Attempting to publish a tree
Dependencies: BLE_API mbed-dev-bin nRF51822
Fork of microbit-dal by
MicroBitPin Class Reference
Class definition for MicroBitPin. More...
#include <MicroBitPin.h>
Inherits MicroBitComponent.
Public Member Functions | |
MicroBitPin (int id, PinName name, PinCapability capability) | |
Constructor. | |
int | setDigitalValue (int value) |
Configures this IO pin as a digital output (if necessary) and sets the pin to 'value'. | |
int | getDigitalValue () |
Configures this IO pin as a digital input (if necessary) and tests its current value. | |
int | setAnalogValue (int value) |
Configures this IO pin as an analog/pwm output, and change the output value to the given level. | |
int | setServoValue (int value, int range=MICROBIT_PIN_DEFAULT_SERVO_RANGE, int center=MICROBIT_PIN_DEFAULT_SERVO_CENTER) |
Configures this IO pin as an analog/pwm output (if necessary) and configures the period to be 20ms, with a duty cycle between 500 us and 2500 us. | |
int | getAnalogValue () |
Configures this IO pin as an analogue input (if necessary), and samples the Pin for its analog value. | |
int | isInput () |
Determines if this IO pin is currently configured as an input. | |
int | isOutput () |
Determines if this IO pin is currently configured as an output. | |
int | isDigital () |
Determines if this IO pin is currently configured for digital use. | |
int | isAnalog () |
Determines if this IO pin is currently configured for analog use. | |
int | isTouched () |
Configures this IO pin as a "makey makey" style touch sensor (if necessary) and tests its current debounced state. | |
int | setServoPulseUs (int pulseWidth) |
Configures this IO pin as an analog/pwm output if it isn't already, configures the period to be 20ms, and sets the pulse width, based on the value it is given. | |
int | setAnalogPeriod (int period) |
Configures the PWM period of the analog output to the given value. | |
int | setAnalogPeriodUs (int period) |
Configures the PWM period of the analog output to the given value. | |
int | getAnalogPeriodUs () |
Obtains the PWM period of the analog output in microseconds. | |
int | getAnalogPeriod () |
Obtains the PWM period of the analog output in milliseconds. | |
virtual void | systemTick () |
The system timer will call this member function once the component has been added to the array of system components using system_timer_add_component. | |
virtual void | idleTick () |
The idle thread will call this member function once the component has been added to the array of idle components using fiber_add_idle_component. | |
virtual int | isIdleCallbackNeeded () |
When added to the idleThreadComponents array, this function will be called to determine if and when data is ready. |
Detailed Description
Class definition for MicroBitPin.
Commonly represents an I/O pin on the edge connector.
Definition at line 86 of file MicroBitPin.h.
Constructor & Destructor Documentation
MicroBitPin | ( | int | id, |
PinName | name, | ||
PinCapability | capability | ||
) |
Constructor.
Class definition for MicroBitPin.
Create a MicroBitPin instance, generally used to represent a pin on the edge connector.
- Parameters:
-
id the unique EventModel id of this component. name the mbed PinName for this MicroBitPin instance. capability the capabilities this MicroBitPin instance should have. (PIN_CAPABILITY_DIGITAL, PIN_CAPABILITY_ANALOG, PIN_CAPABILITY_TOUCH, PIN_CAPABILITY_AD, PIN_CAPABILITY_ALL)
MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_ALL);
Commonly represents an I/O pin on the edge connector. Constructor. Create a MicroBitPin instance, generally used to represent a pin on the edge connector.
- Parameters:
-
id the unique EventModel id of this component. name the mbed PinName for this MicroBitPin instance. capability the capabilities this MicroBitPin instance should have. (PIN_CAPABILITY_DIGITAL, PIN_CAPABILITY_ANALOG, PIN_CAPABILITY_TOUCH, PIN_CAPABILITY_AD, PIN_CAPABILITY_ALL)
MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_ALL);
Definition at line 52 of file MicroBitPin.cpp.
Member Function Documentation
int getAnalogPeriod | ( | ) |
Obtains the PWM period of the analog output in milliseconds.
- Returns:
- the period on success, or MICROBIT_NOT_SUPPORTED if the given pin is not configured as an analog output.
Definition at line 429 of file MicroBitPin.cpp.
int getAnalogPeriodUs | ( | ) |
Obtains the PWM period of the analog output in microseconds.
- Returns:
- the period on success, or MICROBIT_NOT_SUPPORTED if the given pin is not configured as an analog output.
Definition at line 415 of file MicroBitPin.cpp.
int getAnalogValue | ( | ) |
Configures this IO pin as an analogue input (if necessary), and samples the Pin for its analog value.
- Returns:
- the current analogue level on the pin, in the range 0 - 1024, or MICROBIT_NOT_SUPPORTED if the given pin does not have analog capability.
MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_BOTH); P0.getAnalogValue(); // P0 is a value in the range of 0 - 1024
Definition at line 254 of file MicroBitPin.cpp.
int getDigitalValue | ( | ) |
Configures this IO pin as a digital input (if necessary) and tests its current value.
- Returns:
- 1 if this input is high, 0 if input is LO, or MICROBIT_NOT_SUPPORTED if the given pin does not have analog capability.
MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_BOTH); P0.getDigitalValue(); // P0 is either 0 or 1;
Definition at line 146 of file MicroBitPin.cpp.
virtual void idleTick | ( | ) | [virtual, inherited] |
The idle thread will call this member function once the component has been added to the array of idle components using fiber_add_idle_component.
Updates are determined by the isIdleCallbackNeeded() member function.
Reimplemented in MicroBitBLEManager, MicroBitEventService, MicroBitIOPinService, MicroBitAccelerometer, MicroBitCompass, and MicroBitThermometer.
Definition at line 127 of file MicroBitComponent.h.
int isAnalog | ( | ) |
Determines if this IO pin is currently configured for analog use.
- Returns:
- 1 if pin is analog, 0 otherwise.
Definition at line 306 of file MicroBitPin.cpp.
int isDigital | ( | ) |
Determines if this IO pin is currently configured for digital use.
- Returns:
- 1 if pin is digital, 0 otherwise.
Definition at line 296 of file MicroBitPin.cpp.
virtual int isIdleCallbackNeeded | ( | ) | [virtual, inherited] |
When added to the idleThreadComponents array, this function will be called to determine if and when data is ready.
- Note:
- override this if you want to request to be scheduled as soon as possible.
Reimplemented in MicroBitAccelerometer, MicroBitCompass, and MicroBitThermometer.
Definition at line 138 of file MicroBitComponent.h.
int isInput | ( | ) |
Determines if this IO pin is currently configured as an input.
- Returns:
- 1 if pin is an analog or digital input, 0 otherwise.
Definition at line 276 of file MicroBitPin.cpp.
int isOutput | ( | ) |
Determines if this IO pin is currently configured as an output.
- Returns:
- 1 if pin is an analog or digital output, 0 otherwise.
Definition at line 286 of file MicroBitPin.cpp.
int isTouched | ( | ) |
Configures this IO pin as a "makey makey" style touch sensor (if necessary) and tests its current debounced state.
Users can also subscribe to MicroBitButton events generated from this pin.
- Returns:
- 1 if pin is touched, 0 if not, or MICROBIT_NOT_SUPPORTED if this pin does not support touch capability.
MicroBitMessageBus bus; MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_ALL); if(P0.isTouched()) { //do something! } // subscribe to events generated by this pin! bus.listen(MICROBIT_ID_IO_P0, MICROBIT_BUTTON_EVT_CLICK, someFunction);
Definition at line 332 of file MicroBitPin.cpp.
int setAnalogPeriod | ( | int | period ) |
Configures the PWM period of the analog output to the given value.
- Parameters:
-
period The new period for the analog output in milliseconds.
- Returns:
- MICROBIT_OK on success, or MICROBIT_NOT_SUPPORTED if the given pin is not configured as an analog output.
Definition at line 404 of file MicroBitPin.cpp.
int setAnalogPeriodUs | ( | int | period ) |
Configures the PWM period of the analog output to the given value.
- Parameters:
-
period The new period for the analog output in microseconds.
- Returns:
- MICROBIT_OK on success, or MICROBIT_NOT_SUPPORTED if the given pin is not configured as an analog output.
Definition at line 388 of file MicroBitPin.cpp.
int setAnalogValue | ( | int | value ) |
Configures this IO pin as an analog/pwm output, and change the output value to the given level.
- Parameters:
-
value the level to set on the output pin, in the range 0 - 1024
- Returns:
- MICROBIT_OK on success, MICROBIT_INVALID_PARAMETER if value is out of range, or MICROBIT_NOT_SUPPORTED if the given pin does not have analog capability.
Definition at line 182 of file MicroBitPin.cpp.
int setDigitalValue | ( | int | value ) |
Configures this IO pin as a digital output (if necessary) and sets the pin to 'value'.
- Parameters:
-
value 0 (LO) or 1 (HI)
- Returns:
- MICROBIT_OK on success, MICROBIT_INVALID_PARAMETER if value is out of range, or MICROBIT_NOT_SUPPORTED if the given pin does not have digital capability.
MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_BOTH); P0.setDigitalValue(1); // P0 is now HI
Definition at line 112 of file MicroBitPin.cpp.
int setServoPulseUs | ( | int | pulseWidth ) |
Configures this IO pin as an analog/pwm output if it isn't already, configures the period to be 20ms, and sets the pulse width, based on the value it is given.
- Parameters:
-
pulseWidth the desired pulse width in microseconds.
- Returns:
- MICROBIT_OK on success, MICROBIT_INVALID_PARAMETER if value is out of range, or MICROBIT_NOT_SUPPORTED if the given pin does not have analog capability.
Definition at line 357 of file MicroBitPin.cpp.
int setServoValue | ( | int | value, |
int | range = MICROBIT_PIN_DEFAULT_SERVO_RANGE , |
||
int | center = MICROBIT_PIN_DEFAULT_SERVO_CENTER |
||
) |
Configures this IO pin as an analog/pwm output (if necessary) and configures the period to be 20ms, with a duty cycle between 500 us and 2500 us.
A value of 180 sets the duty cycle to be 2500us, and a value of 0 sets the duty cycle to be 500us by default.
This range can be modified to fine tune, and also tolerate different servos.
- Parameters:
-
value the level to set on the output pin, in the range 0 - 180. range which gives the span of possible values the i.e. the lower and upper bounds (center +/- range/2). Defaults to MICROBIT_PIN_DEFAULT_SERVO_RANGE. center the center point from which to calculate the lower and upper bounds. Defaults to MICROBIT_PIN_DEFAULT_SERVO_CENTER
- Returns:
- MICROBIT_OK on success, MICROBIT_INVALID_PARAMETER if value is out of range, or MICROBIT_NOT_SUPPORTED if the given pin does not have analog capability.
Definition at line 218 of file MicroBitPin.cpp.
virtual void systemTick | ( | ) | [virtual, inherited] |
The system timer will call this member function once the component has been added to the array of system components using system_timer_add_component.
This callback will be in interrupt context.
Reimplemented in MicroBitSystemTimerCallback, MicroBitButton, and MicroBitDisplay.
Definition at line 118 of file MicroBitComponent.h.
Generated on Tue Jul 12 2022 19:58:10 by
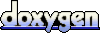