Christian Dupaty 03/2021 Library and demo for BMM150 see datasheet here : https://www.bosch-sensortec.com/products/motion-sensors/magnetometers-bmm150/ Adaptation of BOSCH driver https://github.com/BoschSensortec/BMM150-Sensor-API for ARM MBED and tested on NUCLEO-L073RZ and GEOMAGNETIC CLICK https://www.mikroe.com/geomagnetic-click
Dependents: BMM150_HelloWorld2
BMM150 Class Reference
Includes. More...
#include <bmm150.h>
Public Member Functions | |
int8_t | initialize (void) |
initialze device | |
void | read_mag_data () |
Read magnetometer data. | |
int16_t | compensate_x (int16_t mag_data_z, uint16_t data_rhall) |
This internal API is used to obtain the compensated magnetometer x axis data(micro-tesla) in float. | |
int16_t | compensate_y (int16_t mag_data_z, uint16_t data_rhall) |
This internal API is used to obtain the compensated magnetometer Y axis data(micro-tesla) in int. | |
int16_t | compensate_z (int16_t mag_data_z, uint16_t data_rhall) |
This internal API is used to obtain the compensated magnetometer Z axis data(micro-tesla) in int. | |
void | set_op_mode (uint8_t op_mode) |
Set power mode. | |
void | read_trim_registers () |
This internal API reads the trim registers of the sensor and stores the trim values in the "trim_data" of device structure. | |
void | write_op_mode (uint8_t op_mode) |
This internal API writes the op_mode value in the Opmode bits (bits 1 and 2) of 0x4C register. | |
void | set_preset_mode (uint8_t mode) |
Set preset mode mode. | |
void | set_power_control_bit (uint8_t pwrcntrl_bit) |
This internal API sets/resets the power control bit of 0x4B register. | |
void | suspend_to_sleep_mode () |
This internal API sets the device from suspend to sleep mode by setting the power control bit to '1' of 0x4B register. | |
void | set_presetmode (uint8_t preset_mode) |
This API is used to set the preset mode of the sensor. | |
void | set_odr_xyz_rep (struct bmm150_settings settings) |
Self test functionality. | |
void | set_xy_rep (struct bmm150_settings settings) |
This internal API sets the xy repetition value in the 0x51 register. | |
void | set_z_rep (struct bmm150_settings settings) |
This internal API sets the z repetition value in the 0x52 register. | |
void | set_odr (struct bmm150_settings settings) |
This internal API is used to set the output data rate of the sensor. | |
void | soft_reset () |
This API is used to perform soft-reset of the sensor where all the registers are reset to their default values except 0x4B. |
Detailed Description
Includes.
Definition at line 17 of file bmm150.h.
Member Function Documentation
int16_t compensate_x | ( | int16_t | mag_data_z, |
uint16_t | data_rhall | ||
) |
This internal API is used to obtain the compensated magnetometer x axis data(micro-tesla) in float.
Definition at line 91 of file bmm150.cpp.
int16_t compensate_y | ( | int16_t | mag_data_z, |
uint16_t | data_rhall | ||
) |
This internal API is used to obtain the compensated magnetometer Y axis data(micro-tesla) in int.
Definition at line 146 of file bmm150.cpp.
int16_t compensate_z | ( | int16_t | mag_data_z, |
uint16_t | data_rhall | ||
) |
This internal API is used to obtain the compensated magnetometer Z axis data(micro-tesla) in int.
Definition at line 198 of file bmm150.cpp.
int8_t initialize | ( | void | ) |
initialze device
Definition at line 20 of file bmm150.cpp.
void read_mag_data | ( | ) |
Read magnetometer data.
Definition at line 48 of file bmm150.cpp.
void read_trim_registers | ( | ) |
This internal API reads the trim registers of the sensor and stores the trim values in the "trim_data" of device structure.
Definition at line 407 of file bmm150.cpp.
void set_odr | ( | struct bmm150_settings | settings ) |
This internal API is used to set the output data rate of the sensor.
Definition at line 312 of file bmm150.cpp.
void set_odr_xyz_rep | ( | struct bmm150_settings | settings ) |
Self test functionality.
This internal API sets the preset mode ODR and repetition settings.
Definition at line 278 of file bmm150.cpp.
void set_op_mode | ( | uint8_t | op_mode ) |
Set power mode.
Definition at line 367 of file bmm150.cpp.
void set_power_control_bit | ( | uint8_t | pwrcntrl_bit ) |
This internal API sets/resets the power control bit of 0x4B register.
Definition at line 446 of file bmm150.cpp.
void set_preset_mode | ( | uint8_t | mode ) |
Set preset mode mode.
void set_presetmode | ( | uint8_t | preset_mode ) |
This API is used to set the preset mode of the sensor.
Definition at line 239 of file bmm150.cpp.
void set_xy_rep | ( | struct bmm150_settings | settings ) |
This internal API sets the xy repetition value in the 0x51 register.
Definition at line 287 of file bmm150.cpp.
void set_z_rep | ( | struct bmm150_settings | settings ) |
This internal API sets the z repetition value in the 0x52 register.
Definition at line 294 of file bmm150.cpp.
void soft_reset | ( | ) |
This API is used to perform soft-reset of the sensor where all the registers are reset to their default values except 0x4B.
Definition at line 301 of file bmm150.cpp.
void suspend_to_sleep_mode | ( | void | ) |
This internal API sets the device from suspend to sleep mode by setting the power control bit to '1' of 0x4B register.
Definition at line 400 of file bmm150.cpp.
void write_op_mode | ( | uint8_t | op_mode ) |
This internal API writes the op_mode value in the Opmode bits (bits 1 and 2) of 0x4C register.
Definition at line 438 of file bmm150.cpp.
Generated on Sat Jul 16 2022 16:40:58 by
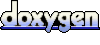