
uIP 1.0 based webserver for LPC1114 + ENC28J60
{@ More...
Data Structures | |
union | uip_ip4addr_t |
Representation of an IP address. More... | |
struct | uip_802154_shortaddr |
16 bit 802.15.4 address More... | |
struct | uip_802154_longaddr |
64 bit 802.15.4 address More... | |
struct | uip_80211_addr |
802.11 address More... | |
struct | uip_eth_addr |
802.3 address More... | |
struct | uip_conn |
Representation of a uIP TCP connection. More... | |
struct | uip_udp_conn |
Representation of a uIP UDP connection. More... | |
struct | uip_stats |
The structure holding the TCP/IP statistics that are gathered if UIP_STATISTICS is set to 1. More... | |
Modules | |
uIP packet forwarding | |
uIP configuration functions | |
The uIP configuration functions are used for setting run-time parameters in uIP such as IP addresses. | |
uIP initialization functions | |
The uIP initialization functions are used for booting uIP. | |
uIP device driver functions | |
These functions are used by a network device driver for interacting with uIP. | |
uIP application functions | |
Functions used by an application running of top of uIP. | |
uIP conversion functions | |
These functions can be used for converting between different data formats used by uIP. | |
Variables used in uIP device drivers | |
uIP has a few global variables that are used in device drivers for uIP. | |
uIP Address Resolution Protocol | |
The Address Resolution Protocol ARP is used for mapping between IP addresses and link level addresses such as the Ethernet MAC addresses. | |
Architecture specific uIP functions | |
The functions in the architecture specific module implement the IP check sum and 32-bit additions. | |
Files | |
file | uip.c |
The uIP TCP/IP stack code. | |
file | uip.h |
Header file for the uIP TCP/IP stack. | |
Typedefs | |
typedef union uip_ip4addr_t | uip_ip4addr_t |
Representation of an IP address. | |
typedef struct uip_802154_shortaddr | uip_802154_shortaddr |
16 bit 802.15.4 address | |
typedef struct uip_802154_longaddr | uip_802154_longaddr |
64 bit 802.15.4 address | |
typedef struct uip_80211_addr | uip_80211_addr |
802.11 address | |
typedef struct uip_eth_addr | uip_eth_addr |
802.3 address | |
typedef uip_802154_longaddr | uip_lladdr_t |
802.15.4 address | |
Functions | |
void | uip_setipid (uint16_t id) |
uIP initialization function. | |
uint16_t | uip_chksum (uint16_t *buf, uint16_t len) |
Calculate the Internet checksum over a buffer. | |
uint16_t | uip_ipchksum (void) |
Calculate the IP header checksum of the packet header in uip_buf. | |
uint16_t | uip_icmp6chksum (void) |
Calculate the ICMP checksum of the packet in uip_buf. | |
uint16_t | uip_tcpchksum (void) |
Calculate the TCP checksum of the packet in uip_buf and uip_appdata. | |
uint16_t | uip_udpchksum (void) |
Calculate the UDP checksum of the packet in uip_buf and uip_appdata. | |
void | uip_init (void) |
uIP initialization function. | |
struct uip_conn * | uip_connect (uip_ipaddr_t *ripaddr, uint16_t rport) |
Connect to a remote host using TCP. | |
struct uip_udp_conn * | uip_udp_new (const uip_ipaddr_t *ripaddr, uint16_t rport) |
Set up a new UDP connection. | |
void | uip_unlisten (uint16_t port) |
Stop listening to the specified port. | |
void | uip_listen (uint16_t port) |
Start listening to the specified port. | |
void | uip_process (uint8_t flag) |
process the options within a hop by hop or destination option header | |
uint16_t | uip_htons (uint16_t val) |
Convert a 16-bit quantity from host byte order to network byte order. | |
void | uip_send (const void *data, int len) |
Send data on the current connection. | |
Variables | |
void * | uip_appdata |
Pointer to the application data in the packet buffer. | |
uint16_t | uip_len |
The length of the packet in the uip_buf buffer. | |
struct uip_conn * | uip_conn |
Pointer to the current TCP connection. | |
struct uip_udp_conn * | uip_udp_conn |
The current UDP connection. | |
uint8_t | uip_acc32 [4] |
4-byte array used for the 32-bit sequence number calculations. | |
struct uip_stats | uip_stat |
The uIP TCP/IP statistics. | |
CCIF void * | uip_appdata |
Pointer to the application data in the packet buffer. | |
CCIF struct uip_conn * | uip_conn |
Pointer to the current TCP connection. | |
struct uip_udp_conn * | uip_udp_conn |
The current UDP connection. | |
struct uip_stats | uip_stat |
The uIP TCP/IP statistics. |
Detailed Description
{@
Typedef Documentation
typedef struct uip_80211_addr uip_80211_addr |
802.11 address
typedef struct uip_802154_longaddr uip_802154_longaddr |
64 bit 802.15.4 address
typedef struct uip_802154_shortaddr uip_802154_shortaddr |
16 bit 802.15.4 address
typedef struct uip_eth_addr uip_eth_addr |
802.3 address
typedef union uip_ip4addr_t uip_ip4addr_t |
Representation of an IP address.
typedef uip_eth_addr uip_lladdr_t |
Function Documentation
uint16_t uip_chksum | ( | uint16_t * | buf, |
uint16_t | len | ||
) |
Calculate the Internet checksum over a buffer.
The Internet checksum is the one's complement of the one's complement sum of all 16-bit words in the buffer.
See RFC1071.
- Parameters:
-
buf A pointer to the buffer over which the checksum is to be computed. len The length of the buffer over which the checksum is to be computed.
- Returns:
- The Internet checksum of the buffer.
struct uip_conn* uip_connect | ( | uip_ipaddr_t * | ripaddr, |
uint16_t | port | ||
) | [read] |
Connect to a remote host using TCP.
This function is used to start a new connection to the specified port on the specified host. It allocates a new connection identifier, sets the connection to the SYN_SENT state and sets the retransmission timer to 0. This will cause a TCP SYN segment to be sent out the next time this connection is periodically processed, which usually is done within 0.5 seconds after the call to uip_connect().
- Note:
- This function is available only if support for active open has been configured by defining UIP_ACTIVE_OPEN to 1 in uipopt.h.
- Since this function requires the port number to be in network byte order, a conversion using UIP_HTONS() or uip_htons() is necessary.
uip_ipaddr_t ipaddr; uip_ipaddr(&ipaddr, 192,168,1,2); uip_connect(&ipaddr, UIP_HTONS(80));
- Parameters:
-
ripaddr The IP address of the remote host. port A 16-bit port number in network byte order.
- Returns:
- A pointer to the uIP connection identifier for the new connection, or NULL if no connection could be allocated.
uint16_t uip_htons | ( | uint16_t | val ) |
uint16_t uip_icmp6chksum | ( | void | ) |
void uip_init | ( | void | ) |
uint16_t uip_ipchksum | ( | void | ) |
void uip_listen | ( | uint16_t | port ) |
Start listening to the specified port.
- Note:
- Since this function expects the port number in network byte order, a conversion using UIP_HTONS() or uip_htons() is necessary.
uip_listen(UIP_HTONS(80));
- Parameters:
-
port A 16-bit port number in network byte order.
void uip_process | ( | uint8_t | flag ) |
void uip_send | ( | const void * | data, |
int | len | ||
) |
Send data on the current connection.
This function is used to send out a single segment of TCP data. Only applications that have been invoked by uIP for event processing can send data.
The amount of data that actually is sent out after a call to this function is determined by the maximum amount of data TCP allows. uIP will automatically crop the data so that only the appropriate amount of data is sent. The function uip_mss() can be used to query uIP for the amount of data that actually will be sent.
- Note:
- This function does not guarantee that the sent data will arrive at the destination. If the data is lost in the network, the application will be invoked with the uip_rexmit() event being set. The application will then have to resend the data using this function.
- Parameters:
-
data A pointer to the data which is to be sent. len The maximum amount of data bytes to be sent.
void uip_setipid | ( | uint16_t | id ) |
uint16_t uip_tcpchksum | ( | void | ) |
Calculate the TCP checksum of the packet in uip_buf and uip_appdata.
The TCP checksum is the Internet checksum of data contents of the TCP segment, and a pseudo-header as defined in RFC793.
- Returns:
- The TCP checksum of the TCP segment in uip_buf and pointed to by uip_appdata.
struct uip_udp_conn* uip_udp_new | ( | const uip_ipaddr_t * | ripaddr, |
uint16_t | rport | ||
) | [read] |
Set up a new UDP connection.
This function sets up a new UDP connection. The function will automatically allocate an unused local port for the new connection. However, another port can be chosen by using the uip_udp_bind() call, after the uip_udp_new() function has been called.
Example:
uip_ipaddr_t addr; struct uip_udp_conn *c; uip_ipaddr(&addr, 192,168,2,1); c = uip_udp_new(&addr, UIP_HTONS(12345)); if(c != NULL) { uip_udp_bind(c, UIP_HTONS(12344)); }
- Parameters:
-
ripaddr The IP address of the remote host. rport The remote port number in network byte order.
- Returns:
- The uip_udp_conn structure for the new connection, or NULL if no connection could be allocated.
uint16_t uip_udpchksum | ( | void | ) |
Calculate the UDP checksum of the packet in uip_buf and uip_appdata.
The UDP checksum is the Internet checksum of data contents of the UDP segment, and a pseudo-header as defined in RFC768.
- Returns:
- The UDP checksum of the UDP segment in uip_buf and pointed to by uip_appdata.
void uip_unlisten | ( | uint16_t | port ) |
Stop listening to the specified port.
- Note:
- Since this function expects the port number in network byte order, a conversion using UIP_HTONS() or uip_htons() is necessary.
uip_unlisten(UIP_HTONS(80));
- Parameters:
-
port A 16-bit port number in network byte order.
Variable Documentation
uint8_t uip_acc32[4] |
CCIF void* uip_appdata |
Pointer to the application data in the packet buffer.
This pointer points to the application data when the application is called. If the application wishes to send data, the application may use this space to write the data into before calling uip_send().
void* uip_appdata |
Pointer to the application data in the packet buffer.
This pointer points to the application data when the application is called. If the application wishes to send data, the application may use this space to write the data into before calling uip_send().
uint16_t uip_len |
The length of the packet in the uip_buf buffer.
The global variable uip_len holds the length of the packet in the uip_buf buffer.
When the network device driver calls the uIP input function, uip_len should be set to the length of the packet in the uip_buf buffer.
When sending packets, the device driver should use the contents of the uip_len variable to determine the length of the outgoing packet.
struct uip_udp_conn* uip_udp_conn |
struct uip_udp_conn* uip_udp_conn |
Generated on Tue Jul 12 2022 12:52:12 by
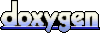