
uIP 1.0 based webserver for LPC1114 + ENC28J60
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 extern "C" { 00004 #include "uip.h" 00005 #include "uip_arp.h" 00006 } 00007 #include "tapdev.h" 00008 00009 #define BUF ((struct uip_eth_hdr *)&uip_buf[0]) 00010 00011 #ifndef NULL 00012 #define NULL (void *)0 00013 #endif /* NULL */ 00014 00015 //DigitalOut myled(LED1); 00016 00017 /* Ethernet Pin configuration 00018 => /dev-enc28j60/tapdev.cpp 00019 */ 00020 00021 //Serial pc(USBTX, USBRX); // tx, rx 00022 //RawSerial pc(USBTX, USBRX); // tx, rx 00023 00024 int main() { 00025 //pc.baud(9600); 00026 //pc.baud(115200); 00027 00028 wait_ms(100); 00029 00030 int i; 00031 uip_ipaddr_t ipaddr; 00032 Timer periodic_timer, arp_timer; 00033 00034 periodic_timer.start(); 00035 arp_timer.start(); 00036 00037 /* Initialize the device driver. */ 00038 printf("tapdev_init()\n"); 00039 tapdev_init(); 00040 00041 /* Initialize the uIP TCP/IP stack. */ 00042 printf("uip_init()\n"); 00043 uip_init(); 00044 00045 struct uip_eth_addr mac = {UIP_ETHADDR0, UIP_ETHADDR1, UIP_ETHADDR2, UIP_ETHADDR3, UIP_ETHADDR4, UIP_ETHADDR5}; 00046 uip_setethaddr(mac); 00047 00048 uip_ipaddr(&ipaddr, 192,168,0,12); 00049 uip_sethostaddr(&ipaddr); 00050 uip_ipaddr(&ipaddr, 192,168,0,1); 00051 uip_setdraddr(&ipaddr); 00052 uip_ipaddr(&ipaddr, 255,255,255,0); 00053 uip_setnetmask(&ipaddr); 00054 00055 printf("httpd_init()\n"); 00056 httpd_init(); 00057 00058 /* telnetd_init();*/ 00059 00060 /* hello_world_init();*/ 00061 00062 /* { 00063 u8_t mac[6] = {1,2,3,4,5,6}; 00064 dhcpc_init(&mac, 6); 00065 }*/ 00066 00067 /*uip_ipaddr(ipaddr, 127,0,0,1); 00068 smtp_configure("localhost", ipaddr); 00069 SMTP_SEND("adam@sics.se", NULL, "uip-testing@example.com", 00070 "Testing SMTP from uIP", 00071 "Test message sent by uIP\r\n");*/ 00072 00073 /* 00074 webclient_init(); 00075 resolv_init(); 00076 uip_ipaddr(ipaddr, 195,54,122,204); 00077 resolv_conf(ipaddr); 00078 resolv_query("www.sics.se");*/ 00079 00080 00081 printf("uIP Start.\n"); 00082 00083 while(1) { 00084 uip_len = tapdev_read(); 00085 if(uip_len > 0) { 00086 //printf("recv = %d\n", uip_len); 00087 00088 if(BUF->type == UIP_HTONS(UIP_ETHTYPE_IP)) { 00089 uip_arp_ipin(); 00090 uip_input(); 00091 /* If the above function invocation resulted in data that 00092 should be sent out on the network, the global variable 00093 uip_len is set to a value > 0. */ 00094 if(uip_len > 0) { 00095 uip_arp_out(); 00096 tapdev_send(); 00097 } 00098 } else if(BUF->type == UIP_HTONS(UIP_ETHTYPE_ARP)) { 00099 uip_arp_arpin(); 00100 /* If the above function invocation resulted in data that 00101 should be sent out on the network, the global variable 00102 uip_len is set to a value > 0. */ 00103 if(uip_len > 0) { 00104 tapdev_send(); 00105 } 00106 } 00107 00108 } else if(periodic_timer.read_ms() >= 500) { 00109 periodic_timer.reset(); 00110 //printf("periodic_timer tick\n"); 00111 for(i = 0; i < UIP_CONNS; i++) { 00112 uip_periodic(i); 00113 /* If the above function invocation resulted in data that 00114 should be sent out on the network, the global variable 00115 uip_len is set to a value > 0. */ 00116 if(uip_len > 0) { 00117 uip_arp_out(); 00118 tapdev_send(); 00119 } 00120 } 00121 00122 #if UIP_UDP 00123 for(i = 0; i < UIP_UDP_CONNS; i++) { 00124 uip_udp_periodic(i); 00125 /* If the above function invocation resulted in data that 00126 should be sent out on the network, the global variable 00127 uip_len is set to a value > 0. */ 00128 if(uip_len > 0) { 00129 uip_arp_out(); 00130 tapdev_send(); 00131 } 00132 } 00133 #endif /* UIP_UDP */ 00134 00135 /* Call the ARP timer function every 10 seconds. */ 00136 if(arp_timer.read_ms() >= 10000) { 00137 arp_timer.reset(); 00138 //printf("arp_timer tick\n"); 00139 uip_arp_timer(); 00140 } 00141 } 00142 } 00143 //return 0; 00144 } 00145 /*---------------------------------------------------------------------------*/ 00146 void 00147 uip_log(char *m) 00148 { 00149 printf("uIP log message: %s\n", m); 00150 } 00151 /*---------------------------------------------------------------------------*/
Generated on Tue Jul 12 2022 12:52:12 by
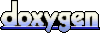