
Wallbot_CaaS
Dependencies: MPU6050 mbed PID
Fork of BLE_MPU6050_test6_challenge_sb by
UUID.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <string.h> 00018 00019 #include "UUID.h" 00020 00021 UUID::UUID(ShortUUIDBytes_t shortUUID) : type(UUID_TYPE_SHORT), baseUUID(), shortUUID(shortUUID) { 00022 /* empty */ 00023 } 00024 00025 /**************************************************************************/ 00026 /*! 00027 @brief Creates a new 128-bit UUID 00028 00029 @note The UUID is a unique 128-bit (16 byte) ID used to identify 00030 different service or characteristics on the BLE device. 00031 00032 @note When creating a UUID, the constructor will check if all bytes 00033 except bytes 2/3 are equal to 0. If only bytes 2/3 have a 00034 value, the UUID will be treated as a short/BLE UUID, and the 00035 .type field will be set to UUID::UUID_TYPE_SHORT. If any 00036 of the bytes outside byte 2/3 have a non-zero value, the UUID 00037 will be considered a 128-bit ID, and .type will be assigned 00038 as UUID::UUID_TYPE_LONG. 00039 00040 @param[in] uuid_base 00041 The 128-bit (16-byte) UUID value. For 128-bit values, 00042 assign all 16 bytes. For 16-bit values, assign the 00043 16-bits to byte 2 and 3, and leave the rest of the bytes 00044 as 0. 00045 00046 @section EXAMPLE 00047 00048 @code 00049 00050 // Create a short UUID (0x180F) 00051 uint8_t shortID[16] = { 0, 0, 0x0F, 0x18, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; 00052 UUID ble_uuid = UUID(shortID); 00053 // ble_uuid.type = UUID_TYPE_SHORT 00054 // ble_uuid.value = 0x180F 00055 00056 // Creeate a long UUID 00057 uint8_t longID[16] = { 0x00, 0x11, 0x22, 0x33, 00058 0x44, 0x55, 0x66, 0x77, 00059 0x88, 0x99, 0xAA, 0xBB, 00060 0xCC, 0xDD, 0xEE, 0xFF }; 00061 UUID custom_uuid = UUID(longID); 00062 // custom_uuid.type = UUID_TYPE_LONG 00063 // custom_uuid.value = 0x3322 00064 // custom_uuid.base = 00 11 22 33 44 55 66 77 88 99 AA BB CC DD EE FF 00065 00066 @endcode 00067 */ 00068 /**************************************************************************/ 00069 UUID::UUID(const LongUUIDBytes_t longUUID) : type(UUID_TYPE_SHORT), baseUUID(), shortUUID(0) 00070 { 00071 memcpy(baseUUID, longUUID, LENGTH_OF_LONG_UUID); 00072 shortUUID = (uint16_t)((longUUID[2] << 8) | (longUUID[3])); 00073 00074 /* Check if this is a short of a long UUID */ 00075 unsigned index; 00076 for (index = 0; index < LENGTH_OF_LONG_UUID; index++) { 00077 if ((index == 2) || (index == 3)) { 00078 continue; /* we should not consider bytes 2 and 3 because that's 00079 * where the 16-bit relative UUID is placed. */ 00080 } 00081 00082 if (baseUUID[index] != 0) { 00083 type = UUID_TYPE_LONG; 00084 00085 /* zero out the 16-bit part in the base; this will help equate long 00086 * UUIDs when they differ only in this 16-bit relative part.*/ 00087 baseUUID[2] = 0; 00088 baseUUID[3] = 0; 00089 00090 return; 00091 } 00092 } 00093 } 00094
Generated on Tue Jul 12 2022 18:33:09 by
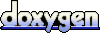