wallbot control Library
wallbotble Class Reference
wallbot ble control class More...
#include <wallbotble.h>
Public Member Functions | |
wallbotble () | |
Create the wallbot object connected to the default pins. | |
void | f_sensor_calibrate (void) |
Sensor calibrate. | |
void | left_motor (float duty) |
Directly control the speed and direction of the left motor. | |
void | right_motor (float duty) |
Directly control the speed and direction of the right motor. | |
void | forward (float duty) |
Drive both motors forward as the same speed. | |
void | backward (float duty) |
Drive both motors backward as the same speed. | |
void | left_turn (float duty) |
Drive left motor backwards and right motor forwards at the same speed to turn on the spot. | |
void | right_turn (float duty) |
Drive left motor forward and right motor backwards at the same speed to turn on the spot. | |
void | stop (void) |
Stop both motors. | |
short | GetLinePosition (void) |
ラインの推定値を返す。 左端-1500~中央0~右端1500 一定以上の検出がない場合は前回値から右端、左端と判定 | |
int | GetSw (void) |
Get switch . | |
void | set_led (char bit) |
Set status led . | |
void | set_led1 (char bit) |
Set led1 . | |
void | set_led2 (char bit) |
Set led2 . |
Detailed Description
wallbot ble control class
Example:
// Drive the wwallbot forward, turn left, back, turn right, at half speed for half a second #include "mbed.h" #include "wallbotble.h" wallbotble wb; int main() { wb.sensor_calibrate(); while(!wb.GetSw()) { wb.set_led(wb.GetLinePosition()); } wb.forward(1.0); wait (1.0); wb.left(1.0); wait (1.0); wb.backward(1.0); wait (1.0); wb.right(1.0); wait (1.0); wb.stop(); while(1); }
Definition at line 78 of file wallbotble.h.
Constructor & Destructor Documentation
wallbotble | ( | ) |
Create the wallbot object connected to the default pins.
Definition at line 31 of file wallbotble.cpp.
Member Function Documentation
void backward | ( | float | duty ) |
Drive both motors backward as the same speed.
- Parameters:
-
speed A normalised number 0 - 1.0 represents the full range.
Definition at line 211 of file wallbotble.cpp.
void f_sensor_calibrate | ( | void | ) |
Sensor calibrate.
センサ値を10回読み込み、最大値と最小値を取得する センサ毎にライン→ライン外で2回この関数を呼ぶイメージ
Definition at line 269 of file wallbotble.cpp.
void forward | ( | float | duty ) |
Drive both motors forward as the same speed.
- Parameters:
-
speed A normalised number 0 - 1.0 represents the full range.
Definition at line 206 of file wallbotble.cpp.
short GetLinePosition | ( | void | ) |
ラインの推定値を返す。 左端-1500~中央0~右端1500 一定以上の検出がない場合は前回値から右端、左端と判定
Definition at line 307 of file wallbotble.cpp.
int GetSw | ( | void | ) |
void left_motor | ( | float | duty ) |
Directly control the speed and direction of the left motor.
- Parameters:
-
speed A normalised number -1.0 - 1.0 represents the full range.
Definition at line 198 of file wallbotble.cpp.
void left_turn | ( | float | duty ) |
Drive left motor backwards and right motor forwards at the same speed to turn on the spot.
- Parameters:
-
speed A normalised number 0 - 1.0 represents the full range.
Definition at line 216 of file wallbotble.cpp.
void right_motor | ( | float | duty ) |
Directly control the speed and direction of the right motor.
- Parameters:
-
speed A normalised number -1.0 - 1.0 represents the full range.
Definition at line 202 of file wallbotble.cpp.
void right_turn | ( | float | duty ) |
Drive left motor forward and right motor backwards at the same speed to turn on the spot.
- Parameters:
-
speed A normalised number 0 - 1.0 represents the full range.
Definition at line 221 of file wallbotble.cpp.
void set_led | ( | char | bit ) |
Set status led .
- Parameters:
-
led (bit0:LEFT bit2:RIGHT)
Definition at line 231 of file wallbotble.cpp.
void set_led1 | ( | char | bit ) |
void set_led2 | ( | char | bit ) |
void stop | ( | void | ) |
Stop both motors.
Definition at line 226 of file wallbotble.cpp.
Generated on Mon Aug 22 2022 09:01:58 by
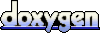