
Programm for decoding radio-signals sent by a ETH-Window-Shutter-Contact, received with a RFM12B-module
Embed:
(wiki syntax)
Show/hide line numbers
rfm12b.cpp
Go to the documentation of this file.
00001 #include "mbed.h" 00002 #include "rfm12b.h" 00003 #include "rfm.h" 00004 00005 /*! 00006 * \file rfm12b.cpp 00007 * \brief class for rfm2b in rawmode - only receive part implemented 00008 * \author Karl Zweimüller based on code from WED 6.9.2009 00009 */ 00010 00011 00012 /** Class rfm12b for RFM12B transceiver module 00013 http://www.hoperf.com/rf_fsk/rfm12b.htm 00014 */ 00015 00016 /** rfm12b object 00017 */ 00018 rfm12b::rfm12b(PinName mosi, PinName miso, PinName sclk, PinName nsel, PinName rxdata) { 00019 00020 rfm12b_spi = new SPI(mosi, miso, sclk); // mosi, miso, sclk 00021 cs = new DigitalOut(nsel); // nsel for chipselect 00022 m_pinRXData = new InterruptIn(rxdata); // rxData- generates interrupts 00023 00024 init_spi(); // init the spi-device 00025 } 00026 00027 /** init the spi-communication 00028 */ 00029 void rfm12b::init_spi() { 00030 // Setup the spi for 16 bit data : 1RW-bit 7 adressbit, 8 databit 00031 // second edge capture, with a 5MHz clock rate 00032 rfm12b_spi->format(16,0); 00033 rfm12b_spi->frequency(5000000); 00034 } 00035 00036 /////////////////////////////////////////////////////////////////////////////// 00037 // 00038 // Initialise RF module 00039 // This are parameters for ETH Comfort by ELV 00040 /////////////////////////////////////////////////////////////////////////////// 00041 void rfm12b::RFM_init(void) { 00042 00043 // 0. Init the SPI backend 00044 //RFM_TESTPIN_INIT; 00045 00046 //RFM_READ_STATUS(); 00047 00048 // 1. Configuration Setting Command 00049 RFM_SPI_16( 00050 //RFM_CONFIG_EL | 00051 //RFM_CONFIG_EF | 00052 RFM_CONFIG_BAND_868 | 00053 RFM_CONFIG_X_11_0pf 00054 ); 00055 00056 // 2. Power Management Command 00057 //RFM_SPI_16( 00058 // RFM_POWER_MANAGEMENT // switch all off 00059 // ); 00060 00061 // 3. Frequency Setting Command 00062 RFM_SPI_16( 00063 RFM_FREQUENCY | 00064 RFM_FREQ_868Band(868.30) 00065 ); 00066 00067 // 4. Data Rate Command 00068 RFM_SPI_16(RFM_DATA_RATE_9600); 00069 00070 // 5. Receiver Control Command 00071 RFM_SPI_16( 00072 RFM_RX_CONTROL_P20_VDI | 00073 RFM_RX_CONTROL_VDI_FAST | 00074 //RFM_RX_CONTROL_BW(RFM_BAUD_RATE) | 00075 RFM_RX_CONTROL_BW_134 | 00076 RFM_RX_CONTROL_GAIN_0 | 00077 RFM_RX_CONTROL_RSSI_73 00078 ); 00079 00080 // 6. Data Filter Command 00081 RFM_SPI_16( 00082 //RFM_DATA_FILTER_AL | 00083 //RFM_DATA_FILTER_ML | 00084 //RFM_DATA_FILTER_DQD(3) 00085 RFM_DATA_FILTER_ANALOG 00086 ); 00087 00088 // 7. FIFO and Reset Mode Command 00089 RFM_SPI_16( 00090 RFM_FIFO_IT(8) | 00091 RFM_FIFO_DR 00092 ); 00093 00094 // 8. Receiver FIFO Read 00095 00096 // 9. AFC Command 00097 RFM_SPI_16( 00098 RFM_AFC_AUTO_VDI | 00099 RFM_AFC_RANGE_LIMIT_7_8 | 00100 RFM_AFC_EN | 00101 RFM_AFC_OE | 00102 RFM_AFC_FI 00103 ); 00104 00105 // 10. TX Configuration Control Command 00106 RFM_SPI_16( 00107 RFM_TX_CONTROL_MOD_30 | 00108 RFM_TX_CONTROL_POW_0 00109 ); 00110 00111 // 11. Transmitter Register Write Command 00112 00113 // 12. Wake-Up Timer Command 00114 00115 // 13. Low Duty-Cycle Command 00116 00117 // 14. Low Battery Detector Command 00118 00119 //RFM_SPI_16( 00120 // RFM_LOW_BATT_DETECT | 00121 // 3 // 2.2V + v * 0.1V 00122 // ); 00123 00124 // 15. Status Read Command 00125 //RFM_SPI_16(RFM_TX_ON()); 00126 RFM_SPI_16(RFM_RX_ON()); 00127 00128 } 00129 00130 /////////////////////////////////////////////////////////////////////////////// 00131 00132 00133 00134 /** write and read 16 bit to device 00135 */ 00136 uint16_t rfm12b::rfm_spi16(uint16_t outval) { 00137 00138 uint16_t readval; 00139 // Select the device by seting chip select low 00140 cs->write(0); 00141 wait_ms(1); // wait before going on 00142 //write and read 00143 readval = rfm12b_spi->write(outval); 00144 // Deselect the device 00145 cs->write(1); 00146 wait_ms(1); // wait before going on 00147 return(readval); 00148 } 00149 00150
Generated on Thu Jul 14 2022 01:29:01 by
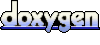