Class mRotaryEncoder for mechanical incremental rotary encoders with pushbuttons. Use debouncing and callback-functions for rotation and pressing of button. This version is for old mbed. New version for mbed-os see https://os.mbed.com/users/charly/code/mRotaryEncoder-os/
Dependents: SimplePIDBot FinalProgram VS1053Player SPK-DVIMXR ... more
mRotaryEncoder.cpp
00001 #include "mbed.h" 00002 #include "mRotaryEncoder.h" 00003 00004 00005 mRotaryEncoder::mRotaryEncoder(PinName pinA, PinName pinB, PinName pinSW, PinMode pullMode, int debounceTime_us, int detectRise, int detectFall) { 00006 m_pinA = new PinDetect(pinA); // interrrupts on pinA 00007 m_pinB = new DigitalIn(pinB); // only digitalIn for pinB 00008 00009 //set pins with internal PullUP-default 00010 m_pinA->mode(pullMode); 00011 m_pinB->mode(pullMode); 00012 00013 // attach interrrupts on pinA 00014 if (detectRise != 0){ 00015 m_pinA->attach_asserted(this, &mRotaryEncoder::rise); 00016 } 00017 if (detectFall != 0){ 00018 m_pinA->attach_deasserted(this, &mRotaryEncoder::fall); 00019 } 00020 00021 //start sampling pinA 00022 m_pinA->setSampleFrequency(debounceTime_us); // Start timers an Defaults debounce time. 00023 00024 // Switch on pinSW 00025 m_pinSW = new PinDetect(pinSW); // interrupt on press switch 00026 m_pinSW->mode(pullMode); 00027 00028 m_pinSW->setSampleFrequency(debounceTime_us); // Start timers an Defaults debounce time. 00029 00030 00031 m_position = 0; 00032 00033 m_debounceTime_us = debounceTime_us; 00034 } 00035 00036 mRotaryEncoder::~mRotaryEncoder() { 00037 delete m_pinA; 00038 delete m_pinB; 00039 delete m_pinSW; 00040 } 00041 00042 int mRotaryEncoder::Get(void) { 00043 return m_position; 00044 } 00045 00046 00047 00048 void mRotaryEncoder::Set(int value) { 00049 m_position = value; 00050 } 00051 00052 00053 void mRotaryEncoder::fall(void) { 00054 // debouncing does PinDetect for us 00055 //pinA still low? 00056 if (*m_pinA == 0) { 00057 if (*m_pinB == 1) { 00058 m_position++; 00059 rotCWIsr.call(); 00060 } else { 00061 m_position--; 00062 rotCCWIsr.call(); 00063 } 00064 rotIsr.call(); // call the isr for rotation 00065 } 00066 } 00067 00068 void mRotaryEncoder::rise(void) { 00069 //PinDetect does debouncing 00070 //pinA still high? 00071 if (*m_pinA == 1) { 00072 if (*m_pinB == 1) { 00073 m_position--; 00074 rotCCWIsr.call(); 00075 } else { 00076 m_position++; 00077 rotCWIsr.call(); 00078 } 00079 rotIsr.call(); // call the isr for rotation 00080 } 00081 } 00082
Generated on Tue Jul 12 2022 14:09:21 by
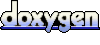