is rotated 00105 * keep this function short, as no interrrupts can occour within 00106 * 00107 * @param fprt Pointer to callback-function 00108 */ 00109 void attachROT(void (*fptr)(void)) { 00110 rotIsr.attach(fptr); 00111 } 00112 00113 00114 template<typename T> 00115 /** attach an object member function to be called when shaft is rotated 00116 * 00117 * @param tptr pointer to object 00118 * @param mprt pointer ro member function 00119 * 00120 */ 00121 void attachROT(T* tptr, void (T::*mptr)(void)) { 00122 if ((mptr != NULL) && (tptr != NULL)) { 00123 rotIsr.attach(tptr, mptr); 00124 } 00125 } 00126 00127 /** callback-System for rotation of shaft CW 00128 * 00129 * attach a function to be called when the shaft is rotated clockwise 00130 * keep this function short, as no interrrupts can occour within 00131 * 00132 * @param fprt Pointer to callback-function 00133 */ 00134 void attachROTCW(void (*fptr)(void)) { 00135 rotCWIsr.attach(fptr); 00136 } 00137 00138 00139 template<typename T> 00140 /** attach an object member function to be called when shaft is rotated clockwise 00141 * 00142 * @param tptr pointer to object 00143 * @param mprt pointer ro member function 00144 * 00145 */ 00146 void attachROTCW(T* tptr, void (T::*mptr)(void)) { 00147 if ((mptr != NULL) && (tptr != NULL)) { 00148 rotCWIsr.attach(tptr, mptr); 00149 } 00150 } 00151 00152 /** callback-System for rotation of shaft CCW 00153 * 00154 * attach a function to be called when the shaft is rotated counterclockwise 00155 * keep this function short, as no interrrupts can occour within 00156 * 00157 * @param fprt Pointer to callback-function 00158 */ 00159 void attachROTCCW(void (*fptr)(void)) { 00160 rotCCWIsr.attach(fptr); 00161 } 00162 00163 00164 template<typename T> 00165 /** attach an object member function to be called when shaft is rotated CCW 00166 * 00167 * @param tptr pointer to object 00168 * @param mprt pointer ro member function 00169 * 00170 */ 00171 void attachROTCCW(T* tptr, void (T::*mptr)(void)) { 00172 if ((mptr != NULL) && (tptr != NULL)) { 00173 rotCCWIsr.attach(tptr, mptr); 00174 } 00175 } 00176 00177 private: 00178 PinDetect *m_pinA; 00179 DigitalIn *m_pinB; 00180 volatile int m_position; 00181 00182 int m_debounceTime_us; 00183 00184 00185 PinDetect *m_pinSW; 00186 00187 void rise(void); 00188 void fall(void); 00189 00190 protected: 00191 /** 00192 * Callback system. 00193 * @ingroup INTERNALS 00194 */ 00195 /** 00196 * rotated any direction 00197 */ 00198 FunctionPointer rotIsr; 00199 /** 00200 * clockwise rotated 00201 */ 00202 FunctionPointer rotCWIsr; 00203 00204 /** 00205 * counterclockwise rotated 00206 */ 00207 FunctionPointer rotCCWIsr; 00208 00209 00210 }; 00211 00212 00213 #endif
Generated on Tue Jul 12 2022 14:09:21 by
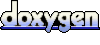