
for frequency correction testing
Dependencies: FreescaleIAP SimpleDMA mbed-rtos mbed
Fork of CDMS_CODE by
i2c.h
00001 #define tm_len 134 00002 #define tc_len 135 00003 #define tc_test_len 135 00004 00005 const int addr = 0x20; //slave address 00006 bool write_ack = false; 00007 bool read_ack = false; 00008 const int addr_pl = 0x20<<1; //PL address 00009 const int addr_bae = 0x20; ///bae address 00010 //uint8_t rcv_isr = 0; 00011 00012 int count = 0; 00013 00014 char PL_I2C_DATA[134];//Payload i2c array 00015 uint8_t PL_TM_SIZE;//size of data to bev read from i2c 00016 uint32_t pdirr1; 00017 uint32_t pdirw1; 00018 00019 void I2C_busreset() 00020 { 00021 PORTE->PCR[1] &= 0xfffffffb; //Enabling high slew rates for SDA and SCL lines 00022 PORTE->PCR[0] &= 0xfffffffb; //Enabling high slew rates for SDA and SCL lines 00023 I2C0->C1 &= 0x7f; //Disabling I2C module 00024 SIM->SCGC4 &= 0xffffffbf; //Disabling clock to I2C module 00025 SIM->SCGC4 |= 0x00000040; //Enabling clock to I2C module 00026 I2C0->C1 |= 0x80; //Enabling I2C module 00027 PORTE->PCR[1] |= 0x00000004; //Disabling high slew rates for SDA and SCL lines 00028 PORTE->PCR[0] |= 0x00000004; //Disabling high slew rates for SDA and SCL lines 00029 Thread::wait(1); //Wait for all I2C registers to be updates to their their values 00030 } 00031 bool FCTN_I2C_READ_PL(char *data,int length) // Returns 0 for success 00032 { 00033 PL_I2C_GPIO = 1; 00034 read_ack = master.read(addr_pl|1,data,length); 00035 Thread::wait(1); //as per tests Thread::wait not required on master side. But its safe to give 1ms 00036 pdirr1=PTE->PDIR; 00037 uint8_t i2c_count = 0; 00038 if(read_ack == 0) //if read_ack says success, it may or may not be successful.Hence we check SCL and SDA 00039 { 00040 while(((pdirr1 & 0x03000000)!=0x03000000)&& i2c_count<10)//checking SCL and SDA for time=10ms 00041 { 00042 Thread::wait(1); 00043 pdirr1=PTE->PDIR; 00044 i2c_count++; 00045 } 00046 if(((pdirr1 & 0x03000000)==0x03000000))//if SCL and SDA are both high 00047 { 00048 gPC.printf("\n\rData received from PL"); 00049 } 00050 else 00051 { 00052 I2C_busreset(); 00053 read_ack = 1; 00054 } 00055 } 00056 else if (read_ack == 1) 00057 { 00058 CDMS_I2C_ERR_SPEED_COUNTER++; 00059 I2C_busreset(); 00060 } 00061 PL_I2C_GPIO = 0; 00062 i2c_count = 0; 00063 return read_ack; 00064 00065 } 00066 bool FCTN_I2C_WRITE_PL(char *data2,uint8_t tc_len2) // Returns 0 for success 00067 { 00068 write_ack = master.write(addr_pl|0x00,data2,tc_len2);//address to be defined in payload 00069 Thread::wait(1); //As per the tests Thread::wait is not required on master side but its safe to give 1ms 00070 pdirw1=PTE->PDIR; 00071 uint8_t i2c_count = 0; 00072 if(write_ack == 0) 00073 { 00074 while(((pdirw1 & 0x03000000)!=0x03000000)&& i2c_count<10) 00075 { 00076 Thread::wait(1); 00077 pdirw1=PTE->PDIR; 00078 i2c_count++; 00079 } 00080 if(((pdirw1 & 0x03000000)==0x03000000)) 00081 { 00082 gPC.printf("\n\r Data sent"); 00083 } 00084 else 00085 { 00086 I2C_busreset(); 00087 write_ack = 1; 00088 } 00089 } 00090 if (write_ack == 1) 00091 { 00092 I2C_busreset(); 00093 CDMS_I2C_ERR_SPEED_COUNTER++; 00094 } 00095 i2c_count = 0; 00096 return write_ack; 00097 } 00098 bool FCTN_I2C_READ(char *data,int length) // Returns 0 for success 00099 { 00100 CDMS_I2C_GPIO = 1; 00101 read_ack = master.read(addr_bae|1,data,length); 00102 Thread::wait(1); //as per tests Thread::wait not required on master side. But its safe to give 1ms 00103 pdirr1=PTE->PDIR; 00104 uint8_t i2c_count = 0; 00105 if(read_ack == 0) //if read_ack says success, it may or may not be successful.Hence we check SCL and SDA 00106 { 00107 while(((pdirr1 & 0x03000000)!=0x03000000)&& i2c_count<10)//checking SCL and SDA for time=10ms 00108 { 00109 Thread::wait(1); 00110 pdirr1=PTE->PDIR; 00111 i2c_count++; 00112 } 00113 if(((pdirr1 & 0x03000000)==0x03000000))//if SCL and SDA are both high 00114 { 00115 gPC.printf("\n\rData received from BAE"); 00116 } 00117 else 00118 { 00119 I2C_busreset(); 00120 read_ack = 1; 00121 } 00122 } 00123 else if (read_ack == 1) 00124 { 00125 I2C_busreset(); 00126 CDMS_I2C_ERR_BAE_COUNTER++; 00127 00128 } 00129 CDMS_I2C_GPIO = 0; 00130 i2c_count = 0; 00131 return read_ack; 00132 } 00133 00134 bool FCTN_I2C_WRITE(char *data,int tc_len2) // Returns 0 for success 00135 { 00136 CDMS_I2C_GPIO = 1; 00137 write_ack = master.write(addr_bae|0x00,data,tc_len2); 00138 Thread::wait(1); //As per the tests Thread::wait is not required on master side but its safe to give 1ms 00139 pdirw1=PTE->PDIR; 00140 uint8_t i2c_count = 0; 00141 if(write_ack == 0) 00142 { 00143 while(((pdirw1 & 0x03000000)!=0x03000000)&& i2c_count<10) 00144 { 00145 Thread::wait(1); 00146 pdirw1=PTE->PDIR; 00147 i2c_count++; 00148 } 00149 if(((pdirw1 & 0x03000000)==0x03000000)) 00150 { 00151 gPC.printf("\n\r Data sent"); 00152 } 00153 else 00154 { 00155 I2C_busreset(); 00156 write_ack = 1; 00157 } 00158 } 00159 if (write_ack == 1) 00160 { 00161 I2C_busreset(); 00162 CDMS_I2C_ERR_BAE_COUNTER++; 00163 } 00164 CDMS_I2C_GPIO = 0; 00165 i2c_count = 0; 00166 return write_ack; 00167 }
Generated on Sat Jul 16 2022 01:27:53 by
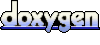