Library for Real Time Clock module MCP97410 based on Library for DS1307
Fork of RTC-DS1307 by
Rtc_Mcp97410 Class Reference
Class Rtc_Mcp97410 implements the real time clock module MCP97410. More...
#include <Rtc_Mcp97410.h>
Inherited by RtcCls.
Data Structures | |
struct | Time_rtc |
Structure which is used to exchange the time and date. More... | |
Public Types | |
enum | SqwRateSelect_t |
RateSelect specifies the valid frequency values for the square wave output. More... | |
Public Member Functions | |
Rtc_Mcp97410 (I2C *i2c) | |
public constructor which creates the real time clock object | |
virtual bool | getTime (Time_rtc &time) |
Read the current time from RTC chip. | |
virtual bool | setTime (Time_rtc &time, bool start, bool thm) |
Write the given time onto the RTC chip (and enable Battery-Backup) | |
bool | startClock () |
Start the clock. | |
bool | stopClock () |
Stop the clock. | |
const char * | weekdayToString (int wday) |
Service function to convert a weekday into a string representation. | |
bool | setSquareWaveOutput (bool ena, SqwRateSelect_t rs) |
Enable Square Wave output. | |
bool | enableEEPROMWrite () |
enable write-Operations to EEPROM | |
bool | disableEEPROMWrite () |
disable write-Operations to EEPROM | |
uint8_t | readTrim () |
read the OSCTRIM-Register (0x08) | |
bool | incTrim () |
Increment TRIM-Value. | |
bool | decTrim () |
Derement TRIM-Value. | |
bool | readEEPROM (int address, char *buffer, int len) |
read from EEPROM | |
bool | writeEEPROM (int address, char *buffer, int len) |
write to EEPROM | |
bool | readEUI48 (uint8_t *eui48) |
read 6Byte EUI-48 address from EEPROM | |
bool | writeEUI48 (uint8_t *eui48) |
write 6Byte EUI-48 address to EEPROM | |
bool | readEUI64 (uint8_t *eui64) |
read 8Byte EUI-64 address from EEPROM | |
bool | writeEUI64 (uint8_t *eui64) |
write 8Byte EUI-64 address to EEPROM | |
bool | unlockEUI () |
unlock the EUI area in the EEPROM |
Detailed Description
Class Rtc_Mcp97410 implements the real time clock module MCP97410.
You can read the clock and set a new time and date. It is also possible to start and stop the clock. Rtc_Mcp97410 allows you to display the time in a 12h or 24h format. Based on Library for DS1307 by Henry Leinen. Ported for MC97410 by Karl Zweimueller.
Definition at line 114 of file Rtc_Mcp97410.h.
Member Enumeration Documentation
enum SqwRateSelect_t |
RateSelect specifies the valid frequency values for the square wave output.
Definition at line 132 of file Rtc_Mcp97410.h.
Constructor & Destructor Documentation
Rtc_Mcp97410 | ( | I2C * | i2c ) |
public constructor which creates the real time clock object
- Parameters:
-
i2c : Pointer to I2C-Object for I2C-Interface.
Definition at line 12 of file Rtc_Mcp97410.cpp.
Member Function Documentation
bool decTrim | ( | ) |
bool disableEEPROMWrite | ( | ) |
disable write-Operations to EEPROM
Definition at line 303 of file Rtc_Mcp97410.cpp.
bool enableEEPROMWrite | ( | ) |
enable write-Operations to EEPROM
Definition at line 312 of file Rtc_Mcp97410.cpp.
bool getTime | ( | Time_rtc & | time ) | [virtual] |
Read the current time from RTC chip.
- Parameters:
-
time : reference to a struct tm which will be filled with the time from rtc
- Returns:
- true if successful, otherwise an acknowledge error occured
Definition at line 66 of file Rtc_Mcp97410.cpp.
bool incTrim | ( | ) |
bool readEEPROM | ( | int | address, |
char * | buffer, | ||
int | len | ||
) |
read from EEPROM
address from 0x00 to 0x7F all 128 Bytes can be read in one chunk
- Parameters:
-
address : start address to read from *buffer : buffer of char to write the result to. Be sure to have enough storage len : number of bytes to read
Definition at line 196 of file Rtc_Mcp97410.cpp.
bool readEUI48 | ( | uint8_t * | eui48 ) |
read 6Byte EUI-48 address from EEPROM
- Parameters:
-
eui48 : array to hold 6 Bytes of EUI48
Definition at line 320 of file Rtc_Mcp97410.cpp.
bool readEUI64 | ( | uint8_t * | eui64 ) |
read 8Byte EUI-64 address from EEPROM
- Parameters:
-
eui64 : array to hold 8 Bytes of EUI64
Definition at line 332 of file Rtc_Mcp97410.cpp.
uint8_t readTrim | ( | ) |
read the OSCTRIM-Register (0x08)
-127 .. +127 bit 7 is Sign bit 0:6 Trim-Value
Definition at line 236 of file Rtc_Mcp97410.cpp.
bool setSquareWaveOutput | ( | bool | ena, |
SqwRateSelect_t | rs | ||
) |
Enable Square Wave output.
The function enables or disables the square wave output of the module and sets the desired frequency.
- Parameters:
-
ena : if set to true, the square wave output is enabled. rs : rate select, can be either one of the four values defined by type /c RateSelect_t
- Returns:
- true if the operation was successful or false otherwise
Definition at line 143 of file Rtc_Mcp97410.cpp.
bool setTime | ( | Time_rtc & | time, |
bool | start, | ||
bool | thm | ||
) | [virtual] |
Write the given time onto the RTC chip (and enable Battery-Backup)
- Parameters:
-
time : refereence to a struct which contains valid date and time information start : contains true if the clock shall start (or keep on running). thm : 12-hour-mode if set to true, otherwise 24-hour-mode will be set.
- Returns:
- true if successful, otherwise an acknowledge error occured
Definition at line 29 of file Rtc_Mcp97410.cpp.
bool startClock | ( | ) |
Start the clock.
Please note that the seconds register need to be read and written in order to start or stop the clock. This can lead to an error in the time value. The recommended way of starting and stoping the clock is to write the actual date and time and set the start bit accordingly.
- Returns:
- true if the clock was started, false if a communication error occured
Definition at line 98 of file Rtc_Mcp97410.cpp.
bool stopClock | ( | ) |
Stop the clock.
Please note that the seconds register need to be read and written in order to start or stop the clock. This can lead to an error in the time value. The recommended way of starting and stoping the clock is to write the actual date and time and set the start bit accordingly.
- Returns:
- true if the clock was stopped, false if a communication error occured
Definition at line 121 of file Rtc_Mcp97410.cpp.
bool unlockEUI | ( | ) |
unlock the EUI area in the EEPROM
write operation must immediatly follow the unlock procedure after a write the EEPROM is automatically locked again
Definition at line 345 of file Rtc_Mcp97410.cpp.
const char* weekdayToString | ( | int | wday ) |
Service function to convert a weekday into a string representation.
- Parameters:
-
wday : day of week to convert (starting with sunday = 1, monday = 2, ..., saturday = 7
- Returns:
- the corresponding string representation
Definition at line 199 of file Rtc_Mcp97410.h.
bool writeEEPROM | ( | int | address, |
char * | buffer, | ||
int | len | ||
) |
write to EEPROM
address from 0x00 to 0x7F only maximum 8 Bytes can be written in one chunk
- Parameters:
-
address : start address to write *buffer : buffer of chars to write len : number of bytes to write
Definition at line 225 of file Rtc_Mcp97410.cpp.
bool writeEUI48 | ( | uint8_t * | eui48 ) |
write 6Byte EUI-48 address to EEPROM
be sure to enable EUI-write-Operation (UNLOCK) before
- Parameters:
-
eui48 : array with 6 Bytes of EUI48
Definition at line 326 of file Rtc_Mcp97410.cpp.
bool writeEUI64 | ( | uint8_t * | eui64 ) |
write 8Byte EUI-64 address to EEPROM
be sure to enable EUI-write-Operation (UNLOCK) before
- Parameters:
-
eui64 : array with 8 Bytes of EUI64
Definition at line 339 of file Rtc_Mcp97410.cpp.
Generated on Tue Jul 12 2022 22:54:37 by
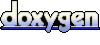