
VNG bot battle
Dependencies: BLE_API mbed nRF51822
MotorController.cpp
00001 /* 00002 * MotorController.cpp 00003 * 00004 * Created on: Aug 23, 2016 00005 * Author: tanpt 00006 */ 00007 00008 #include "MotorController.h" 00009 00010 DigitalOut MotorController::motorLeftDirection_(p25); 00011 PwmOut MotorController::motorLeftSpeed_(p23); 00012 DigitalOut MotorController::motorRightDirection_(p28); 00013 PwmOut MotorController::motorRightSpeed_(p24); 00014 00015 DigitalOut MotorController::motorBoostSpeed_(p20); 00016 00017 MotorController::MotorController() { 00018 motorRightDirection_ = DIRECTION_FORWARD; 00019 motorRightSpeed_.period_ms(1000); 00020 motorLeftDirection_ = DIRECTION_FORWARD; 00021 motorLeftSpeed_.period_ms(1000); 00022 } 00023 00024 MotorController::~MotorController() { 00025 00026 } 00027 00028 int8_t MotorController::setMotorRight(Direction direction, uint16_t speed) { 00029 motorRightDirection_ = direction; 00030 motorRightSpeed_.pulsewidth_ms(speed); 00031 return 0; 00032 } 00033 00034 int8_t MotorController::setMotorLeft(Direction direction, uint16_t speed) { 00035 motorLeftDirection_ = direction; 00036 motorLeftSpeed_.pulsewidth_ms(speed); 00037 return 0; 00038 } 00039 00040 int8_t MotorController::moveForward(uint16_t speed) { 00041 motorRightDirection_ = DIRECTION_FORWARD; 00042 motorRightSpeed_.pulsewidth_ms(speed); 00043 motorLeftDirection_ = DIRECTION_FORWARD; 00044 motorLeftSpeed_.pulsewidth_ms(speed); 00045 return 0; 00046 } 00047 00048 int8_t MotorController::moveBackward(uint16_t speed) { 00049 motorRightDirection_ = DIRECTION_BACKWARD; 00050 motorRightSpeed_.pulsewidth_ms(speed); 00051 motorLeftDirection_ = DIRECTION_BACKWARD; 00052 motorLeftSpeed_.pulsewidth_ms(speed); 00053 return 0; 00054 } 00055 00056 int8_t MotorController::turnRight(uint16_t speed) { 00057 motorRightDirection_ = DIRECTION_BACKWARD; 00058 motorRightSpeed_.pulsewidth_ms(speed); 00059 motorLeftDirection_ = DIRECTION_FORWARD; 00060 motorLeftSpeed_.pulsewidth_ms(speed); 00061 return 0; 00062 } 00063 00064 int8_t MotorController::turnLeft(uint16_t speed) { 00065 motorRightDirection_ = DIRECTION_FORWARD; 00066 motorRightSpeed_.pulsewidth_ms(speed); 00067 motorLeftDirection_ = DIRECTION_BACKWARD; 00068 motorLeftSpeed_.pulsewidth_ms(speed); 00069 return 0; 00070 } 00071 00072 int8_t MotorController::moveBackRight(uint16_t speed) { 00073 motorRightDirection_ = DIRECTION_BACKWARD; 00074 motorRightSpeed_.pulsewidth_ms(speed); 00075 motorLeftDirection_ = DIRECTION_BACKWARD; 00076 motorLeftSpeed_.pulsewidth_ms(speed / 3); 00077 return 0; 00078 00079 } 00080 00081 int8_t MotorController::moveBackLeft(uint16_t speed) { 00082 motorRightDirection_ = DIRECTION_BACKWARD; 00083 motorRightSpeed_.pulsewidth_ms(speed / 3); 00084 motorLeftDirection_ = DIRECTION_BACKWARD; 00085 motorLeftSpeed_.pulsewidth_ms(speed); 00086 return 0; 00087 } 00088 00089 int8_t MotorController::setBoost(BoostState state) { 00090 motorBoostSpeed_ = state; 00091 } 00092
Generated on Fri Aug 5 2022 18:35:57 by
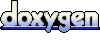