Attempting to publish a tree
Dependencies: BLE_API mbed-dev-bin nRF51822
Fork of microbit-dal by
MicroBitCompass Class Reference
Class definition for MicroBit Compass. More...
#include <MicroBitCompass.h>
Inherits MicroBitComponent.
Public Member Functions | |
MicroBitCompass (MicroBitI2C &_i2c, MicroBitAccelerometer &_accelerometer, MicroBitStorage &_storage, uint16_t address=MAG3110_DEFAULT_ADDR, uint16_t id=MICROBIT_ID_COMPASS) | |
Constructor. | |
MicroBitCompass (MicroBitI2C &_i2c, MicroBitAccelerometer &_accelerometer, uint16_t address=MAG3110_DEFAULT_ADDR, uint16_t id=MICROBIT_ID_COMPASS) | |
Constructor. | |
MicroBitCompass (MicroBitI2C &_i2c, MicroBitStorage &_storage, uint16_t address=MAG3110_DEFAULT_ADDR, uint16_t id=MICROBIT_ID_COMPASS) | |
Constructor. | |
MicroBitCompass (MicroBitI2C &_i2c, uint16_t address=MAG3110_DEFAULT_ADDR, uint16_t id=MICROBIT_ID_COMPASS) | |
Constructor. | |
int | configure () |
Configures the compass for the sample rate defined in this object. | |
int | setPeriod (int period) |
Attempts to set the sample rate of the compass to the specified value (in ms). | |
int | getPeriod () |
Reads the currently configured sample rate of the compass. | |
int | heading () |
Gets the current heading of the device, relative to magnetic north. | |
int | whoAmI () |
Attempts to read the 8 bit ID from the magnetometer, this can be used for validation purposes. | |
int | getX (MicroBitCoordinateSystem system=SIMPLE_CARTESIAN) |
Reads the value of the X axis from the latest update retrieved from the magnetometer. | |
int | getY (MicroBitCoordinateSystem system=SIMPLE_CARTESIAN) |
Reads the value of the Y axis from the latest update retrieved from the magnetometer. | |
int | getZ (MicroBitCoordinateSystem system=SIMPLE_CARTESIAN) |
Reads the value of the Z axis from the latest update retrieved from the magnetometer. | |
int | getFieldStrength () |
Determines the overall magnetic field strength based on the latest update from the magnetometer. | |
int | readTemperature () |
Reads the current die temperature of the compass. | |
int | calibrate () |
Perform a calibration of the compass. | |
void | setCalibration (CompassSample calibration) |
Configure the compass to use the calibration data that is supplied to this call. | |
CompassSample | getCalibration () |
Provides the calibration data currently in use by the compass. | |
int | updateSample () |
Updates the local sample, only if the compass indicates that data is stale. | |
virtual void | idleTick () |
Periodic callback from MicroBit idle thread. | |
int | isCalibrated () |
Returns 0 or 1. | |
int | isCalibrating () |
Returns 0 or 1. | |
void | clearCalibration () |
Clears the calibration held in persistent storage, and sets the calibrated flag to zero. | |
virtual int | isIdleCallbackNeeded () |
Returns 0 or 1. | |
~MicroBitCompass () | |
Destructor for MicroBitCompass, where we deregister this instance from the array of fiber components. | |
virtual void | systemTick () |
The system timer will call this member function once the component has been added to the array of system components using system_timer_add_component. |
Detailed Description
Class definition for MicroBit Compass.
Represents an implementation of the Freescale MAG3110 I2C Magnetmometer. Also includes basic caching, calibration and on demand activation.
Definition at line 147 of file MicroBitCompass.h.
Constructor & Destructor Documentation
MicroBitCompass | ( | MicroBitI2C & | _i2c, |
MicroBitAccelerometer & | _accelerometer, | ||
MicroBitStorage & | _storage, | ||
uint16_t | address = MAG3110_DEFAULT_ADDR , |
||
uint16_t | id = MICROBIT_ID_COMPASS |
||
) |
Constructor.
Create a software representation of an e-compass.
- Parameters:
-
_i2c an instance of i2c, which the compass is accessible from. _accelerometer an instance of the accelerometer, used for tilt compensation. _storage an instance of MicroBitStorage, used to persist calibration data across resets. address the default address for the compass register on the i2c bus. Defaults to MAG3110_DEFAULT_ADDR. id the ID of the new MicroBitCompass object. Defaults to MAG3110_DEFAULT_ADDR.
MicroBitI2C i2c(I2C_SDA0, I2C_SCL0); MicroBitAccelerometer accelerometer(i2c); MicroBitStorage storage; MicroBitCompass compass(i2c, accelerometer, storage);
Definition at line 100 of file MicroBitCompass.cpp.
MicroBitCompass | ( | MicroBitI2C & | _i2c, |
MicroBitAccelerometer & | _accelerometer, | ||
uint16_t | address = MAG3110_DEFAULT_ADDR , |
||
uint16_t | id = MICROBIT_ID_COMPASS |
||
) |
Constructor.
Create a software representation of an e-compass.
- Parameters:
-
_i2c an instance of i2c, which the compass is accessible from. _accelerometer an instance of the accelerometer, used for tilt compensation. address the default address for the compass register on the i2c bus. Defaults to MAG3110_DEFAULT_ADDR. id the ID of the new MicroBitCompass object. Defaults to MAG3110_DEFAULT_ADDR.
MicroBitI2C i2c(I2C_SDA0, I2C_SCL0); MicroBitAccelerometer accelerometer(i2c); MicroBitCompass compass(i2c, accelerometer, storage);
Definition at line 131 of file MicroBitCompass.cpp.
MicroBitCompass | ( | MicroBitI2C & | _i2c, |
MicroBitStorage & | _storage, | ||
uint16_t | address = MAG3110_DEFAULT_ADDR , |
||
uint16_t | id = MICROBIT_ID_COMPASS |
||
) |
Constructor.
Create a software representation of an e-compass.
- Parameters:
-
_i2c an instance of i2c, which the compass is accessible from. _storage an instance of MicroBitStorage, used to persist calibration data across resets. address the default address for the compass register on the i2c bus. Defaults to MAG3110_DEFAULT_ADDR. id the ID of the new MicroBitCompass object. Defaults to MAG3110_DEFAULT_ADDR.
MicroBitI2C i2c(I2C_SDA0, I2C_SCL0); MicroBitStorage storage; MicroBitCompass compass(i2c, storage);
Definition at line 162 of file MicroBitCompass.cpp.
MicroBitCompass | ( | MicroBitI2C & | _i2c, |
uint16_t | address = MAG3110_DEFAULT_ADDR , |
||
uint16_t | id = MICROBIT_ID_COMPASS |
||
) |
Constructor.
Create a software representation of an e-compass.
- Parameters:
-
_i2c an instance of i2c, which the compass is accessible from. address the default address for the compass register on the i2c bus. Defaults to MAG3110_DEFAULT_ADDR. id the ID of the new MicroBitCompass object. Defaults to MAG3110_DEFAULT_ADDR.
MicroBitI2C i2c(I2C_SDA0, I2C_SCL0); MicroBitCompass compass(i2c);
Definition at line 189 of file MicroBitCompass.cpp.
~MicroBitCompass | ( | ) |
Destructor for MicroBitCompass, where we deregister this instance from the array of fiber components.
Definition at line 761 of file MicroBitCompass.cpp.
Member Function Documentation
int calibrate | ( | ) |
Perform a calibration of the compass.
This method will be called automatically if a user attempts to read a compass value when the compass is uncalibrated. It can also be called at any time by the user.
The method will only return once the compass has been calibrated.
- Returns:
- MICROBIT_OK, MICROBIT_I2C_ERROR if the magnetometer could not be accessed, or MICROBIT_CALIBRATION_REQUIRED if the calibration algorithm failed to complete successfully.
- Note:
- THIS MUST BE CALLED TO GAIN RELIABLE VALUES FROM THE COMPASS
Definition at line 666 of file MicroBitCompass.cpp.
void clearCalibration | ( | ) |
Clears the calibration held in persistent storage, and sets the calibrated flag to zero.
Definition at line 743 of file MicroBitCompass.cpp.
int configure | ( | ) |
Configures the compass for the sample rate defined in this object.
The nearest values are chosen to those defined that are supported by the hardware. The instance variables are then updated to reflect reality.
- Returns:
- MICROBIT_OK or MICROBIT_I2C_ERROR if the magnetometer could not be configured.
Definition at line 528 of file MicroBitCompass.cpp.
CompassSample getCalibration | ( | ) |
Provides the calibration data currently in use by the compass.
More specifically, the x, y and z zero offsets of the compass.
- Returns:
- calibration A CompassSample containing the offsets for the x, y and z axis.
Definition at line 719 of file MicroBitCompass.cpp.
int getFieldStrength | ( | ) |
Determines the overall magnetic field strength based on the latest update from the magnetometer.
- Returns:
- The magnetic force measured across all axis, in nano teslas.
compass.getFieldStrength();
Definition at line 512 of file MicroBitCompass.cpp.
int getPeriod | ( | ) |
Reads the currently configured sample rate of the compass.
- Returns:
- The time between samples, in milliseconds.
Definition at line 608 of file MicroBitCompass.cpp.
int getX | ( | MicroBitCoordinateSystem | system = SIMPLE_CARTESIAN ) |
Reads the value of the X axis from the latest update retrieved from the magnetometer.
- Parameters:
-
system The coordinate system to use. By default, a simple cartesian system is provided.
- Returns:
- The magnetic force measured in the X axis, in nano teslas.
compass.getX();
Definition at line 429 of file MicroBitCompass.cpp.
int getY | ( | MicroBitCoordinateSystem | system = SIMPLE_CARTESIAN ) |
Reads the value of the Y axis from the latest update retrieved from the magnetometer.
- Parameters:
-
system The coordinate system to use. By default, a simple cartesian system is provided.
- Returns:
- The magnetic force measured in the Y axis, in nano teslas.
compass.getY();
Definition at line 458 of file MicroBitCompass.cpp.
int getZ | ( | MicroBitCoordinateSystem | system = SIMPLE_CARTESIAN ) |
Reads the value of the Z axis from the latest update retrieved from the magnetometer.
- Parameters:
-
system The coordinate system to use. By default, a simple cartesian system is provided.
- Returns:
- The magnetic force measured in the Z axis, in nano teslas.
compass.getZ();
Definition at line 487 of file MicroBitCompass.cpp.
int heading | ( | ) |
Gets the current heading of the device, relative to magnetic north.
If the compass is not calibrated, it will raise the MICROBIT_COMPASS_EVT_CALIBRATE event.
Users wishing to implement their own calibration algorithms should listen for this event, using MESSAGE_BUS_LISTENER_IMMEDIATE model. This ensures that calibration is complete before the user program continues.
- Returns:
- the current heading, in degrees. Or MICROBIT_CALIBRATION_IN_PROGRESS if the compass is calibrating.
compass.heading();
Definition at line 360 of file MicroBitCompass.cpp.
void idleTick | ( | ) | [virtual] |
Periodic callback from MicroBit idle thread.
Calls updateSample().
Reimplemented from MicroBitComponent.
Definition at line 413 of file MicroBitCompass.cpp.
int isCalibrated | ( | ) |
Returns 0 or 1.
1 indicates that the compass is calibrated, zero means the compass requires calibration.
Definition at line 727 of file MicroBitCompass.cpp.
int isCalibrating | ( | ) |
Returns 0 or 1.
1 indicates that the compass is calibrating, zero means the compass is not currently calibrating.
Definition at line 735 of file MicroBitCompass.cpp.
int isIdleCallbackNeeded | ( | ) | [virtual] |
Returns 0 or 1.
1 indicates data is waiting to be read, zero means data is not ready to be read.
Reimplemented from MicroBitComponent.
Definition at line 751 of file MicroBitCompass.cpp.
int readTemperature | ( | ) |
Reads the current die temperature of the compass.
- Returns:
- the temperature in degrees celsius, or MICROBIT_I2C_ERROR if the temperature reading could not be retreived from the accelerometer.
Definition at line 641 of file MicroBitCompass.cpp.
void setCalibration | ( | CompassSample | calibration ) |
Configure the compass to use the calibration data that is supplied to this call.
Calibration data is comprised of the perceived zero offset of each axis of the compass.
After calibration this should now take into account trimming errors in the magnetometer, and any "hard iron" offsets on the device.
- Parameters:
-
calibration A CompassSample containing the offsets for the x, y and z axis.
Definition at line 703 of file MicroBitCompass.cpp.
int setPeriod | ( | int | period ) |
Attempts to set the sample rate of the compass to the specified value (in ms).
- Parameters:
-
period the requested time between samples, in milliseconds.
- Returns:
- MICROBIT_OK or MICROBIT_I2C_ERROR if the magnetometer could not be updated.
// sample rate is now 20 ms.
compass.setPeriod(20);
- Note:
- The requested rate may not be possible on the hardware. In this case, the nearest lower rate is chosen.
Definition at line 597 of file MicroBitCompass.cpp.
virtual void systemTick | ( | ) | [virtual, inherited] |
The system timer will call this member function once the component has been added to the array of system components using system_timer_add_component.
This callback will be in interrupt context.
Reimplemented in MicroBitSystemTimerCallback, MicroBitButton, and MicroBitDisplay.
Definition at line 118 of file MicroBitComponent.h.
int updateSample | ( | ) |
Updates the local sample, only if the compass indicates that data is stale.
- Note:
- Can be used to trigger manual updates, if the device is running without a scheduler. Also called internally by all get[X,Y,Z]() member functions.
Adds the compass to idle, if it hasn't been added already. This is an optimisation so that the compass is only added on first 'use'.
Definition at line 381 of file MicroBitCompass.cpp.
int whoAmI | ( | ) |
Attempts to read the 8 bit ID from the magnetometer, this can be used for validation purposes.
- Returns:
- the 8 bit ID returned by the magnetometer, or MICROBIT_I2C_ERROR if the request fails.
compass.whoAmI();
Definition at line 623 of file MicroBitCompass.cpp.
Generated on Tue Jul 12 2022 19:58:10 by
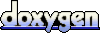