Starter project for Bluetooth SIG hands-on training course
Embed:
(wiki syntax)
Show/hide line numbers
Animator.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO Animation SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #include "Animator.h" 00027 #include "MicroBit.h" 00028 #include <math.h> 00029 #include <mbed.h> 00030 00031 MicroBitImage pattern(5,5); 00032 00033 Serial pc(USBTX, USBRX); 00034 00035 int inx=0; 00036 int delta = 1; 00037 int pixel_value=255; 00038 int flash_state=1; 00039 int num_rnd_pixels = 4; 00040 00041 int ripple_pixels[4][25] = { 00042 {0,0,0,0,0, 0,0,0,0,0, 0,0,0,0,0, 0,0,0,0,0, 0,0,0,0,0}, 00043 {0,0,0,0,0, 0,0,0,0,0, 0,0,1,0,0, 0,0,0,0,0, 0,0,0,0,0}, 00044 {0,0,0,0,0, 0,1,1,1,0, 0,1,0,1,0, 0,1,1,1,0, 0,0,0,0,0}, 00045 {1,1,1,1,1, 1,0,0,0,1, 1,0,0,0,1, 1,0,0,0,1, 1,1,1,1,1} 00046 }; 00047 00048 int spiral_pixels[25] = { 00049 12, 13, 8, 7, 6, 11, 16, 17, 18, 19, 14, 9, 4, 3, 2, 1, 0, 5, 10, 15, 20, 21, 22, 23, 24 00050 }; 00051 00052 Animator::Animator(MicroBitDisplay &_display) : 00053 display(_display), sleep_time(500), playing(0), current_animation(1) 00054 { 00055 } 00056 00057 void Animator::setAnimationType(uint16_t animation) 00058 { 00059 pc.printf("Animator::setAnimationType %d\n",animation); 00060 if (animation <= NUMBER_OF_ANIMATIONS) { 00061 current_animation = animation; 00062 pattern.clear(); 00063 display.clear(); 00064 switch (current_animation) { 00065 case ANIMATION_TYPE_FLASH: 00066 pc.printf("Animator::setAnimationType FLASH\n"); 00067 sleep_time = 500; 00068 break; 00069 case ANIMATION_TYPE_RIPPLE: 00070 pc.printf("Animator::setAnimationType RIPPLE\n"); 00071 sleep_time = 100; 00072 inx=0; 00073 delta = 1; 00074 break; 00075 case ANIMATION_TYPE_SPIRAL: 00076 pc.printf("Animator::setAnimationType SPIRAL\n"); 00077 sleep_time = 50; 00078 break; 00079 default: 00080 pc.printf("Animator::setAnimationType default=FLASH\n"); 00081 current_animation = ANIMATION_TYPE_FLASH; 00082 sleep_time = 500; 00083 break; } 00084 } 00085 } 00086 00087 void Animator::start() 00088 { 00089 pc.printf("Animator::start\n"); 00090 playing = 1; 00091 MicroBitEvent(ANIMATION_STATUS_EVENT,ANIMATION_STATUS_BUSY); 00092 } 00093 00094 void Animator::stop() 00095 { 00096 pc.printf("Animator::stop\n"); 00097 playing = 0; 00098 display.clear(); 00099 MicroBitEvent(ANIMATION_STATUS_EVENT,ANIMATION_STATUS_FREE); 00100 } 00101 00102 void Animator::faster() 00103 { 00104 if (sleep_time > 0) { 00105 sleep_time = sleep_time - ANIMATION_SPEED_DELTA; 00106 } 00107 } 00108 00109 void Animator::slower() 00110 { 00111 sleep_time = sleep_time + ANIMATION_SPEED_DELTA; 00112 } 00113 00114 void Animator::ripple() { 00115 int i=0; 00116 for (i=0;i<25;i++) { 00117 pattern.setPixelValue(i % 5 , floor(static_cast<double>(i / 5)), ripple_pixels[inx][i]); 00118 } 00119 display.image.paste(pattern); 00120 inx = inx + delta; 00121 if (inx == 3 || inx == 0) { 00122 delta = delta * -1; 00123 } 00124 } 00125 00126 void Animator::flash() { 00127 int i; 00128 for (i=0;i<25;i++) { 00129 pattern.setPixelValue(i % 5 , floor(static_cast<double>(i / 5)), flash_state * 255); 00130 } 00131 display.image.paste(pattern); 00132 flash_state = !flash_state; 00133 } 00134 00135 void Animator::spiral() { 00136 int x = spiral_pixels[inx] % 5; 00137 int y = floor(static_cast<double>(spiral_pixels[inx] / 5)); 00138 pattern.setPixelValue(x,y, pixel_value); 00139 display.image.paste(pattern); 00140 inx = inx + delta; 00141 if (inx == 25 || inx == -1) { 00142 delta = delta * -1; 00143 inx = inx + delta; 00144 if (pixel_value == 255) { 00145 pixel_value = 0; 00146 } else { 00147 pixel_value = 255; 00148 } 00149 } 00150 }
Generated on Sun Jul 24 2022 05:43:06 by
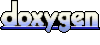