
Programm for decoding radio-signals sent by a ETH-Window-Shutter-Contact, received with a RFM12B-module
Embed:
(wiki syntax)
Show/hide line numbers
rfm12b.h
Go to the documentation of this file.
00001 #ifndef rfm12B_H 00002 #define rfm12B_H 00003 00004 #include <mbed.h> 00005 00006 /*! 00007 * \file rfm12b.h 00008 * \brief class for rfm2b in rawmode - only receive part implemented 00009 * \author Karl Zweimüller based on code from WED 6.9.2009 00010 */ 00011 00012 typedef unsigned char Byte; // used to be uint8_t : something a byte wide, whatever .... 00013 00014 /** This Class handles a rfm12b transceiver 00015 * see http://www.hoperf.com/rf_fsk/rfm12b.htm 00016 * 00017 */ 00018 class rfm12b { 00019 public: 00020 /** Create a rfm12b object 00021 * 00022 * @param mosi SPI-Interface. One of the 2 PSI-Interfaces of mbed. Pin p5 or p11 00023 * @param miso SPI-Interface. One of the 2 PSI-Interfaces of mbed. Pin p6 or p12 00024 * @param sclk SPI-Interface. One of the 2 PSI-Interfaces of mbed. Pin p7 or p13 00025 * @param nsel Chip-Select. A Digial Output of mbed 00026 * @param rxdata Data-Pin for received data. A DigitalIn of mbed 00027 */ 00028 rfm12b(PinName mosi, PinName miso, PinName sclk, PinName nsel, PinName rxdata); 00029 00030 /** init the spi-interface 00031 */ 00032 void init_spi(); 00033 00034 /** initialize the device 00035 */ 00036 void RFM_init(void); 00037 00038 /** write and read 16 bit 00039 */ 00040 uint16_t rfm_spi16(uint16_t outval); 00041 00042 /** attach a function to be called when the data-pin changes from 0->1 and from 1->0 00043 * this function has to do all the decoding 00044 * keep this function short, as no interrrupts can occour within 00045 * 00046 * @param fptr Pointer to callback-function 00047 */ 00048 void attachISR(void (*fptr)(void)) { 00049 m_pinRXData->fall(fptr); 00050 m_pinRXData->rise(fptr); 00051 } 00052 00053 template<typename T> 00054 /** attach an object member function to be called when the data-pin changes from 0->1 and from 1->0 00055 * 00056 * @param tptr pointer to object 00057 * @param mprt pointer ro member function 00058 * 00059 */ 00060 void attachISR(T* tptr, void (T::*mptr)(void)) { 00061 if ((mptr != NULL) && (tptr != NULL)) { 00062 m_pinRXData->fall(tptr, mptr); 00063 m_pinRXData->rise(tptr, mptr); 00064 } 00065 } 00066 00067 00068 00069 private: 00070 00071 00072 DigitalOut *cs; //chipselect 00073 InterruptIn *m_pinRXData; //rx data pin 00074 SPI *rfm12b_spi; //spi-interface 00075 00076 00077 }; 00078 00079 #endif
Generated on Thu Jul 14 2022 01:29:01 by
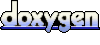