
The present code executes a counter that checks current state and determines the next state.
Fork of 2645_FSM_Counter by
main.cpp
00001 /* 00002 00003 Task1_FSM_Counter 00004 00005 Sample code from ELEC2645 Week 16 Lab 00006 00007 Demonstrates how to implement a simple FSM counter 00008 00009 (c) Bonny Ngangu, University of Leeds, Jan 2016 00010 00011 */ 00012 00013 #include "mbed.h" 00014 00015 // K64F on-board 4 LEDs 00016 DigitalOut r_led(LED_RED); 00017 DigitalOut g_led(LED_GREEN); 00018 DigitalOut b_led(LED_BLUE); 00019 DigitalOut y_led(LED_BLUE); 00020 00021 // K64F on-board switches 00022 InterruptIn sw2(SW2); 00023 InterruptIn sw3(SW3); 00024 00025 // LEDs to display counter output 00026 // connect up external LEDs to these pins with appropriate current-limiting resistor 00027 BusOut output(PTB2,PTB3,PTB10,PTB11); 00028 00029 // array of states in the FSM, each element is the output of the counter 00030 int fsm[4] = {8,4,2,1}; //reversed order counter going down 00031 00032 // function prototypes 00033 // error function hangs flashing an LED 00034 void error(); 00035 // set-up the on-board LEDs and switches 00036 void init_K64F(); 00037 00038 int main() 00039 { 00040 init_K64F(); 00041 // set inital state 00042 int state = 0; 00043 00044 while(1) { // loop forever 00045 00046 output = fsm[state]; // output current state 00047 00048 // check which state we are in and see which the next state should be 00049 switch(state) { 00050 case 0://0 00051 state = 1;//1 00052 break; 00053 case 1://1 00054 state = 2;//2 00055 break; 00056 case 2://2 00057 state = 3;//3 00058 break; 00059 case 3://3 00060 state = 0;//0 00061 break; 00062 default: 00063 error(); //invalid state - call error routine 00064 // or could jump to starting state i.e. state = 0 00065 break; 00066 } 00067 00068 wait(0.2); // small delay 00069 00070 } 00071 } 00072 00073 void init_K64F() 00074 { 00075 // on-board LEDs are active-low, so set pin high to turn them off. 00076 r_led = 1; 00077 g_led = 1; 00078 b_led = 1; 00079 y_led = 1; 00080 00081 // since the on-board switches have external pull-ups, we should disable the internal pull-down 00082 // resistors that are enabled by default using InterruptIn 00083 sw2.mode(PullNone); 00084 sw3.mode(PullNone); 00085 00086 } 00087 00088 void error() 00089 { 00090 while(1) { // if error, hang while flashing error message 00091 r_led = 0; 00092 wait(0.2); 00093 r_led = 1; 00094 wait(0.2); 00095 } 00096 }
Generated on Tue Jul 12 2022 19:59:25 by
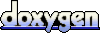