
Wallbot_CaaS
Dependencies: MPU6050 mbed PID
Fork of BLE_MPU6050_test6_challenge_sb by
GattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVER_H__ 00018 #define __GATT_SERVER_H__ 00019 00020 #include "GattService.h" 00021 #include "GattServerEvents.h" 00022 #include "GattCharacteristicWriteCBParams.h" 00023 #include "CallChainOfFunctionPointersWithContext.h" 00024 00025 class GattServer { 00026 public: 00027 /* Event callback handlers. */ 00028 typedef void (*EventCallback_t)(uint16_t attributeHandle); 00029 typedef void (*ServerEventCallback_t)(void); /**< independent of any particular attribute */ 00030 typedef void (*ServerEventCallbackWithCount_t)(unsigned count); /**< independent of any particular attribute */ 00031 00032 protected: 00033 GattServer() : serviceCount(0), characteristicCount(0), onDataSent(NULL), onDataWritten(), onUpdatesEnabled(NULL), onUpdatesDisabled(NULL), onConfirmationReceived(NULL) { 00034 /* empty */ 00035 } 00036 00037 friend class BLEDevice; 00038 private: 00039 /* These functions must be defined in the sub-class */ 00040 virtual ble_error_t addService(GattService &) = 0; 00041 virtual ble_error_t readValue(uint16_t handle, uint8_t buffer[], uint16_t *const lengthP) = 0; 00042 virtual ble_error_t updateValue(uint16_t, uint8_t[], uint16_t, bool localOnly = false) = 0; 00043 virtual ble_error_t initializeGATTDatabase(void) = 0; 00044 00045 // ToDo: For updateValue, check the CCCD to see if the value we are 00046 // updating has the notify or indicate bits sent, and if BOTH are set 00047 // be sure to call sd_ble_gatts_hvx() twice with notify then indicate! 00048 // Strange use case, but valid and must be covered! 00049 00050 void setOnDataSent(ServerEventCallbackWithCount_t callback) {onDataSent = callback;} 00051 void setOnDataWritten(void (*callback)(const GattCharacteristicWriteCBParams *eventDataP)) {onDataWritten.add(callback);} 00052 template <typename T> 00053 void setOnDataWritten(T *objPtr, void (T::*memberPtr)(const GattCharacteristicWriteCBParams *context)) { 00054 onDataWritten.add(objPtr, memberPtr); 00055 } 00056 void setOnUpdatesEnabled(EventCallback_t callback) {onUpdatesEnabled = callback;} 00057 void setOnUpdatesDisabled(EventCallback_t callback) {onUpdatesDisabled = callback;} 00058 void setOnConfirmationReceived(EventCallback_t callback) {onConfirmationReceived = callback;} 00059 00060 protected: 00061 void handleDataWrittenEvent(const GattCharacteristicWriteCBParams *params) { 00062 if (onDataWritten.hasCallbacksAttached()) { 00063 onDataWritten.call(params); 00064 } 00065 } 00066 00067 void handleEvent(GattServerEvents::gattEvent_e type, uint16_t charHandle) { 00068 switch (type) { 00069 case GattServerEvents::GATT_EVENT_UPDATES_ENABLED: 00070 if (onUpdatesEnabled) { 00071 onUpdatesEnabled(charHandle); 00072 } 00073 break; 00074 case GattServerEvents::GATT_EVENT_UPDATES_DISABLED: 00075 if (onUpdatesDisabled) { 00076 onUpdatesDisabled(charHandle); 00077 } 00078 break; 00079 case GattServerEvents::GATT_EVENT_CONFIRMATION_RECEIVED: 00080 if (onConfirmationReceived) { 00081 onConfirmationReceived(charHandle); 00082 } 00083 break; 00084 } 00085 } 00086 00087 void handleDataSentEvent(unsigned count) { 00088 if (onDataSent) { 00089 onDataSent(count); 00090 } 00091 } 00092 00093 protected: 00094 uint8_t serviceCount; 00095 uint8_t characteristicCount; 00096 00097 private: 00098 ServerEventCallbackWithCount_t onDataSent; 00099 CallChainOfFunctionPointersWithContext<const GattCharacteristicWriteCBParams *> onDataWritten; 00100 EventCallback_t onUpdatesEnabled; 00101 EventCallback_t onUpdatesDisabled; 00102 EventCallback_t onConfirmationReceived; 00103 00104 private: 00105 /* disallow copy and assignment */ 00106 GattServer(const GattServer &); 00107 GattServer& operator=(const GattServer &); 00108 }; 00109 00110 #endif // ifndef __GATT_SERVER_H__
Generated on Tue Jul 12 2022 18:33:09 by
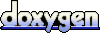