Attempting to publish a tree
Dependencies: BLE_API mbed-dev-bin nRF51822
Fork of microbit-dal by
MicroBitStorage Class Reference
Class definition for the MicroBitStorage class. More...
#include <MicroBitStorage.h>
Public Member Functions | |
MicroBitStorage () | |
Default constructor. | |
int | writeBytes (uint8_t *buffer, uint32_t address, int length) |
Writes the given number of bytes to the address specified. | |
void | flashPageErase (uint32_t *page_address) |
Method for erasing a page in flash. | |
void | flashWordWrite (uint32_t *address, uint32_t value) |
Method for writing a word of data in flash with a value. | |
int | put (const char *key, uint8_t *data) |
Places a given key, and it's corresponding value into flash at the earliest available point. | |
int | put (ManagedString key, uint8_t *data) |
Places a given key, and it's corresponding value into flash at the earliest available point. | |
KeyValuePair * | get (const char *key) |
Retreives a KeyValuePair identified by a given key. | |
KeyValuePair * | get (ManagedString key) |
Retreives a KeyValuePair identified by a given key. | |
int | remove (const char *key) |
Removes a KeyValuePair identified by a given key. | |
int | remove (ManagedString key) |
Removes a KeyValuePair identified by a given key. | |
int | size () |
The size of the flash based KeyValueStore. |
Detailed Description
Class definition for the MicroBitStorage class.
This allows reading and writing of small blocks of data to FLASH memory.
This class operates as a key value store, it allows the retrieval, addition and deletion of KeyValuePairs.
The first 8 bytes are reserved for the KeyValueStore struct which gives core information such as the number of KeyValuePairs in the store, and whether the store has been initialised.
After the KeyValueStore struct, KeyValuePairs are arranged contiguously until the end of the block used as persistent storage.
|-------8-------|--------48-------|-----|---------48--------| | KeyValueStore | KeyValuePair[0] | ... | KeyValuePair[N-1] | |---------------|-----------------|-----|-------------------|
Definition at line 86 of file MicroBitStorage.h.
Constructor & Destructor Documentation
MicroBitStorage | ( | ) |
Default constructor.
Class definition for the MicroBitStorage class.
Creates an instance of MicroBitStorage which acts like a KeyValueStore that allows the retrieval, addition and deletion of KeyValuePairs.
This allows reading and writing of FLASH memory. Default constructor.
Creates an instance of MicroBitStorage which acts like a KeyValueStore that allows the retrieval, addition and deletion of KeyValuePairs.
Definition at line 41 of file MicroBitStorage.cpp.
Member Function Documentation
void flashPageErase | ( | uint32_t * | page_address ) |
Method for erasing a page in flash.
- Parameters:
-
page_address Address of the first word in the page to be erased.
Definition at line 72 of file MicroBitStorage.cpp.
void flashWordWrite | ( | uint32_t * | address, |
uint32_t | value | ||
) |
Method for writing a word of data in flash with a value.
- Parameters:
-
address Address of the word to change. value Value to be written to flash.
Definition at line 124 of file MicroBitStorage.cpp.
KeyValuePair * get | ( | const char * | key ) |
Retreives a KeyValuePair identified by a given key.
- Parameters:
-
key the unique name used to identify a KeyValuePair in flash.
- Returns:
- a pointer to a heap allocated KeyValuePair struct, this pointer will be NULL if the key was not found in storage.
- Note:
- it is up to the user to free memory after use.
Definition at line 310 of file MicroBitStorage.cpp.
KeyValuePair * get | ( | ManagedString | key ) |
Retreives a KeyValuePair identified by a given key.
- Parameters:
-
key the unique name used to identify a KeyValuePair in flash.
- Returns:
- a pointer to a heap allocated KeyValuePair struct, this pointer will be NULL if the key was not found in storage.
- Note:
- it is up to the user to free memory after use.
Definition at line 362 of file MicroBitStorage.cpp.
int put | ( | const char * | key, |
uint8_t * | data | ||
) |
Places a given key, and it's corresponding value into flash at the earliest available point.
- Parameters:
-
key the unique name that should be used as an identifier for the given data. The key is presumed to be null terminated. data a pointer to the beginning of the data to be persisted.
- Returns:
- MICROBIT_OK on success, or MICROBIT_NO_RESOURCES if the storage page is full
Definition at line 214 of file MicroBitStorage.cpp.
int put | ( | ManagedString | key, |
uint8_t * | data | ||
) |
Places a given key, and it's corresponding value into flash at the earliest available point.
- Parameters:
-
key the unique name that should be used as an identifier for the given data. data a pointer to the beginning of the data to be persisted.
- Returns:
- MICROBIT_OK on success, or MICROBIT_NO_RESOURCES if the storage page is full
Definition at line 295 of file MicroBitStorage.cpp.
int remove | ( | const char * | key ) |
Removes a KeyValuePair identified by a given key.
- Parameters:
-
key the unique name used to identify a KeyValuePair in flash.
- Returns:
- MICROBIT_OK on success, or MICROBIT_NO_DATA if the given key was not found in flash.
Definition at line 375 of file MicroBitStorage.cpp.
int remove | ( | ManagedString | key ) |
Removes a KeyValuePair identified by a given key.
- Parameters:
-
key the unique name used to identify a KeyValuePair in flash.
- Returns:
- MICROBIT_OK on success, or MICROBIT_NO_DATA if the given key was not found in flash.
Definition at line 447 of file MicroBitStorage.cpp.
int size | ( | ) |
The size of the flash based KeyValueStore.
- Returns:
- the number of entries in the key value store
Definition at line 457 of file MicroBitStorage.cpp.
int writeBytes | ( | uint8_t * | buffer, |
uint32_t | address, | ||
int | length | ||
) |
Writes the given number of bytes to the address specified.
- Parameters:
-
buffer the data to write. address the location in memory to write to. length the number of bytes to write.
- Note:
- currently not implemented.
Definition at line 58 of file MicroBitStorage.cpp.
Generated on Tue Jul 12 2022 19:58:10 by
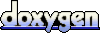